Install Dev Dependencies with NPM | Node.js User Guide
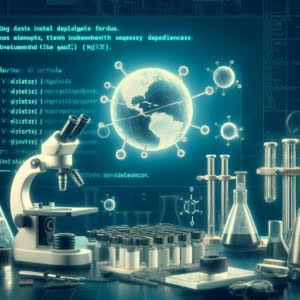
To ensure smooth project development at IOFLOOD, managing development dependencies is always crucial. By using npm, we have developed processes to effortlessly install necessary development dependencies with a few simple commands. To help others that are facing similar issues, we’ve created this guide.
This guide will walk you through the process of installing development dependencies using npm, ensuring your development environment is both powerful and efficient. By understanding how to manage these dependencies, you can streamline your development process, avoid common pitfalls, and ensure that your project is built on a solid foundation.
Let’s streamline our project setup and make development a breeze!
TL;DR: How Do I Install Development Dependencies Using npm?
To install a package as a development dependency in your Node.js project using npm, execute the command
npm install --save-dev
in your terminal.
Here’s a quick example:
npm install eslint --save-dev
# Output:
# + [email protected]
# added 1 package in 0.567s
In this example, we’ve installed eslint
, a popular linting tool for JavaScript, as a development dependency. This command adds eslint
to the devDependencies
section of your project’s package.json
file, ensuring it’s only used during development and not in your production build.
Curious about more npm commands and best practices for managing your Node.js project’s dependencies? Keep reading for a deeper dive into the world of npm and development dependencies.
Table of Contents
Basic npm Commands for Beginners
Understanding the --save-dev
Flag
When diving into Node.js projects, one of the first steps is setting up your development environment with the necessary tools and libraries. These are known as development dependencies. They’re essential during the development phase but not required in the production environment. This distinction is crucial for maintaining an efficient and lightweight application.
The --save-dev
flag in npm commands plays a pivotal role in this process. By appending --save-dev
to your npm install command, you instruct npm to add the package under the devDependencies
section of your package.json
file. This differentiation ensures that the package is only installed in development environments.
Let’s look at an example of adding a package, nodemon
, which is a utility that monitors for any changes in your source and automatically restarts your server.
npm install nodemon --save-dev
# Output:
# + [email protected]
# added 75 packages from 53 contributors and audited 123 packages in 4.568s
In this code block, we’ve installed nodemon
as a development dependency. The output indicates the successful addition of nodemon
to our project, showing the number of packages added and the time taken for the operation. This command effectively segregates nodemon
to the development phase, ensuring that it doesn’t bloat the production build. Understanding and utilizing the --save-dev
flag is a foundational skill for any developer working in the Node.js ecosystem.
Advanced Install Techniques: npm
Managing Package Versions with npm
As you progress in your development journey, understanding how to manage package versions becomes crucial. npm allows you to specify which version of a package you wish to install as a development dependency, ensuring compatibility and stability within your project.
Consider you want to install a specific version of the webpack
package. You can achieve this by appending @
followed by the version number to the package name in your install command.
npm install [email protected] --save-dev
# Output:
# + [email protected]
# added 297 packages from 181 contributors in 27.45s
In this example, specifying @4.44.2
ensures that version 4.44.2 of webpack
is installed. This level of precision is invaluable when working on projects that require a specific package version for compatibility reasons.
Installing Multiple Dev Dependencies
Installing multiple development dependencies in one go can significantly streamline your setup process. npm supports this functionality, allowing you to install several packages with a single command.
Imagine you’re setting up a new project and need both babel
and jest
for development. You can install them simultaneously using npm.
npm install babel jest --save-dev
# Output:
# added 1245 packages in 58.92s
This command installs both babel
and jest
as development dependencies, significantly reducing setup time. It demonstrates npm’s flexibility and efficiency in managing project dependencies.
Understanding the package.json
File
The package.json
file acts as the heart of your Node.js project, storing crucial information about the project and its dependencies. When you install development dependencies, npm updates this file, adding each package to the devDependencies
section.
