Java Project Ideas | Code Examples for All Skill Levels
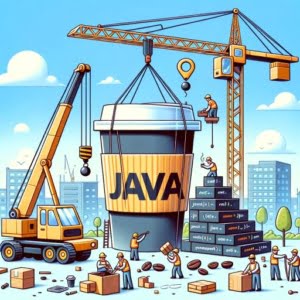
Are you on the hunt for Java project ideas to hone your skills or for your upcoming assignment? You’re not alone. Many developers find themselves in a similar situation, but Java, like a treasure chest, is brimming with project opportunities.
Think of Java projects as a multi-layered cake – offering you a variety of layers to explore and experiment with, from simple applications to complex systems.
This guide will walk you through a variety of Java projects, from basic to advanced, to help you get started. We’ll cover everything from creating simple applications like calculators and text editors, to more complex projects like chat applications and blog systems, as well as alternative approaches using different Java frameworks.
So, let’s dive in and start exploring the world of Java projects!
TL;DR: What are some Java project ideas?
Java project ideas can range from simple applications like calculators to more complex systems like chat applications, blog systems, or even games. For instance, you could start with a basic calculator project using Java like this:
Calculator calc = new Calculator(); calc.add(5, 10);
.
Here’s a simple example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Calculator calc = new Calculator();
System.out.println(calc.add(5, 10));
# Output:
# 15
In this example, we’ve created a simple calculator class with an add
method. We then create an instance of the Calculator class and use it to add 5 and 10, resulting in the output ’15’.
This is just a basic example of a Java project, but there’s so much more you can do with Java. Continue reading for more detailed examples and step-by-step guides for these projects.
Table of Contents
- Beginner’s Guide to Java Projects
- Intermediate Level Java Projects
- Expert Level: Exploring Java Frameworks
- Troubleshooting Java Projects: Common Issues and Solutions
- Understanding Java: The Powerhouse Behind Your Projects
- Expanding Horizons: Java in Larger Projects and Real-World Applications
- Wrapping Up: Mastering Java Projects from Basics to Advanced
Beginner’s Guide to Java Projects
Let’s start with some basic Java projects that are perfect for beginners. These projects will help you understand the fundamental concepts of Java and give you a solid foundation to build upon.
Building a Java Calculator
One of the simplest Java projects you can start with is a basic calculator. This project will help you understand how to create methods and use them in Java.
Here’s a simple example of how you could create a calculator in Java:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
public int multiply(int a, int b) {
return a * b;
}
public int divide(int a, int b) {
return a / b;
}
}
Calculator calc = new Calculator();
System.out.println(calc.add(5, 10));
System.out.println(calc.subtract(15, 5));
System.out.println(calc.multiply(3, 5));
System.out.println(calc.divide(10, 2));
# Output:
# 15
# 10
# 15
# 5
In this code block, we’ve created a Calculator class with four methods: add, subtract, multiply, and divide. Each method performs a different arithmetic operation. We then create an instance of the Calculator class and use it to perform some calculations. The results of these calculations are then printed to the console.
Creating a Basic Text Editor
Another basic Java project you can work on is a text editor. This project will help you understand how to work with strings in Java, as well as how to read from and write to files.
Here’s a simple example of how you could create a text editor in Java:
import java.io.*;
public class TextEditor {
public void writeToFile(String text, String filename) throws IOException {
BufferedWriter writer = new BufferedWriter(new FileWriter(filename));
writer.write(text);
writer.close();
}
public String readFromFile(String filename) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(filename));
StringBuilder text = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
text.append(line).append("
");
}
reader.close();
return text.toString();
}
}
TextEditor editor = new TextEditor();
editor.writeToFile("Hello, World!", "hello.txt");
System.out.println(editor.readFromFile("hello.txt"));
# Output:
# Hello, World!
In this code block, we’ve created a TextEditor class with two methods: writeToFile and readFromFile. The writeToFile method writes a string of text to a file, and the readFromFile method reads a file and returns its contents as a string. We then create an instance of the TextEditor class, use it to write some text to a file, and then read that text back from the file.
Intermediate Level Java Projects
Once you’ve mastered the basics, you can move on to more complex Java projects. These projects will help you understand more advanced concepts in Java and give you a chance to apply what you’ve learned in a more challenging context.
Building a Java Chat Application
One intermediate-level project you can work on is a chat application. This project will help you understand how to use Java for network programming, as well as how to handle multiple client connections simultaneously.
