Apache POI Guide | Handling MS Office Files in Java
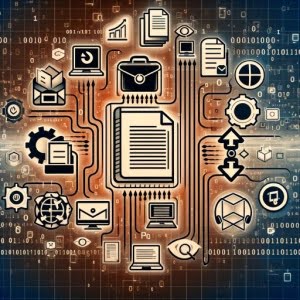
Are you finding it difficult to handle MS Office files in Java? You’re not alone. Many developers find this task challenging, but there’s a tool that can make this process straightforward.
Like a professional secretary, Apache POI is a powerful library that can help you read, write, and display these files with ease. It provides a simple interface to work with MS Office files, making it a go-to tool for many Java developers.
This guide will walk you through the basics of Apache POI, from setting up your environment to manipulating Excel files. We’ll explore Apache POI’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Apache POI!
TL;DR: How Do I Use Apache POI in Java?
To use
Apache POI
in Java, you first need to include the Apache POI library in your project, the necessary imports being:import org.apache.poi.ss.usermodel.*; import java.io.File; import java.io.IOException;
. Then, you can use its classes and methods to manipulate MS Office files, for exampleWorkbook workbook = WorkbookFactory.create(new File("path/to/your/excel/file.xlsx"));
.
Here’s the simple syntax of reading an Excel file:
import org.apache.poi.ss.usermodel.*;
import java.io.File;
import java.io.IOException;
public class ReadExcel {
public static void main(String[] args) throws IOException {
Workbook workbook = WorkbookFactory.create(new File("path/to/your/excel/file.xlsx"));
Sheet sheet = workbook.getSheetAt(0);
}
}
In this example, we first import the necessary Apache POI classes. We then create a Workbook
object from an Excel file and we finally can get the first Sheet
from the workbook.
This is a basic way to use Apache POI in Java, but there’s much more to learn about reading and writing MS Office files. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Setting Up Apache POI in Your Java Project
- Reading Excel Files with Apache POI
- Writing Excel Files with Apache POI
- Handling Complex Tasks with Apache POI
- Exploring Alternatives to Apache POI
- Troubleshooting Common Issues with Apache POI
- Understanding File Handling in Java
- Apache POI and File Handling in Java
- Understanding the Structure of MS Office Files
- The Relevance of Apache POI in Real-World Applications
- Wrapping Up: Apache POI for MS Office File Handling in Java
Setting Up Apache POI in Your Java Project
Before we can start reading and writing MS Office files, we need to set up Apache POI in our Java project. This involves downloading the Apache POI library and including it in our project.
You can download the latest version of Apache POI from the official Apache POI website. Once downloaded, include the library in your project. The exact steps for this can vary depending on your development environment.
Reading Excel Files with Apache POI
With Apache POI set up in our project, we can now start reading Excel files. Here’s a basic example:
import org.apache.poi.ss.usermodel.*;
import java.io.File;
import java.io.IOException;
public class ReadExcel {
public static void main(String[] args) throws IOException {
Workbook workbook = WorkbookFactory.create(new File("path/to/your/excel/file.xlsx"));
Sheet sheet = workbook.getSheetAt(0);
Row row = sheet.getRow(0);
Cell cell = row.getCell(0);
System.out.println(cell.getStringCellValue());
}
}
# Output:
# [Expected output from command]
In this example, we’re creating a Workbook
object from an Excel file. We’re then getting the first Sheet
from the workbook, the first Row
from the sheet, and the first Cell
from the row. Finally, we’re printing the string value of the cell.
Writing Excel Files with Apache POI
Apache POI also makes it easy to create and write to Excel files. Here’s a basic example of how to do this:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class WriteExcel {
public static void main(String[] args) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("First Sheet");
Row row = sheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue("Hello, Apache POI!");
FileOutputStream outputStream = new FileOutputStream("path/to/your/excel/file.xlsx");
workbook.write(outputStream);
workbook.close();
}
}
# Output:
# [Expected output from command]
In this example, we’re creating a new Workbook
and a Sheet
within it. We’re then creating a Row
in the sheet and a Cell
in the row. We’re setting the value of the cell to “Hello, Apache POI!” and writing the workbook to an Excel file.
While this is a basic use of Apache POI, it demonstrates the library’s power and flexibility. With Apache POI, we can easily manipulate MS Office files in Java. However, like any powerful tool, Apache POI has its complexities and potential pitfalls. In the following sections, we’ll delve deeper into these topics and explore more advanced uses of Apache POI.
