Java Expressions Explained: A Detailed Guide
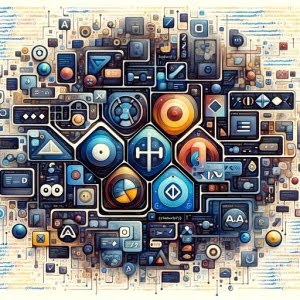
Are you finding it challenging to understand Java expressions? You’re not alone. Many developers find themselves puzzled when it comes to handling Java expressions, but we’re here to help.
Think of Java expressions as the building blocks of a complex structure – they are the fundamental units of calculation in your Java code, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of understanding Java expressions, from their basic use to more advanced techniques. We’ll cover everything from the basics of Java expressions to more advanced usage scenarios, as well as alternative approaches.
Let’s get started and master Java expressions!
TL;DR: What are Java Expressions?
In Java, an expression, such as
int sum = 1 + 2
, is a construct made up of variables, operators, and method invocations that evaluates to a single value. which are constructed according to the syntax of the language, . For instance, consider the following code snippet:
int sum = 10 + 20;
System.out.println(sum);
// Output:
// 30
In this example, 10 + 20
is a Java expression which evaluates to 30
. The variable sum
is then assigned this value.
This is a basic example of a Java expression, but there’s much more to learn about them, including their types and how they are used in Java programming. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Understanding Java Expressions: The Basics
- Advanced Java Expressions: A Deeper Dive
- Alternative Approaches to Java Expressions
- Troubleshooting Java Expressions: Common Errors and Solutions
- The Fundamentals: Digging Deeper into Java Expressions
- Java Expressions in the Real World
- Wrapping up Expressions in Java
Understanding Java Expressions: The Basics
Java expressions are the fundamental constructs in Java programming that evaluate to a single value. They can be made up of variables, operators, and method invocations, all constructed according to the syntax of the language.
There are several types of Java expressions, including:
- Arithmetic expressions: These involve mathematical operations. For instance,
int result = 10 * 20;
is an arithmetic expression.
int result = 10 * 20;
System.out.println(result);
// Output:
// 200
In the above example, 10 * 20
is the arithmetic expression that evaluates to 200
. The variable result
is then assigned this value.
- Relational expressions: These expressions compare two values and determine the relationship between them. For example,
boolean isTrue = (10 > 9);
is a relational expression.
boolean isTrue = (10 > 9);
System.out.println(isTrue);
// Output:
// true
In this code snippet, (10 > 9)
is a relational expression that evaluates to true
. The variable isTrue
is then assigned this value.
- Logical expressions: These involve logical operations and return a boolean value. For instance,
boolean result = (10 > 9) && (20 > 10);
is a logical expression.
boolean result = (10 > 9) && (20 > 10);
System.out.println(result);
// Output:
// true
In the above example, (10 > 9) && (20 > 10)
is a logical expression that evaluates to true
. The variable result
is then assigned this value.
Understanding and using Java expressions effectively can greatly enhance your Java programming skills. However, it’s important to be aware of potential pitfalls, such as operator precedence and type compatibility, to avoid unexpected results.
Advanced Java Expressions: A Deeper Dive
As you progress in your Java journey, you’ll encounter more complex expressions. Here, we’ll discuss some of these advanced Java expressions.
Conditional Expressions
In Java, a conditional expression (also known as a ternary operator) is a simple form of if-else
statement that returns a value based on the result of a condition. It follows the syntax: condition ? value_if_true : value_if_false
.
int a = 10;
int b = 20;
int max = (a > b) ? a : b;
System.out.println(max);
// Output:
// 20
In this code block, (a > b) ? a : b
is a conditional expression that evaluates to 20
, which is then assigned to the variable max
. If a
was greater than b
, a
would have been the result.
Method Invocation Expressions
Method invocation expressions involve calling a method. The method returns a value that can be used in your Java program.
public class Main {
static int multiply(int a, int b) {
return a * b;
}
public static void main(String[] args) {
int result = multiply(10, 20);
System.out.println(result);
}
}
// Output:
// 200
In this example, multiply(10, 20)
is a method invocation expression that calls the multiply
method with 10
and 20
as arguments. The method then returns 200
, which is assigned to the variable result
.
