Learn Python: List Files In a Directory
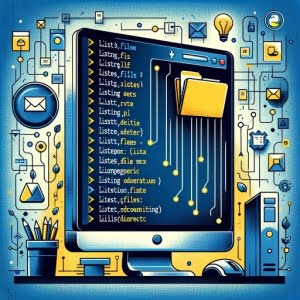
Ever found yourself wrestling with the task of listing files in a directory using Python? Think of Python as a diligent librarian, capable of sorting and organizing your vast collection of files with ease.
This comprehensive guide is your roadmap to mastering the art of listing files in a directory using Python. Whether you’re a beginner just starting out or an experienced coder looking to brush up on advanced techniques, we’ve got you covered.
Let’s dive in and start exploring Python’s powerful file-handling capabilities.
TL;DR: How Do I List Files in a Directory Using Python?
You can leverage Python’s built-in
os
module to list files in a directory. Here’s a quick example to illustrate this:
import os
print(os.listdir('/path/to/directory'))
# Output:
# ['file1.txt', 'file2.txt', 'file3.txt']
This piece of code imports the os
module, then uses the os.listdir()
function to retrieve and print a list of files in the specified directory. The output is a list of filenames, just like you’d see if you were viewing the directory in a file explorer.
Intrigued? Keep reading for a more in-depth exploration of listing files in a directory using Python, including basic usage, advanced techniques, and common pitfalls to avoid.
Table of Contents
Harnessing Python’s os.listdir() Function
The os.listdir()
function is a simple yet powerful tool in Python, particularly when it comes to listing files in a directory.
This function is a part of Python’s built-in os
module, which provides a portable way of using operating system dependent functionality like reading or writing to the file system.
Here’s a basic example of how to use os.listdir()
:
import os
files = os.listdir('/path/to/directory')
print(files)
# Output:
# ['file1.txt', 'file2.txt', 'file3.txt']
In this example, we first import the os
module. Next, we call the os.listdir()
function with the path to the directory as an argument. The function returns a list of filenames in the given directory, which we store in the files
variable. Finally, we print this list to the console.
One of the key advantages of os.listdir()
is its simplicity and straightforwardness. With just a couple of lines of code, you can retrieve a list of all files in a directory.
It’s important to note that
os.listdir()
does not list files in any specific order, and it includes all entries in the directory including subdirectories and special files like device nodes.
Advanced File Listing Techniques
While the os.listdir()
function is a great starting point, Python offers more advanced techniques to refine your file listing. Let’s explore how you can list files with specific extensions and sort the files.
Filtering Files by Extension
Suppose you’re only interested in .txt
files. You can use list comprehension along with os.listdir()
to achieve this. Here’s an example:
import os
files = os.listdir('/path/to/directory')
# Filter out .txt files
txt_files = [file for file in files if file.endswith('.txt')]
print(txt_files)
# Output:
# ['file1.txt', 'file2.txt', 'file3.txt']
In this code, we use the endswith()
string method to filter out files ending with .txt
. The result is a list of .txt
files in the directory.
Sorting Files
Sorting the files can be done using Python’s built-in sorted()
function. Here’s how you can sort the files in ascending order:
import os
files = os.listdir('/path/to/directory')
# Sort files in ascending order
sorted_files = sorted(files)
print(sorted_files)
# Output:
# ['file1.txt', 'file2.txt', 'file3.txt']
In this code, we pass the list of files to the sorted()
function. The function returns a new list containing all items from the original list in ascending order.
These advanced techniques allow you to customize and refine the output of os.listdir()
, making it a more versatile tool in your Python toolkit.
Alternative Methods for File Listing
While os.listdir()
is a powerful function, Python offers other equally compelling methods to list files in a directory. Let’s explore two such alternatives: the glob
module and the os.walk()
function.
Listing Files with Glob
The glob
module in Python is used to retrieve files/pathnames matching a specified pattern. Here’s how you can use it to list all .txt
files in a directory:
import glob
txt_files = glob.glob('/path/to/directory/*.txt')
print(txt_files)
# Output:
# ['/path/to/directory/file1.txt', '/path/to/directory/file2.txt', '/path/to/directory/file3.txt']
In this code, glob.glob()
returns a list of pathnames that match the specified pattern. The advantage of using glob
is that it supports wildcard characters, allowing you to filter files more flexibly.
However, glob
does not support recursive directory traversal, meaning it cannot list files in subdirectories.
Traversing Directories with os.walk()
The os.walk()
function generates the file names in a directory tree by walking the tree either top-down or bottom-up.
Here’s how you can use it to list all files in a directory and its subdirectories:
import os
for root, dirs, files in os.walk('/path/to/directory'):
for file in files:
print(os.path.join(root, file))
# Output:
# /path/to/directory/file1.txt
# /path/to/directory/file2.txt
# /path/to/directory/subdirectory/file3.txt
In this code, os.walk()
returns a generator that produces tuples containing the path to a directory, a list of the subdirectories, and a list of the filenames in the directory.
