Java Online Compilers and Playgrounds | Proper Usage Guide
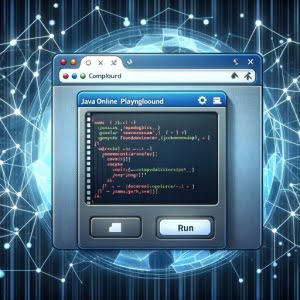
Ever felt like you’re wrestling with compiling and running your Java code online? You’re not alone. Many developers find Java online compilers or playgrounds a bit daunting. Think of Java online compilers as a virtual sandbox – a sandbox that allows you to experiment with Java coding without any setup.
Online compilers are a powerful way to test and debug your Java scripts, making them extremely popular for learning Java, practicing coding problems, and quick prototyping.
This guide will walk you through the process of using an online Java compiler, from basic to advanced features. We’ll cover everything from writing simple Java code, compiling and running the code, to dealing with compilation errors and even troubleshooting common issues.
Let’s kick things off and learn to use Java online compiler / playground!
TL;DR: How Do I Use a Java Online Compiler or Playground?
There are various online compilers available for free use, such as
jdoodle.com
,onecompiler.com
,dev.java playground
, andtutorialspoint.com
. To use these Java online compilers or playground, you simply write your Java code in the provided editor, then click ‘Run’ or ‘Compile’. Here’s a basic example using JDoodle:
// Write your code
public class Main {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
// Click 'Run'
# Output:
# 'Hello, World!'
In this example, we’ve written a simple Java program that prints ‘Hello, World!’ to the console. We then run the program using the ‘Run’ button in JDoodle, an online Java compiler. The output of the program is displayed in the console.
This is a basic way to use a Java online compiler or playground, but there’s much more to learn about using these tools effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- A Beginner’s Guide to Using a Java Online Compiler
- Delving Deeper: Advanced Features of Java Online Compilers
- Exploring Alternatives: Comparing Online Java Compilers
- Troubleshooting Common Issues in Java Online Compilers
- The Fundamentals: Understanding Java and Online Compilers
- Expanding Horizons: Beyond the Java Online Compiler
- Wrapping Up: Java Online Compilers
A Beginner’s Guide to Using a Java Online Compiler
If you’re new to Java or online compilers, fear not. Here’s a simple step-by-step guide to help you get started with using a Java online compiler or playground.
Step 1: Write Your Code
First things first, you’ll need to write your Java code. In the online compiler’s editor, type in your code. Here’s a simple Java program that prints ‘Hello, Java!’:
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Java!');
}
}
Step 2: Compile and Run Your Code
Once you’ve written your code, it’s time to compile and run it. Look for a button labeled ‘Run’ or ‘Compile’ and click it. The online compiler will then process your code.
Step 3: View the Output
After your code has been run, the output will be displayed in the console or output section of the compiler. For our ‘Hello, Java!’ program, the output should be:
# Output:
# 'Hello, Java!'
And there you have it! You’ve successfully written, compiled, and run a simple Java program using an online compiler. Remember, practice makes perfect, so don’t be afraid to experiment with different codes and see the results.
Delving Deeper: Advanced Features of Java Online Compilers
Now that you’re comfortable with the basics of using a Java online compiler, let’s explore some of the more complex features these tools offer. These advanced features can make your coding experience much more efficient and enjoyable.
Using Different Java Versions
Different online compilers support different versions of Java. This flexibility allows you to test your code under various Java versions. Here’s how you can switch between Java versions in JDoodle:
// Choose Java version from dropdown
// Write your code
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Java 11!');
}
}
// Click 'Run'
# Output:
# 'Hello, Java 11!'
In this example, we’ve selected Java 11 from the dropdown menu before running our code. The output confirms that the code was run using Java 11.
Importing Libraries
Online compilers also allow you to import various Java libraries. This feature is essential when you want to use specific Java classes or methods that aren’t part of the standard Java library. Here’s an example of importing and using the Java ArrayList
class:
// Import ArrayList from Java library
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add('Hello, Java!');
System.out.println(list.get(0));
}
}
# Output:
# 'Hello, Java!'
In this example, we’ve imported the ArrayList
class and used it to create a list. We then added a string to the list and printed it to the console.
