Java PriorityQueue: Ordering and Organizing Elements
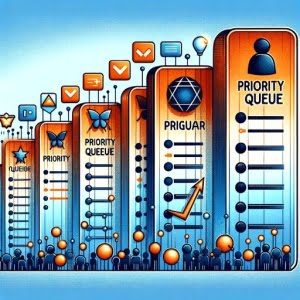
Are you finding it challenging to work with Java’s Priority Queue? You’re not alone. Many developers find themselves puzzled when it comes to handling Priority Queue in Java, but we’re here to help.
Think of Java’s Priority Queue as a well-organized assistant, ensuring that elements are served based on their priorities. It’s a powerful tool that can make your coding tasks more efficient and organized.
In this guide, we’ll walk you through the process of using Java’s Priority Queue, from basic usage to advanced techniques. We’ll cover everything from creating a PriorityQueue, adding elements to it, to more complex uses such as using a custom Comparator to determine the order of elements.
So, let’s dive in and start mastering Java’s Priority Queue!
TL;DR: How Do I Use a Priority Queue in Java?
To use a priority queue in Java, you create an instance of PriorityQueue as follows:
PriorityQueue<Integer> pq = new PriorityQueue<>();
. Once elements are added, they will be ordered based on their natural ordering or by a Comparator provided at queue construction time.
Here’s a simple example:
PriorityQueue<Integer> pq = new PriorityQueue<>();
pq.add(10);
pq.add(20);
pq.add(15);
// Output:
// [10, 15, 20]
In this example, we create an instance of PriorityQueue and add three integers to it. The PriorityQueue automatically orders the elements based on their natural ordering, so when we print the queue, we see the elements in ascending order.
This is just a basic way to use PriorityQueue in Java, but there’s much more to learn about managing elements based on their priorities. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Java’s PriorityQueue
- Advanced PriorityQueue Usage: Custom Comparators
- Exploring Alternatives: TreeMap and Custom Objects
- Handling Common PriorityQueue Pitfalls
- Understanding Java’s PriorityQueue and Binary Heap
- Exploring PriorityQueue in Real-World Applications
- Delving Deeper: Binary Heap and Tree Data Structures
- Wrapping Up: Java’s PriorityQueue
Getting Started with Java’s PriorityQueue
Java’s PriorityQueue is a queue data structure where elements are served based on their priority. It’s part of Java’s Collections Framework and implements the Queue interface. Let’s explore how to create, add, and retrieve elements from a PriorityQueue.
Creating a PriorityQueue
Creating a PriorityQueue in Java is straightforward. You create an instance of PriorityQueue as follows:
PriorityQueue<Integer> pq = new PriorityQueue<>();
In this example, we’ve created a PriorityQueue that will hold Integer objects.
Adding Elements to PriorityQueue
To add elements to the PriorityQueue, we use the add()
method. Here’s how you can add elements to the PriorityQueue:
pq.add(10);
pq.add(20);
pq.add(15);
In this example, we’ve added three integers to our PriorityQueue.
Retrieving Elements from PriorityQueue
To retrieve and remove the highest priority element from the PriorityQueue, we use the poll()
method. If the PriorityQueue is empty, the poll()
method returns null.
Here’s how you can retrieve elements:
Integer element = pq.poll();
System.out.println(element);
// Output:
// 10
In this example, poll()
retrieves and removes the least element from the PriorityQueue, which is 10.
Understanding PriorityQueue’s Behavior
One of the key advantages of PriorityQueue is that it automatically orders its elements according to their natural ordering or a provided Comparator. In our example, since we didn’t provide a Comparator, PriorityQueue uses the natural ordering of integers.
However, one potential pitfall to remember is that PriorityQueue is not thread-safe. If multiple threads modify a PriorityQueue concurrently, its behavior is not specified. For a thread-safe PriorityQueue, consider using PriorityBlockingQueue.
Advanced PriorityQueue Usage: Custom Comparators
Java’s PriorityQueue offers a lot more than just basic functionality. One of the more advanced features is the ability to use a custom Comparator to determine the order of elements in the queue.
Using a Custom Comparator
A Comparator is a comparison function, which provide an ordering for collections of objects that don’t have a natural ordering.
Here’s an example of how you can use a custom Comparator to order elements in a PriorityQueue:
Comparator<Integer> comparator = new Comparator<Integer>() {
@Override
public int compare(Integer num1, Integer num2) {
return num2 - num1;
}
};
PriorityQueue<Integer> pq = new PriorityQueue<>(comparator);
pq.add(10);
pq.add(20);
pq.add(15);
while(!pq.isEmpty()) {
Integer element = pq.poll();
System.out.println(element);
}
// Output:
// 20
// 15
// 10
In this example, we’ve created a custom Comparator that orders integers in descending order. We then use this Comparator to create a PriorityQueue. When we add elements to the PriorityQueue and then retrieve them, they are served in descending order, which is the opposite of their natural ordering.
Understanding the Differences and Best Practices
When using a custom Comparator, it’s important to remember that the Comparator’s compare()
method must be consistent with equals, as required by the general contract of Comparator.compare()
. This means that compare(x, y)==0
implies that sgn(compare(x, z))==sgn(compare(y, z))
for all z
.
