NPM Run Command | Executing Scripts in Node.js
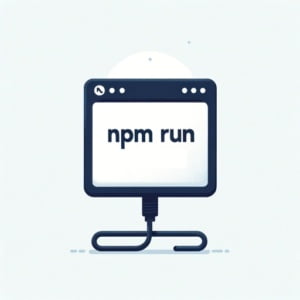
In IOFLOOD’s past, we struggled with utilizing npm run effectively for managing scripts in our projects. To address this common challenge, we’ve created a guide on how to properly use npm run
. By following our step-by-step instructions, you’ll gain a clear understanding of how the npm run command works and how to leverage it to streamline your workflow.
This guide will walk you through the npm run command, a crucial tool for executing scripts defined in your project’s package.json file. By understanding how to utilize ‘npm run’, you can streamline your development workflow, automate repetitive tasks, and ensure your project operates like a well-oiled machine.
Let’s dive into the world of npm run and unlock its full potential for managing scripts in your projects!
TL;DR: How Do I Use npm run?
You can use npm run with the syntax,
npm run [script_name]
. It requires that you follow thenpm run
command with the name of the script you want to execute from your package.json file.
Here’s a quick example:
npm run start
# Output:
# 'Your app is running on http://localhost:3000'
In this example, executing npm run start
initiates the ‘start’ script defined in your package.json, typically used to start your application. This command might, for instance, start a server that listens on a local port, displaying the message ‘Your app is running on http://localhost:3000’.
This is just the beginning of what you can accomplish with npm run scripts. Keep reading for more detailed instructions and tips on harnessing the full potential of npm run in your Node.js projects.
Table of Contents
Beginner’s Guide to npm run
Defining and Executing Simple Scripts
Entering the world of Node.js development introduces you to various tools and commands that make life easier. One such command is npm run
, an essential tool for executing scripts defined in your project’s package.json
file. Let’s dive into a basic use case of npm run
that every beginner should know: defining and executing a simple script to start a server.
First, you need to define a script in your package.json
. Suppose you want to start a server using Node.js. You might have a file named server.js
that starts your server. In your package.json
, you would define a script named start-server
like this:
"scripts": {
"start-server": "node server.js"
}
To execute this script, you would open your terminal and run:
npm run start-server
# Output:
# Server running at http://localhost:3000
By running this command, npm executes the node server.js
command specified in your start-server
script. This starts your server, typically listening on a port like 3000. The console logs Server running at http://localhost:3000
, indicating that your server is up and running. This process showcases the power of npm run
in automating tasks like starting a server, making it a staple in the development workflows of Node.js projects.
Intermediate Techniques of npm run
Passing Arguments and Utilizing Lifecycle Hooks
As you delve deeper into the capabilities of npm run
, you’ll discover its potential to handle more complex scenarios. This includes passing arguments to your scripts and leveraging npm’s built-in lifecycle hooks for more granular control over your project’s execution flow. Let’s explore these advanced uses with a practical example.
Passing Arguments to Scripts
Suppose you have a script in your package.json
that lints your code. You might want to pass additional arguments to this script, such as specifying which files to lint. Here’s how you could define such a script:
"scripts": {
"lint": "eslint"
}
To pass arguments to this script, you would use the --
syntax followed by your arguments, like so:
npm run lint -- --fix src/
# Output:
# Files in 'src/' have been linted and fixed where possible.
This command tells npm to run the lint
script, and everything after --
is passed as arguments to the eslint
command. In this case, --fix src/
instructs eslint to automatically fix fixable issues in files located in the src/
directory.
Utilizing Lifecycle Hooks
Lifecycle hooks offer a powerful way to automate tasks before or after certain npm events. For example, you might want to run tests before every push to your repository. You can achieve this by using the prepush
hook. Here’s how you could set it up:
"scripts": {
"prepush": "npm test"
}
With this configuration, npm run prepush
will execute the npm test
command, running your project’s tests before you push your code. This ensures that only passing code makes its way to your repository, enhancing your project’s reliability.
These intermediate techniques showcase npm run
‘s flexibility and power, enabling you to tailor your development workflow to your project’s specific needs. By mastering arguments passing and lifecycle hooks, you can automate and streamline your Node.js project’s tasks more effectively.
Beyond npm run
: Expert Alternatives
Exploring npm start
and npm test
When mastering Node.js and npm, understanding when and why to use alternative commands like npm start
or npm test
becomes crucial. These commands offer shortcuts for common tasks, enhancing your project’s efficiency and clarity.
