The Long Data Type in Java: A Detailed How-To Guide
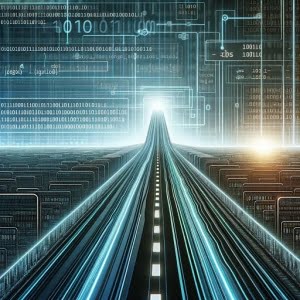
Are you finding it challenging to handle large numerical values in Java? You’re not alone. Many developers grapple with this task, but there’s a data type in Java that can make this process a breeze.
Like a marathon runner, the long data type in Java can go the distance, handling much larger numerical values than the standard int. It provides a versatile and handy tool for various tasks, especially when dealing with large data sets or high-precision calculations.
This guide will provide a comprehensive understanding of the long data type in Java, from its basic usage to more advanced techniques. We’ll explore everything from declaring and initializing long variables, performing basic operations, to more complex uses such as using it with arrays, in methods, and with the Long wrapper class. We’ll also delve into alternative approaches for handling even larger numerical values, common issues and their solutions, and best practices for optimization.
So, let’s dive in and start mastering the long data type in Java!
TL;DR: What is long in Java?
In Java, long is a data type used to store large numerical values, declared by the syntax:
long num = (yourInteger)L;
It has a width of 64 bits and can hold values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. This data type is particularly useful when you need to work with numbers that exceed the capacity of other integer data types.
Here’s a simple example of declaring a long variable in Java:
long num = 30000000000L;
System.out.println(num);
// Output:
// 30000000000
In this example, we declare a long variable num
and assign it a value of 30000000000. The ‘L’ at the end of the number is necessary to indicate that the number is a long literal. We then print the value of num
, which outputs 30000000000.
This is just a basic introduction to the long data type in Java. Continue reading for a more comprehensive guide, including its usage, common issues, and their solutions, as well as alternative approaches for handling even larger numerical values.
Table of Contents
- Declaring and Initializing Long Variables in Java
- Performing Basic Operations with Long Variables
- The Importance of the ‘L’ or ‘l’ Suffix
- Using Long with Arrays
- Using Long in Methods
- Working with the Long Wrapper Class
- Exploring BigInteger for Larger Numeric Values
- Benefits and Drawbacks of BigInteger
- Making the Right Decision
- Common Errors and Solutions with Java Long
- Best Practices and Optimization
- Fundamentals of Numeric Data Types in Java
- Applying Long Data Type in Larger Projects
- Exploring Related Topics: BigInteger and BigDecimal
- Wrapping Up: Mastering the Long Data Type in Java
Declaring and Initializing Long Variables in Java
In Java, declaring a long variable is simple. You use the keyword long
followed by the variable name. Here’s an example:
long num;
In this line of code, we’ve declared a long variable named num
. However, we haven’t assigned any value to it yet.
To initialize a long variable (i.e., to assign a value to it), you simply use the assignment operator (=
). Here’s how you can declare and initialize a long variable in one line:
long num = 15000000000L;
System.out.println(num);
// Output:
// 15000000000
In this example, we’ve declared a long variable num
and assigned it a value of 15000000000. The ‘L’ at the end of the number is necessary to indicate that the number is a long literal. We then print the value of num
, which outputs 15000000000.
Performing Basic Operations with Long Variables
You can perform basic arithmetic operations with long variables just like with any other numeric data types. Here’s an example of adding two long variables:
long num1 = 10000000000L;
long num2 = 20000000000L;
long sum = num1 + num2;
System.out.println(sum);
// Output:
// 30000000000
In this code, we’ve declared and initialized two long variables num1
and num2
. We then add these two variables and store the result in another long variable sum
. Finally, we print the value of sum
, which outputs 30000000000.
The Importance of the ‘L’ or ‘l’ Suffix
When declaring and initializing long variables in Java, it’s important to include the ‘L’ or ‘l’ suffix at the end of the number. This suffix indicates that the number is a long literal. If you omit this suffix, Java will treat the number as an integer, which could lead to errors if the number is out of the range of the int data type. Here’s an example:
long num = 15000000000; // This will cause an error
This line of code will cause a compilation error because 15000000000 is out of the range of the int data type and we didn’t include the ‘L’ suffix to indicate that it’s a long literal. To fix this error, simply add the ‘L’ suffix:
long num = 15000000000L; // This is correct
In this corrected line of code, we’ve included the ‘L’ suffix, so Java correctly treats the number as a long literal.
Using Long with Arrays
Long data type in Java can be used with arrays to store large numeric values. Here’s an example:
long[] nums = {15000000000L, 20000000000L, 25000000000L};
for (long num : nums) {
System.out.println(num);
}
// Output:
// 15000000000
// 20000000000
// 25000000000
In this code, we’ve created an array nums
of long data type and initialized it with three long literals. We then use a for-each loop to iterate over the array and print each number.
