Python Delete File | How To Remove File or Folder in Python
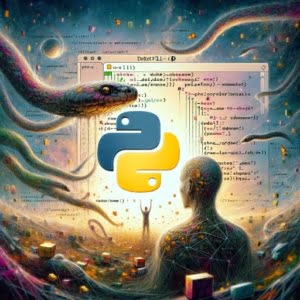
Ever felt overwhelmed by a clutter of files and directories in your Python project, unsure of how to efficiently delete them? You’re not alone. Managing files and directories is a routine task in Python, which can often seem intimidating.
But there’s good news! Python comes loaded with a rich library of pre-built modules, such as os
, pathlib
, and shutil
, that simplify file and directory deletion.
In this guide, we aim to unravel the mystery of file and directory deletion in Python. We’ll investigate various methods and modules, debate their pros and cons, and provide practical examples to help you comprehend their application.
So, fasten your seatbelts and let’s navigate the world of file and directory deletion in Python!
TL;DR: How do I delete files and directories in Python?
Python offers several methods to delete files and directories using the
os
,pathlib
, andshutil
modules. For instance, to delete a file, you can useos.remove('file_path')
orpathlib.Path('file_path').unlink()
. To delete directories, Python providesos.rmdir()
for empty directories andshutil.rmtree()
for non-empty directories. Read the rest of the article for more advanced methods, background, tips, and tricks.
# Deleting a file
import os
os.remove('file_path')
# Deleting an empty directory
os.rmdir('directory_path')
# Deleting a non-empty directory
import shutil
shutil.rmtree('directory_path')
Table of Contents
Understanding File Deletion
Before diving into the process of deleting files, it’s crucial to familiarize ourselves with the tools Python provides for this purpose. Two powerful modules, namely os
and pathlib
, are at our disposal for file deletion. Each module brings unique methods to the table, and comprehending these methods is essential for managing your file system efficiently.
Using the os module
The os
module offers a commonly used method for file deletion – os.remove()
. This method requires the path of the file you wish to delete as an argument. Here’s a quick demonstration:
import os
os.remove('path_to_file')
Simple, isn’t it? But what if we want to utilize the pathlib
module instead?
Using the pathlib module
Pathlib
houses a similar method for file deletion called pathlib.Path.unlink()
. This method also takes the file path as an argument. However, it comes with a twist: you need to create a path object first. Here’s how you can achieve this:
from pathlib import Path
file_path = Path('path_to_file')
file_path.unlink()
You might be pondering, ‘Why would I opt for pathlib
when os
appears simpler?’ That’s an excellent question. The choice between os.remove()
, pathlib.Path.unlink()
, and the pathlib
module in general can depend on several factors, including your Python version and operating system compatibility. For example, pathlib
is a newer module introduced in Python 3.4. So, if you’re working with older Python versions, os.remove()
might be a better fit.
That’s not all. Pathlib
also offers object-oriented filesystem paths, which can be a significant advantage if you prefer working with object-oriented programming. Additionally, some developers find pathlib
to be more intuitive and user-friendly than os
.
So, which method should you choose? The answer hinges on your specific needs and circumstances. Both os.remove()
and pathlib.Path.unlink()
are potent tools for file deletion in Python, and understanding their usage can simplify your file management tasks immensely.
Key Takeaways
- Python offers two powerful modules for file deletion:
os
andpathlib
. os.remove()
andpathlib.Path.unlink()
are the primary methods for deleting files in Python.- The choice between
os
andpathlib
depends on your Python version, operating system compatibility, and preference for object-oriented programming.
Deleting Files from Directories
Having grasped the basics of file deletion in Python, let’s elevate our understanding. Deleting single files is handy, but what if the requirement is to delete multiple files simultaneously, or even all files from a directory? Python has solutions for these scenarios too.
Deleting All Files in a Directory
To purge all files from a directory, we can employ a combination of os.listdir()
and os.remove()
. The os.listdir()
function fetches a list of all files and directories in the specified directory. We can then iterate over this list and use os.remove()
to delete each file. Here’s a demonstration:
import os
for filename in os.listdir('directory_path'):
if os.path.isfile(os.path.join('directory_path', filename)):
os.remove(os.path.join('directory_path', filename))
Deleting Files Matching a Specific Pattern
But what if we only want to delete files that match a certain pattern? That’s where the glob
module shines. The glob
module identifies all the pathnames matching a specified pattern and returns them in a list. We can then iterate over this list and use os.remove()
to delete each file. Here’s how you can accomplish this:
import glob
import os
for filename in glob.glob('directory_path/*.txt'):
os.remove(filename)
In this example, we’re deleting all .txt
files from the specified directory.
