Mockito Spy Function | Guide to Java Code Inspection
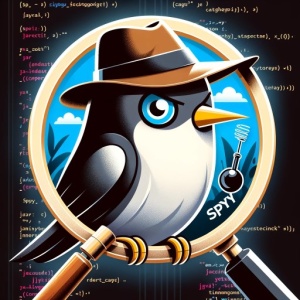
Are you finding it challenging to navigate Mockito’s spy function? Like a secret agent, Mockito spy can help you keep an eye on your code’s behavior, but it can be a bit elusive if you’re not familiar with it. Many developers find themselves in a bind when it comes to using Mockito spy in their Java unit tests, but we’re here to help.
Think of Mockito spy as a stealthy observer – it allows you to keep track of your code’s behavior without interfering with it. It’s a powerful tool in your Java unit testing arsenal, but it requires a bit of finesse to use effectively.
In this guide, we’ll walk you through the process of using Mockito spy in your Java unit tests, from the basics to more advanced techniques. We’ll cover everything from creating a spy object using Mockito spy, discussing its pros and cons, to troubleshooting common issues and their solutions.
So, let’s get started and start mastering Mockito spy!
TL;DR: What is the Mockito Spy?
Mockito.spy()
is a function that creates a spy of a real object with the syntax,[dataType] spy = Mockito.spy([variabletoSpy]);
. The spy calls all methods of the real object unless they’re stubbed. This is a powerful feature that allows you to observe the behavior of your code in a non-intrusive way.
Here’s a simple example:
List list = new LinkedList();
List spy = Mockito.spy(list);
In this example, we create a LinkedList object and then create a spy of it using Mockito.spy(). This spy object behaves just like the original list, but it also allows us to track its interactions and even change its behavior if we want to.
This is just a basic introduction to Mockito spy, but there’s much more to learn about it. Continue reading for more detailed usage and advanced scenarios.
Table of Contents
- Creating a Spy Object with Mockito Spy
- Partial Mocking and Stubbing with Mockito Spy
- Exploring Alternatives to Mockito Spy
- Troubleshooting Common Issues with Mockito Spy
- Understanding Mockito and Java Unit Testing
- Mockito Spy in Larger Projects
- Mockito Spy and Other Mockito Features
- Unit Testing Best Practices
- Wrapping Up: Mockito Spy
Creating a Spy Object with Mockito Spy
To start with, let’s create a spy object using Mockito spy. This is the most basic use of Mockito spy and a good starting point for beginners. Here’s a simple code example:
List<String> list = new LinkedList<>();
List<String> spy = Mockito.spy(list);
spy.add("Mockito");
spy.add("Spy");
System.out.println(spy.get(0)); // Mockito
System.out.println(spy.get(1)); // Spy
# Output:
# Mockito
# Spy
In the example above, we first create a LinkedList object. We then create a Mockito spy of this list. When we add elements to the spy object, it behaves just like the original list. We can see this when we print the elements of the spy list, which outputs ‘Mockito’ and ‘Spy’.
Pros and Cons of Using Mockito Spy
Using Mockito spy has its advantages and disadvantages. On the positive side, it allows you to observe the behavior of your code in a non-intrusive way. You can track the interactions of your code and even change its behavior if needed. This can be very useful for debugging and testing.
However, there are also some downsides to using Mockito spy. One of the main drawbacks is that it can lead to overuse of spies, which can make your tests more complicated and harder to understand. It’s also worth noting that Mockito spy calls the real methods of the object, which can lead to unexpected behavior if not handled carefully. It’s therefore crucial to use Mockito spy judiciously and only when necessary.
Partial Mocking and Stubbing with Mockito Spy
As you become more comfortable with Mockito spy, you can start exploring more complex uses such as partial mocking and stubbing spy methods. Let’s dive into these concepts.
Partial Mocking with Mockito Spy
Partial mocking is a technique where you mock only some methods of an object while keeping the real behavior of others. This can be useful when you want to isolate some parts of your code for testing. Here’s an example of how you can do this with Mockito spy:
List<String> list = new LinkedList<>();
List<String> spy = Mockito.spy(list);
// Use doReturn for partial mocking
Mockito.doReturn("Mockito Spy").when(spy).get(0);
spy.add("Mockito");
spy.add("Spy");
System.out.println(spy.get(0)); // Mockito Spy
System.out.println(spy.get(1)); // Spy
# Output:
# Mockito Spy
# Spy
In this example, we’re using Mockito’s doReturn
method to change the behavior of the get
method on our spy object. Even though we add “Mockito” to the spy list, when we call spy.get(0)
, it returns “Mockito Spy” instead. This is because we have partially mocked the get
method to always return “Mockito Spy” for the index 0.
