Java’s FileWriter Class: Detailed Usage Guide
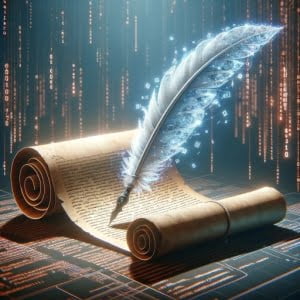
Ever found yourself wrestling with writing data to a file in Java? You’re not alone. Many developers find this task a bit challenging, but there’s a tool in Java that can make this process a breeze.
Think of Java’s FileWriter class as a diligent scribe – capable of recording data with ease. FileWriter is a class in Java that allows you to write character-oriented data to a file, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using FileWriter in Java, from basic usage to advanced techniques. We’ll cover everything from writing simple data to a file, handling exceptions, to dealing with file permissions and even troubleshooting common issues.
So, let’s dive in and start mastering FileWriter in Java!
TL;DR: How Do I Use FileWriter in Java?
FileWriter
is a class in Java used to write character-oriented data to a file. It is instantiated with the syntax,FileWriter writer = new FileWriter('file.txt');
It’s a powerful tool that can simplify the process of writing data to files in your Java applications.
Here’s a simple example:
FileWriter writer = new FileWriter('file.txt');
writer.write('Hello, World!');
writer.close();
# Output:
# This will write 'Hello, World!' to a file named 'file.txt'.
In this example, we create a new FileWriter object that’s connected to ‘file.txt’. We then use the write
method to write the string ‘Hello, World!’ to the file. Finally, we close the FileWriter with the close
method, which is an important step to prevent potential memory leaks and to ensure that the data is actually written to the file.
This is just a basic way to use FileWriter in Java, but there’s much more to learn about writing data to files in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Using FileWriter for Beginners
When it comes to writing data to a file in Java, the FileWriter class is a beginner-friendly tool that can be easily understood and implemented. Let’s start with a basic example of using FileWriter to write data to a file.
try {
FileWriter writer = new FileWriter('file.txt');
writer.write('Hello, Java!');
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a file named 'file.txt' and write 'Hello, Java!' into it.
In this example, we create a new FileWriter object that’s connected to ‘file.txt’. We use the write
method to write the string ‘Hello, Java!’ to the file. We wrap the code in a try-catch block to handle any IOExceptions that might occur. Finally, we close the FileWriter with the close
method.
The close
method is crucial because it releases the resources associated with the FileWriter. If you forget to call close
, you might encounter a resource leak, which can slow down your application or even cause it to crash.
One of the advantages of FileWriter is its simplicity. With just a few lines of code, you can write data to a file. However, it’s important to handle exceptions properly and to always close the FileWriter when you’re done with it.
Intermediate FileWriter Techniques
Once you’ve grasped the basics of FileWriter in Java, you can start exploring more advanced techniques. These can involve writing to different types of files, handling exceptions more effectively, and leveraging FileWriter with other I/O classes.
Let’s start with writing to different types of files. FileWriter isn’t just limited to text files; it can write to any file that can store text. Here’s an example of writing to a CSV file:
try {
FileWriter writer = new FileWriter('data.csv');
writer.write('Name,Age,Email
');
writer.write('John,30,[email protected]
');
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a CSV file named 'data.csv' and write two lines of text into it.
In this example, we’re writing a CSV file with column headers ‘Name’, ‘Age’, and ‘Email’, and then adding a line of data. Notice the use of ‘
‘ to create a new line in the file, which is necessary to separate rows in the CSV file.
Next, let’s discuss handling exceptions. While the previous examples used a simple try-catch block to handle IOExceptions, in a real-world application, you might want to provide more specific error messages or take different actions based on the type of the exception. Here’s a more advanced way to handle exceptions with FileWriter:
try {
FileWriter writer = new FileWriter('file.txt');
writer.write('Hello, Java!');
writer.close();
} catch (FileNotFoundException e) {
System.out.println('The specified file does not exist.');
} catch (IOException e) {
System.out.println('An error occurred while writing to the file.');
}
In this example, we’re catching two types of exceptions: FileNotFoundException and IOException. This allows us to provide a more specific error message depending on the type of the exception.
Finally, FileWriter can be used in combination with other I/O classes for more complex file writing tasks. For instance, you can use FileWriter with BufferedWriter for more efficient writing of multiple lines of text:
try {
FileWriter writer = new FileWriter('file.txt');
BufferedWriter bufferedWriter = new BufferedWriter(writer);
bufferedWriter.write('Hello, Java!');
bufferedWriter.newLine();
bufferedWriter.write('This is another line.');
bufferedWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a file named 'file.txt' and write two lines of text into it.