Deleting Files from All Subfolders
Python also facilitates deleting files from all subfolders of a directory using the iglob()
function, which returns an iterator instead of a list. This can be more efficient if you’re dealing with a large number of files. Here’s how you can execute this:
import glob
import os
for filename in glob.iglob('directory_path/**/*.txt', recursive=True):
os.remove(filename)
In this example, we’re deleting all .txt
files from the specified directory and all its subfolders.
Python offers various methods for deleting multiple files, such as loops, list comprehension, and the glob
module. These methods can prove incredibly useful in practical applications, such as managing large datasets or freeing up storage space. For instance, you might need to delete all log files older than a month, or all .tmp
files left over from a previous operation. With Python, tasks like these become straightforward.
Pattern-based file deletion can be particularly vital when managing large datasets. By deleting files based on specific patterns or conditions, you can maintain a clean and manageable dataset, conserve valuable storage space, and make your data processing tasks more efficient.
Key Takeaways
- Python provides methods to delete all files from a directory or only those matching a specific pattern.
- The
os.listdir()
function can be used in combination withos.remove()
to delete all files from a directory. - The
glob
module can be used to delete files that match a specific pattern. - Python also allows deletion of files from all subfolders of a directory using the
iglob()
function from theglob
module.
Understanding Directory Deletion
While deleting files is an essential aspect of file system management, deleting directories is equally important. Python offers several methods for directory deletion. However, deleting directories can be slightly more complex than deleting files, especially when it comes to non-empty directories. Let’s delve deeper and explore how to delete directories in Python.
Deleting Empty Directories
Firstly, let’s focus on deleting empty directories. Python offers two methods for this: os.rmdir()
and pathlib.Path.rmdir()
. Both methods function similarly: they delete the directory specified in the path. Here’s how you can use them:
import os
os.rmdir('directory_path')
from pathlib import Path
directory_path = Path('directory_path')
directory_path.rmdir()
Deleting Non-Empty Directories
What if the directory is not empty? This is where the shutil
module proves useful. The shutil.rmtree()
method can delete a directory regardless of whether it’s empty or not. Here’s how you can use it:
import shutil
shutil.rmtree('directory_path')
When using shutil.rmtree()
, it’s important to note that it can raise an exception if it fails to delete the directory. This can occur due to various reasons, such as lack of permissions or the directory being used by another process. Therefore, it’s advisable to handle these exceptions using a try/except block, like so:
Example of exception handling with shutil.rmtree()
:
import shutil
try:
shutil.rmtree('directory_path')
except Exception as e:
print(f'Failed to delete directory: {e}')
import shutil
try:
shutil.rmtree('directory_path')
except Exception as e:
print(f'Failed to delete directory: {e}')
As you can see, Python’s shutil
module uniquely enables non-empty directory removal, unlike the os.rmdir()
function. This makes shutil.rmtree()
a powerful tool for managing your file system.
Deleting directories, especially non-empty ones, should be done with caution. Unlike deleting files, deleting a directory removes all its contents as well, which can lead to permanent data loss.
Before you delete a directory, ensure you no longer need any of the files or subdirectories inside it. If you’re uncertain, it’s a good practice to back up your data or move it to a different location before deleting the directory.
Key Takeaways
- Python provides methods to delete both empty and non-empty directories.
os.rmdir()
andpathlib.Path.rmdir()
can be used to delete empty directories.shutil.rmtree()
can delete both empty and non-empty directories.- Deleting directories should be done with caution to avoid permanent data loss.
Further Resources for Python File I/O Operations
- Python OS Module Mastery – Explore “os” functions for working with processes, including process IDs and status.
Python os.listdir() – Dive into the details of directory traversal and listing files using “os.listdir.”
Reading Files in Python – Explore Python’s file reading and data extraction techniques.
The
readlines
method in Python – This page details how to read all lines in a file.How to Read a Text File in Python – This article provides a step-by-step approach to reading text files in Python.
Guide on reading and writing files in Python – This tutorial gives beginners a straightforward introduction to reading and writing files in Python.
Conclusion
Navigating through the world of file and directory deletion in Python, we’ve covered substantial ground. We initiated our journey with the basics of file deletion, exploring the os
and pathlib
modules and their respective methods like os.remove()
and pathlib.Path.unlink()
. We then escalated to deleting multiple files from directories using an array of techniques, such as loops, list comprehensions, and the glob
module.
Our exploration didn’t stop there. We ventured further into the realm of directory deletion, discussing how to delete both empty and non-empty directories using methods like os.rmdir()
, pathlib.Path.rmdir()
, and shutil.rmtree()
.
Navigate the intricacies of Python with ease using our syntax map.
With a firm grasp of Python’s powerful deletion methods, you’re now equipped to manage your file system like a pro. So, don’t wait. Start leveraging these techniques and take your Python skills to the next level!