Understanding how to read and interpret the package.json
file is essential for managing your project’s dependencies effectively. It allows you to quickly assess which development dependencies are installed and their respective versions, providing insight into your project’s setup at a glance.
Exploring npm Alternatives
Why Consider Alternatives?
When managing development dependencies, npm is not the only game in town. As your projects grow in complexity, exploring alternative tools can provide insights into optimizing your development workflow. One notable alternative is Yarn, a package manager that has gained popularity for its speed and reliability.
Yarn: A Viable npm Alternative
Yarn emerged as a strong contender to npm, introducing features like deterministic package installation and an offline mode that npm later adopted. To install a development dependency using Yarn, the command slightly differs from npm but retains simplicity.
yarn add <package-name> --dev
# Output:
# success Saved 1 new dependency.
# └─ <package-name>@<version>
In this example, the --dev
flag functions similarly to npm’s --save-dev
, specifying that the package is for development purposes. The output is more concise, focusing on the success of the operation and the details of the added package.
npm vs. Yarn: Comparing Tools
While npm and Yarn share many functionalities, there are differences worth noting. Yarn’s lock file, yarn.lock
, is created to ensure that the same versions of dependencies are installed across all environments, enhancing consistency. npm also introduced a similar feature with package-lock.json
, but Yarn was the pioneer.
Yarn’s installation process tends to be faster than npm’s, thanks to its efficient caching and parallel installation features. However, npm has made significant improvements in speed and efficiency with recent updates, closing the gap between the two.
Choosing between npm and Yarn often comes down to personal preference or specific project requirements. Both tools are powerful allies in managing development dependencies, but understanding their nuances can help you make an informed decision for your workflow.
Resolving Issues of npm Installs
Version Conflicts and Resolutions
One common hurdle when using npm install
for development dependencies is encountering version conflicts. These conflicts occur when a project requires a specific version of a package that is incompatible with another required package. Addressing this requires a careful balance to ensure all dependencies work harmoniously.
Consider a scenario where your project requires webpack
version 4.44.2
, but another dependency necessitates webpack
version 5.0.0
or higher. Attempting to install these conflicting versions can lead to errors. To troubleshoot, you can use the npm list
command to identify the dependencies causing the conflict.
npm list webpack
# Output:
# [email protected]
# ├─┬ [email protected]
# └─┬ [email protected]
This output shows the different versions of webpack
required by your project’s dependencies. Understanding this hierarchy allows you to make informed decisions, such as updating other packages to be compatible with the required webpack
version or finding alternative packages that align with your project’s needs.
Handling Installation Failures
Installation failures are another common issue when managing development dependencies with npm. These can be caused by a variety of factors, including network issues, corrupted npm cache, or permissions problems.
To address an installation failure, first, try clearing the npm cache with the command npm cache verify
. This ensures that any corrupt cache data is removed and often resolves the issue.
npm cache verify
# Output:
# Cache verified and compressed (~/.npm/_cacache):
# Content verified: 0 (0 bytes)
# Index entries: 0
# Finished in 0.02s
If the issue persists, check your network connection and ensure you have the necessary permissions to install packages. In some cases, using an alternative registry or setting a proxy might be required.
Navigating npm installation issues can be challenging, but understanding these common pitfalls and how to address them can significantly streamline your development workflow. By employing these troubleshooting techniques, you can ensure a smoother experience when managing your project’s development dependencies.
Understanding Dev Dependencies
The Role of Development Dependencies
In the realm of Node.js and JavaScript development, the term ‘dependencies’ encompasses libraries and packages that your project needs to function correctly. However, not all dependencies are created equal. There’s a critical distinction between production dependencies and development dependencies — a distinction that npm, Node.js’s package manager, handles with precision.
Development dependencies, or ‘devDependencies’, are packages required only during the development phase of your project. These might include compilers like Babel, linters like ESLint, or testing frameworks like Jest. They are not needed in the production environment where your application runs for end-users.