Using Long in Methods
Long data type can also be used as the parameter type and return type of methods. Here’s an example:
public static long square(long num) {
return num * num;
}
long num = 5000000L;
long result = square(num);
System.out.println(result);
// Output:
// 25000000000000
In this code, we’ve created a method square
that takes a long parameter num
and returns its square. We then call this method with a long argument and print the result.
Working with the Long Wrapper Class
Java provides a Long wrapper class that offers several useful methods for working with long data type. For example, you can use the Long.valueOf
method to convert a string to a long, and the Long.toString
method to convert a long to a string. Here’s an example:
String str = "15000000000";
long num = Long.valueOf(str);
System.out.println(num);
str = Long.toString(num);
System.out.println(str);
// Output:
// 15000000000
// 15000000000
In this code, we first convert a string str
to a long num
using the Long.valueOf
method. We then convert num
back to a string using the Long.toString
method. Both conversions are printed to the console, showing the same value of 15000000000.
Exploring BigInteger for Larger Numeric Values
While the long data type in Java is capable of handling relatively large numbers, there might be situations where even larger numbers are required. In such cases, Java provides the BigInteger class.
The BigInteger class can handle arbitrary-precision integers, which means you can use it to store integer values of any size, limited only by the memory available on your machine.
Here’s an example of how to use BigInteger:
import java.math.BigInteger;
BigInteger num = new BigInteger("9223372036854775808");
System.out.println(num);
// Output:
// 9223372036854775808
In this code, we’ve created a BigInteger object num
and initialized it with a number that’s one greater than the maximum value a long can hold. We then print the value of num
, which outputs the correct number.
Benefits and Drawbacks of BigInteger
One of the main benefits of BigInteger is its ability to handle extremely large numbers. However, this comes at a cost. BigInteger objects are immutable, which means their values cannot be changed once they’re created. Every operation on BigInteger objects creates a new object, which can lead to increased memory usage and reduced performance. In addition, operations on BigInteger objects are slower than those on primitive data types.
Therefore, you should use BigInteger only when necessary, i.e., when you need to handle numbers that cannot be represented by the long data type.
Making the Right Decision
When deciding between long and BigInteger, consider the size of the numbers you need to handle and the performance requirements of your application. If the long data type can accommodate your numbers and performance is a concern, stick with long. If you need to handle extremely large numbers and can afford the performance cost, go with BigInteger.
Common Errors and Solutions with Java Long
Working with the long data type in Java can sometimes lead to common errors. Let’s look at some of these potential issues and their solutions.
Numeric Overflow and Underflow
One common issue when working with long (and other numeric data types) is numeric overflow and underflow. This occurs when a calculation results in a value that is greater than the maximum or less than the minimum value that the data type can hold.
long max = Long.MAX_VALUE;
System.out.println(max);
max = max + 1;
System.out.println(max);
// Output:
// 9223372036854775807
// -9223372036854775808
In this example, we first print the maximum value a long can hold, which is 9223372036854775807. We then try to add 1 to this maximum value. Instead of getting an error, the value wraps around to the minimum value a long can hold, which is -9223372036854775808. This is an example of numeric overflow.
To prevent numeric overflow and underflow, you should always ensure that your calculations do not result in values outside the range of the data type.
Missing ‘L’ or ‘l’ Suffix
Another common error is forgetting to include the ‘L’ or ‘l’ suffix when initializing a long variable with a literal. This can lead to a compilation error if the literal is out of the range of the int data type.
long num = 15000000000; // This will cause a compilation error
To fix this error, simply add the ‘L’ suffix:
long num = 15000000000L; // This is correct
Best Practices and Optimization
When working with the long data type in Java, it’s important to follow some best practices for optimal performance:
- Use the long data type only when necessary, i.e., when you need to handle numbers that cannot be represented by the int data type.
- When performing calculations, ensure that the results do not exceed the range of the long data type to prevent numeric overflow and underflow.
- Always include the ‘L’ or ‘l’ suffix when initializing a long variable with a literal.
- Consider using the BigInteger class if you need to handle extremely large numbers, but be aware of the performance implications.
Fundamentals of Numeric Data Types in Java
Java provides several data types to handle numbers, each with its own range and precision. Understanding these data types is essential for effective programming in Java.
