Insertion Sort in Java: Implementation Guide
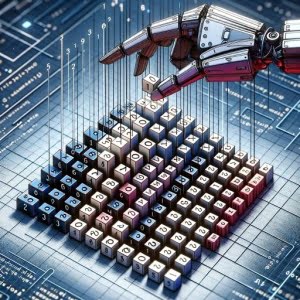
Are you finding it challenging to implement the Insertion Sort algorithm in Java? You’re not alone. Many developers find themselves puzzled when it comes to sorting algorithms, but we’re here to help.
Think of the Insertion Sort as a meticulous librarian, carefully placing each book (or in our case, data element) in its rightful place. It’s a simple yet powerful algorithm that can help you arrange your data in a desired order.
In this guide, we’ll walk you through the process of implementing Insertion Sort in Java, from the basics to more advanced techniques. We’ll cover everything from the core logic of the algorithm, its implementation in code, to optimizations for handling larger data sets.
So, let’s dive in and start mastering Insertion Sort in Java!
TL;DR: How Do I Implement Insertion Sort in Java?
To implement the
Insertion Sort algorithm
in Java, you can use a simple method that sorts an array by comparing each element with its predecessor and swapping them if necessary. The method may combinefor
loops,for (int i = 1; i < n; ++i) {}
andwhile
loops,while (j >= 0 && array[j] > key) {}
. Here’s a basic implementation:
public void insertionSort(int array[]) {
int n = array.length;
for (int i = 1; i < n; ++i) {
int key = array[i];
int j = i - 1;
while (j >= 0 && array[j] > key) {
array[j + 1] = array[j];
j = j - 1;
}
array[j + 1] = key;
}
}
In this example, we define a method insertionSort
that takes an array as input. It iterates over each element in the array, starting from the second one. For each element, it compares it with the previous elements and moves it to its correct position in the sorted part of the array.
This is a basic way to implement Insertion Sort in Java, but there’s much more to learn about optimizing this algorithm and using it in different contexts. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Implementing Insertion Sort in Java: A Step-by-Step Guide
- Optimizing Insertion Sort for Larger Data Sets
- Exploring Alternative Sorting Algorithms in Java
- Common Issues and Solutions with Insertion Sort
- Understanding Sorting Algorithms and Their Importance
- Insertion Sort in Larger Java Projects and Real-World Applications
- Wrapping Up: Implementing Insertion Sort in Java
Implementing Insertion Sort in Java: A Step-by-Step Guide
Let’s start with the basics. The Insertion Sort algorithm is a simple sorting algorithm that works in a similar way to how you might sort a hand of playing cards. Imagine you’re holding a hand of cards, and you want to sort them in ascending order. You would start from the left and compare each card with the one before it, swapping them if necessary until each card is in its correct position. That’s the essence of Insertion Sort.
A Simple Code Example
Let’s see how this translates into Java code. Here’s a simple implementation of the Insertion Sort algorithm:
public void insertionSort(int array[]) {
int a = array.length;
for (int b = 1; b < a; ++b) {
int key = array[b];
int c = b - 1;
while (c >= 0 && array[c] > key) {
array[c + 1] = array[c];
c = c - 1;
}
array[c + 1] = key;
}
}
In this code, we define a method insertionSort
that takes an array as input. It iterates over each element in the array, starting from the second one. For each element, it compares it with the previous elements and moves it to its correct position in the sorted part of the array.
Understanding the Algorithm
The algorithm works by dividing the array into a sorted and an unsorted region. The sorted region starts with the first element, and the unsorted region contains the rest. The algorithm repeatedly picks the first element in the unsorted region and inserts it into the correct position in the sorted region.
Advantages and Potential Pitfalls
Insertion Sort is a great choice for small arrays or arrays that are already partially sorted, as it has a best-case time complexity of O(n) in these scenarios. However, for larger arrays or arrays that are in reverse order, the worst-case time complexity is O(n^2), which means it can be quite slow compared to other sorting algorithms like Quick Sort or Merge Sort.
In the next section, we’ll discuss how to optimize the Insertion Sort algorithm for larger data sets in Java.
Optimizing Insertion Sort for Larger Data Sets
While the basic implementation of Insertion Sort is straightforward, it’s not the most efficient for larger data sets. The worst-case and average time complexity of Insertion Sort is O(n^2), which can lead to performance issues with larger arrays. But don’t worry, there are ways to optimize it.