The advantage of os.walk()
is that it can traverse directories recursively, making it ideal for tasks that involve subdirectories. However, it can be slower than os.listdir()
and glob.glob()
for tasks that don’t require recursive traversal.
These alternative approaches offer more flexibility and control than os.listdir()
, making them valuable additions to your Python file-handling toolkit.
Troubleshooting Common Issues
While Python provides robust tools for listing files in a directory, you may occasionally encounter issues such as ‘FileNotFoundError’ or ‘PermissionError’. Let’s discuss these common problems and their solutions.
Handling FileNotFoundError
A ‘FileNotFoundError’ typically occurs when the directory you’re trying to list files from doesn’t exist. Here’s an example:
import os
try:
files = os.listdir('/non/existent/directory')
except FileNotFoundError:
print('Directory not found')
# Output:
# Directory not found
In this code, we use a try/except block to catch the ‘FileNotFoundError’. If the directory doesn’t exist, Python raises a ‘FileNotFoundError’, which we catch and print a friendly message instead of letting the program crash.
Resolving PermissionError
A ‘PermissionError’ occurs when you don’t have the necessary permissions to read the directory. You can handle this error similarly to ‘FileNotFoundError’:
import os
try:
files = os.listdir('/protected/directory')
except PermissionError:
print('Permission denied')
# Output:
# Permission denied
In this example, we catch the ‘PermissionError’ and print a friendly message. Note that resolving a ‘PermissionError’ usually involves changing the permissions of the directory, which is beyond the scope of this guide.
Understanding these common issues and their solutions can save you a lot of time and frustration when listing files in a directory using Python.
Understanding Python’s OS Module
Python’s os
module is a powerful tool for interacting with the operating system. It contains numerous functions for file and directory manipulation, including the os.listdir()
function we’ve been exploring.
The Role of the OS Module
The os
module provides a way of using operating system dependent functionality. These functions include reading or writing to the file system, starting or killing processes, and more.
In the context of our current discussion, the os
module allows us to interact with directories and files stored on our system.
Here’s a simple example of using the os
module to get the current working directory:
import os
current_directory = os.getcwd()
print(current_directory)
# Output:
# /path/to/current/directory
In this code, os.getcwd()
returns the path of the current working directory. This is the directory where Python is currently operating.
File Paths and Directories in Python
A file path in Python is just a string that represents the location of a file or a directory on your file system. For instance, '/path/to/directory'
is a file path that points to a directory named 'directory'
.
Remember that different operating systems use different path conventions. For instance, Windows uses backslashes (
\
) while Unix-based systems like Linux and macOS use forward slashes (/
).
The os
module provides functions like os.path.join()
to handle these differences automatically, making your code more portable.
Exploring Related Concepts
Mastering the art of listing files in a directory opens the door to related file handling concepts in Python. These include file I/O operations like reading and writing files, and file manipulation tasks like renaming or deleting files.
These skills, combined with file listing, can significantly enhance your productivity and efficiency in Python.
# Example of reading a file
with open('/path/to/directory/file.txt', 'r') as file:
print(file.read())
# Output:
# Contents of file.txt
In this code, we open a file in read mode, read the contents of the file, and print them. This is a simple example of file I/O in Python.
Further Resources for Python File I/O Operations
There are many resources available online, including these free resources:
- Python OS Module Fundamentals – Discover how to execute system commands and shell scripts from within Python.
Python “open()” Explained – Discover the flexibility of “open” for text and binary file operations.
Deleting Files in Python – A guide on various methods to delete files in Python.
FreeCodeCamp’s Python file handling guide: This article explains Python’s built-in functions for writing, reading, and appending files.
Python’s official filesys library documentation details the functions and methods in Python’s file system manipulation library.
Programiz’s guide on Python file operations offers an understanding of various file operations in Python.
We encourage you to explore these related concepts and delve deeper into Python’s powerful file handling capabilities.
Recap:
In our journey to understand how to list files in a directory using Python, we’ve explored a variety of techniques, from the basic os.listdir()
function to more advanced approaches involving the glob
module and the os.walk()
function.
We’ve seen how simple it is to get a list of files in a directory with os.listdir()
, and how we can refine this list by filtering files by extension or sorting them. We’ve also explored how to handle common issues like ‘FileNotFoundError’ and ‘PermissionError’.
In our exploration of alternative methods, we’ve seen how the glob
module can provide more flexible file filtering, and how os.walk()
can traverse directories recursively.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
os.listdir() | Simple, easy to use | Does not support recursive directory traversal, does not list files in any specific order |
glob.glob() | Supports wildcard characters for flexible file filtering | Does not support recursive directory traversal |
os.walk() | Supports recursive directory traversal | Can be slower for tasks that don’t require recursive traversal |
As we’ve seen, Python provides a rich set of tools for listing files in a directory, each with its own strengths and weaknesses. By understanding these tools and how to use them, you can handle a wide range of file listing tasks with ease and efficiency.