Int vs Long
The int
and long
data types are both used to store integer values, but they differ in their size and range:
int
is a 32-bit data type and can hold values from -2,147,483,648 to 2,147,483,647.long
is a 64-bit data type and can hold values from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Here’s an example of declaring an int
and a long
variable:
int num1 = 2000000000;
long num2 = 3000000000L;
System.out.println(num1);
System.out.println(num2);
// Output:
// 2000000000
// 3000000000
In this code, we declare an int
variable num1
and a long
variable num2
. We then print their values, which outputs 2000000000 and 3000000000, respectively.
Float vs Double
The float
and double
data types are used to store floating-point numbers, i.e., numbers with decimal points. They also differ in their size and precision:
float
is a 32-bit data type and can hold values from approximately ±1.4E-45 to ±3.4028235E38.double
is a 64-bit data type and can hold values from approximately ±4.9E-324 to ±1.7976931348623157E308.
Here’s an example of declaring a float
and a double
variable:
float num1 = 1.23F;
double num2 = 4.56;
System.out.println(num1);
System.out.println(num2);
// Output:
// 1.23
// 4.56
In this code, we declare a float
variable num1
and a double
variable num2
. We then print their values, which outputs 1.23 and 4.56, respectively.
Understanding Overflow and Underflow
As mentioned earlier, each numeric data type in Java has a specific range of values it can hold. If a calculation results in a value that is outside this range, it can lead to numeric overflow (if the value is too large) or underflow (if the value is too small).
For example, if you add 1 to the maximum value an int
can hold, you’ll get numeric overflow, and the result will wrap around to the minimum value an int
can hold. Similarly, if you subtract 1 from the minimum value an int
can hold, you’ll get numeric underflow, and the result will wrap around to the maximum value an int
can hold.
To prevent numeric overflow and underflow, you should always ensure that your calculations do not result in values outside the range of the data type.
Applying Long Data Type in Larger Projects
The long data type in Java is not just for small programs or simple calculations. It can also be a crucial tool in larger projects where you need to handle large data sets or perform high-precision calculations.
For example, if you’re developing a scientific simulation or a financial application, you might need to work with very large numbers that cannot be accurately represented by the int or float data types. In such cases, the long data type can be a lifesaver.
Here’s an example of how you might use the long data type to calculate the factorial of a large number:
public static long factorial(int n) {
long result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
System.out.println(factorial(20));
// Output:
// 2432902008176640000
In this code, we’ve created a method factorial
that calculates the factorial of a number. We use a long variable result
to store the factorial, which can be a very large number even for relatively small input values.
Exploring Related Topics: BigInteger and BigDecimal
If you’re interested in handling even larger numbers or need more precision than the long data type can provide, you might want to explore Java’s BigInteger and BigDecimal classes.
- BigInteger is similar to long, but it can handle arbitrary-precision integers, i.e., integers of any size. This can be useful in applications such as cryptography or number theory.
BigDecimal is used for arbitrary-precision decimal numbers. It’s especially useful in financial applications where exact calculations are necessary and floating-point errors are unacceptable.
Further Resources for Mastering Java Long
If you want to dive deeper into the long data type in Java and related topics, here are some resources you might find useful:
- Simpligying Primitive Data Types in Java – Discover how to utilize keywords to manipulate values with primitive data types.
Integer Max Value in Java – Learn about the Integer.MAX_VALUE constant in Java and the maximum value of an int.
Java Char – Understand the Java char data type for representing single characters.
Java Primitive Data Types tutorial from Oracle provides a thorough overview of the primitive data types in Java.
Java BigInteger Class from Geeks for Geeks provides a comprehensive guide to the BigInteger class in Java.
How to Use Java Long Type tutorial by Java2s provides a focused exploration into the usage of Java’s long data type.
Wrapping Up: Mastering the Long Data Type in Java
In this comprehensive guide, we’ve delved into the intricacies of the long data type in Java, a key tool for handling large numerical values.
We started with the basics, learning how to declare and initialize long variables, perform basic operations, and the importance of the ‘L’ or ‘l’ suffix. We then ventured into more advanced territory, exploring how to use long with arrays, in methods, and with the Long wrapper class.
We also tackled common challenges you might face when using long, such as numeric overflow and underflow and missing ‘L’ or ‘l’ suffix, providing you with solutions and best practices for each issue. We looked at alternative approaches for handling even larger numerical values, introducing you to the BigInteger class, its benefits, drawbacks, and decision-making considerations.
Here’s a quick comparison of the numeric data types discussed:
Data Type | Size | Range |
---|---|---|
int | 32-bit | -2,147,483,648 to 2,147,483,647 |
long | 64-bit | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
BigInteger | Arbitrary-precision | Any integer value, limited only by the memory available |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its data types, we hope this guide has given you a comprehensive overview of the long data type, its usage, common issues, and their solutions.
With its ability to handle large numerical values, long is a powerful tool in your Java programming toolbox. Happy coding!