It’s also worth noting that the compare()
method must throw an exception if the comparison is not possible. This is to ensure that PriorityQueue’s invariants are not violated.
In terms of best practices, it’s generally a good idea to provide a custom Comparator when creating a PriorityQueue if the natural ordering of the elements is not what you want. This gives you full control over the order of elements in the PriorityQueue.
Exploring Alternatives: TreeMap and Custom Objects
Java offers a variety of data structures that can manage elements based on their priorities. Besides PriorityQueue, you can also use TreeMap or a PriorityQueue with a custom object. Let’s explore these alternatives.
Using TreeMap for Priority-Based Management
TreeMap in Java is a Red-Black tree based NavigableMap implementation. It provides an efficient means of storing key-value pairs in sorted order. Here’s an example of using TreeMap to manage elements based on their priorities:
TreeMap<Integer, String> tm = new TreeMap<>();
tm.put(10, "Ten");
tm.put(20, "Twenty");
tm.put(15, "Fifteen");
System.out.println(tm.firstEntry());
// Output:
// 10=Ten
In this example, we create a TreeMap and add three entries to it. When we retrieve the first entry, it gives us the entry with the least key, which is 10.
Using PriorityQueue with Custom Objects
You can also use PriorityQueue with custom objects to manage elements based on their priorities. However, you’ll need to provide a Comparator that can compare the custom objects. Here’s an example:
class CustomObject {
String name;
int priority;
// constructor, getters and setters omitted for brevity
}
Comparator<CustomObject> comparator = Comparator.comparing(CustomObject::getPriority);
PriorityQueue<CustomObject> pq = new PriorityQueue<>(comparator);
// add elements to pq
// Output:
// CustomObject with the least priority
In this example, we create a custom object with a priority field. We then create a PriorityQueue that holds these custom objects and uses a Comparator to order them based on their priorities.
Weighing the Options
Both TreeMap and PriorityQueue with custom objects offer the ability to manage elements based on their priorities. However, they have their own advantages and disadvantages.
TreeMap maintains a sorted order of its elements at all times, which can be beneficial if you need to frequently retrieve the least or greatest element. However, it uses more memory than PriorityQueue.
On the other hand, PriorityQueue with custom objects gives you full control over the ordering of elements. But you need to provide a Comparator that can compare the custom objects, which can be a bit more complex.
In terms of recommendations, if memory usage is a concern, PriorityQueue is a better option. But if you need to frequently retrieve the least or greatest element, TreeMap might be more suitable.
Handling Common PriorityQueue Pitfalls
While PriorityQueue is a powerful tool, it’s not without its quirks. Developers often encounter a few common issues when working with this data structure. Let’s delve into these issues and how to resolve them.
Dealing with ClassCastException
One of the most common issues is the ClassCastException, which occurs when trying to insert elements that cannot be compared. This is because PriorityQueue needs to compare its elements to order them.
PriorityQueue<Object> pq = new PriorityQueue<>();
pq.add("string");
pq.add(new Object());
// Output:
// Exception in thread "main" java.lang.ClassCastException: java.base/java.lang.Object cannot be cast to java.base/java.lang.Comparable
In this example, we try to add a string and an Object to the same PriorityQueue. Since Object doesn’t implement Comparable, it throws a ClassCastException.
Solution
To avoid this issue, ensure that all elements added to the PriorityQueue are instances of a class that implements Comparable, or provide a custom Comparator when creating the PriorityQueue.
PriorityQueue<String> pq = new PriorityQueue<>();
pq.add("string1");
pq.add("string2");
// Output:
// [string1, string2]
In this example, we create a PriorityQueue that holds strings, which implement Comparable. So, it doesn’t throw a ClassCastException.
Other Considerations
Besides these issues, there are a few other considerations to keep in mind when using PriorityQueue:
- PriorityQueue is not synchronized. If multiple threads modify a PriorityQueue concurrently, its behavior is not specified. For a thread-safe PriorityQueue, consider using PriorityBlockingQueue.
The
Iterator
provided in methoditerator()
is not guaranteed to traverse the elements of the PriorityQueue in any particular order.While PriorityQueue is a queue, it doesn’t enforce its elements to be non-null. Adding null elements will result in NullPointerException.
By keeping these considerations in mind, you can avoid common pitfalls and effectively use PriorityQueue in your projects.
Understanding Java’s PriorityQueue and Binary Heap
To fully grasp Java’s PriorityQueue, it’s essential to understand its underlying data structure – the Binary Heap, and how it manages to maintain the priority of elements. Let’s delve into these fundamentals.
The Binary Heap: PriorityQueue’s Backbone
A Binary Heap is a complete binary tree that maintains the Heap Invariant (parent nodes are either greater than or equal to their child nodes for a max heap, or less than or equal to their child nodes for a min heap). Java’s PriorityQueue uses a Binary Heap to store its elements.
PriorityQueue<Integer> pq = new PriorityQueue<>();
pq.add(10);
pq.add(20);
pq.add(15);
// Output: [10, 20, 15]
In this code block, we add three integers to the PriorityQueue. The PriorityQueue uses a Binary Heap to store these integers. When we print the PriorityQueue, we see the elements in an order that maintains the Heap Invariant.
