Java’s Integer.MAX_VALUE Explained: A Complete Guide
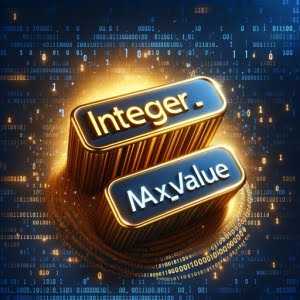
Ever found yourself wondering about the upper limit of an integer in Java? You’re not alone. Just like a car’s speedometer has a maximum limit, Java’s Integer class also has a maximum value it can represent. This limit is not arbitrary but is deeply rooted in the fundamental workings of computer systems.
In this guide, we will delve into the concept of Integer.MAX_VALUE in Java, its use cases, and how to work with it effectively. We’ll cover everything from the basics of Integer.MAX_VALUE, its implications, to more advanced techniques and alternative approaches.
So, let’s get started and master the Integer.MAX_VALUE in Java!
TL;DR: What is the Integer.MAX_VALUE() Function in Java?
In Java, The
Integer.MAX_VALUE
function returns the maximum value
that an integer can have. This value is2,147,483,647
which is the same as 2^31 – 1.
Here’s a simple example:
int max = Integer.MAX_VALUE;
System.out.println(max);
// Output:
// 2147483647
In this example, we declare an integer max
and assign it the value of Integer.MAX_VALUE
. When we print max
, it outputs 2147483647
, which is the maximum value an integer can hold in Java.
This is just the tip of the iceberg when it comes to understanding
Integer.MAX_VALUE
and how integers work in Java. Continue reading for a more detailed explanation, including why this value is the limit and how to effectively work with it.
Table of Contents
- Unveiling the Integer.MAX_VALUE Constant in Java
- Dealing with Integer Overflow in Java
- Exploring Larger Data Types in Java
- Navigating Integer Limit Issues in Java
- Behind the Scenes: Integer Limits in Computer Programming
- The Bigger Picture: Integer Limits and Large Datasets
- Wrapping Up: Integer.MAX_VALUE in Java
Unveiling the Integer.MAX_VALUE Constant in Java
In Java, Integer.MAX_VALUE
is a constant in the Integer class that holds the maximum possible value for an integer, which is 2,147,483,647. But why this specific number? The reason lies in how integers are represented in binary form in computer systems. An integer in Java is represented by 32 bits, and when all these bits are set to 1, the decimal equivalent is 2,147,483,647.
Using Integer.MAX_VALUE in Your Code
Let’s see a simple example of how to use Integer.MAX_VALUE
in your Java code:
int maxValue = Integer.MAX_VALUE;
System.out.println("The maximum integer value in Java is: " + maxValue);
// Output:
// The maximum integer value in Java is: 2147483647
In this code, we’re declaring an integer variable maxValue
and assigning it the value of Integer.MAX_VALUE
. When we print out maxValue
, it displays the maximum integer value in Java, which is 2147483647. This constant is especially useful when you’re dealing with large numbers and want to ensure that the value doesn’t exceed the limit of an integer in Java.
Dealing with Integer Overflow in Java
When you exceed the Integer.MAX_VALUE
in Java, an event known as an ‘overflow’ occurs. This is a common pitfall that can lead to unexpected results and bugs in your program. When an integer overflow happens, the value wraps around from the maximum value to the minimum value, which is -2147483648.
Integer Overflow: An Example
Let’s see an example of an integer overflow in action:
int maxValue = Integer.MAX_VALUE;
System.out.println("Max value: " + maxValue);
maxValue++;
System.out.println("Max value after increment: " + maxValue);
// Output:
// Max value: 2147483647
// Max value after increment: -2147483648
In this example, we first print out the maxValue
which is Integer.MAX_VALUE
. Then, we increment maxValue
by 1. Instead of getting a larger number, we get -2147483648 due to the overflow.
Preventing Integer Overflow
One way to prevent integer overflow is by using the Math.addExact()
method, which throws an exception when an overflow or underflow occurs.
