Bash Functions: Your Reference Guide to Linux Shell Script
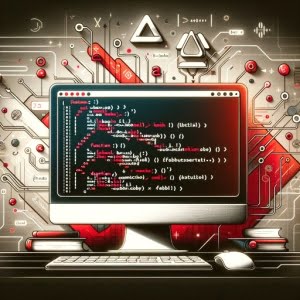
Are Bash functions leaving you puzzled? You’re not alone. Many developers find themselves in a maze when it comes to understanding and using Bash functions. But think of Bash functions as a well-oiled machine – they can automate repetitive tasks and make your scripts more efficient.
This guide will walk you through everything you need to know about Bash functions, from the basics to advanced techniques. We’ll cover everything from creating simple Bash functions, understanding their syntax, to more complex aspects like passing arguments, using local variables, and returning values.
So, let’s dive in and start mastering Bash functions!
TL;DR: How Do I Create a Function in Bash?
To create a function in Bash, you use the following syntax:
function_name() { command }
. This command defines a function namedfunction_name
that executes the command when called.
Here’s a simple example:
function greet() {
echo 'Hello, world!'
}
# Call the function
greet
# Output:
# Hello, world!
In this example, we’ve created a function named greet
that prints ‘Hello, world!’ when called. We then call the function by typing its name, greet
, which executes the command within the function and prints ‘Hello, world!’.
This is just a basic way to create and use a Bash function, but there’s much more to learn about Bash functions. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Crafting and Calling Bash Functions: A Beginner’s Guide
- Delving Deeper: Arguments, Variables, and Returns
- Exploring Alternatives: Aliases and External Scripts
- Troubleshooting Bash Functions: Common Issues and Fixes
- Bash Shell and Scripting: The Foundation of Bash Functions
- Bash Functions in the Bigger Picture
- Wrapping Up: Mastering Bash Functions
Crafting and Calling Bash Functions: A Beginner’s Guide
Creating and invoking Bash functions is a straightforward process. Let’s start by crafting a simple Bash function. In this example, we’ll create a function to greet a user by their name.
function greet_user() {
echo "Hello, $1!"
}
# Call the function with an argument
greet_user 'Anton'
# Output:
# Hello, Anton!
In this example, $1
is a special variable that represents the first argument passed to the function. When we call greet_user 'Anton'
, the function replaces $1
with ‘Anton’ and prints ‘Hello, Anton!’.
Creating a function in Bash allows us to reuse this block of code whenever we need to greet a user. This is a huge advantage as it prevents us from having to write the same code over and over again, improving the readability and maintainability of our scripts.
However, it’s crucial to remember that Bash functions don’t behave exactly like functions in other programming languages. For instance, they don’t allow us to return a value like we would in languages like Python or Java. Instead, Bash functions return a status (a 0 for success and a non-zero integer for failure), which can sometimes be a pitfall for beginners.
Delving Deeper: Arguments, Variables, and Returns
As you become more comfortable with Bash functions, you can start exploring more complex aspects. Let’s look at passing arguments, using local variables, and returning values.
Passing Arguments to Bash Functions
Bash functions can accept arguments, much like functions in other programming languages. These arguments are accessible within the function through special variables $1
, $2
, $3
, and so on, corresponding to the first, second, third, etc., arguments.
Here’s an example of a Bash function that takes two arguments:
function greet_user() {
echo "Hello, $1! You are from $2."
}
# Call the function with two arguments
greet_user 'Anton' 'Germany'
# Output:
# Hello, Anton! You are from Germany.
In this example, $1
is replaced by ‘Anton’, and $2
is replaced by ‘Germany’.
Using Local Variables
In Bash functions, you can declare local variables using the local
keyword. These variables are only accessible within the function they are declared in.
function display_greeting() {
local greeting='Hello'
echo "$greeting, $1!"
}
display_greeting 'Anton'
# Output:
# Hello, Anton!
In this function, greeting
is a local variable. It’s only accessible within display_greeting
.
Returning Values
As mentioned earlier, Bash functions return a status rather than a value. However, you can simulate the behavior of returning a value by using the echo
command inside the function and capturing its output when you call the function.
function get_greeting() {
local greeting="Hello, $1!"
echo $greeting
}
# Call the function and capture the output
output=$(get_greeting 'Anton')
echo $output
# Output:
# Hello, Anton!
In this example, get_greeting
echoes a greeting, which we capture into the output
variable when we call the function. We can then use output
as if it were the returned value of the function.
These advanced techniques can make your Bash functions more flexible and powerful, allowing you to write more complex and efficient scripts.
Exploring Alternatives: Aliases and External Scripts
While Bash functions are a powerful tool for managing tasks, they aren’t the only approach. Let’s delve into some alternatives, such as using aliases and external scripts.
Bash Aliases: Quick Commands
An alias in Bash is a sort of shortcut for a command or a series of commands. They are particularly useful for long commands that you use frequently.
Here’s an example of how to create an alias:
alias ll='ls -l'
# Use the alias
ll
# Output:
# total 0
# -rw-r--r-- 1 anton staff 0 Feb 2 12:34 file1
# -rw-r--r-- 1 anton staff 0 Feb 2 12:34 file2
In this example, we’ve created an alias ll
for the command ls -l
, which lists files in long format. Now, whenever we type ll
, Bash will execute ls -l
.
External Scripts: Reusable Code
Another alternative is to write your tasks in external scripts. This is especially useful when your tasks involve complex logic or when you want to reuse the same code across multiple scripts.