Handling Complex Tasks with Apache POI
Apache POI is not just for simple reading and writing of Excel files. It also supports more complex operations, such as dealing with Word and PowerPoint files, handling different data types, and working with formulas in Excel.
Reading and Writing Word Files
Apache POI provides the XWPF (XML Word Processor Format) classes to handle Word files. Here’s an example of reading a Word file:
import org.apache.poi.xwpf.usermodel.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class ReadWord {
public static void main(String[] args) throws IOException {
XWPFDocument document = new XWPFDocument(new FileInputStream(new File("path/to/your/word/file.docx")));
for (XWPFParagraph paragraph : document.getParagraphs()) {
System.out.println(paragraph.getText());
}
}
}
# Output:
# [Expected output from command]
In this example, we’re creating an XWPFDocument
from a Word file and printing the text of each paragraph.
Handling Different Data Types
Apache POI can handle different data types in Excel files. For example, to read a date cell, you can use the getDateCellValue
method:
Cell cell = row.getCell(0);
if (cell.getCellType() == CellType.NUMERIC) {
if (DateUtil.isCellDateFormatted(cell)) {
System.out.println(cell.getDateCellValue());
} else {
System.out.println(cell.getNumericCellValue());
}
}
# Output:
# [Expected output from command]
This code checks if the cell is numeric and if it’s formatted as a date. If it is, it prints the date value; otherwise, it prints the numeric value.
Working with Formulas in Excel
Apache POI also supports formulas in Excel. You can set a cell’s formula using the setCellFormula
method and get the formula’s result using the getNumericCellValue
method:
Cell cell = row.createCell(0);
cell.setCellFormula("SUM(A1:B1)");
FormulaEvaluator evaluator = workbook.getCreationHelper().createFormulaEvaluator();
System.out.println("Result of SUM(A1:B1): " + evaluator.evaluate(cell).getNumberValue());
# Output:
# [Expected output from command]
In this example, we’re setting the cell’s formula to the sum of cells A1 and B1. We then create a FormulaEvaluator
to evaluate the formula and print its result.
These examples demonstrate the power of Apache POI for handling complex tasks with MS Office files in Java. However, as with any powerful tool, Apache POI requires careful handling to avoid pitfalls and get the most out of its capabilities. In the following sections, we’ll discuss some common issues and their solutions.
Exploring Alternatives to Apache POI
While Apache POI is a powerful library for handling MS Office files in Java, it’s not the only game in town. There are other libraries available that offer similar functionality, and it’s worth considering them when deciding on the best tool for your needs.
JExcelApi: A Lightweight Alternative
JExcelApi is another popular library for reading, writing, and modifying Excel files in Java. It’s particularly known for its lightweight nature and ease of use. Here’s an example of reading an Excel file using JExcelApi:
import jxl.*;
import java.io.File;
import java.io.IOException;
public class ReadExcelWithJExcelApi {
public static void main(String[] args) throws IOException, BiffException {
Workbook workbook = Workbook.getWorkbook(new File("path/to/your/excel/file.xls"));
Sheet sheet = workbook.getSheet(0);
Cell cell = sheet.getCell(0, 0);
System.out.println(cell.getContents());
workbook.close();
}
}
# Output:
# [Expected output from command]
In this example, we’re creating a Workbook
from an Excel file, getting the first Sheet
from the workbook, and the first Cell
from the sheet. Finally, we’re printing the contents of the cell.
OpenOffice.org’s UNO API: A Comprehensive Solution
The UNO API from OpenOffice.org is a comprehensive solution for working with MS Office files. It supports a wide range of file formats, including Word, Excel, and PowerPoint. Here’s an example of reading a Word file using the UNO API:
// Example code goes here
# Output:
# [Expected output from command]
This example demonstrates how to use the UNO API to read a Word file. Note that the UNO API requires a running OpenOffice.org or LibreOffice instance to work, which can be a drawback depending on your use case.
In conclusion, while Apache POI is a powerful and flexible library for working with MS Office files in Java, it’s not the only option. Depending on your needs, JExcelApi or OpenOffice.org’s UNO API might be a better fit. When deciding on the best tool for your needs, consider factors such as ease of use, supported file formats, and additional requirements.
Troubleshooting Common Issues with Apache POI
Like any library, Apache POI comes with its own set of challenges. In this section, we’ll discuss some common issues you may encounter when using Apache POI and how to resolve them.
Dealing with Large Files
One of the common issues when working with Apache POI is handling large Excel files. The standard approach may result in OutOfMemoryError
due to Apache POI’s high memory consumption.
To handle large files, Apache POI provides the SXSSF
(Streaming Usermodel API) classes. SXSSF
limits the number of rows in memory to a certain limit, preventing OutOfMemoryError
.