In this example, we’re using BufferedWriter to write multiple lines of text to the file. BufferedWriter provides a newLine
method that inserts a new line separator, which can be more convenient and efficient than manually writing ‘
‘.
Alternative Methods for File Writing in Java
While FileWriter is a powerful tool, Java provides alternative methods for writing data to a file. Two popular alternatives are the Files class and OutputStreamWriter. These classes offer different benefits and drawbacks, and the best choice depends on your specific needs.
Using the Files Class
The Files class is part of the java.nio.file package, which provides methods for file operations. One of its methods, write
, can write a list of lines to a file in a single operation.
try {
List<String> lines = Arrays.asList('Hello, Java!', 'This is another line.');
Files.write(Paths.get('file.txt'), lines, StandardCharsets.UTF_8);
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a file named 'file.txt' and write two lines of text into it.
In this example, we’re using the write
method of the Files class to write a list of lines to a file. This method is convenient when you have multiple lines of text to write to a file, as it requires less code than using FileWriter and BufferedWriter.
However, the write
method of the Files class has a drawback: it opens and closes the file for each operation, which can be inefficient if you’re writing to the file multiple times.
Using OutputStreamWriter
OutputStreamWriter is another alternative for writing data to a file. It’s a bridge from character streams to byte streams: characters written to it are encoded into bytes using a specified charset.
try {
OutputStreamWriter writer = new OutputStreamWriter(new FileOutputStream('file.txt'), StandardCharsets.UTF_8);
writer.write('Hello, Java!');
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a file named 'file.txt' and write 'Hello, Java!' into it.
In this example, we’re using OutputStreamWriter to write a string to a file. OutputStreamWriter is useful when you need to write text to a byte stream, such as a network socket. However, for writing to a file, FileWriter is usually more convenient and provides better performance.
In conclusion, while FileWriter is a versatile tool for writing data to a file in Java, it’s not the only option. Depending on your specific needs, you might find that the Files class or OutputStreamWriter is a better fit. It’s important to understand the benefits and drawbacks of each approach, and to choose the one that best meets your needs.
Troubleshooting FileWriter Issues
While FileWriter is a powerful tool, like any other, it’s not without its challenges. Here, we’ll discuss some common issues you might encounter when using FileWriter, from handling IOExceptions to managing resources and dealing with file permissions.
Handling IOExceptions
IOExceptions are a common issue when working with FileWriter. These exceptions can occur for a variety of reasons, such as the file not being found or an error occurring during writing. Here’s an example of an IOException and how to handle it:
try {
FileWriter writer = new FileWriter('nonexistent.txt');
writer.write('Hello, Java!');
writer.close();
} catch (IOException e) {
System.out.println('An error occurred: ' + e.getMessage());
}
# Output:
# An error occurred: (The system cannot find the file specified)
In this example, we’re trying to write to a file that doesn’t exist, which results in a FileNotFoundException, a subclass of IOException. We catch the exception and print a specific error message.
Managing Resources
Another common issue is forgetting to close the FileWriter. If a FileWriter is not closed, it can lead to resource leaks, which can slow down your application or even cause it to crash. Therefore, it’s crucial to always close your FileWriter in a finally block or use a try-with-resources statement:
try (FileWriter writer = new FileWriter('file.txt')) {
writer.write('Hello, Java!');
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a file named 'file.txt' and write 'Hello, Java!' into it.
In this example, we’re using a try-with-resources statement, which automatically closes the FileWriter, even if an exception occurs.
Dealing with File Permissions
File permissions can also cause issues when using FileWriter. If you don’t have permission to write to a file, an IOException will occur. To solve this issue, you need to check the file permissions and change them if necessary.
In conclusion, while FileWriter is a handy tool, it’s important to be aware of these common issues and know how to handle them. This will help you write more robust and reliable Java programs.
Understanding Java’s I/O Classes
To truly master FileWriter in Java, it’s important to understand the broader context of Java’s I/O classes and interfaces. These classes and interfaces provide the building blocks for input and output operations in Java, including reading from and writing to files.
Character-Oriented Streams
Java’s I/O classes can be broadly divided into two categories: byte-oriented streams and character-oriented streams. Byte-oriented streams, represented by InputStream and OutputStream, are used for reading and writing binary data.
Character-oriented streams, on the other hand, are designed for reading and writing text data. They are represented by Reader and Writer, which are the superclasses for all character-oriented streams in Java. The key advantage of character-oriented streams is that they automatically handle character encoding, making it easier to read and write text in different languages.
try {
Reader reader = new FileReader('file.txt');
int data = reader.read();
while (data != -1) {
char dataAsChar = (char) data;
System.out.print(dataAsChar);
data = reader.read();
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will read and print the contents of 'file.txt'.