Binary Insertion Sort
One method to optimize Insertion Sort is to use a binary search to find the position where an element needs to be inserted. This variant is known as Binary Insertion Sort. The binary search reduces the number of comparisons in the algorithm, although the number of swaps remains the same.
public void binaryInsertionSort(int array[]) {
for (int i = 1; i < array.length; i++) {
int key = array[i];
int j = Math.abs(Arrays.binarySearch(array, 0, i, key) + 1);
System.arraycopy(array, j, array, j+1, i-j);
array[j] = key;
}
}
In this code, we use Arrays.binarySearch()
to find the position where the key needs to be inserted. System.arraycopy()
is then used to shift the larger values to the right. This method reduces the number of comparisons but the number of swaps (array rewrites) remains the same.
Time and Space Complexity
The time complexity of the binary insertion sort is O(n log n) for comparisons but the total time complexity remains O(n^2) due to swaps. The space complexity is O(1) because it’s an in-place sorting algorithm.
The optimization with binary search can be useful when the cost of comparisons outweighs the cost of swaps. In the next section, we’ll explore other sorting algorithms in Java and how they compare to Insertion Sort.
Exploring Alternative Sorting Algorithms in Java
While Insertion Sort has its advantages, particularly with smaller or partially sorted arrays, it’s not always the most efficient option. Let’s explore two other popular sorting algorithms in Java: Quick Sort and Merge Sort, and see how they compare to Insertion Sort.
Quick Sort: A Speedy Alternative
Quick Sort is a divide-and-conquer algorithm that’s often faster than Insertion Sort for larger arrays. It works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The algorithm then recursively sorts the sub-arrays.
Here’s a basic implementation of Quick Sort in Java:
public void quickSort(int[] array, int low, int high) {
if (low < high) {
int pi = partition(array, low, high);
quickSort(array, low, pi - 1);
quickSort(array, pi + 1, high);
}
}
private int partition(int[] array, int low, int high) {
int pivot = array[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (array[j] < pivot) {
i++;
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
int temp = array[i + 1];
array[i + 1] = array[high];
array[high] = temp;
return i + 1;
}
Merge Sort: Merging for Efficiency
Merge Sort is another divide-and-conquer algorithm that can be more efficient than Insertion Sort for larger arrays. It works by dividing the unsorted list into N sublists, each containing one element, and then repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining.
Here’s a basic implementation of Merge Sort in Java:
public void mergeSort(int[] array, int left, int right) {
if (left < right) {
int mid = (left + right) / 2;
mergeSort(array, left, mid);
mergeSort(array , mid + 1, right);
merge(array, left, mid, right);
}
}
private void merge(int[] array, int left, int mid, int right) {
// code for merging two sorted arrays
}
Comparing Quick Sort, Merge Sort, and Insertion Sort
While Quick Sort and Merge Sort can be more efficient than Insertion Sort for larger arrays, they also have their drawbacks. Quick Sort’s performance depends heavily on the choice of pivot, and it’s not a stable sort. Merge Sort, on the other hand, requires additional space proportional to the size of the input array.
When choosing a sorting algorithm, it’s important to consider the characteristics of the data you’re working with, as well as the specific requirements of your application. In some cases, the simplicity and in-place nature of Insertion Sort might make it the best choice.
Common Issues and Solutions with Insertion Sort
While insertion sort is easy to understand and implement, you may encounter some common issues when working with it, especially when dealing with special cases. Let’s discuss these potential pitfalls and how to handle them.
Handling Duplicate Values
One common issue is handling duplicate values. In the standard implementation of Insertion Sort, duplicate values are treated just like any other value. This means that if there are duplicate values in your array, they will be sorted just like any other value. Here’s an example:
public void insertionSort(int array[]) {
int n = array.length;
for (int i = 1; i < n; ++i) {
int key = array[i];
int j = i - 1;
while (j >= 0 && array[j] > key) {
array[j + 1] = array[j];
j = j - 1;
}
array[j + 1] = key;
}
}
int[] array = {4, 3, 2, 4, 1};
insertionSort(array);
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3, 4, 4]
In this example, the duplicate ‘4’ values are sorted just like any other value in the array.
Sorting in Descending Order
Another common requirement is to sort the array in descending order. To achieve this, you can simply modify the comparison in the while loop from array[j] > key
to array[j] < key
. Here’s an example:
public void insertionSortDescending(int array[]) {
int n = array.length;
for (int i = 1; i < n; ++i) {
int key = array[i];
int j = i - 1;
while (j >= 0 && array[j] < key) {
array[j + 1] = array[j];
j = j - 1;
}
array[j + 1] = key;
}
}
int[] array = {1, 2, 3, 4, 5};
insertionSortDescending(array);
System.out.println(Arrays.toString(array));
// Output:
// [5, 4, 3, 2, 1]
In this example, the array is sorted in descending order.
Remember, while Insertion Sort is a simple and effective sorting algorithm, it’s important to understand its limitations and how to handle special cases. With these tips in mind, you’ll be able to use Insertion Sort effectively in your Java projects.