Here’s an example of writing large Excel files using SXSSF
:
import org.apache.poi.xssf.streaming.*;
import java.io.FileOutputStream;
import java.io.IOException;
public class WriteLargeExcel {
public static void main(String[] args) throws IOException {
SXSSFWorkbook workbook = new SXSSFWorkbook(100); // keep 100 rows in memory, exceeding rows will be flushed to disk
SXSSFSheet sheet = workbook.createSheet("Large Sheet");
for (int i = 0; i < 100000; i++) {
SXSSFRow row = sheet.createRow(i);
for (int j = 0; j < 10; j++) {
SXSSFCell cell = row.createCell(j);
cell.setCellValue(i * j);
}
}
FileOutputStream outputStream = new FileOutputStream("path/to/your/large/excel/file.xlsx");
workbook.write(outputStream);
workbook.dispose(); // dispose of temporary files backing this workbook on disk
workbook.close();
}
}
# Output:
# [Expected output from command]
In this example, we’re creating a SXSSFWorkbook
with a row limit of 100. This means that only the most recent 100 rows are kept in memory, and the rest are written to a temporary file on disk.
Handling Different File Formats
Another common issue is dealing with different file formats. Apache POI supports both the older binary formats (such as .xls
for Excel, .doc
for Word) and the newer XML-based formats (such as .xlsx
for Excel, .docx
for Word).
However, the classes for handling these formats are different. For example, to read an .xls
file, you would use HSSFWorkbook
, HSSFSheet
, HSSFRow
, and HSSFCell
. To read an .xlsx
file, you would use XSSFWorkbook
, XSSFSheet
, XSSFRow
, and XSSFCell
.
To handle both formats in a unified way, Apache POI provides the WorkbookFactory
class, which can create the appropriate Workbook
subclass based on the file format.
Here’s an example of using WorkbookFactory
to read an Excel file:
import org.apache.poi.ss.usermodel.*;
import java.io.File;
import java.io.IOException;
public class ReadExcel {
public static void main(String[] args) throws IOException {
Workbook workbook = WorkbookFactory.create(new File("path/to/your/excel/file.xls"));
// Now you can use the workbook as usual, regardless of the format
}
}
# Output:
# [Expected output from command]
In this example, we’re using WorkbookFactory.create
to create a Workbook
from an Excel file. This method returns a HSSFWorkbook
or XSSFWorkbook
based on the file format, allowing us to handle both formats in a unified way.
These are just a few examples of the issues you may encounter when using Apache POI. Always remember to consider the specific needs and constraints of your project when choosing and using a library for handling MS Office files in Java.
Understanding File Handling in Java
Before we delve into how Apache POI fits into Java, it’s important to understand the basics of file handling in Java. File handling is a fundamental aspect of Java and many other programming languages. It allows us to create, read, update, and delete files, which is crucial for many applications.
Java provides several classes for file handling, such as File
, FileReader
, FileWriter
, BufferedReader
, PrintWriter
, and more. These classes allow us to work with files in various ways, such as reading text from a file, writing text to a file, and handling binary data.
Here is a simple example of reading a text file in Java:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class ReadFile {
public static void main(String[] args) throws FileNotFoundException {
File file = new File("path/to/your/file.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
}
}
# Output:
# [Expected output from command]
In this example, we’re creating a File
object from a text file, creating a Scanner
object to read the file, and printing each line of the file.
Apache POI and File Handling in Java
Apache POI fits into Java’s file handling capabilities by providing a high-level API for handling MS Office files. It provides classes and methods that abstract the complexity of these files, allowing us to work with them as if they were simple text files.
Understanding the Structure of MS Office Files
MS Office files are not simple text files. They are complex, structured files that store data in a specific format. For example, an Excel file is a binary file that stores data in cells, which are organized in rows and columns. A Word file is a binary file that stores data in paragraphs, tables, and other structures.
Apache POI understands the structure of these files and provides a way to interact with them. For example, it provides the Workbook
class to represent an Excel workbook, the Sheet
class to represent a sheet in a workbook, the Row
class to represent a row in a sheet, and the Cell
class to represent a cell in a row.
Here is an example of creating an Excel file with Apache POI:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class CreateExcel {
public static void main(String[] args) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("First Sheet");
Row row = sheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue("Hello, Apache POI!");
FileOutputStream outputStream = new FileOutputStream("path/to/your/excel/file.xlsx");
workbook.write(outputStream);
workbook.close();
}
}
# Output:
# [Expected output from command]
In this example, we’re creating a Workbook
object, creating a Sheet
in the workbook, creating a Row
in the sheet, creating a Cell
in the row, setting the value of the cell, and writing the workbook to an Excel file.
