NPM FS Module | How to Install and Use for Node.js
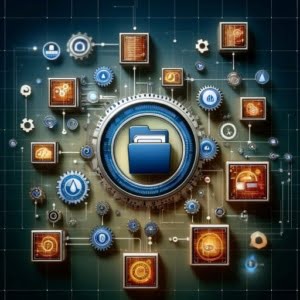
Navigating the file system in Node.js? The fs
module is your map and compass, allowing you to interact with the file system efficiently. In today’s article, we will discuss the file system functionalities provided by fs
.
During software development projects at IOFLOOD, we’ve encountered situations that required streamlined methods for reading and writing files, creating directories, and handling file permissions. While searching for solutions, we explored the fs
module in Node.js. We have gathered together our findings and tips for install and usage, to assist our dedicated server customers that face similar challenges.
This guide will walk you through using the fs
module, from basic file operations to advanced techniques, ensuring you have the tools needed to manage files in your Node.js applications. With practical examples and step-by-step instructions, we aim to make file management in Node.js not just possible, but also intuitive and straightforward.
Let’s embark on this journey together, unlocking the full potential of file management in your Node.js projects.
TL;DR: How Do I Use the fs
Module in Node.js?
The
fs
module in Node.js comes part of node.js core, so you only need to require it in your code with,const fs = require('fs')
. It usually does not require installation, however if there is an error, you can try installing withnpm install fs --save
.
Here’s a quick example to read a file:
const fs = require('fs');
fs.readFile('/path/to/file', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
# Output:
# Contents of the file
In this example, we’ve demonstrated how to read the contents of a file asynchronously using the fs.readFile
method. By specifying the file path and the encoding (in this case, ‘utf8’), we can easily read and output the file’s contents. This is just the beginning of what you can achieve with the fs
module in Node.js.
Ready to dive deeper into file operations? Continue reading for more detailed instructions, examples, and advanced usage.
Table of Contents
Installing and Requiring fs
in Node.js
Before diving into the file system’s vast ocean with Node.js, you need the right equipment. The fs
module, a core Node.js library, is your first tool. To use it, there’s no need for installation via npm, as it comes pre-packaged with Node.js. However, you do need to require it in your project to start working with files. Here’s how you do it:
const fs = require('fs');
With this simple line of code, you’ve unlocked the door to Node.js file system operations.
Reading Files Asynchronously
One of the first tasks you might want to tackle is reading a file. Doing this asynchronously allows your Node.js application to continue running other code while it waits for the file reading operation to complete. Here’s an example of how to read a file asynchronously:
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file:', err);
return;
}
console.log('File content:', data);
});
# Output:
# File content: [Contents of example.txt]
In this example, fs.readFile
takes three arguments: the file path (example.txt
), the encoding (utf8
), and a callback function. The callback function is executed once the file has been read, displaying the file’s contents or an error message.
Writing Files Asynchronously
Creating or updating files is just as crucial as reading them. Here’s how you can write to a file asynchronously, ensuring your app remains responsive:
fs.writeFile('example.txt', 'Hello, npm fs!', (err) => {
if (err) {
console.error('Error writing to the file:', err);
return;
}
console.log('File written successfully');
});
# Output:
# File written successfully
This method, fs.writeFile
, also accepts three arguments: the file path, the data to write, and a callback function. The callback confirms the operation’s success or reports any errors encountered.
Synchronous vs. Asynchronous
While asynchronous file operations are non-blocking and allow for a more fluid user experience, there are times when synchronous methods are more appropriate. For instance, when you need to ensure operations complete in a specific order. However, synchronous operations can halt your application’s execution, which might not be ideal in performance-sensitive environments.
Understanding when to use asynchronous versus synchronous file operations is key to effectively managing your Node.js projects’ file systems. Experiment with both, but remember, the asynchronous path often leads to more responsive applications.
Advanced fs
Techniques
As you become more comfortable with basic file operations in Node.js, it’s time to explore some of the more advanced capabilities of the fs
module. These techniques can significantly enhance the efficiency and performance of your applications.