Class Instance Creation Expressions
These expressions involve creating an instance of a class. The new
keyword is used followed by the class constructor.
public class Main {
static class Dog {
String name;
Dog(String name) {
this.name = name;
}
void bark() {
System.out.println(this.name + " says woof!");
}
}
public static void main(String[] args) {
Dog myDog = new Dog("Rover");
myDog.bark();
}
}
// Output:
// Rover says woof!
In this code snippet, new Dog("Rover")
is a class instance creation expression that creates a new Dog
object with the name Rover
. The bark
method is then called on this object.
Understanding these advanced Java expressions can significantly enhance your programming skills and help you write more efficient and effective code.
Alternative Approaches to Java Expressions
While Java expressions are a fundamental part of Java programming, there are alternative approaches to achieve the same results. These alternatives can provide a different perspective, allowing you to choose the approach that best suits your specific scenario.
Using Java Statements
Java statements can often achieve the same results as Java expressions. For example, an if-else
statement can be used in place of a conditional (ternary) expression.
int a = 10;
int b = 20;
int max;
if (a > b) {
max = a;
} else {
max = b;
}
System.out.println(max);
// Output:
// 20
In this code block, the if-else
statement achieves the same result as the conditional expression we saw earlier. The variable max
is assigned the larger of a
and b
.
Using Lambda Expressions
Lambda expressions, introduced in Java 8, provide a concise way to create anonymous methods. They can be used as an alternative to certain types of Java expressions.
import java.util.function.BiFunction;
public class Main {
public static void main(String[] args) {
BiFunction<Integer, Integer, Integer> multiply = (a, b) -> a * b;
int result = multiply.apply(10, 20);
System.out.println(result);
}
}
// Output:
// 200
In this example, the lambda expression (a, b) -> a * b
is used to define a function that multiplies two integers. This achieves the same result as the method invocation expression we saw earlier.
These alternative approaches can offer more flexibility and readability in certain scenarios. However, they may also have drawbacks, such as increased verbosity or complexity. Therefore, it’s important to consider the specific needs and constraints of your program when choosing an approach.
Troubleshooting Java Expressions: Common Errors and Solutions
While Java expressions are powerful tools, they can sometimes lead to unexpected results or errors. Here, we’ll discuss some common issues and how to resolve them.
Operator Precedence Issues
Java follows a specific order of operations, known as operator precedence. Misunderstanding this can lead to unexpected results.
int result = 1 + 2 * 3;
System.out.println(result);
// Output:
// 7
In this example, the multiplication operation is performed before the addition due to operator precedence, resulting in 7 rather than 9. To get the expected result, parentheses can be used to change the order of operations.
int result = (1 + 2) * 3;
System.out.println(result);
// Output:
// 9
Type Compatibility Issues
Java is a strongly typed language, meaning that the types of all variables and expressions must be compatible. Attempting to assign a value of one type to a variable of a different type can result in a compilation error.
int result = 10 / 3;
System.out.println(result);
// Output:
// 3
In this example, the division operation results in a decimal value, but because result
is an integer, the decimal part is discarded. To get the expected result, one or both of the operands should be a floating-point type.
double result = 10.0 / 3;
System.out.println(result);
// Output:
// 3.3333333333333335
Best Practices and Optimization
To write effective Java expressions, keep the following tips in mind:
- Understand operator precedence: Knowing the order in which operations are performed can help avoid unexpected results.
- Use parentheses for clarity: Even if they’re not necessary, parentheses can make your expressions easier to read and understand.
- Consider type compatibility: Be aware of the types of your variables and expressions to prevent compilation errors and achieve the expected results.
- Use meaningful variable names: This can make your code easier to read and maintain.
By understanding these common issues and how to resolve them, you can write more effective and reliable Java expressions.
The Fundamentals: Digging Deeper into Java Expressions
To fully grasp Java expressions, we need to understand the fundamental role they play not only in Java but also in other programming languages. Expressions are a cornerstone of most, if not all, programming languages, and their understanding is crucial for any developer.