In this example, we’re using FileReader, a subclass of Reader, to read text from a file. Notice how we’re reading data as integers and then converting them to characters. This is because character-oriented streams read and write data as 16-bit Unicode characters, which can represent text in almost any language.
The Role of FileWriter
FileWriter is a subclass of Writer and provides methods for writing character-oriented data to a file. It’s essentially a bridge between your Java program and a file on your computer, allowing your program to write text data to the file.
try {
Writer writer = new FileWriter('file.txt');
writer.write('Hello, Java!');
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will create a file named 'file.txt' and write 'Hello, Java!' into it.
In this example, we’re using FileWriter to write text to a file. Notice how we’re writing data as a string, which is automatically converted to characters by the FileWriter.
In conclusion, FileWriter is a key component of Java’s I/O classes and plays an essential role in writing text data to files. By understanding how FileWriter fits into the broader context of Java’s I/O classes, you can write more efficient and effective Java programs.
Applying FileWriter in Larger Projects
As you become more comfortable with FileWriter, you’ll find it’s not just for small tasks. FileWriter can play a crucial role in larger projects, including logging, data processing, and much more.
FileWriter for Logging
Logging is a common use case for FileWriter. By writing log messages to a file, you can keep a record of what’s happening in your application, which is invaluable for debugging and monitoring. Here’s a simple example of using FileWriter for logging:
try {
FileWriter writer = new FileWriter('log.txt', true);
writer.write('Starting application at ' + new Date() + '
');
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will append a log message with the current date and time to 'log.txt'.
In this example, we’re opening the file in append mode by passing true
as the second argument to the FileWriter constructor. This means that each log message will be appended to the end of the file, rather than overwriting the file.
FileWriter for Data Processing
FileWriter can also be useful in data processing tasks. For instance, if you’re processing a large amount of data and want to write the results to a file, FileWriter can do the job. Here’s an example of using FileWriter to write processed data to a file:
try {
FileWriter writer = new FileWriter('processed-data.txt');
for (Data data : dataList) {
String processedData = process(data);
writer.write(processedData + '
');
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# This will write processed data to 'processed-data.txt'.
In this example, we’re processing a list of data and writing the processed data to a file. FileWriter makes it easy to write each piece of processed data to a new line in the file.
Further Resources for FileWriter Mastery
To continue your journey in mastering FileWriter in Java, here are some additional resources that you might find helpful:
- Java File Class: Essential File Operations – Master Java’s File class for reading and writing data to files and directories.
How to Create Files in Java – Master Java file creation methods for generating files dynamically in applications.
Writing to Files in Java – Learn how to write data to files in Java for saving application output or storing user data.
Oracle’s Official Java I/O Tutorial – Grasp the fundamentals of Java’s Input and Output mechanisms in this comprehensive guide.
Baeldung’s Java FileWriter Guide can help you master writing text to files with Java FileWriter class.
DigitalOcean’s Java FileWriter Tutorial explains how to implement the FileWriter class in Java.
These resources provide more in-depth information about FileWriter and related topics, helping you to further enhance your skills and knowledge.
Wrapping Up: FileWriter in Java
In this comprehensive guide, we’ve delved into the depths of FileWriter in Java, a class that simplifies the process of writing character-oriented data to a file. Whether you’re just starting out with Java or looking to level up your skills, we’ve explored everything you need to know about FileWriter.
We began with the basics, learning how to use FileWriter to write data to a file. We then explored more advanced techniques, such as writing to different types of files, handling exceptions more effectively, and using FileWriter with other I/O classes. Along the way, we tackled common challenges that you might encounter when using FileWriter, providing solutions and workarounds for each issue.
We also ventured into alternative approaches to file writing in Java, comparing FileWriter with other methods like the Files class and OutputStreamWriter. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
FileWriter | Easy to use, good performance | Requires manual resource management |
Files class | Convenient for writing multiple lines, handles character encoding | Opens and closes the file for each operation |
OutputStreamWriter | Useful for writing text to a byte stream | Less convenient and slower for writing to a file |
As we’ve seen, FileWriter is a versatile tool, but it’s not the only option for writing data to a file in Java. Depending on your specific needs, you might find that an alternative method is a better fit.
Whether you’re just getting started with FileWriter or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. With the knowledge you’ve gained, you’re now well-equipped to write data to files in Java with confidence and ease. Happy coding!