Here’s a simple example of how you could create a chat application server in Java:
import java.io.*;
import java.net.*;
public class ChatServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(1234);
Socket clientSocket = serverSocket.accept();
BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
String inputLine;
while ((inputLine = in.readLine()) != null) {
out.println(inputLine);
}
}
}
In this code block, we’ve created a simple chat server that listens for connections on port 1234. When a client connects, the server reads lines of text from the client and sends them back. This is a very basic example, but you could expand on this by adding support for multiple clients, user authentication, and more.
Creating a Java Blog System
Another intermediate-level project you can work on is a blog system. This project will help you understand how to use Java to interact with a database, as well as how to create a simple web interface for your blog.
Here’s a simple example of how you could create a blog post class in Java:
public class BlogPost {
private String title;
private String content;
private String author;
public BlogPost(String title, String content, String author) {
this.title = title;
this.content = content;
this.author = author;
}
// Getters and setters omitted for brevity
}
In this code block, we’ve created a BlogPost class with three properties: title, content, and author. This class could be used as part of a larger blog system, where each post is represented by an instance of the BlogPost class. You could expand on this by adding methods to save and load blog posts from a database, as well as a web interface to create, read, update, and delete blog posts.
Expert Level: Exploring Java Frameworks
As you progress further in your Java journey, you’ll encounter a variety of frameworks that can streamline your development process. In this section, we’ll discuss two popular Java frameworks: Spring and Hibernate.
Spring: The Comprehensive Framework
Spring is a powerful, feature-rich framework that can be used for developing complex Java applications. It offers a range of functionalities, from dependency injection to security and transaction management.
Here’s a simple example of a Spring application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In this code block, we’ve created a basic Spring Boot application. The @SpringBootApplication
annotation tells Spring that this is the main class for our application, and the SpringApplication.run
method starts the application.
Hibernate: Simplifying Database Interaction
Hibernate is another popular Java framework that simplifies database interaction. It maps Java classes to database tables and Java data types to SQL data types, making it easier to perform CRUD operations.
Here’s a simple example of a Hibernate entity class:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class BlogPost {
@Id
private int id;
private String title;
private String content;
// Getters and setters omitted for brevity
}
In this code block, we’ve created a BlogPost
class and annotated it with @Entity
to tell Hibernate that this class should be mapped to a database table. The @Id
annotation indicates that the id
field is the primary key for the table.
By exploring different Java frameworks like Spring and Hibernate, you can deepen your understanding of Java and enhance your project development skills.
Troubleshooting Java Projects: Common Issues and Solutions
As with any programming endeavor, working on Java projects can sometimes present challenges. Here, we’ll discuss some common issues you may encounter and their solutions.
Dealing with Null Pointer Exceptions
One of the most common issues you’ll face in Java is the dreaded NullPointerException
. This occurs when you try to access a method or field of an object that’s null.
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length());
}
}
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve attempted to get the length of a null string, resulting in a NullPointerException
. To fix this issue, always ensure that an object is not null before trying to access its methods or fields.
Handling Number Format Exceptions
Another common issue in Java is the NumberFormatException
. This occurs when you try to convert a string that doesn’t represent a valid number into a numeric type.
public class Main {
public static void main(String[] args) {
String str = "abc";
int num = Integer.parseInt(str);
}
}
# Output:
# Exception in thread "main" java.lang.NumberFormatException: For input string: "abc"
In this example, we’ve tried to convert a string that doesn’t represent a number into an integer, leading to a NumberFormatException
. To avoid this, always ensure that a string represents a valid number before trying to convert it.
Understanding Out of Memory Errors
Java programs can also run into OutOfMemoryError
. This happens when the Java Virtual Machine (JVM) can’t allocate an object because it’s out of memory, and no more memory could be made available by the garbage collector.
public class Main {
public static void main(String[] args) {
int[] arr = new int[Integer.MAX_VALUE];
}
}
# Output:
# Exception in thread "main" java.lang.OutOfMemoryError: Requested array size exceeds VM limit
In this example, we’ve attempted to create an array with a size greater than the maximum array size that the JVM supports, resulting in an OutOfMemoryError
. To prevent this, always ensure that your Java programs use memory efficiently and clean up unused objects to free up memory.
Understanding these common issues and their solutions can help you troubleshoot effectively when you encounter problems in your Java projects.
Understanding Java: The Powerhouse Behind Your Projects
Java is a high-level, object-oriented programming language, widely used for developing web-based, mobile, and desktop applications. Understanding its basic features and how it works is crucial for developing Java projects.