The Role of Expressions in Programming
Expressions are the building blocks of any program. They represent values and, when evaluated, they produce another value. This value can be a number, a string, a boolean (true or false), or even more complex data structures like objects or arrays in object-oriented languages like Java.
int a = 5;
int b = 10;
boolean result = a < b;
System.out.println(result);
// Output:
// true
In this example, a < b
is an expression that evaluates to true
. This result is then stored in the result
variable. Expressions like these form the basis of the logic in our programs.
Expressions Across Languages
While the syntax may vary, the concept of expressions is a universal one in programming. Whether you’re working in Python, JavaScript, C++, or any other language, you’ll find that expressions work in a similar way.
a = 5
b = 10
result = a < b
print(result)
# Output:
# True
In this Python example, a < b
is an expression just like in our Java example. It also evaluates to True
, demonstrating that the fundamental concept of expressions remains the same across different languages.
Understanding the role and significance of expressions in programming will help you write more efficient and effective code, regardless of the language you’re using.
Java Expressions in the Real World
Java expressions are not just confined to small programs or academic examples. They are a fundamental part of real-world applications and large-scale projects. Understanding and mastering Java expressions can significantly enhance your programming skills and your ability to write efficient, maintainable code.
Real-World Applications of Java Expressions
Java expressions are used in a variety of real-world applications. For instance, they are a key part of control flow in programs, used in conditions for if
statements and loops. They are also used in calculations, data processing, and algorithm implementation.
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
// Output:
// 0
// 1
// 2
// ...
// 9
In this code snippet, the expression i 9);
|
| Logical | Involves logical operations | boolean result = (10 > 9) && (20 > 10);
|
| Conditional | Returns a value based on a condition | int max = (a > b) ? a : b;
|
| Method Invocation | Involves calling a method | int result = multiply(10, 20);
|
| Class Instance Creation | Involves creating an instance of a class | Dog myDog = new Dog("Rover");
|
Further Resources for Mastering Java Expressions
To continue your learning journey to master Java expressions, check out these resources:
- Beginner’s Guide to Operators in Java – Understand the precedence and associativity of Java operators.
Understanding Java Operator Order – Explore the hierarchy of arithmetic, bitwise, and logical operators in Java.
Java Modulus Operator Overview – Usage of the modulus operator “%” in Java to find the remainder of a division operation.
Oracle’s Java Expression Tutorial offers in-depth information about Java expressions.
Expressions, Statements, and Blocks by Programiz dives into expressions, statements, and blocks in Java.
Java Expressions Tutorial with Examples by CodeGym is an excellent resource to understand Java expressions.
Wrapping up Expressions in Java
In this comprehensive guide, we’ve delved into the intricate world of Java expressions, examining their structure, importance, and uses. We started off by understanding the basic definition and types of expressions, moving onto understanding their evaluation and precedence. We also learned about expression statements and how they are used in conjunction with control flow statements.
By looking at the evaluation of complex expressions, we’ve dissected the importance of parentheses, the role of operator precedence, and the consequences of side effects. We discussed the use of common operators like assignment, unary and arithmetic, while also exploring their role in manipulating and evaluating expressions.
The guide also involved a practical analysis of the potential pitfalls to avoid when working with floating-point arithmetics, integer division, and operator shortcuts.
We realized that being aware of the nuances within expressions – such as automatic type conversions, potential pitfalls, and operator side effects – is crucial for avoiding bugs, designing efficient code, and enhancing code readability.
Here’s a quick comparison of the concepts in Java expressions we’ve discussed:
Expression Type | Pros | Cons |
---|---|---|
Arithmetic Expressions | Enables numerical operations | Requires care in order of operations |
Logical Expressions | Facilitates decision-making in code | Must clearly understand logical concepts |
Relational Expressions | Allows comparisons between variables | Complexity increases with more variables |
Conditional Expressions | Offers code execution based on conditions | Improper use can lead to confusing code |
Method Invocation Expressions | Enhances code reuse and modularity | Requires proper method design and usage |
Class Instance Creation Expressions | Enables object-oriented programming | Requires thorough knowledge of classes |
Lambda Expressions | Provides concise, functional programming | Can be difficult to understand for beginners |
Whether you’re just starting out with Java or looking to deepen your understanding, we hope this guide has given you a comprehensive understanding of Java expressions and their applications.
With a solid grasp of Java expressions, you’re well-equipped to write more efficient, effective, and maintainable Java code. Happy coding!