How to Set Variables in Bash: Shell Script Syntax Guide
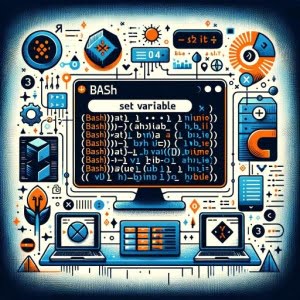
Do you find setting variables in Bash a bit tricky? You’re not alone. Many developers find Bash variable assignment a bit puzzling, but we’re here to help! You can think of Bash variables as small storage boxes – they allow us to store data temporarily for later use, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of setting variables in Bash, from the basics to more advanced techniques. We’ll cover everything from simple variable assignment, manipulating and using variables, to more complex uses such as setting environment variables or using special variable types.
So, let’s dive in and start mastering Bash variables!
TL;DR: How Do I Set a Variable in Bash?
To set a variable in Bash, you use the
'='
operator with the syntax,VAR=Value
. There must be no spaces between the variable name, the'='
operator, and the value you want to assign to the variable.
Here’s a simple example:
VAR='Hello, World!'
echo $VAR
# Output:
# 'Hello, World!'
In this example, we’ve set a variable named VAR
to the string ‘Hello, World!’. We then use the echo
command to print the value of VAR
, resulting in ‘Hello, World!’ being printed to the console.
This is just the basics of setting variables in Bash. There’s much more to learn about using variables effectively in your scripts. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Bash Variable Basics: Setting and Using Variables
- Advanced Bash Variable Usage
- Alternative Approaches to Bash Variables
- Troubleshooting Bash Variables: Common Pitfalls and Solutions
- Understanding Variables in Programming and Bash
- Expanding Bash Variable Usage in Larger Projects
- Wrapping Up: Mastering Bash Variables
Bash Variable Basics: Setting and Using Variables
In Bash, setting a variable is a straightforward process. It’s done using the ‘=’ operator with no spaces between the variable name, the ‘=’ operator, and the value you want to assign to the variable. Let’s dive into a basic example:
myVar='Bash Beginner'
echo $myVar
# Output:
# 'Bash Beginner'
In this example, we’ve created a variable named myVar
and assigned it the value ‘Bash Beginner’. The echo
command is then used to print the value of myVar
, which results in ‘Bash Beginner’ being printed to the console.
The advantages of using variables in Bash are numerous. They allow you to store and manipulate data, making your scripts more flexible and efficient. However, there are potential pitfalls to be aware of. One common mistake is adding spaces around the ‘=’ operator when assigning values to variables. In Bash, this will result in an error.
Here’s what happens when you add spaces around the ‘=’ operator:
myVar = 'Bash Beginner'
# Output:
# myVar: command not found
As you can see, Bash interprets myVar
as a command, which is not what we intended. So, remember, no spaces around the ‘=’ operator when setting variables in Bash!
Advanced Bash Variable Usage
As you progress in Bash scripting, you’ll encounter instances where you need to use more advanced techniques for setting variables. One such technique is setting environment variables, which are variables that are available system-wide and to other programs. Another is using special variable types, such as arrays.
Setting Environment Variables
Environment variables are an essential part of Bash scripting. They allow you to set values that are going to be used by other programs or processes. To create an environment variable, you can use the export
command:
export GREETING='Hello, World!'
echo $GREETING
# Output:
# 'Hello, World!'
In this example, we’ve created an environment variable called GREETING
with the value ‘Hello, World!’. The export
command makes GREETING
available to child processes.
Using Array Variables
Bash also supports array variables. An array is a variable that can hold multiple values. Here’s how you can create an array variable:
myArray=('Bash' 'Python' 'JavaScript')
echo ${myArray[1]}
# Output:
# 'Python'
In this case, we’ve created an array myArray
containing three elements. We then print the second element (arrays in Bash are zero-indexed, so index 1 corresponds to the second element) using the echo
command.
These are just a couple of examples of the more advanced ways you can use variables in Bash. As you continue to learn and experiment, you’ll find that variables are a powerful tool in your Bash scripting arsenal.
Alternative Approaches to Bash Variables
While setting variables in Bash is typically straightforward, there are alternative approaches and techniques that can offer more flexibility or functionality in certain scenarios. These can include using command substitution, arithmetic expansion, or parameter expansion.
Command Substitution
Command substitution allows you to assign the output of a command to a variable. This can be particularly useful for dynamic assignments. Here’s an example:
myDate=$(date)
echo $myDate
# Output:
# 'Mon Sep 20 21:30:10 UTC 2021'
In this example, we’ve used command substitution ($(command)
) to assign the current date to the myDate
variable.
Arithmetic Expansion
Arithmetic expansion allows the evaluation of an arithmetic expression and the substitution of the result. For instance:
num=$((5+5))
echo $num
# Output:
# '10'
Here, we’ve used arithmetic expansion ($((expression))
) to add 5 and 5 and assign the result to the num
variable.
Parameter Expansion
Parameter expansion provides advanced operations and manipulations on variables. This can include string replacement, substring extraction, and more. Here’s an example of string replacement:
greeting='Hello, World!'
newGreeting=${greeting/World/Reader}
echo $newGreeting
# Output:
# 'Hello, Reader!'
In this case, we’ve used parameter expansion (${variable/search/replace}
) to replace ‘World’ with ‘Reader’ in the greeting
variable.