Stubbing Spy Methods with Mockito
Stubbing spy methods is another powerful feature of Mockito spy. It allows you to change the behavior of a spy object for certain methods. Here’s an example:
List<String> list = new LinkedList<>();
List<String> spy = Mockito.spy(list);
// Stubbing the size method
Mockito.when(spy.size()).thenReturn(100);
System.out.println(spy.size()); // 100
# Output:
# 100
In the above example, we stub the size
method of our spy object to always return 100, regardless of the actual size of the list. When we call spy.size()
, it returns 100, even though the list is actually empty.
By mastering these advanced uses of Mockito spy, you can gain more control over your code’s behavior and make your Java unit tests more robust and reliable.
Exploring Alternatives to Mockito Spy
While Mockito spy is a powerful tool for Java unit testing, it’s not the only one. There are other ways to achieve similar results without using Mockito spy. Two such alternatives are using mock objects or real objects. Let’s discuss these in detail.
Using Mock Objects
Mock objects can serve as a viable alternative to Mockito spy. They simulate the behavior of real objects in controlled ways. Here’s an example of how you can use a mock object in place of a spy:
List<String> mockList = Mockito.mock(LinkedList.class);
Mockito.when(mockList.get(0)).thenReturn("Mockito");
Mockito.when(mockList.get(1)).thenReturn("Spy");
System.out.println(mockList.get(0)); // Mockito
System.out.println(mockList.get(1)); // Spy
# Output:
# Mockito
# Spy
In this example, we create a mock object of LinkedList using Mockito.mock()
. We then stub the get
method to return specific values. When we call mockList.get(0)
and mockList.get(1)
, it returns “Mockito” and “Spy” respectively, just like how we stubbed it.
Using Real Objects
Real objects can also be used to achieve the same results as Mockito spy. This approach can be useful when you want to test the actual behavior of your code without any mocking or spying. Here’s an example:
List<String> list = new LinkedList<>();
list.add("Mockito");
list.add("Spy");
System.out.println(list.get(0)); // Mockito
System.out.println(list.get(1)); // Spy
# Output:
# Mockito
# Spy
In this example, we use a real LinkedList object without any spying or mocking. We add elements to the list and retrieve them using the get
method, just like in a regular Java program.
While Mockito spy can be very useful, it’s important to know that there are alternatives available. Depending on your specific needs and the complexity of your code, you might find mock objects or real objects to be more suitable for your Java unit tests.
Troubleshooting Common Issues with Mockito Spy
While Mockito spy is a powerful tool, like any other tool, it can sometimes lead to unexpected results. Let’s discuss some common issues you might face when using Mockito spy and how to resolve them.
Unexpected Behavior Due to Real Method Calls
One of the key features of Mockito spy is that it calls the real methods of an object. While this can be useful, it can also lead to unexpected behavior if not handled carefully. Let’s consider an example:
List<String> list = new LinkedList<>();
List<String> spy = Mockito.spy(list);
Mockito.when(spy.size()).thenReturn(100);
spy.add("Mockito");
spy.add("Spy");
System.out.println(spy.size()); // 100
# Output:
# 100
In this example, we stub the size
method of our spy object to return 100. However, we then add two elements to the spy list. Despite this, when we call spy.size()
, it still returns 100. This is because the real add
method of the list is called, but the stubbed size
method isn’t aware of this.
To avoid this issue, you can use the doReturn
method for stubbing, which is recommended for stubbing methods on spy objects. Here’s how to do it:
List<String> list = new LinkedList<>();
List<String> spy = Mockito.spy(list);
Mockito.doReturn(100).when(spy).size();
spy.add("Mockito");
spy.add("Spy");
System.out.println(spy.size()); // 100
# Output:
# 100
In this version of the code, even though we add elements to the list, the size
method still returns 100 as stubbed.
By being aware of these potential issues and knowing how to resolve them, you can use Mockito spy more effectively in your Java unit tests.
Understanding Mockito and Java Unit Testing
Before diving deeper into Mockito spy, it’s crucial to understand the fundamental concepts underlying Mockito and unit testing in Java. This understanding will provide a solid foundation for mastering Mockito spy.