File Streaming with fs
File streaming is a powerful method for handling large files without consuming excessive memory. Instead of reading or writing files in one go, streaming processes the file in chunks, making it ideal for large data sets.
const fs = require('fs');
let readStream = fs.createReadStream('largefile.txt', 'utf8');
readStream.on('data', function(chunk) {
console.log('Reading chunk:
', chunk);
});
readStream.on('end', function() {
console.log('File read completed.');
});
# Output:
# Reading chunk:
# [First chunk of data]
# Reading chunk:
# [Second chunk of data]
# File read completed.
This example demonstrates how to read a large file in segments. By creating a read stream, we can handle each piece of data as it’s read, reducing the overall memory footprint of our application.
Promises with fs
for Better Async Handling
Promises are a modern approach to asynchronous programming in JavaScript, allowing for cleaner and more manageable code. The fs
module can be promisified to use this pattern, especially with the fs/promises
API.
const fsp = require('fs').promises;
async function readFileAsync() {
try {
const data = await fsp.readFile('example.txt', 'utf8');
console.log('File content:', data);
} catch (err) {
console.error('Error reading the file:', err);
}
}
readFileAsync();
# Output:
# File content: [Contents of example.txt]
In this code snippet, we’ve converted the traditional callback-based fs.readFile
method into a promise-based approach using fs.promises
. This allows us to use async/await
syntax for more readable and efficient error handling and data processing.
Working with Directories
Managing directories is another crucial aspect of file system operations. Creating, reading, and removing directories can be done efficiently with the fs
module.
const fs = require('fs');
// Creating a new directory
fs.mkdir('newDirectory', { recursive: true }, (err) => {
if (err) {
console.error('Error creating directory:', err);
return;
}
console.log('Directory created successfully');
});
# Output:
# Directory created successfully
This example shows how to create a new directory. The { recursive: true }
option allows for the creation of parent directories if they don’t exist, making this method versatile for various use cases.
By mastering these advanced fs
techniques, you’re well on your way to becoming a more proficient Node.js developer. The ability to handle files and directories efficiently opens up a wide range of possibilities for your applications.
Leveraging fs-extra
for Enhanced Operations
While Node.js’s native fs
module is powerful, there are scenarios where you might seek more functionality or a simplified API. This is where third-party libraries like fs-extra
come into play. fs-extra
builds on the original fs
module, offering additional methods and promising support out of the box, making it a valuable tool for developers looking for more than the standard module provides.
const fse = require('fs-extra');
// Copying a file with fs-extra
fse.copy('source.txt', 'destination.txt')
.then(() => console.log('File copied successfully'))
.catch(err => console.error('Error copying file:', err));
# Output:
# File copied successfully
In this example, we utilize fs-extra
to copy a file. The copy
method is a straightforward way to duplicate files, showcasing fs-extra
‘s promise-based syntax for cleaner, more readable code. The success of the operation is confirmed with a simple console log, demonstrating the library’s ease of use and efficiency.
Embracing fs/promises
for Modern Async/Await
Node.js introduced fs/promises
as a way to use the fs
module with modern async/await syntax, offering an alternative to the traditional callback pattern. This approach simplifies asynchronous file operations, making your code cleaner and more intuitive.
const fsp = require('fs').promises;
async function renameFile() {
try {
await fsp.rename('oldName.txt', 'newName.txt');
console.log('File renamed successfully');
} catch (err) {
console.error('Error renaming file:', err);
}
}
renameFile();
# Output:
# File renamed successfully
Here, the rename
operation is performed using fs/promises
. By wrapping the operation in an async function, we can await the promise returned by fsp.rename
, streamlining the process. This example highlights the benefits of using fs/promises
for asynchronous file operations, including error handling and code readability.
Comparing Approaches
Both fs-extra
and fs/promises
offer significant advantages over the traditional fs
module, particularly in terms of syntax and additional functionality. However, choosing between them depends on your specific project needs. fs-extra
is excellent for developers seeking extended file system operations beyond what’s available in the native module. On the other hand, fs/promises
is a perfect fit for those looking to leverage modern JavaScript features like async/await with the standard fs
functionality.
Understanding these alternative approaches to file system operations in Node.js can significantly enhance your development workflow. Whether you opt for the enriched feature set of fs-extra
or the modern syntax of fs/promises
, both libraries are powerful tools that extend the capabilities of npm fs, ensuring your projects are both efficient and maintainable.
