How to Declare Arrays in Bash: A Shell Scripting Guide
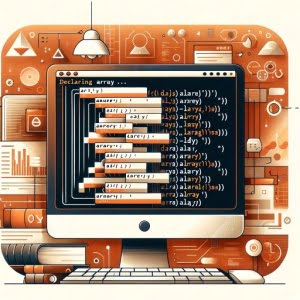
Are you finding it challenging to handle arrays in Bash? You’re not alone. Many developers find themselves puzzled when it comes to declaring and manipulating arrays in Bash, but we’re here to help.
Think of Bash arrays as a toolbox – each slot holding a different tool. These tools can be anything from strings to integers, giving you a versatile and handy tool for various tasks.
This guide will walk you through the process of declaring arrays in Bash, from the basics to more advanced techniques. We’ll cover everything from the simple declaration of arrays, accessing and manipulating array elements, to more complex uses like multi-dimensional and associative arrays.
So, let’s dive in and start mastering Bash arrays!
TL;DR: How Do I Declare an Array in Bash?
In Bash, you declare an array using the following syntax:
array_name=(item1 item2 item3)
. This creates an array named ‘array_name’ with three items.
Here’s a simple example:
array_name=('Bash' 'Python' 'JavaScript')
echo ${array_name[0]}
echo ${array_name[1]}
echo ${array_name[2]}
# Output:
# Bash
# Python
# JavaScript
In this example, we’ve declared an array named ‘array_name’ with three items: ‘Bash’, ‘Python’, and ‘JavaScript’. We then use the echo command to print each item. The numbers in the square brackets are the indices of the array items. In Bash, arrays are zero-indexed, meaning the first item is at index 0.
This is just a basic way to declare an array in Bash, but there’s much more to learn about accessing and manipulating arrays. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
Bash Arrays: Declaration and Manipulation
Let’s get started with the basics of declaring, accessing, and manipulating arrays in Bash. We’ll cover each step in detail and provide a simple code example for better understanding.
Declaring an Array
In Bash, we declare an array using the parentheses ()
syntax. Here’s how:
programming_languages=('Bash' 'Python' 'JavaScript' 'Ruby')
In this example, we’ve declared an array named ‘programming_languages’ with four items.
Accessing Array Elements
To access an element in an array, we use the array name followed by the index of the element in square brackets []
:
echo ${programming_languages[0]}
# Output:
# Bash
In this example, we’ve printed the first item of the ‘programming_languages’ array. Remember, Bash arrays are zero-indexed, so the first item is at index 0.
Manipulating Arrays
You can also add, modify, or remove elements from an array. Here’s how you can add an item to the array:
programming_languages+=('Java')
echo ${programming_languages[@]}
# Output:
# Bash Python JavaScript Ruby Java
In this example, we’ve added ‘Java’ to the ‘programming_languages’ array and then printed all items.
Arrays in Bash are incredibly versatile, but they come with potential pitfalls. For instance, Bash doesn’t support multi-dimensional arrays natively, and arrays are zero-indexed, which can lead to off-by-one errors if you’re not careful. But don’t worry, with practice, you’ll get the hang of it!
Expanding Bash Array Knowledge: Advanced Usage
While Bash is limited to one-dimensional indexed arrays, we can simulate multi-dimensional arrays with a bit of creativity. Also, Bash supports associative arrays, which are arrays that use named keys instead of numerical indexes. Let’s take a closer look.
Simulating Multi-Dimensional Arrays
To simulate a multi-dimensional array in Bash, we can use a single-dimensional array and calculate the equivalent index. Here’s an example:
# Declare the array
matrix=(1 2 3 4 5 6 7 8 9)
# Access elements
rows=3
columns=3
for ((i=0; i<rows; i++)); do
for ((j=0; j<columns; j++)); do
index=$((columns * i + j))
echo ${matrix[index]}
done
done
# Output:
# 1
# 2
# 3
# 4
# 5
# 6
# 7
# 8
# 9
In this example, we’ve declared a single-dimensional array ‘matrix’ and accessed it as if it were a 3×3 matrix. We calculated the index using the formula index = columns * row + column
.
Associative Arrays
Associative arrays are a type of array that uses named keys rather than numerical indexes. Here’s how you declare and use an associative array:
declare -A employee
# Assign values
employee[name]='John Doe'
employee[title]='Software Engineer'
employee[department]='Development'
# Access values
echo ${employee[name]}
echo ${employee[title]}
echo ${employee[department]}
# Output:
# John Doe
# Software Engineer
# Development
In this example, we’ve declared an associative array ’employee’ and assigned values to it using named keys. We then accessed the values using these keys.