Natural Ordering and Comparator in Java
Java’s PriorityQueue orders its elements based on their natural ordering or a Comparator provided at queue construction time. Natural ordering is the ordering provided by the compareTo()
method of the Comparable
interface.
PriorityQueue<String> pq = new PriorityQueue<>();
pq.add("apple");
pq.add("banana");
pq.add("cherry");
// Output: [apple, banana, cherry]
In this code block, we add three strings to the PriorityQueue. Since String implements Comparable, the PriorityQueue uses the compareTo()
method to order the strings.
A Comparator, on the other hand, is a comparison function that provides an ordering for objects that don’t have a natural ordering. It’s an interface in Java that needs to be implemented by any class that wishes to define an ordering for objects.
Comparator<String> comparator = Comparator.reverseOrder();
PriorityQueue<String> pq = new PriorityQueue<>(comparator);
pq.add("apple");
pq.add("banana");
pq.add("cherry");
// Output: [cherry, banana, apple]
In this code block, we create a Comparator that orders strings in reverse of their natural ordering. We then use this Comparator to create a PriorityQueue. The PriorityQueue uses the Comparator to order the strings.
By understanding these fundamentals, you can better understand how PriorityQueue works and use it more effectively in your projects.
Exploring PriorityQueue in Real-World Applications
Java’s PriorityQueue isn’t just a theoretical concept; it has practical applications in various real-world scenarios. Let’s explore some of these applications and how PriorityQueue plays a crucial role in them.
Task Scheduling
One of the most common uses of PriorityQueue is in task scheduling, where tasks are assigned priorities, and the task with the highest priority is executed first. For instance, in an operating system, processes are often scheduled based on their priorities.
PriorityQueue<Task> taskQueue = new PriorityQueue<>(Comparator.comparing(Task::getPriority));
// add tasks to taskQueue
Task nextTask = taskQueue.poll();
// Output:
// Task with the highest priority
In this code block, we create a PriorityQueue of Task objects, where each Task has a priority. The PriorityQueue ensures that the task with the highest priority is served first.
Data Compression
PriorityQueue is also used in data compression algorithms like Huffman coding. In Huffman coding, characters are assigned codes based on their frequencies, with more frequent characters getting shorter codes. PriorityQueue is used to manage the characters and their frequencies.
Graph Algorithms
In graph algorithms like Dijkstra’s algorithm and Prim’s algorithm, PriorityQueue is used to select the next node to visit. PriorityQueue ensures that the node with the least cost is visited first, which is crucial for these algorithms’ performance.
Delving Deeper: Binary Heap and Tree Data Structures
If you’re interested in learning more about PriorityQueue and its underlying concepts, we recommend exploring binary heap and tree data structures. Binary heap is the data structure that Java’s PriorityQueue uses to store its elements, while tree data structures are closely related to binary heap and offer a broader understanding of how data can be organized and managed.
Further Resources for Mastering PriorityQueue
To deepen your understanding of PriorityQueue and its related concepts, here are some resources that you might find helpful:
- Tips and Techniques for Data Structures in Java – Learn about generics concepts and their use in Java data structure implementations.
Understanding Java Stack – Learn about stack operations like push, pop, and peek for efficient data manipulation.
Exploring Java Queue – Explore Queue implementations like LinkedList and PriorityQueue for various use cases.
Java’s Official Documentation on PriorityQueue explores the PriorityQueue class.
GeeksforGeeks’s Guide on PriorityQueue in Java is a great resource on using PriorityQueue in Java.
Baeldung’s Tutorial on Java PriorityQueue explains how to effectively use a PriorityQueue using Java.
These resources offer in-depth explanations and examples on PriorityQueue, binary heap, and tree data structures, which can help you master these concepts and apply them effectively in your projects.
Wrapping Up: Java’s PriorityQueue
In this comprehensive guide, we’ve delved into the world of Java’s PriorityQueue, a powerful tool for managing elements based on their priorities.
We began with the basics, understanding how to create a PriorityQueue, add elements to it, and retrieve elements based on their priorities. We then ventured into more advanced usage, exploring how to use a custom Comparator to determine the order of elements in the PriorityQueue.
We’ve also discussed common issues that developers often encounter when using PriorityQueue, such as ClassCastException, and provided solutions and workarounds for each issue. Additionally, we explored alternative approaches to handle priority-based data, such as TreeMap and PriorityQueue with custom objects.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
PriorityQueue | Efficient, orders elements based on their priorities | Not thread-safe, requires Comparable elements |
TreeMap | Maintains sorted order at all times, provides key-value pairs | Uses more memory than PriorityQueue |
PriorityQueue with Custom Objects | Full control over ordering of elements | Requires a Comparator that can compare the custom objects |
Whether you’re just starting out with PriorityQueue or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of PriorityQueue and its capabilities.
The ability to manage elements based on their priorities is a powerful tool for many applications, such as task scheduling and graph algorithms. With this guide, you’re now well equipped to use PriorityQueue effectively in your projects. Happy coding!