Working with the fs
module in Node.js can sometimes feel like navigating a minefield. Let’s discuss some common pitfalls and their solutions to ensure your journey with npm fs is as smooth as possible.
Handling ENOENT
Errors
One of the most common errors you’ll encounter is ENOENT
, indicating that the specified file or directory does not exist. This can happen during read or write operations. Here’s how you can handle it gracefully:
const fs = require('fs');
fs.readFile('nonexistent.txt', 'utf8', (err, data) => {
if (err && err.code === 'ENOENT') {
console.error('File does not exist.');
return;
} else if (err) {
console.error('An unexpected error occurred:', err);
return;
}
console.log(data);
});
# Output:
# File does not exist.
In this code snippet, we attempt to read a file that doesn’t exist. By checking if err.code
is 'ENOENT'
, we can provide a clear message indicating the file’s absence. This approach prevents our application from crashing and allows us to handle the error more effectively.
Permission Issues
Another hurdle you might face are permission issues, where you don’t have the rights to read, write, or execute a file. Implementing a check before attempting an operation can save time and avoid errors.
fs.access('file.txt', fs.constants.R_OK | fs.constants.W_OK, (err) => {
if (err) {
console.error('No access to the file.');
return;
}
console.log('File is readable and writable.');
});
# Output:
# File is readable and writable.
Here, fs.access
is used to check if file.txt
is readable and writable. By using fs.constants.R_OK
and fs.constants.W_OK
, we specify the permissions to check for. This preemptive approach helps avoid running into permission-related errors during file operations.
Large File Memory Considerations
Handling large files requires careful consideration to avoid excessive memory usage. Streaming, as opposed to reading or writing a file in one go, is a more memory-efficient approach.
const fs = require('fs');
const stream = fs.createReadStream('largefile.txt');
stream.on('data', (chunk) => {
console.log('Received a chunk of data.');
});
stream.on('end', () => {
console.log('File reading completed.');
});
# Output:
# Received a chunk of data.
# Received a chunk of data.
# File reading completed.
This example illustrates reading a large file using streams. By processing the file in chunks (stream.on('data', callback)
), we significantly reduce the memory footprint of our application. This method is especially useful for large files, ensuring that your Node.js application remains responsive and efficient.
Understanding and mitigating these common issues will enhance your proficiency with the fs
module, making your Node.js file handling tasks smoother and more reliable.
Understanding fs
and Node.js
The fs
module is a cornerstone of Node.js, allowing developers to perform file system operations, such as reading and writing files, directly from their Node.js applications. But how does it actually work with the Node.js runtime and the underlying operating system? Let’s dive into the mechanics.
Synchronous vs. Asynchronous Operations
Node.js is designed to be non-blocking and asynchronous, meaning operations can run in parallel without stopping the execution of your application. This is crucial for developing efficient, scalable applications.
// Synchronous read example
const fs = require('fs');
const data = fs.readFileSync('example.txt', 'utf8');
console.log(data);
# Output:
# Contents of example.txt
In this synchronous example, fs.readFileSync
blocks the Node.js event loop until the file read operation is complete. This means no other operations can be performed until this one finishes. While straightforward, synchronous operations can lead to performance bottlenecks in your application.
// Asynchronous read example
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
# Output:
# File content: [Contents of example.txt]
Contrastingly, the asynchronous fs.readFile
method allows the rest of your application to continue running while the file is being read. This non-blocking behavior is a hallmark of efficient Node.js applications, enabling multiple operations to occur simultaneously.
Blocking vs. Non-Blocking I/O
The distinction between blocking and non-blocking I/O is fundamental to understanding Node.js’s efficiency. Blocking I/O operations wait for completion before moving on, whereas non-blocking I/O operations allow the execution to continue, handling operations’ results asynchronously.
Error Handling in fs
Error handling is paramount when working with file system operations. Incorrect handling can lead to uncaught exceptions and application crashes. Asynchronous methods in the fs
module use callbacks that provide an error object (err
) as the first argument, allowing developers to gracefully handle errors.
fs.readFile('nonexistent.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error encountered:', err);
return;
}
// Handle file content
});
# Output:
# Error encountered: [Error details]
This example demonstrates handling an error during an asynchronous file read operation. By checking the err
object, we can determine whether the operation was successful or not, and take appropriate action, such as logging the error or retrying the operation.