Here’s how you can use it:
try {
int result = Math.addExact(Integer.MAX_VALUE, 1);
} catch (ArithmeticException e) {
System.out.println("Overflow occurred!");
}
// Output:
// Overflow occurred!
In this code, we are trying to add 1 to Integer.MAX_VALUE
using Math.addExact()
. Since this operation causes an overflow, an ArithmeticException
is thrown, and we print out “Overflow occurred!”.
Exploring Larger Data Types in Java
While the Integer.MAX_VALUE
limit suits most purposes, there might be scenarios where you need to work with larger numbers. In such cases, Java provides other data types such as long
and BigInteger
.
The Long Data Type
The long
data type in Java can hold much larger values than int
. The maximum value of long
is 9,223,372,036,854,775,807, which is retrieved by Long.MAX_VALUE
.
Here’s a simple usage example:
long maxLongValue = Long.MAX_VALUE;
System.out.println("The maximum long value in Java is: " + maxLongValue);
// Output:
// The maximum long value in Java is: 9223372036854775807
In this example, we declare a long
variable maxLongValue
and assign it the value of Long.MAX_VALUE
. When we print out maxLongValue
, it displays the maximum long
value in Java.
The BigInteger Class
For even larger numbers, Java provides the BigInteger
class. BigInteger
can theoretically hold any number, no matter how large, as long as you have enough memory to store it.
Here’s an example of how to use BigInteger
:
import java.math.BigInteger;
BigInteger bigValue = new BigInteger("9223372036854775808");
System.out.println("A BigInteger value in Java is: " + bigValue);
// Output:
// A BigInteger value in Java is: 9223372036854775808
In this code, we create a BigInteger
object bigValue
that holds the value 9223372036854775808
, which is larger than Long.MAX_VALUE
. When we print out bigValue
, it correctly displays the large number.
In conclusion, while Integer.MAX_VALUE
is sufficient for most tasks, Java provides long
and BigInteger
for handling larger numbers when needed.
Working with integers in Java is not always straightforward. There are several common issues that you might encounter, particularly when dealing with the integer limits, like Integer.MAX_VALUE
.
Arithmetic Overflow and Underflow
One of the most common issues is arithmetic overflow and underflow. As we’ve seen earlier, when an integer exceeds Integer.MAX_VALUE
, it wraps around to Integer.MIN_VALUE
, resulting in an overflow. Similarly, if an integer goes below Integer.MIN_VALUE
, it wraps around to Integer.MAX_VALUE
, causing an underflow.
int minValue = Integer.MIN_VALUE;
System.out.println("Min value: " + minValue);
minValue--;
System.out.println("Min value after decrement: " + minValue);
// Output:
// Min value: -2147483648
// Min value after decrement: 2147483647
In this code, we first print out the minValue
which is Integer.MIN_VALUE
. Then, we decrement minValue
by 1. Instead of getting a smaller number, we get 2147483647 due to the underflow.
Handling Overflow and Underflow
To avoid these issues, it’s important to be aware of the operations that could potentially cause an overflow or underflow. As we’ve seen earlier, the Math.addExact()
and Math.subtractExact()
methods can be used to perform addition and subtraction operations that throw an exception if an overflow or underflow occurs.
try {
int result = Math.subtractExact(Integer.MIN_VALUE, 1);
} catch (ArithmeticException e) {
System.out.println("Underflow occurred!");
}
// Output:
// Underflow occurred!
In this code, we are trying to subtract 1 from Integer.MIN_VALUE
using Math.subtractExact()
. Since this operation causes an underflow, an ArithmeticException
is thrown, and we print out “Underflow occurred!”.
These are just a few of the potential issues and solutions when working with Integer.MAX_VALUE
in Java. Being aware of these pitfalls and how to handle them will help you write more robust and reliable code.
Behind the Scenes: Integer Limits in Computer Programming
In computer programming, one of the fundamental concepts is the limit of integers. But why do these limits exist, and how are they determined?
Why Integer Limits Exist
The limits of integers exist due to the way computers store data. Computers use a binary system, which means they can only understand 0s and 1s. Each 0 or 1 is called a ‘bit’, and a group of 8 bits forms a ‘byte’.