Array Operations
Bash provides several operations to manipulate arrays, such as adding and removing elements, and finding the length of an array. Here’s an example of how to find the length of an array:
programming_languages=('Bash' 'Python' 'JavaScript' 'Ruby')
# Find length
echo ${#programming_languages[@]}
# Output:
# 4
In this example, we’ve found the length of the ‘programming_languages’ array using ${#array_name[@]}
.
These advanced techniques can greatly extend the utility of arrays in Bash, providing you with more flexibility and power in your scripting.
Harnessing External Tools for Data Handling
While arrays are a powerful tool in Bash, they’re not the only way to handle collections of data. Sometimes, using external tools such as awk or sed can be more suitable, especially when dealing with large data sets or complex string manipulation.
Leveraging Awk for Data Manipulation
Awk is a powerful text processing language that can be used to manipulate data in Bash. It can be used to split strings into arrays based on a delimiter. Here’s an example:
string='Bash:Python:JavaScript:Ruby'
# Split string into array using awk
read -a programming_languages <<< $(echo $string | awk -F: '{ for(i=1; i<=NF; i++) print $i }')
# Print all items
for language in ${programming_languages[@]}; do
echo $language
done
# Output:
# Bash
# Python
# JavaScript
# Ruby
In this example, we’ve used awk to split a string into an array based on the colon ‘:’ delimiter. We then printed all items in the array.
Using Sed for String Manipulation
Sed is another text processing tool that can be used in Bash. It’s particularly useful for find and replace operations on strings. Here’s an example of how you can use sed to manipulate a string and store the results in an array:
string='Bash-Python-JavaScript-Ruby'
# Replace hyphens with spaces using sed
string=$(echo $string | sed 's/-/ /g')
# Convert string to array
programming_languages=($string)
# Print all items
echo ${programming_languages[@]}
# Output:
# Bash Python JavaScript Ruby
In this example, we’ve used sed to replace hyphens ‘-‘ with spaces in a string. We then converted the manipulated string into an array and printed all items.
While these external tools provide additional flexibility, they also come with their own drawbacks. For instance, they can be slower than native Bash operations, especially with large data sets. Moreover, they add external dependencies to your scripts, which can lead to portability issues.
The decision to use arrays or external tools depends on your specific use case. If you’re dealing with simple data collections, arrays might be the way to go. However, if you need to perform complex string manipulation or deal with large data sets, external tools like awk or sed might be more suitable.
Common Pitfalls and Best Practices with Bash Arrays
Working with arrays in Bash can sometimes lead to unexpected results. Let’s discuss some common errors and how to avoid them. We’ll cover off-by-one errors, uninitialized array elements, and how to handle spaces in array elements.
Off-By-One Errors
A common mistake when working with arrays in Bash is off-by-one errors. This happens because arrays in Bash are zero-indexed, which means the first item is at index 0, not 1. Here’s an example of an off-by-one error:
programming_languages=('Bash' 'Python' 'JavaScript' 'Ruby')
# Try to access the fifth item
echo ${programming_languages[4]}
# Output:
# (blank line)
In this example, we tried to access the fifth item of the ‘programming_languages’ array, but there are only four items. The echo command didn’t print anything because there’s no fifth item. Always remember that the index of the last item is one less than the length of the array.
Uninitialized Array Elements
Another common mistake is trying to access uninitialized array elements. In Bash, uninitialized array elements don’t cause an error; they simply return an empty string. Here’s an example:
programming_languages=('Bash' 'Python' 'JavaScript' 'Ruby')
# Try to access an uninitialized element
echo ${programming_languages[10]}
# Output:
# (blank line)
In this example, we tried to access the eleventh item of the ‘programming_languages’ array, but it’s uninitialized. The echo command didn’t print anything because the eleventh item doesn’t exist.
Handling Spaces in Array Elements
Spaces in array elements can cause unexpected behavior if not handled properly. To avoid issues, always quote your array elements and expansions. Here’s an example:
programming_languages=('Bash' 'Python' 'JavaScript' 'Ruby' 'C++' 'C#')
# Print all items with spaces
for language in "${programming_languages[@]}"; do
echo $language
done
# Output:
# Bash
# Python
# JavaScript
# Ruby
# C++
# C#
In this example, we’ve declared an array with six items, two of which (‘C++’ and ‘C#’) contain spaces. We then printed all items correctly by quoting the array expansion.
Remember, arrays in Bash are a powerful tool, but they come with potential pitfalls. Always remember to consider the zero-indexing, initialize your array elements, and handle spaces properly.
Bash Arrays: The Fundamentals
To truly master Bash arrays, it’s essential to understand how they’re implemented in Bash and how they differ from arrays in other programming languages.