Understanding these fundamentals of the fs
module, including the difference between synchronous and asynchronous operations, blocking versus non-blocking I/O, and the importance of error handling, is crucial for mastering file system operations in Node.js. By leveraging npm fs effectively, developers can create more efficient and robust applications.
Expanding fs
Module Horizons
As you become adept at using the fs
module for basic and intermediate tasks, the next step is to explore how it integrates with other Node.js modules and real-world applications. This exploration will not only solidify your understanding of fs
but also open up new possibilities for your Node.js projects.
Building a Simple Static File Server
One practical application of the fs
module is creating a static file server. This can be particularly useful for serving HTML, CSS, and JavaScript files in a development environment or small-scale production setup.
const http = require('http');
const fs = require('fs');
const server = http.createServer((req, res) => {
fs.readFile('index.html', (err, data) => {
if (err) {
res.writeHead(404);
res.end('Error: File Not Found');
} else {
res.writeHead(200, {'Content-Type': 'text/html'});
res.end(data);
}
});
});
server.listen(3000, () => {
console.log('Server listening on port 3000');
});
# Output:
# Server listening on port 3000
In this example, we create a basic HTTP server that listens on port 3000. When a request is made, it attempts to serve index.html
using the fs
module to read the file. If the file is found, it’s served to the client; otherwise, a 404 error is returned. This showcases how fs
can be seamlessly integrated with http
to serve static files.
Integrating fs
with Express
For more complex applications, integrating fs
with the Express framework can enhance your project’s functionality, such as dynamically generating file listings or serving files based on user input.
const express = require('express');
const fs = require('fs');
const app = express();
app.get('/files', (req, res) => {
fs.readdir('./', (err, files) => {
if (err) {
res.status(500).send('Error reading directory.');
} else {
res.status(200).json(files);
}
});
});
app.listen(3000, () => console.log('Express server running on port 3000'));
# Output:
# Express server running on port 3000
This snippet demonstrates using fs.readdir
within an Express route to list all files in the current directory. The file list is then returned as a JSON response. This integration exemplifies how fs
can be utilized within a web application framework to create dynamic, file-based features.
Further Resources for Mastering Node.js fs
To continue your journey with the fs
module and Node.js, here are three recommended resources that provide in-depth tutorials, guides, and documentation:
- Node.js Official Documentation – A comprehensive resource for all things Node.js, including the
fs
module. The Net Ninja Node.js Tutorial Series – A video tutorial series that covers Node.js basics to advanced topics, including file system operations.
Mozilla Developer Network (MDN) Server-Side Development with Node.js – MDN offers a detailed guide on server-side development with Node.js, including working with files and the
fs
module.
These resources will help deepen your understanding of the fs
module and its applications, ensuring you’re well-equipped to tackle more complex Node.js projects.
Wrapping Up: Mastering fs
in Node.js
In this comprehensive guide, we’ve explored the vast capabilities of the fs
module, a fundamental tool for interacting with the file system in Node.js applications. From basic file operations to advanced file handling techniques, this guide has aimed to equip you with the knowledge to efficiently manage files in your Node.js projects.
We began with the basics, introducing how to install and require the fs
module in your Node.js applications. We covered reading and writing files asynchronously, emphasizing the importance of non-blocking operations for maintaining application performance.
Moving on, we delved into more sophisticated file handling techniques, such as file streaming and utilizing promises with fs
for better asynchronous control. We also discussed managing directories and explored how to leverage third-party libraries like fs-extra
and the newer fs/promises
API for enhanced file system operations.
In addressing common challenges, we provided solutions for troubleshooting issues like ENOENT
errors, permission problems, and considerations for handling large files efficiently to prevent memory overload.
Challenge | Solution |
---|---|
ENOENT Errors | Graceful error handling |
Permission Issues | Preemptive access checks |
Large Files | Streaming to reduce memory usage |
As we wrap up, remember that mastering file system operations with the fs
module opens up a world of possibilities for your Node.js applications. The ability to read, write, and manipulate files is a powerful capability that, when used effectively, can greatly enhance your applications’ functionality and performance.
We encourage you to experiment with the code examples provided throughout this guide and to explore further into the Node.js file system capabilities. With practice and exploration, you’ll find that the fs
module is an invaluable tool in your development toolkit. Happy coding!