These alternative approaches provide advanced ways of working with variables in Bash. They can offer more functionality and flexibility, but they also come with their own considerations. For instance, they can make scripts more complex and harder to read if not used judiciously. As always, the best approach depends on the specific task at hand.
Troubleshooting Bash Variables: Common Pitfalls and Solutions
While working with Bash variables, you might encounter some common errors or obstacles. Understanding these can help you write more robust and effective scripts.
Unassigned Variables
One common mistake is trying to use a variable that hasn’t been assigned a value. This can lead to unexpected behavior or errors. For instance:
echo $unassignedVar
# Output:
# ''
In this case, because unassignedVar
hasn’t been assigned a value, nothing is printed to the console.
Spaces Around the ‘=’ Operator
As mentioned earlier, adding spaces around the ‘=’ operator when assigning values to variables will result in an error:
myVar = 'Bash'
# Output:
# myVar: command not found
Bash interprets myVar
as a command, which is not what we intended. So, remember, no spaces around the ‘=’ operator when setting variables in Bash!
Variable Name Considerations
Variable names in Bash are case-sensitive, and can include alphanumeric characters and underscores. However, they cannot start with a number. Trying to create a variable starting with a number results in an error:
1var='Bash'
# Output:
# bash: 1var: command not found
In this case, Bash doesn’t recognize 1var
as a valid variable name and throws an error.
Best Practices and Optimization
When working with Bash variables, it’s good practice to use descriptive variable names. This makes your scripts easier to read and understand. Also, remember to unset variables that are no longer needed, especially in large scripts. This can help to optimize memory usage.
Understanding these common pitfalls and best practices can help you avoid errors and write better Bash scripts.
Understanding Variables in Programming and Bash
Variables are a fundamental concept in programming. In simple terms, a variable can be seen as a container that holds a value. This value can be a number, a string, a file path, an array, or even the output of a command.
In Bash, variables are used to store and manipulate data. The data stored in a variable can be accessed by referencing the variable’s name, preceded by a dollar sign ($).
Here is an example:
website='www.google.com'
echo $website
# Output:
# 'www.google.com'
In this example, we have a variable named website
that holds the value ‘www.google.com’. The echo
command is used to print the value of the website
variable, resulting in ‘www.google.com’ being output to the console.
Variables in Bash can be assigned any type of value, and the type of the variable is dynamically determined by the value it holds. This is different from some other languages, where the variable type has to be declared upon creation.
In addition to user-defined variables, Bash also provides a range of built-in variables, such as $HOME
, $PATH
, and $USER
, which hold useful information and can be used in your scripts.
Understanding how variables work in Bash, and in programming in general, is crucial for writing effective scripts and automating tasks.
Expanding Bash Variable Usage in Larger Projects
Setting variables in Bash is not just limited to small scripts. They play a crucial role in larger scripts or projects, helping to store and manage data effectively. The use of variables can streamline your code, making it more readable and maintainable.
Leveraging Variables in Scripting
In larger scripts, variables can be used to store user input, configuration settings, or the results of commands that need to be used multiple times. This can help to make your scripts more dynamic and efficient.
Accompanying Commands and Functions
When setting variables in Bash, you might often find yourself using certain commands or functions. These can include echo
for printing variable values, read
for getting user input into a variable, or unset
for deleting a variable. Understanding these accompanying commands and functions can help you get the most out of using variables in Bash.
read -p 'Enter your name: ' name
echo "Hello, $name!"
# Output (user input 'Alice'):
# 'Hello, Alice!'
In this example, the read
command is used to get user input and store it in the name
variable. The echo
command then uses this variable to greet the user.
Further Resources for Bash Variable Mastery
To deepen your understanding of Bash variables and their usage, you might find the following resources helpful:
- GNU Bash Manual: This is the official manual for Bash, and it provides a comprehensive overview of all its features, including variables.
Bash Scripting Guide: This is a detailed guide to Bash scripting, with plenty of examples and explanations.
Bash Variables: This tutorial offers a detailed look at Bash variables, including their declaration, assignment, and usage.
These resources offer in-depth information about Bash variables and related topics, and can provide valuable insights as you continue your Bash scripting journey.
Wrapping Up: Mastering Bash Variables
In this comprehensive guide, we’ve delved deep into the world of Bash variables. From simple variable assignment to more complex uses such as setting environment variables or using special variable types, we’ve covered it all.
We began with the basics, showing you how to set and use variables in Bash. We then explored more advanced techniques, such as setting environment variables and using array variables. Along the way, we also discussed alternative approaches, including command substitution, arithmetic expansion, and parameter expansion.
We also tackled common pitfalls and provided solutions to help you avoid these errors. Additionally, we compared the different methods of setting variables and their benefits, giving you a broader understanding of Bash variables.
Method | Pros | Cons |
---|---|---|
Simple Variable Assignment | Simple and straightforward | Limited to single values |
Environment Variables | Available system-wide | Could affect other processes |
Array Variables | Can hold multiple values | More complex to use |
Command Substitution | Dynamic assignments | Depends on command output |
Arithmetic Expansion | Allows arithmetic operations | Limited to numeric values |
Parameter Expansion | Advanced string operations | Can make scripts complex |
Whether you’re just getting started with Bash scripting or you’re looking to advance your skills, we hope this guide has given you a deeper understanding of Bash variables and how to use them effectively.
Mastering Bash variables is a key step in becoming proficient in Bash scripting. With the knowledge and techniques you’ve gained from this guide, you’re now well-equipped to use variables effectively in your scripts. Happy scripting!