Mockito: A Powerful Tool for Java Unit Testing
Mockito is a popular mocking framework used in conjunction with testing frameworks like JUnit. It simplifies the process of creating mock objects in unit tests, allowing developers to focus on the behavior of the system under test rather than setting up complex test environments.
// Creating a mock object with Mockito
List<String> mockList = Mockito.mock(LinkedList.class);
In the example above, we’re using Mockito to create a mock object of a LinkedList. This mock object can then be used in our unit tests, behaving in ways we define.
The Role of Mock Objects and Spy Objects in Unit Testing
In unit testing, mock objects play a crucial role. They simulate the behavior of complex real objects, allowing you to focus on the unit of code you’re testing. Mock objects can be controlled to return specific values or behave in certain ways, making them predictable and reliable for testing.
Spy objects, on the other hand, are a special type of mock objects. They are based on real objects, and by default, they call the real methods of the object. However, their behavior can be controlled just like regular mock objects.
// Creating a spy object with Mockito
List<String> list = new LinkedList<>();
List<String> spyList = Mockito.spy(list);
In the example above, we’re creating a spy object based on a real LinkedList. This spy object behaves just like the real list, but we can also control its behavior as needed for our tests.
Understanding these fundamental concepts is key to mastering Mockito spy and using it effectively in your Java unit tests.
Mockito Spy in Larger Projects
In larger projects, Mockito spy can play a significant role in your testing strategies. It allows you to observe the behavior of specific parts of your code, which can be particularly useful in complex systems where different components interact with each other.
For instance, you might have a service that interacts with a database. By spying on this service, you can see how it interacts with the database, which methods it calls, and what data it retrieves or modifies. This can help you understand how your code behaves in real-world scenarios and identify any potential issues.
Mockito Spy and Other Mockito Features
While Mockito spy is a powerful tool, it’s just one of many features offered by Mockito. Other features like mock objects, stubbing, and argument matchers can also be used in conjunction with Mockito spy to create robust and comprehensive unit tests.
For example, you might use Mockito spy to observe a real object, while using mock objects to simulate the behavior of other components in your system. By combining these features, you can create a controlled testing environment that closely mirrors your actual system.
Unit Testing Best Practices
Finally, it’s important to remember that Mockito spy is a tool to aid in unit testing, and like any tool, it’s most effective when used properly. Following unit testing best practices can help you get the most out of Mockito spy.
For instance, it’s generally recommended to keep your unit tests small and focused. Each test should cover a single aspect of your code’s behavior. If you find yourself needing to spy on multiple objects or methods in a single test, it might be a sign that your test is too complex or that your code needs refactoring.
Further Resources for Mastering Mockito Spy
If you’re interested in learning more about Mockito spy and how to use it effectively in your Java unit tests, here are some resources that might help:
- Your Solution for Java Code Quality: Java Code Testing – Learn how to improve code quality through testing.
Understanding Mockito Framework – Learn about the mocking of dependencies and behavior verification in Java tests.
Mockito Verify in Java explains Mockito verify, for verifying interactions between mock objects and code in Java unit tests.
Mockito’s Official Documentation provides a comprehensive overview of all the features Mockito offers.
Baeldung’s Guide on Mockito covers a wide range of topics, including how to use Mockito spy.
JUnit & Mockito Crash Course on Udemy covers both JUnit and Mockito and provides a hands-on approach to learning.
Wrapping Up: Mockito Spy
In this comprehensive guide, we’ve delved deep into the world of Mockito spy, a powerful tool for observing your Java code’s behavior during unit testing.
We began with the basics, understanding how to create a spy object using Mockito spy and discussing its pros and cons. We then moved on to more advanced usage, exploring partial mocking and stubbing with Mockito spy. We also covered alternative approaches to Mockito spy, discussing the use of mock objects and real objects for similar results.
Along the way, we addressed common issues that you might encounter when using Mockito spy, such as unexpected behavior due to real method calls. We provided solutions and tips to help you navigate these challenges and use Mockito spy more effectively.
To give you a sense of the broader landscape, here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Mockito Spy | High | Moderate | When you need to observe and control real objects |
Mock Objects | Moderate | Low | When you need to simulate the behavior of other components |
Real Objects | Low | Low | When you want to test the actual behavior of your code |
Whether you’re just starting out with Mockito spy or you’re looking to level up your Java unit testing skills, we hope this guide has provided you with a deeper understanding of Mockito spy and its capabilities.
Mastering Mockito spy can significantly enhance your ability to write effective and robust unit tests in Java. With this guide, you’re now well-equipped to leverage the power of Mockito spy in your projects. Happy coding!