How to Write to Files in Java: A Step-by-Step Guide
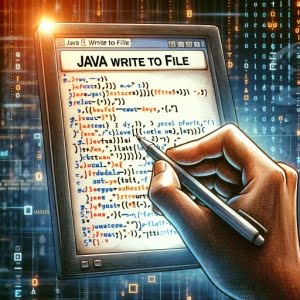
Are you finding it challenging to write data to a file in Java? You’re not alone. Many developers find themselves in a similar predicament, but there’s a tool that can simplify this process for you.
Think of Java as a skilled scribe, capable of recording your data in any file you wish. It comes equipped with a variety of classes and methods that can be used to write data to files, making it a versatile tool for your programming needs.
This guide will walk you through the process of writing to files in Java, from the basics to more advanced techniques. We’ll explore Java’s core file writing functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering file writing in Java!
TL;DR: How Do I Write to a File in Java?
To write to a file in Java, you can create a
PrintWriter
instance with the syntax,PrintWriter writer = new PrintWriter('output.txt');
. You may also utilize aFileWriter
instance with the syntax,FileWriter writer = new FileWriter("output.txt");
. These classes provide simple and efficient methods for writing text to a file.
Here’s a simple example using PrintWriter
:
PrintWriter writer = new PrintWriter('output.txt');
writer.println('Hello, world!');
writer.close();
# Output:
# This will write the string 'Hello, world!' to a file named 'output.txt'.
In this example, we create a PrintWriter
object and pass the name of the file we want to write to (‘output.txt’) to the constructor. We then use the println
method to write a string to the file, and finally close the writer using the close
method.
This is a basic way to write to a file in Java, but there’s much more to learn about file I/O in Java. Continue reading for more detailed explanations and examples.
Table of Contents
Basic File Writing in Java
Java provides several classes for writing to files, but for beginners, the PrintWriter
and FileWriter
classes are a good starting point. They offer simple and intuitive methods for writing text to files.
Using PrintWriter
PrintWriter
is a high-level class that provides methods to write different data types in a convenient way. Here’s a simple example:
import java.io.PrintWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
PrintWriter writer = new PrintWriter("output.txt");
writer.println("Hello, world!");
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# 'Hello, world!' is written to 'output.txt'
In this block of code, we’re creating a PrintWriter
object and passing the name of the file we want to write to (‘output.txt’) to the constructor. The println
method is then used to write a string to the file. After writing, it’s important to close the writer using the close
method to free up system resources.
One advantage of PrintWriter
is its ability to write different data types (int, long, object etc.) conveniently. However, it doesn’t write directly to a file but rather writes to a buffer and the buffer then writes to the file, which can be slower in some scenarios.
Using FileWriter
FileWriter
is another class used to write character-oriented data to a file. Here’s a simple example of how to use FileWriter
:
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
FileWriter writer = new FileWriter("output.txt");
writer.write("Hello, world!");
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# 'Hello, world!' is written to 'output.txt'
Here, we’re creating a FileWriter
object and writing a string to the file using the write
method. FileWriter
writes directly to the file, which can be faster than PrintWriter
in scenarios where writing speed is crucial.
However, FileWriter
only has methods to write arrays of characters. To write different data types (like int, long etc.), you’d need to convert them to strings first, which can be inconvenient.
In both cases, you’ll notice we’re handling IOException
– this is a potential pitfall when writing to files in Java. If the file can’t be opened for writing (for example, if it’s read-only), an IOException
will be thrown. It’s good practice to catch and handle this exception whenever you’re working with file I/O.
Advanced File Writing Techniques in Java
As you become more comfortable with file writing in Java, you might want to explore more advanced techniques like writing to binary files or writing in a specific character encoding.