To illustrate, let’s add a development dependency to a project. Suppose we’re working on a JavaScript application that needs webpack
for bundling our assets during development. Here’s how you would add webpack
as a development dependency using npm:
npm install webpack --save-dev
# Output:
# + [email protected]
# added 297 packages from 205 contributors and audited 9001 packages in 12.34s
This command installs the latest version of webpack
and adds it to the devDependencies
section of your package.json
file. The output indicates the package name and version installed, along with the number of packages added and the time taken for the operation. This process is crucial for ensuring that webpack
is available during development for asset compilation but remains absent from the production environment, thereby keeping the production build lean and optimized.
npm’s Distinction Between Dependencies
npm makes managing these two types of dependencies straightforward. In your package.json
file, dependencies required for your application to run in production are listed under dependencies
, while those needed only for development live under devDependencies
. This clear separation helps manage your project’s packages efficiently, ensuring that only essential packages are included in your production build.
Understanding the fundamental role of development dependencies and npm’s method for managing them sets the stage for efficient project management. It underscores the importance of precise dependency management in creating streamlined, efficient applications.
Practical Uses: Dev Dependency
Integrating Dev Dependencies into Workflows
The management of development dependencies extends beyond the mere installation. It plays a pivotal role in the broader context of project workflows, continuous integration (CI), and deployment strategies. Efficient management of these dependencies ensures that your development environment mirrors the CI/CD pipeline, leading to fewer surprises during deployment.
For instance, ensuring that your linter and test suites specified as dev dependencies run in both your local environment and within your CI pipeline can help catch errors early. Here’s how you might configure a package.json
script to run ESLint:
"scripts": {
"lint": "eslint ."
}
# Output:
# Command to run: npm run lint
# Expected output: Linting complete with no errors (or list of linting errors)
This script, when run as part of both your local development workflow and your CI pipeline, ensures consistency in code quality checks. It illustrates the importance of dev dependencies in maintaining code standards and preventing bugs from reaching production.
Continuous Integration and Deployment Considerations
Incorporating development dependencies into your CI/CD pipeline requires careful consideration. For example, dependencies used for testing should be installed and executed as part of your pipeline to ensure that tests pass before any code is deployed to production. Automating this process minimizes the risk of deploying code that hasn’t been thoroughly tested or doesn’t meet coding standards.
Further Resources for npm Mastery
To deepen your understanding of npm and development dependency management, consider exploring the following resources:
- npm Documentation: The official npm documentation is a comprehensive resource covering all aspects of npm, from basic usage to advanced features.
Node.js Best Practices: A GitHub repository that outlines best practices for Node.js development, including dependency management.
Understanding npm for Frontend Development: An article on Smashing Magazine that explores how npm can be utilized effectively in frontend development projects.
These resources provide valuable insights into npm and its role in modern development workflows, helping you to leverage npm more effectively in your projects.
Recap: Dev Dependencies and npm
In this comprehensive guide, we’ve navigated the nuances of managing development dependencies in your Node.js projects using npm. From the initial setup to advanced management techniques, understanding how to effectively handle these dependencies is key to a streamlined development process.
We began with the basics, illustrating how to use the npm install --save-dev
command to add development dependencies to your project. This foundational knowledge sets the stage for more complex dependency management tasks, ensuring your development environment is equipped with the necessary tools.
Moving forward, we explored advanced npm features, such as specifying package versions and installing multiple dependencies simultaneously. These intermediate skills allow for precise control over your project’s dependency tree, reducing potential conflicts and ensuring compatibility.
We also considered alternative tools like Yarn, highlighting the importance of choosing the right package manager based on your project’s needs. Understanding the strengths and weaknesses of each tool can significantly impact your development workflow efficiency.
Feature | npm | Yarn |
---|---|---|
Speed | Moderate | Fast |
Caching | Efficient | More Efficient |
Deterministic Installs | Yes | Yes |
As we conclude, remember that managing development dependencies is more than just running installation commands. It’s about understanding the ecosystem, knowing when to update or replace dependencies, and troubleshooting issues as they arise. These best practices and tips will serve as a valuable reference as you continue to develop and maintain Node.js applications.
With the knowledge and examples provided in this guide, you’re now better equipped to manage development dependencies effectively. Whether you’re starting a new project or optimizing an existing one, these insights will help ensure a robust and efficient development environment. Happy coding!