Debugging Code
Online compilers often come with built-in debugging tools. These tools can help you identify and fix any errors in your code. When an error occurs, the compiler will highlight the problematic line and provide an error message. Here’s an example of a runtime error:
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]);
}
}
# Output:
# Exception in thread 'main' java.lang.ArrayIndexOutOfBoundsException: 3
In this example, we’ve attempted to access an index of the array that doesn’t exist. The compiler has thrown an ArrayIndexOutOfBoundsException
, indicating that we’ve tried to access an array element beyond its size.
These advanced features of Java online compilers can greatly enhance your coding experience, making it easier to write, test, and debug your Java code.
Exploring Alternatives: Comparing Online Java Compilers
While JDoodle is a powerful tool for compiling Java code online, it’s by no means the only option. Other popular online Java compilers include Repl.it and Codiva. Let’s compare these platforms to help you decide which might be the best fit for your needs.
JDoodle: A Versatile Java Playground
JDoodle is known for its simplicity and versatility. It supports multiple programming languages, including various versions of Java. JDoodle also allows you to save and share your code snippets, making it a great tool for collaboration.
// JDoodle example
public class Main {
public static void main(String[] args) {
System.out.println('Hello, JDoodle!');
}
}
# Output:
# 'Hello, JDoodle!'
In this example, we’re using JDoodle to compile and run a simple Java program that prints ‘Hello, JDoodle!’.
Repl.it: Real-Time Collaboration and More
Repl.it offers a more feature-rich environment. In addition to compiling and running code, Repl.it supports real-time collaboration, version control, and even deployment of web applications.
// Repl.it example
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Repl.it!');
}
}
# Output:
# 'Hello, Repl.it!'
Here, we’re using Repl.it to compile and run a Java program that prints ‘Hello, Repl.it!’.
Codiva: A Compiler for Education
Codiva is specifically designed for educational purposes. It offers a clean, distraction-free interface, and it automatically compiles your code as you type, providing instant feedback.
// Codiva example
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Codiva!');
}
}
# Output:
# 'Hello, Codiva!'
In this example, we’re using Codiva to compile and run a Java program that prints ‘Hello, Codiva!’.
Each of these online Java compilers has its strengths and weaknesses. Your choice will depend on your specific needs. Whether you value real-time collaboration, auto-compilation, or a simple interface, there’s an online Java compiler out there for you.
Troubleshooting Common Issues in Java Online Compilers
Like any tool, Java online compilers are not without their quirks and challenges. Here, we’ll explore some common issues you might encounter when using an online Java compiler and offer some tips for resolving them.
Dealing with Compilation Errors
Compilation errors occur when your code violates the syntax rules of Java. These errors prevent your code from being compiled and run. Here’s an example of a common compilation error:
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Java'
}
}
# Output:
# Error:(3, 35) java: ';' expected
In this example, we’ve forgotten to close the parentheses after the println
statement, resulting in a compilation error. The compiler indicates that a semicolon is expected, pointing us to the exact location of the error.
Handling Runtime Errors
Runtime errors, on the other hand, occur during the execution of your code. These errors are usually due to logical mistakes in your code. Here’s an example of a common runtime error:
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]);
}
}
# Output:
# Exception in thread 'main' java.lang.ArrayIndexOutOfBoundsException: 3
In this example, we’ve attempted to access an index of the array that doesn’t exist. The compiler throws an ArrayIndexOutOfBoundsException
, indicating that we’ve tried to access an array element beyond its size.
Understanding Online Compiler Limitations
It’s also important to understand the limitations of online compilers. For instance, online compilers may not support all Java libraries or features. They may also impose restrictions on execution time and memory usage. Always check the documentation of your chosen online compiler for any limitations.
Remember, troubleshooting is an integral part of the coding process. Don’t be discouraged by errors or limitations. Instead, use them as learning opportunities to enhance your coding skills.
The Fundamentals: Understanding Java and Online Compilers
To fully appreciate the convenience and functionality of Java online compilers or playgrounds, it’s helpful to understand the basics of Java and how online compilers work.
The Magic Behind Java Compilation
Java is a compiled language. This means that Java code, written in human-readable text, must be translated into machine code before it can be executed by a computer. This translation process is called compilation.