Writing to Binary Files
Binary files are not human-readable but they allow you to write data in a compact form. In Java, you can use the DataOutputStream
class to write primitive Java data types to an output stream in a portable way. Here’s an example:
import java.io.DataOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
FileOutputStream fileOut = new FileOutputStream("output.bin");
DataOutputStream dataOut = new DataOutputStream(fileOut);
dataOut.writeInt(123);
dataOut.writeDouble(123.45);
dataOut.writeBoolean(true);
dataOut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# 'output.bin' file is created with binary data
In this example, we first create a FileOutputStream
object for the file we want to write to. We then create a DataOutputStream
from the FileOutputStream
. We can then use DataOutputStream
methods like writeInt
, writeDouble
, writeBoolean
etc. to write data in a binary format.
Writing in a Specific Character Encoding
Java uses Unicode system to represent characters, but it allows you to write to files using any character encoding that your system supports. You can do this using the OutputStreamWriter
class, which is a bridge from character streams to byte streams. Here’s how you can write to a file using UTF-8 encoding:
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) {
try {
FileOutputStream fileOut = new FileOutputStream("output.txt");
OutputStreamWriter writer = new OutputStreamWriter(fileOut, StandardCharsets.UTF_8);
writer.write("Hello, world!");
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# 'Hello, world!' is written to 'output.txt' in UTF-8 encoding
In this example, we’re creating an OutputStreamWriter
with UTF-8 encoding from a FileOutputStream
. We then write a string to the file using this writer. The resulting file will be encoded in UTF-8.
Exploring Alternative File Writing Techniques in Java
Java provides alternative ways to write to files, offering more flexibility and efficiency. One such method is using the Files
class from the java.nio.file
package. This class provides several methods for operations on file/directory paths.
Using the Files Class
The Files
class allows you to write to a file in a single line of code. Here’s an example:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) {
String data = "Hello, world!";
try {
Files.write(Paths.get("output.txt"), data.getBytes(StandardCharsets.UTF_8));
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# 'Hello, world!' is written to 'output.txt'
In this example, we’re using the Files.write
method, which writes a sequence of bytes to a file. We first convert our string to bytes using the getBytes
method of the string class, and then write these bytes to the file.
The Files.write
method is atomic, meaning that the write operation is done in a single step. This can be beneficial in multi-threading environments where you don’t want other threads to interfere with the writing process.
However, this method is not suitable for large files, as it requires all data to be held in memory. For large files, you’d still want to use FileWriter
or FileOutputStream
.
In all these examples, you’ll notice that we’re handling IOException
. This is a common issue when writing to files in Java, and it’s always good practice to catch and handle this exception whenever you’re working with file I/O.
Troubleshooting Common Issues in Java File Writing
While Java provides robust tools for file writing, it’s not uncommon to encounter issues. One of the most common problems is IOExceptions
. Let’s discuss how to handle these exceptions and some best practices for error handling in file I/O.
Handling IOExceptions
IOException
is a checked exception that can be thrown when an I/O operation fails for some reason. This could be due to a file being inaccessible, the disk being full, or even a network connection dropping while reading a file over a network.
Here’s an example of how to catch and handle an IOException
when writing to a file:
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
FileWriter writer = new FileWriter("output.txt");
writer.write("Hello, world!");
writer.close();
} catch (IOException e) {
System.out.println("An error occurred while writing to the file.");
e.printStackTrace();
}
}
}
# Output:
# If an error occurs, 'An error occurred while writing to the file.' is printed and the stack trace of the exception is printed.
In this example, we’re writing to a file inside a try
block. If an IOException
occurs during this operation, the control is transferred to the catch
block where we print a custom error message and the stack trace of the exception.
Best Practices for Error Handling in File I/O
When dealing with file I/O in Java, it’s important to follow some best practices for error handling:
- Always catch and handle
IOExceptions
. Ignoring these exceptions can lead to unpredictable results and hard-to-debug issues. Close your streams in a
finally
block or use a try-with-resources statement. This ensures that your streams are closed even if an exception occurs, which can prevent resource leaks.Provide meaningful error messages. If an error occurs, provide a message that can help the user (or you) understand what went wrong.
Understanding Java File I/O Fundamentals
Before we delve further into writing to files in Java, let’s cover some of the fundamental concepts involved in file I/O (input/output). Understanding these principles will give you a solid foundation to build on.
