How To Reverse a String in Python: 3 Easy Options
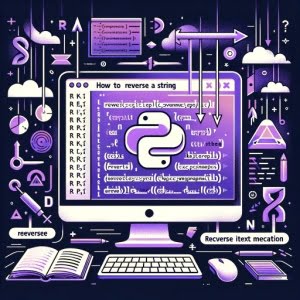
Are you grappling with the task of reversing strings in Python? It can be as tricky as attempting to say a sentence backwards, but don’t worry. Python, with its versatile features, provides you with a plethora of ways to accomplish this task.
Whether you’re a beginner just getting your feet wet or a seasoned programmer looking for advanced techniques, this comprehensive guide will walk you through the process of reversing a string in Python. So, let’s flip the script and dive right in!
TL;DR: How Can I Reverse a String in Python?
Python offers a variety of ways to reverse a string. The most straightforward method is by using slicing with the operator
[::-1]
. Here’s a quick example:
s = 'Hello'
reversed_s = s[::-1]
print(reversed_s)
# Output:
# 'olleH'
In this example, we’ve used Python’s slicing feature to reverse the string ‘Hello’. The [::-1]
slice means start at the end of the string and end at position 0, move with the step -1 (which means one step backwards).
While slicing is the simplest method, Python provides several other ways to achieve the same result. So, if you’re curious to explore more detailed understanding and advanced usage scenarios, continue reading!
Table of Contents
Slicing for Easy Python String Reversal
Python’s slicing feature is a versatile tool for manipulating sequences, including strings. It’s the simplest method to reverse a string in Python. Let’s break down how it works.
s = 'Hello, World!'
reversed_s = s[::-1]
print(reversed_s)
# Output:
# '!dlroW ,olleH'
In this example, [::-1]
is a slice that starts at the end of the string (s
), and ends at position 0, moving with the step -1
(which means one step backwards). The result is a reversed string.
While slice it works perfectly for ASCII strings, it can produce unexpected results with Unicode strings, especially those containing characters with diacritical marks or emojis.
Despite these potential pitfalls, slicing is a quick and efficient way to reverse a string in Python, making it a great starting point for beginners exploring string manipulation.
Advanced String Reversal
Python is known for its rich set of built-in functions, and when it comes to reversing a string, functions like reversed()
and join()
come in handy. Let’s see how we can use these functions to reverse a string.
s = 'Hello, World!'
reversed_s = ''.join(reversed(s))
print(reversed_s)
# Output:
# '!dlroW ,olleH'
In this example, we first use the reversed()
function, which returns a reverse iterator. Then, we use the join()
function to concatenate all the characters in the reverse iterator into a new string.
This method is a bit more complex than slicing, but it’s more readable and can handle Unicode strings better. However, it might be a bit slower than slicing for very long strings due to the overhead of function calls.
Understanding the nuances of these different methods will help you choose the best approach for your specific use case. While slicing is quick and efficient, using built-in functions like reversed()
and join()
can offer more readability and better handling of Unicode strings.
Expert Methods: Recursion and functools
While slicing and built-in functions are the go-to methods for reversing a string in Python, there are other techniques that offer more flexibility and control. Two such methods are recursion and the reduce()
function from the functools
module.
Recursion
Recursion involves a function calling itself to solve smaller instances of the same problem. Here’s how you can use recursion to reverse a string:
def reverse_string(s):
if len(s) == 0:
return s
else:
return reverse_string(s[1:]) + s[0]
s = 'Hello, World!'
print(reverse_string(s))
# Output:
# '!dlroW ,olleH'
In this example, the function reverse_string()
calls itself with a progressively smaller string (s[1:]
), and then concatenates the first character of the current string (s[0]
) at the end. This results in a reversed string.
Recursion can be a powerful tool, but it’s more complex and can lead to a stack overflow for very long strings.
functools.reduce()
The reduce()
function from the functools
module can also be used to reverse a string. Here’s an example:
from functools import reduce
s = 'Hello, World!'
reversed_s = reduce(lambda x, y: y + x, s)
print(reversed_s)
# Output:
# '!dlroW ,olleH'
In this example, reduce()
applies the lambda function to the string s
, effectively reversing it. The lambda function takes two arguments, x
and y
, and concatenates them in reverse order (y + x
).
While reduce()
is a powerful function, it’s not as readable as other methods, and it might be slower for very long strings.
When considering which method to use, keep in mind your specific requirements, the size of the strings you’re dealing with, and the trade-off between readability and performance.
Troubleshooting Common Issues
While reversing a string in Python can be straightforward, you may encounter some issues, especially when dealing with Unicode strings. Let’s discuss these common issues and how to troubleshoot them.