Here’s a simple illustration of the compilation process:
// Java code (human-readable)
public class Main {
public static void main(String[] args) {
System.out.println('Hello, Java!');
}
}
// After compilation (machine code)
01101000 01100101 01101100 01101100 01101111 00101100 00100000 01101010 01100001 01110110 01100001 00100001
In this example, the Java code is a simple program that prints ‘Hello, Java!’. After compilation, the Java code is translated into machine code, represented here as binary numbers.
The Power of Online Compilers
Traditionally, you would need to install a Java Development Kit (JDK) on your computer to compile and run Java code. This process can be time-consuming and requires a certain level of technical knowledge.
Java online compilers simplify this process. They allow you to write, compile, and run Java code directly in your web browser, without any setup. This makes them an excellent tool for learning Java, testing code snippets, or even prototyping applications.
In addition, online compilers often come with additional features, such as code sharing, collaboration tools, and support for multiple programming languages. These features make online compilers a versatile tool for both beginners and experienced developers.
By understanding the fundamentals of Java and online compilers, you can better appreciate the convenience and power of Java online compilers or playgrounds.
Expanding Horizons: Beyond the Java Online Compiler
Java online compilers or playgrounds are not only tools for coding; they’re also platforms for learning, practicing, and prototyping. Let’s delve into these applications and how they can enhance your Java journey.
Learning Java with Online Compilers
Online compilers are a boon for new learners. They offer a hassle-free environment to learn Java, eliminating the need for any setup. You can focus on learning the language and its concepts, rather than worrying about installing and configuring a development environment.
Practicing Coding Problems
Java online compilers are also great for practicing coding problems. Whether you’re preparing for a coding interview or participating in a coding challenge, these compilers allow you to compile and run your solutions quickly and easily.
Quick Prototyping
Got an idea for a Java application? Online compilers can help you bring it to life. You can quickly write and test your code, making these compilers an excellent tool for prototyping.
Exploring Related Topics
Once you’re comfortable with using a Java online compiler, you might want to explore related topics. For instance, you could learn about Java Integrated Development Environments (IDEs) or setting up a local Java development environment. These topics can provide a deeper understanding of Java development.
Further Resources for Java Online Compilers
To further your knowledge and skills, check out these compilers:
- Mastering Essential Java Techniques – Dive into Java syntax, data types, and control flow with this beginner-friendly tutorial.
Java Projects Overview – Discover a variety of Java project ideas and inspirations for your next coding endeavor.
Java Code Editing Tools – Learn how to use online Java editors for collaborative coding, learning, or quick prototyping.
OneCompiler Online Java Compiler offers an online Java compiler to write and execute Java code from the browser.
Compile Java Online by Tutorialspoint provides an online Java compiler to write and compile Java programs.
JDoodle Online Java Compiler offers an online Java compiler to write, compile, and run Java code from their browser.
Remember, mastering a tool like a Java online compiler is a journey. Keep exploring, learning, and experimenting, and you’ll continue to grow as a Java developer.
Wrapping Up: Java Online Compilers
In this comprehensive guide, we’ve journeyed through the ins and outs of using a Java online compiler or playground. We’ve explored its capabilities and how it can be a powerful tool for learning, practicing, and prototyping Java applications.
We began with the basics, learning how to write, compile, and run simple Java programs. We then ventured into more advanced territory, exploring features such as using different Java versions, importing libraries, and debugging code. Along the way, we tackled common issues you might face when using an online Java compiler, providing you with solutions and workarounds for each issue.
We also compared different online Java compilers, including JDoodle, Repl.it, and Codiva, giving you a sense of the broader landscape of online Java compilers and playgrounds. Here’s a quick comparison of these platforms:
Platform | Versatility | Real-Time Collaboration | Auto-Compilation |
---|---|---|---|
JDoodle | High | Moderate | Moderate |
Repl.it | High | High | Moderate |
Codiva | Moderate | Low | High |
Whether you’re just starting out with Java or you’re looking to level up your coding skills, we hope this guide has given you a deeper understanding of Java online compilers and their capabilities.
With the ability to write, compile, and run Java code directly in your web browser, Java online compilers offer a convenient and powerful tool for developers of all levels. Now, you’re well equipped to harness the power of these tools. Happy coding!