The npm start
Shortcut
For many Node.js projects, starting the application is a frequent necessity. While you can define a custom script in package.json
using npm run
, npm start
provides a direct and standardized approach. Here’s an example of how npm start
can be utilized:
"scripts": {
"start": "node app.js"
}
Executing npm start
in your terminal will run the node app.js
command, similar to if you had defined a custom script and ran it with npm run start
. However, npm start
is recognized universally across Node.js projects, providing a common language for developers.
npm start
# Output:
# App running on http://localhost:3000
This command starts your application, typically opening a server on a local port. The output indicates success and where the application is accessible, streamlining the startup process for developers familiar with the npm start
convention.
Leveraging npm test
for Automated Testing
Automated testing is a cornerstone of modern development practices. npm test
simplifies the execution of your project’s test suite. By defining a test
script in your package.json
, you can run your entire test suite with a single command. Here’s an example:
"scripts": {
"test": "jest"
}
Executing npm test
initiates the test runner (in this case, Jest) and runs your project’s tests.
npm test
# Output:
# All tests passed!
The output provides immediate feedback on the status of your tests, making npm test
an invaluable tool for continuous integration environments and local development alike.
Integrating with Other Tools
npm run
scripts are not isolated; they can be part of a larger ecosystem in your development workflow. For complex projects, integrating npm scripts with other tools like Webpack for bundling, or Babel for transpiling, can automate and simplify your build process. For example, a script in package.json
might look like this:
"scripts": {
"build": "webpack"
}
This script uses Webpack to bundle your project, which can then be executed with npm run build
, showcasing the versatility and power of npm scripts in managing and automating tasks across your development lifecycle.
By understanding and utilizing these alternative approaches and integrations, you can elevate your Node.js projects, ensuring they are efficient, consistent, and aligned with industry best practices.
Solving npm run Hurdles
Tackling Script Execution Errors
When diving into the world of npm run
, you might encounter script execution errors. These can range from simple typos in your package.json
to more complex issues related to your script’s logic. Let’s address a common scenario: a script that fails due to an undefined variable in your Node.js environment.
Imagine you have a script intended to greet users based on an environment variable:
npm run greet
And in your package.json
, the script is defined as:
"scripts": {
"greet": "echo Hello, $USERNAME!"
}
If $USERNAME
is not set in your environment, the script will fail to execute as expected, leading to an error. Here’s how you might see this play out:
npm run greet
# Output:
# Hello, !
The output shows that the greeting is incomplete because $USERNAME
is not defined. To resolve this, ensure that environment variables are correctly set before running your scripts. This can be done by exporting the variable in your terminal session (export USERNAME=John
) or defining it inline with the npm command (USERNAME=John npm run greet
).
Environment Variables and Cross-Platform Compatibility
Another common issue is ensuring cross-platform compatibility, especially concerning environment variables. Windows, for example, does not handle environment variables in the same way as Unix-based systems. This discrepancy can lead to scripts that run perfectly on one system but fail on another.
To address this, consider using a package like cross-env
which allows you to set and use environment variables in a cross-platform compatible way. Here’s an example of how to modify the previous script using cross-env
:
"scripts": {
"greet": "cross-env echo Hello, $USERNAME!"
}
By incorporating cross-env
, you make your npm scripts more robust and portable across different development environments, reducing the likelihood of platform-specific errors.
Best Practices for Script Execution
- Validate Scripts Regularly: Regularly test your npm scripts in different environments to catch and address compatibility issues early.
Use Descriptive Script Names: Choose clear and descriptive names for your scripts. This not only improves readability but also aids in troubleshooting by making it easier to identify the purpose of each script.
Leverage Community Tools: Don’t reinvent the wheel. Utilize community-developed tools and packages to solve common problems, such as
cross-env
for environment variable management.
By following these guidelines and being mindful of common pitfalls, you can enhance the reliability and efficiency of your npm run scripts, making your development process smoother and more productive.
Understanding npm and package.json
The Heart of Node.js Projects
Before diving deep into the npm run
command, it’s crucial to grasp the fundamentals of npm (Node Package Manager) and the pivotal role of the package.json
file in Node.js projects. npm not only manages packages but also serves as a powerful tool for defining and running scripts which automate various tasks.