Handling Unicode Strings
Python’s string reversal methods can behave unexpectedly with Unicode strings, especially those containing characters with diacritical marks or emojis.
For instance, consider the following example using slicing:
s = 'Hello, World! '
reversed_s = s[::-1]
print(reversed_s)
# Output:
# ' !dlroW ,olleH'
In this case, the emoji is handled correctly. However, if you’re dealing with a string that contains a character composed of multiple Unicode code points (like a letter with a diacritical mark), slicing might separate these code points, resulting in an incorrect result.
To handle such cases, you can use the unicodedata
module to normalize the string before reversing it. Here’s an example:
import unicodedata
s = 'résumé'
normalized_s = unicodedata.normalize('NFC', s)
reversed_s = normalized_s[::-1]
print(reversed_s)
# Output:
# 'émusér'
In this example, we first normalize the string using the unicodedata.normalize()
function, which combines the separate code points for each character. Then, we reverse the normalized string using slicing.
Understanding Python Strings
Before diving deeper into string reversal techniques, it’s important to understand the basics of Python’s string data type and the concept of slicing.
Python Strings: More Than Just Text
In Python, a string is a sequence of characters. It’s an immutable data type, which means you can’t change an existing string. However, you can create a new string based on the old one, which is what we do when we reverse a string.
s = 'Hello, World!'
print(s[4])
# Output:
# 'o'
In this example, we access the character at index 4 of the string s
. Python’s strings are zero-indexed, so the character at index 4 is ‘o’.
Slicing: A Powerful Tool
Slicing is a feature in Python that allows you to access parts of a sequence, such as a string. A slice has a start index, an end index, and a step. When reversing a string, we use a slice with a step of -1, which means ‘go backwards’.
s = 'Hello, World!'
print(s[::-1])
# Output:
# '!dlroW ,olleH'
In this example, we use a slice with a step of -1 to create a new string that’s the reverse of s
.
The reversed()
and join()
Functions
Python’s reversed()
function returns a reverse iterator of a sequence, while the join()
function concatenates a sequence of strings.
s = 'Hello, World!'
reversed_s = ''.join(reversed(s))
print(reversed_s)
# Output:
# '!dlroW ,olleH'
In this example, we first use reversed(s)
to get a reverse iterator of the string s
, and then we use ''.join()
to concatenate the characters into a new string.
Understanding these fundamental concepts is key to mastering string reversal in Python.
Beyond String Reversal
Beyond reversing strings, Python provides a rich set of built-in functions and features for string manipulation, such as len()
, split()
, replace()
, and format()
. These functions can be used to perform a wide variety of tasks, from counting the number of characters in a string to replacing substrings.
To deepen your understanding of Python’s string manipulation capabilities, I encourage you to explore these functions and features.
There are many resources available online, including Python’s official documentation and various tutorials. Remember, mastering a programming language is a continuous journey of learning and exploration.
Further Resources for Python Strings
If you’re interested in learning more ways to handle strings in Python, here are a few resources that you might find helpful:
- Understanding Python Strings: From Basics to Advanced: Understand Python strings from the basics to more advanced concepts, making your coding journey smoother and more efficient.
Python F-String: Formatting Strings in Python: Learn how to use F-strings, a new and efficient way to format strings in Python.
Python Int to String Conversion Guide with Examples: This guide provides examples and explanations on how to convert integers to strings in Python using different methods.
Python How To: Reverse a String: A tutorial on w3schools.com that demonstrates different methods to reverse a string in Python.
Reverse String in Python – 5 Different Ways: An article on GeeksforGeeks that showcases five different approaches to reverse a string in Python, providing code examples.
Reverse a String in Python: An Overview of String Reversal Techniques: A comprehensive overview on Real Python that covers various techniques to reverse a string in Python, including slicing, loops, and recursion.
Wrapping Up:
In this guide, we’ve explored various methods to reverse a string in Python. We started with the simplest approach, slicing, which is quick and efficient, yet it can produce unexpected results with Unicode strings.
Next, we delved into more advanced techniques, using built-in functions like reversed()
and join()
, which offer more readability and better handling of Unicode strings.
We also introduced expert-level techniques such as recursion and the reduce()
function from the functools
module. While these methods are more complex, they offer more flexibility and control.
Finally, we discussed common issues one may encounter when reversing a string, particularly when dealing with Unicode strings, and provided solutions and workarounds.
Understanding these different methods and their potential issues will help you effectively reverse strings in Python, whether you’re working on a simple script or a complex algorithm.