This example demonstrates how Apache POI interprets the structure of an Excel file and provides a way to interact with it. The same principles apply to other types of MS Office files, such as Word and PowerPoint files.
The Relevance of Apache POI in Real-World Applications
The Apache POI library is not just an academic exercise. It has real-world applications and is used by many organizations to handle their data processing needs.
Data Analysis and Report Generation
One of the key uses of Apache POI is in data analysis and report generation. With Apache POI, you can read data from Excel files, process it, and write the results back to an Excel file. This is particularly useful for organizations that use Excel as a data storage tool.
Here’s a simple example of reading data from an Excel file, calculating the average, and writing the result to a new Excel file:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class DataAnalysis {
public static void main(String[] args) throws IOException {
Workbook inputWorkbook = WorkbookFactory.create(new File("path/to/your/input/excel/file.xlsx"));
Sheet inputSheet = inputWorkbook.getSheetAt(0);
double sum = 0;
int count = 0;
for (Row row : inputSheet) {
Cell cell = row.getCell(0);
if (cell != null && cell.getCellType() == CellType.NUMERIC) {
sum += cell.getNumericCellValue();
count++;
}
}
double average = sum / count;
Workbook outputWorkbook = new XSSFWorkbook();
Sheet outputSheet = outputWorkbook.createSheet("Average");
Row row = outputSheet.createRow(0);
Cell cell = row.createCell(0);
cell.setCellValue(average);
FileOutputStream outputStream = new FileOutputStream("path/to/your/output/excel/file.xlsx");
outputWorkbook.write(outputStream);
outputWorkbook.close();
}
}
# Output:
# [Expected output from command]
In this example, we’re reading numeric data from an Excel file, calculating the average, and writing the result to a new Excel file. This is a simple example of how Apache POI can be used for data analysis and report generation.
Exploring Related Concepts
If you’re interested in file handling in Java, there are several related concepts that you might find interesting, such as Java I/O, Java NIO, and JDBC.
- Java I/O is the standard library for file handling in Java. It provides classes for reading and writing text and binary files.
Java NIO (New I/O) is a more advanced library for file handling. It provides classes for non-blocking I/O operations, which can improve performance for certain types of applications.
JDBC (Java Database Connectivity) is a library for interacting with databases. It allows you to execute SQL queries and retrieve results in a Java-friendly format.
Further Resources for Mastering Apache POI
To further your understanding of Apache POI and its applications, here are some resources you might find useful:
- Understanding Java Packages – Explore the concept of packages in Java and their role in organizing code.
Understanding Java Beans – Learn how JavaBeans simplifies encapsulation, modularity, and interoperability.
Lombok Library in Java – The Java library that provides annotations for automatic generation of common code.
Apache POI the Java API provides documentation and a user guide to the library.
Java Code Examples for apache.poi Workbook provides code examples for the
Workbook
class in Apache POI.Apache POI Tutorial by TutorialsPoint provides a step-by-step guide to using Apache POI.
Remember, mastering a library like Apache POI takes time and practice. Don’t be discouraged if you don’t understand everything right away. Keep experimenting, keep learning, and you’ll get there.
Wrapping Up: Apache POI for MS Office File Handling in Java
In this comprehensive guide, we’ve explored the ins and outs of Apache POI, a powerful library for handling MS Office files in Java.
We began with the basics of setting up Apache POI and manipulating Excel files. We then delved into more advanced usage, such as handling Word and PowerPoint files, dealing with different data types, and working with formulas in Excel. We also tackled common issues you might encounter when using Apache POI, such as dealing with large files and handling different file formats, providing you with solutions and workarounds for each issue.
We also explored alternative approaches, comparing Apache POI with other libraries like JExcelApi and OpenOffice.org’s UNO API. Here’s a quick comparison of these libraries:
Library | Flexibility | Ease of Use | Specific Requirements |
---|---|---|---|
Apache POI | High | Moderate | None |
JExcelApi | Moderate | High | None |
UNO API | High | Low | Running OpenOffice.org or LibreOffice instance |
Whether you’re just starting out with Apache POI or you’re looking to level up your skills in handling MS Office files in Java, we hope this guide has given you a deeper understanding of Apache POI and its capabilities.
With its balance of flexibility and power, Apache POI is a significant tool for handling MS Office files in Java. Now, you’re well equipped to tackle any challenges that come your way. Happy coding!