In Java, an integer is stored in 4 bytes, or 32 bits. The first bit is used for sign (0 for positive, 1 for negative), and the remaining 31 bits are used for the number’s magnitude.
Binary Representation of Integers
In binary representation, each bit represents a power of 2. For example, the binary number 1011 represents the decimal number 8+2+1=11.
When all 31 bits are set to 1, the maximum value is reached. This is calculated as 2^31 – 1, which equals 2,147,483,647. This is why Integer.MAX_VALUE
in Java is 2,147,483,647.
int binaryRepresentation = 0b1111111111111111111111111111111; // 31 bits set to 1
System.out.println("Decimal representation: " + binaryRepresentation);
// Output:
// Decimal representation: 2147483647
In this code, we declare an integer binaryRepresentation
and assign it a binary number with 31 bits set to 1. When we print out binaryRepresentation
, it displays the decimal equivalent of the binary number, which is 2147483647.
Understanding the concept of integer limits and how binary representation works is crucial when working with large numbers in Java. It helps you make the right decisions when choosing data types and prevent potential issues like overflows and underflows.
The Bigger Picture: Integer Limits and Large Datasets
Understanding the integer limits in Java is not just an academic exercise. It has real-world implications, particularly when working with large datasets or high-precision calculations.
Working with Large Datasets
When processing large datasets, numbers can quickly exceed the Integer.MAX_VALUE
. In such scenarios, being aware of the integer limit can help you avoid potential pitfalls, like overflows, and choose the right data type for your needs.
High-Precision Calculations
Similarly, for high-precision calculations, understanding the limits of integers and how they work is crucial. It can help you make informed decisions about whether to use integers, floating-point numbers, or arbitrary-precision arithmetic for your calculations.
Further Resources for Understanding Integer Limits
To further deepen your understanding of integer limits and related concepts, here are some resources worth exploring:
- Exploring Java’s Primitive Data Types – Understand the characteristics and limitations of Java’s primitive data types.
Java Enum with Specific Values – Explore advanced enum features like constructors, methods, and fields.
Working with Longs in Java – Master using long for handling calculations involving large numbers in Java.
Oracle’s Java Documentation on Primitive Data Types is an excellent resource for understanding the basics of data types in Java.
IEEE 754-2008 Standard for Floating-Point Arithmetic provides a detailed explanation of floating-point arithmetic.
Oracle’s Java Documentation on BigInteger provides an in-depth look at the
BigInteger
class in Java.
Mastering the concept of integer limits in Java is a stepping stone to becoming a proficient Java programmer. So, keep exploring, keep learning, and keep coding!
Wrapping Up: Integer.MAX_VALUE in Java
In this comprehensive guide, we’ve journeyed through the world of Integer.MAX_VALUE
in Java, exploring its implications, use cases, and how to work with it effectively.
We began with the basics, understanding what Integer.MAX_VALUE
is, and how to retrieve this value in your Java code. We then delved into more advanced territory, discussing the implications of reaching the integer limit in Java, such as overflow, and how to handle it. We also tackled common issues that you might encounter when dealing with integer limits in Java, such as arithmetic overflow and underflow, and provided solutions to help you overcome these challenges.
Along the way, we explored alternative approaches for when you need to work with larger numbers. We introduced other data types in Java that can hold larger values, such as long
and BigInteger
, and demonstrated their usage with code examples.
Data Type | Maximum Value | Use Case |
---|---|---|
int | 2,147,483,647 | General purpose, when numbers are within the limit |
long | 9,223,372,036,854,775,807 | When numbers exceed the int limit but within the long limit |
BigInteger | No theoretical limit | When dealing with extremely large numbers |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of integers and their limits, we hope this guide has given you a deeper understanding of Integer.MAX_VALUE
and its implications.
Mastering the concept of integer limits in Java is a stepping stone to becoming a proficient Java programmer. Now, you’re well equipped to handle integers in Java, even when dealing with large numbers. Happy coding!