Understanding Sorting Algorithms and Their Importance
Before we delve deeper into Insertion Sort, it’s essential to understand the broader world of sorting algorithms. Sorting algorithms are fundamental to computer science and are used in a wide range of applications, from database management to computer graphics and machine learning.
The Theory Behind Insertion Sort
Insertion Sort is one of the simplest sorting algorithms. It’s based on the idea of dividing an array into a sorted and an unsorted region. The sorted region starts with the first element, and the unsorted region contains the rest. The algorithm repeatedly picks the first element in the unsorted region and inserts it into the correct position in the sorted region.
Here’s a simple illustration of the process using an array of integers:
int[] array = {5, 2, 4, 6, 1, 3};
insertionSort(array);
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3, 4, 5, 6]
In this example, the Insertion Sort algorithm starts at the second element (2). It compares 2 with the first element (5) and swaps them because 2 is smaller than 5. The algorithm then moves to the next element (4) and shifts it to its correct position in the sorted region, and so on.
Insertion Sort vs. Other Sorting Algorithms
While Insertion Sort is simple and efficient for small or partially sorted arrays, it’s not the most efficient option for larger arrays. Other sorting algorithms like Quick Sort and Merge Sort can handle larger arrays more efficiently, but they are also more complex and require more computational resources.
In the end, the choice of sorting algorithm depends on the specific requirements of your application. Factors to consider include the size of the data set, whether the data is already partially sorted, and the computational resources available. Understanding these factors and how different sorting algorithms work is key to making an informed choice.
Insertion Sort in Larger Java Projects and Real-World Applications
Insertion Sort is more than just a simple sorting algorithm. It plays a crucial role in larger Java projects and has numerous real-world applications. From organizing records in databases to optimizing search results in web applications, Insertion Sort can be a handy tool in any developer’s toolkit.
Insertion Sort in Database Management
In database management systems, Insertion Sort is often used to sort small amounts of data or to sort large data sets that are already partially sorted. It’s also commonly used in conjunction with other, more complex sorting algorithms as part of a hybrid approach. For example, the Timsort algorithm, which is the default sorting algorithm in Java, Python, and Android, starts with Insertion Sort for small data sets before switching to Merge Sort for larger ones.
Insertion Sort in Web Applications
In web applications, Insertion Sort can be used to optimize search results or to sort user-generated content. For example, a social media app might use Insertion Sort to sort posts or comments by date or popularity. While more efficient algorithms are available for larger data sets, the simplicity and in-place nature of Insertion Sort make it a good choice for small to medium-sized data sets.
Further Resources for Java Sorting Algorithms
If you’re interested in delving deeper into Java sorting algorithms, here are some resources you might find useful:
- Java Sorting Algorithms: This tutorial from Baeldung provides a comprehensive overview of various sorting algorithms in Java, including Bubble Sort, Selection Sort, Insertion Sort, Quick Sort, and Merge Sort.
Algorithms, Part I: This online course from Coursera, offered by Princeton University, covers fundamental algorithms, including sorting and searching, as well as data structures in Java.
Data Structures and Algorithms in Java: This book by Robert Lafore provides an introduction to data structures and algorithms, including sorting algorithms like Insertion Sort.
By understanding how Insertion Sort and other sorting algorithms work, you’ll be able to make more informed decisions when it comes to choosing the right algorithm for your Java projects.
Wrapping Up: Implementing Insertion Sort in Java
In this comprehensive guide, we’ve delved into the world of Insertion Sort, a fundamental sorting algorithm in computer science, and learned how to implement it in Java.
We began with the basics, understanding the core logic of the algorithm and implementing it in a simple Java method. We then explored how to optimize Insertion Sort for larger data sets using Binary Insertion Sort, which uses a binary search to reduce the number of comparisons.
Next, we ventured into alternative sorting algorithms, Quick Sort and Merge Sort, comparing their efficiency and characteristics with Insertion Sort. We also discussed common issues you might encounter when implementing Insertion Sort, such as handling duplicate values and sorting in descending order, providing solutions for each issue.
Here’s a quick comparison of the sorting algorithms we’ve discussed:
Algorithm | Best Case | Average Case | Worst Case | Space Complexity |
---|---|---|---|---|
Insertion Sort | O(n) | O(n^2) | O(n^2) | O(1) |
Binary Insertion Sort | O(n) | O(n^2) | O(n^2) | O(1) |
Quick Sort | O(n log n) | O(n log n) | O(n^2) | O(log n) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Whether you’re just starting out with Insertion Sort or looking to deepen your understanding of sorting algorithms in Java, we hope this guide has provided valuable insights and practical knowledge.
The ability to effectively sort data is a fundamental skill in computer science and software development. With the knowledge of Insertion Sort and its alternatives, you’re well equipped to handle a wide range of data sorting tasks in your Java projects. Happy coding!