Here’s an example of an external script:
# greet_user.sh
echo "Hello, $1!"
# Call the script
bash greet_user.sh 'Anton'
# Output:
# Hello, Anton!
In this example, we’ve written a script greet_user.sh
that greets a user. We can call this script from any other script or Bash session, making our code highly reusable.
While aliases and external scripts can be powerful, they also have their downsides. Aliases, for instance, are only available in the current shell session by default, and they can’t accept arguments like functions can. External scripts, on the other hand, can be overkill for simple tasks and can make your code harder to manage if you have too many of them.
In conclusion, while Bash functions are a powerful tool for managing tasks in Bash, they are not the only tool at your disposal. Depending on your needs, aliases or external scripts may be a better fit. The key is to understand the strengths and weaknesses of each approach and to choose the one that best fits your needs.
Troubleshooting Bash Functions: Common Issues and Fixes
Like any programming tool, Bash functions have their quirks and challenges. Let’s discuss some common issues you might encounter when working with Bash functions, and how to solve them.
Scope Issues
One common issue is scope. In Bash, variables are global by default. This means that a variable declared in a function is accessible outside the function, which can lead to unexpected behavior.
Here’s an example:
function set_name() {
name='Anton'
}
set_name
echo $name
# Output:
# Anton
In this example, name
is accessible even outside set_name
. To avoid this, you can declare name
as a local variable using the local
keyword.
Error Handling
Another common issue is error handling. Bash functions don’t throw exceptions like functions in other languages, so you need to manually check for errors.
Here’s an example of how to do this:
function risky_operation() {
command_that_might_fail
if [ $? -ne 0 ]; then
echo 'An error occurred!' >&2
return 1
fi
}
risky_operation
# Output (if an error occurs):
# An error occurred!
In this function, $?
is a special variable that holds the status of the last command. If the command fails (i.e., its status is not 0), we print an error message and return 1 to indicate failure.
Remember, Bash functions are a powerful tool, but they also have their quirks. Understanding these issues and how to handle them can help you use Bash functions more effectively.
Bash Shell and Scripting: The Foundation of Bash Functions
To fully grasp the concept of Bash functions, it’s important to understand the environment they operate in – the Bash shell and Bash scripting.
The Bash Shell: Your Command Line Interface
Bash (Bourne Again SHell) is a command-line interface for interacting with the operating system. It’s where you type commands that the operating system executes. Bash is the default shell for most Unix-based systems, including Linux and macOS.
Here’s an example of a simple Bash command:
echo 'Hello, world!'
# Output:
# Hello, world!
In this example, echo
is a command that prints its arguments. We’re passing the string ‘Hello, world!’ as an argument, so Bash prints ‘Hello, world!’.
Bash Scripting: Automating Tasks
Bash scripting is the art of automating tasks by writing sequences of commands in a file, known as a Bash script. When you run the script, Bash executes the commands in the order they appear in the file.
Here’s an example of a simple Bash script:
# greet.sh
echo 'Hello, world!'
You can run this script with the command bash greet.sh
, and Bash will execute the commands in the script, printing ‘Hello, world!’.
Bash functions are a part of Bash scripting. They allow you to group commands into reusable blocks, making your scripts more organized and efficient. Understanding the Bash shell and Bash scripting is key to mastering Bash functions.
Bash Functions in the Bigger Picture
Bash functions, while seemingly simple, play a crucial role in larger scripts or projects. Their ability to encapsulate a series of commands into a reusable block makes them an indispensable tool for any Bash scripter.
Integrating Bash Functions in Larger Scripts
In larger scripts, Bash functions can be used to modularize the code. Each function can perform a specific task, making the script easier to read and maintain. For instance, in a script that manages user accounts, you might have functions like create_user
, delete_user
, list_users
, etc.
Exploring Related Concepts
Once you’ve mastered Bash functions, there are plenty of related concepts to explore. Shell scripting best practices, for instance, can help you write more robust and efficient scripts. Command line tools can help you perform complex tasks without having to write a lot of code.
Further Resources for Bash Function Mastery
To deepen your understanding of Bash functions and related concepts, here are some resources you might find useful:
- Advanced Bash-Scripting Guide: An in-depth exploration of Bash scripting, including functions.
Bash Guide for Beginners: A beginner-friendly guide to Bash, including scripting and functions.
GNU Bash Manual: The official manual for Bash, which covers all its features in detail, including functions.
Wrapping Up: Mastering Bash Functions
In this comprehensive guide, we’ve explored the ins and outs of Bash functions, a fundamental tool for automating tasks and making your scripts more efficient in the Bash shell.
We began with the basics, learning how to create and call Bash functions. We then delved into more complex aspects, such as passing arguments, using local variables, and returning values. Along the way, we tackled common issues you might encounter when working with Bash functions, such as scope issues and error handling, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to managing tasks in Bash, such as using aliases and external scripts. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Bash Functions | Reusable, Can accept arguments | Limited return values |
Bash Aliases | Quick shortcuts for commands | Can’t accept arguments, Session-specific |
External Scripts | Highly reusable, Good for complex tasks | Can be overkill for simple tasks |
Whether you’re just starting out with Bash functions or you’re looking to level up your Bash scripting skills, we hope this guide has given you a deeper understanding of Bash functions and their capabilities.
With their ability to encapsulate a series of commands into a reusable block, Bash functions are a powerful tool for any Bash scripter. Now, you’re well equipped to enjoy those benefits. Happy scripting!