At its core, package.json
is a JSON file that contains metadata about your project. This includes information like the project’s name, version, dependencies, and, importantly, scripts that can be executed using npm. Here’s a basic example of what a package.json
file might look like:
{
"name": "my-node-project",
"version": "1.0.0",
"description": "A simple Node.js project.",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
In this example, a script named test
is defined. It’s a simple script that outputs an error message because no test has been specified. This illustrates how scripts in package.json
can be used to perform tasks. To execute this script, you would run npm run test
in your terminal.
npm run test
# Output:
# Error: no test specified
Executing the test
script outputs the predefined error message, demonstrating the script’s function. This is a simple example, but it highlights how npm run
serves as a bridge between the command line and the scripts defined in your package.json
, enabling you to automate tasks such as testing, building, and deploying your Node.js application.
The Significance of npm Scripts
npm scripts are a powerful feature for automating repetitive tasks in your development workflow. By defining scripts in package.json
, you can easily share these tasks with other developers working on the project, ensuring consistency across different environments. From starting a development server with npm run start
to running linting and tests with npm run lint
and npm run test
, scripts enhance productivity and can be integrated into continuous integration and deployment pipelines.
Understanding the structure and purpose of package.json
, along with the capabilities of npm scripts, lays the foundation for mastering npm run
and harnessing its full potential to streamline your Node.js projects.
Practical Usage Cases of npm run
Integrating npm Scripts into CI/CD
As your Node.js project grows, the importance of integrating npm run
scripts into larger development workflows, such as Continuous Integration (CI) and Continuous Deployment (CD) processes, cannot be overstated. These scripts can automate testing, linting, building, and deploying phases, significantly enhancing productivity and ensuring code quality.
Consider a scenario where you want to automate testing as part of your CI pipeline. You could have a script in your package.json
like this:
"scripts": {
"test": "mocha"
}
When integrated into a CI pipeline, the npm run test
command can be executed automatically to run your tests using Mocha. Successful execution of this script ensures that only code that passes all tests is merged into your main branch.
npm run test
# Output:
# 4 passing (2s)
This output indicates that all tests have passed, allowing the CI process to proceed to the next step. Automating this step ensures consistent quality checks and allows developers to focus on other tasks.
Enhancing Development with npm Scripts
npm run
scripts are not just for automation; they can also simplify and enhance your development process. For example, you might have a script that watches for file changes and automatically lints and tests your code, providing immediate feedback during development.
"scripts": {
"dev": "nodemon --exec 'npm run lint && npm run test'"
}
This script uses nodemon
to watch for file changes and executes both linting and testing scripts whenever a change is detected. It’s an efficient way to ensure code quality throughout the development process.
Further Resources for Mastering npm Scripts
To deepen your understanding of npm run
and its potential in Node.js projects, consider exploring these resources:
- npm Documentation: The official npm documentation provides information
npm run
and how to use npm effectively. Node.js Best Practices: A GitHub repository for Node.js development, includes npm scripts for reference.
Automating with npm Scripts: A CSS-Tricks article with examples of automating tasks with npm scripts.
By leveraging npm run
scripts and exploring these resources, you can significantly improve your development workflow, automate repetitive tasks, and ensure your Node.js projects are efficient and maintain high-quality standards.
Recap: npm run
Usage Guide
In this comprehensive guide, we’ve navigated through the versatility and power of the npm run
command in Node.js projects, from basic script execution to advanced automation techniques.
We began with the basics, understanding how to define and execute simple scripts in your package.json
. We then explored more complex script executions, such as passing arguments and utilizing npm lifecycle hooks, enhancing our command line skills for Node.js development. Venturing into expert territory, we discussed alternative npm commands and their integration with other tools, broadening our script execution strategies.
Along the way, we addressed common issues such as script execution errors and cross-platform compatibility, offering solutions and best practices to ensure smooth development experiences. We also dove into the fundamentals of npm and the pivotal role of package.json
, laying a solid foundation for mastering npm scripts.
Aspect | Importance in Node.js Development |
---|---|
Basic Script Execution | Essential for beginners to start automating tasks |
Advanced Script Execution | Enables more complex automation and workflow optimization |
Troubleshooting | Crucial for maintaining smooth development processes |
Understanding Fundamentals | Key to leveraging the full potential of npm scripts |
Whether you’re just starting out with npm run
or looking to refine your scripting prowess, we hope this guide has equipped you with the knowledge to enhance your Node.js projects. The power of npm run
lies in its ability to automate tasks, streamline development workflows, and integrate with broader development practices. By experimenting with scripts and incorporating them into your development process, you can unlock new levels of efficiency and productivity.
With the insights and examples provided, you’re now better positioned to harness the capabilities of npm run
in your Node.js projects. Remember, the journey to mastery is ongoing, and there’s always more to learn and experiment with. Happy coding!