Bash Array Implementation
In Bash, arrays are implemented as ordered maps, with keys being represented as integers. This means that arrays in Bash can have gaps in their indices. Here’s an example:
# Declare an array with gaps in indices
declare -a sparse_array
sparse_array[1]='Bash'
sparse_array[3]='Python'
sparse_array[5]='JavaScript'
# Print all items
echo ${sparse_array[@]}
# Output:
# Bash Python JavaScript
In this example, we’ve declared an array ‘sparse_array’ with gaps in its indices. We then printed all items, which are ordered by their indices.
Bash vs. Other Languages
Bash arrays are different from arrays in other programming languages in several ways. First, they’re one-dimensional, meaning they can only hold a list of items. However, you can simulate multi-dimensional arrays using associative arrays or calculated indices.
Second, Bash arrays are zero-indexed, which means the first item is at index 0. This is similar to languages like C, Java, and JavaScript, but different from languages like Lua and MATLAB, which are one-indexed.
Third, Bash arrays can have gaps in their indices, which is not common in other languages. This can be a powerful feature, but it can also lead to unexpected behavior if not handled properly.
Lastly, Bash supports associative arrays, which are arrays that use named keys instead of numerical indexes. This is similar to dictionaries in Python or objects in JavaScript, and it provides a lot of flexibility in handling collections of data.
Understanding these fundamentals will help you use Bash arrays more effectively and avoid common pitfalls.
The Bigger Picture: Arrays in Larger Bash Scripts
Arrays are an integral part of any Bash script, especially those that are more complex and larger in scale. They allow you to manage and manipulate collections of data efficiently and effectively.
Arrays in Larger Bash Projects
In larger Bash projects, arrays can be used to hold data that needs to be processed or manipulated. For example, you might have a script that processes a list of files. You could use an array to hold the file names, and then loop over the array to process each file in turn.
Here’s an example:
# Declare an array of file names
files=('file1.txt' 'file2.txt' 'file3.txt')
# Loop over the array and print each file name
for file in "${files[@]}"; do
echo $file
done
# Output:
# file1.txt
# file2.txt
# file3.txt
In this example, we’ve declared an array of file names and then used a for loop to print each file name. This is a simple demonstration, but you can imagine how this could be expanded to include more complex operations on each file.
Related Topics: String Manipulation and File I/O
While arrays are powerful, they are just one tool in the Bash toolkit. To become a proficient Bash scripter, you’ll need to master several other topics, including string manipulation and file input/output (I/O).
String manipulation in Bash involves operations like splitting strings, replacing substrings, and concatenating strings. These operations often go hand-in-hand with array manipulation, as you might need to split a string into an array, or join an array into a string.
File I/O in Bash involves reading from and writing to files. This is crucial for scripts that need to process data stored in files. You might need to read a file into an array, or write an array to a file.
Further Resources for Bash Array Mastery
If you’re interested in diving deeper into Bash arrays, here are some resources that you might find helpful:
- GNU Bash Manual: This is the official manual for Bash, and it includes a detailed section on arrays.
- Advanced Bash-Scripting Guide: This guide covers advanced topics in Bash scripting, including arrays.
- Introduction to Bash Arrays: This article from Opensource.com serves as an introduction to Bash arrays.
Wrapping Up: Declaring Arrays in Bash
In this comprehensive guide, we’ve explored the ins and outs of declaring arrays in Bash, a fundamental aspect of Bash scripting that enables you to manage and manipulate collections of data efficiently.
We began with the basics, learning how to declare, access, and manipulate arrays in Bash. We then delved into more advanced topics, such as simulating multi-dimensional arrays, working with associative arrays, and leveraging external tools like awk and sed for data handling.
Along the way, we tackled common challenges you might encounter when using arrays in Bash, such as off-by-one errors, uninitialized array elements, and handling spaces in array elements. We provided solutions and best practices to help you avoid these pitfalls and work effectively with arrays.
We also compared Bash arrays with other methods of data handling in Bash, giving you a sense of the broader landscape of tools available to you. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Bash Arrays | Native to Bash, versatile | Limited to one dimension, can have gaps in indices |
Awk | Powerful text processing, can split strings into arrays | Slower than native Bash, adds external dependencies |
Sed | Useful for find and replace operations on strings | Slower than native Bash, adds external dependencies |
Whether you’re a beginner just starting out with Bash or a seasoned scripter looking to brush up on your array handling skills, we hope this guide has provided you with a deeper understanding of Bash arrays and their capabilities.
The ability to declare and manipulate arrays in Bash is a powerful tool in your scripting toolkit. Now, you’re well equipped to handle collections of data in Bash effectively and efficiently. Happy scripting!