Text vs Binary Files
In Java, you can write to two types of files: text and binary. Text files are human-readable and are typically used to store textual data. Binary files, on the other hand, are not designed to be read by humans. They’re used to store data in a format that’s easy for a computer to read and write.
Here’s a simple example of writing to a text file:
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
FileWriter writer = new FileWriter("output.txt");
writer.write("Hello, world!");
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# 'Hello, world!' is written to 'output.txt'
And here’s an example of writing to a binary file:
import java.io.DataOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
FileOutputStream fileOut = new FileOutputStream("output.bin");
DataOutputStream dataOut = new DataOutputStream(fileOut);
dataOut.writeInt(123);
dataOut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# '123' is written to 'output.bin' in binary format
Character Encoding in Java
When you’re writing to a text file in Java, you’re actually writing characters, not bytes. These characters need to be encoded to bytes before they can be written to a file. Java uses Unicode, a universal character encoding standard, to represent characters. However, Java allows you to write to files using any character encoding that your system supports.
The Role of Exceptions in Java’s I/O System
Java’s I/O system uses exceptions to handle errors. An exception is an event that disrupts the normal flow of the program’s instructions. In the context of file I/O, exceptions are thrown when an I/O operation fails for some reason.
For example, if you’re trying to write to a file that doesn’t exist, Java will throw an IOException
. It’s crucial to catch and handle these exceptions to prevent your program from crashing and to provide meaningful error messages.
Integrating File I/O into Larger Java Applications
File I/O is a vital part of many Java applications. It’s used in a variety of scenarios, from reading and writing configuration files to logging program output.
Configuration Files and Java
Configuration files are often used to store settings for an application. In Java, you can use the Properties
class to read and write to these files. This allows you to easily store and retrieve settings for your application.
Logging Program Output
Logging is crucial for understanding the behavior of an application. Java provides the java.util.logging
package, which contains the classes necessary for producing log output. You can write log messages to a file, making it easier to diagnose and debug issues.
Further Reading and Resources for Java File I/O Mastery
To further your understanding of file I/O in Java, you might want to explore the following resources:
- Beginner’s Guide to Java File Class – Learn how to navigate file systems and retrieve file information using Java’s File class.
Java File Reading Basics – Learn Java file reading techniques using FileReader, BufferedReader, or other file handling APIs.
Java FileWriter: Overview – Understand how to use the FileWriter class in Java for writing character streams to files
Oracle’s Java I/O Tutorial covers all aspects of I/O in Java, from reading and writing to files to using streams and channels.
Baeldung’s Guide to Java File I/O is a great resource for understanding the different ways to read and write to files in Java.
Java Code Geeks’ Java File Writing Tutorial goes in-depth into the file writing in Java.
By understanding how to effectively use file I/O in your Java applications, you can enhance their functionality and improve your overall coding skills.
Wrapping Up: Java File Writing
In this comprehensive guide, we’ve navigated the world of writing to files in Java, shedding light on the different classes and methods that can be employed along with their respective use cases.
We kicked off with the basics, exploring how to use the PrintWriter
and FileWriter
classes to write data to a file. We then delved into more complex techniques, such as writing to binary files using the DataOutputStream
class, or writing in a specific character encoding using the OutputStreamWriter
class.
In our journey, we also addressed alternative methods to write to files in Java, like using the Files
class from the java.nio.file
package. We tackled common challenges such as IOExceptions
, and discussed how to handle these exceptions effectively.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
PrintWriter and FileWriter | Simple and intuitive, good for beginners | May require conversion of data types, can be slower due to buffering |
DataOutputStream | Allows writing of primitive data types in binary format | Not human-readable |
OutputStreamWriter | Allows writing in specific character encoding | Requires conversion to byte stream |
Files Class | Simple and atomic operations, good for multi-threading environment | Not suitable for large files |
Whether you’re a beginner just starting out with file writing in Java or an expert seeking to refresh your knowledge, we hope this guide has equipped you with a deeper understanding of the various facets of writing to files in Java.
With its rich set of classes and methods, Java provides a powerful and flexible platform for file I/O operations. Now, you’re well-equipped to handle any file writing task that comes your way. Happy coding!