Java: Object-Oriented Programming Paradigm
Java follows the Object-Oriented Programming (OOP) paradigm, which allows you to create modular programs and reusable code. In Java, everything is an ‘Object’ which possesses some state and behavior and is manipulated through ‘classes’.
public class Car {
// State or Field
String color;
// Behavior or Method
void startCar() {
System.out.println("Car Started");
}
}
Car myCar = new Car();
myCar.startCar();
# Output:
# Car Started
In this example, ‘Car’ is a class that has a state ‘color’ and a method ‘startCar’. We create an object ‘myCar’ from the class and invoke the method on it.
Java and Portability
One of the key features of Java is its portability. Java follows the principle of ‘Write Once, Run Anywhere’ (WORA), meaning compiled Java code (bytecode) can run on any platform that has a Java Virtual Machine (JVM), without the need for recompilation.
Java’s Robustness
Java provides a robust environment by emphasizing early error checking, runtime error checking, and eliminating situations prone to errors. It’s strongly typed, which means you, as a programmer, must explicitly declare the type of every variable you use.
int number = 10; // Correct
num = 10; // Compile-time error
In the first line, we’ve correctly declared the variable ‘number’ as an integer. In the second line, we get a compile-time error since the variable ‘num’ has not been declared before.
Java’s Multithreading Feature
Java supports multithreading, which allows concurrent execution of two or more parts of a program for maximum utilization of CPU. Each part of such a program is called a thread, and each thread defines a separate path of execution.
Understanding these fundamentals of Java will help you in developing efficient and effective Java projects.
Expanding Horizons: Java in Larger Projects and Real-World Applications
Java is not limited to academic or small-scale projects; it’s extensively used in larger projects and real-world applications. Its robustness, scalability, and security make it an excellent choice for various domains.
Java for Web Development
Java is a popular choice for web development, thanks to frameworks like Spring and JavaServer Faces (JSF). These frameworks simplify the process of creating complex, feature-rich web applications.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
In this example, we’ve created a simple Spring Boot web application that returns ‘Hello, World!’ when you access the ‘/hello’ URL.
Java for Android Development
Java is also the primary language for Android development. With Java, you can create anything from games to social media apps, productivity tools, and more for the Android platform.
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textView = new TextView(this);
textView.setText("Hello, Android");
setContentView(textView);
}
}
In this example, we’ve created a simple Android application that displays ‘Hello, Android’ on the screen.
Further Resources for Advancing in Java Projects
To further your understanding and skills in Java, consider exploring these resources:
- Dive into Java: Core Concepts Tutorial – Understand Java methods, parameters, and return types for modular code.
Java Hello World Program – Get started with Java programming by creating a simple “Hello, World!” program.
Java Code Testing Online – Discover features offered by online Java compilers for interactive coding experiences.
Oracle’s Official Java Tutorials cover everything from the basics to more advanced topics in Java.
Baeldung offers a wide range of Java tutorials on Spring, Hibernate, and more.
Java Code Geeks offers a wealth of Java tutorials, examples, and articles on various Java topics.
Wrapping Up: Mastering Java Projects from Basics to Advanced
In this comprehensive guide, we’ve delved deep into the world of Java projects. We’ve explored a variety of projects, from basic applications like calculators to more complex systems like chat applications and blog systems, and even dived into alternative approaches using different Java frameworks.
We began with the basics, guiding you through creating simple projects like calculators and text editors, complete with code examples and explanations. We then ventured into intermediate territory, discussing more complex projects like chat applications and blog systems. We also explored expert-level approaches, introducing you to the world of Java frameworks like Spring and Hibernate.
Along the way, we tackled common issues you might face while working on Java projects, providing solutions to help you overcome these challenges. We also looked at the fundamentals of Java, giving you a deeper understanding of the language powering your projects.
Here’s a quick comparison of the projects we’ve discussed:
Project | Complexity | Skills Developed |
---|---|---|
Calculator Application | Basic | Understanding of methods in Java |
Text Editor | Basic | Working with strings and file I/O in Java |
Chat Application | Intermediate | Network programming in Java |
Blog System | Intermediate | Database interaction in Java |
Using Spring Framework | Expert | Understanding of Spring framework |
Using Hibernate Framework | Expert | Understanding of Hibernate framework |
Whether you’re a beginner just starting out with Java or an experienced developer looking to level up your skills, we hope this guide has given you a wealth of ideas for your next Java project.
With its versatility and wide range of application, Java offers endless possibilities for project development. Now, you’re well-equipped to explore these possibilities. Happy coding!