Check Python Version | Guide for Windows, Mac, and Linux
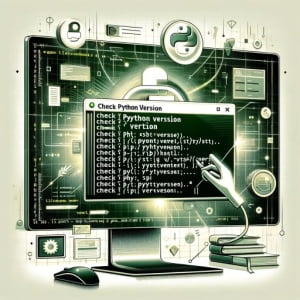
Ever found yourself unsure of which Python version you have installed? Fear not! Like a tech-savvy detective, you can reveal this critical information with a few simple commands.
This blog post is your trusty guide, leading you through the maze of checking your Python version. Whether you’re a novice coder or a seasoned programmer, we’ve got you covered with techniques ranging from basic to advanced.
So, let’s embark on this journey and demystify the process of checking your Python version, one step at a time.
TL;DR: How Do I Check My Python Version?
You can swiftly check your Python version by simply using the command
python3 --version
in your terminal or command prompt. Here’s how it’s done:
python --version
# Output:
# Python 2.7.16
python3 --version
# Output:
# Python 3.8.5
These commands will display the version of Python installed on your system. The first command python --version
is generally used for Python 2.x versions, while python3 --version
is used for Python 3.x versions.
If you’re eager to dive deeper into the world of Python versions, keep reading for more detailed explanations and advanced techniques.
Table of Contents
Unveiling Python Version: The Basics
If you’re just starting your journey in the world of Python, the first step is getting familiar with the command line. This is where you can check your Python version with a simple command. But before we get to that, let’s understand what the command line is.
The command line (also known as terminal on macOS, or command prompt on Windows) is a text-based interface where you can interact with your computer. It’s like having a conversation with your computer, but instead of talking, you type commands, and the computer responds with text output.
Now, let’s get to the heart of the matter. To check your Python version, you’ll need to open your command line and type the following command:
python --version
# Output:
# Python 2.7.16
This command asks your computer to tell you the version of Python installed. The ‘Python 2.7.16’ output means you have Python version 2.7.16 installed on your system.
But what if you’re using Python 3? In that case, you would use the following command:
python3 --version
# Output:
# Python 3.8.5
The output ‘Python 3.8.5’ signifies that Python version 3.8.5 is installed on your system.
Remember, the version number is important because different Python versions can behave differently and have different features. Knowing your Python version ensures that your Python scripts run smoothly and as expected.
Checking Python Version: The Intermediate Guide
As you progress in your Python journey, you’ll find that Python behaves slightly differently across various environments. Whether it’s a different operating system or within a Python script, knowing how to check your Python version is crucial. Let’s delve into these advanced methods.
Python Version Across Different Operating Systems
The method of checking Python version largely remains the same across different operating systems, with minor differences. For instance, on Windows, you’ll use the Command Prompt, while macOS and Linux users will use the Terminal. Here’s how you can check your Python version on these operating systems:
For Windows:
Open the Command Prompt and type:
python --version
# Output:
# Python 2.7.16
For macOS/Linux:
Open the Terminal and type:
python3 --version
# Output:
# Python 3.8.5
Python Version Within a Python Script
If you want to check the Python version from within a Python script, you can use the sys
module, which provides access to some variables used or maintained by the Python interpreter.
Here’s how you can do this:
import sys
print(sys.version)
# Output:
# 3.8.5 (default, Jan 27 2021, 15:41:15)
In this code, import sys
is used to include the Python sys
module. The print(sys.version)
line then prints the Python version in use. The output ‘3.8.5’ signifies that Python version 3.8.5 is being used.
This method is particularly useful when you’re dealing with a large project or script, and you want to ensure that you’re using the correct Python version.
Alternative Methods: Checking Python Version
As you become more proficient with Python, you may find yourself working with third-party tools or libraries. These tools often have their own ways of checking the Python version, which can be quite handy. Let’s explore some of these alternative approaches.
Using Anaconda
Anaconda is a popular Python distribution that simplifies package management and deployment. If you’re using Anaconda, you can check your Python version using the following command in the Anaconda Prompt:
conda list python
# Output:
# packages in environment at /Users/username/anaconda3:
# Name Version Build Channel
# python 3.8.5 hfd3b8b7_1
In the output, under ‘Version’, you can see ‘3.8.5’, which is the Python version being used.
Using Pyenv
Pyenv is a Python version management tool. It allows you to easily switch between multiple versions of Python. If you’re using Pyenv, you can check your Python version by typing the following command in your terminal:
pyenv version
# Output:
# 3.8.5 (set by /Users/username/.pyenv/version)
The output ‘3.8.5’ signifies that Python version 3.8.5 is being used.
Using Python’s Platform Module
Python’s built-in platform module allows you to access underlying platform’s identifying data, including the Python version. Here’s how you can use it:
import platform
print(platform.python_version())
# Output:
# 3.8.5
In this code, import platform
includes the Python platform module. The print(platform.python_version())
line then prints the Python version in use. The output ‘3.8.5’ signifies that Python version 3.8.5 is being used.
These alternative approaches provide flexibility and cater to different use cases. They can be particularly useful when dealing with specific tools or large projects.
Troubleshooting Python Version Checks
While checking your Python version is usually straightforward, you may occasionally encounter issues. Let’s discuss some common problems and their solutions.
Command Not Found Error
If you see a ‘command not found’ error after typing python --version
or python3 --version
, it likely means Python isn’t installed or the path to Python isn’t set correctly.
On Windows, you can add Python to your path during installation by checking the box that says ‘Add Python to PATH’. On macOS/Linux, you can add Python to your path by modifying the .bash_profile
or .bashrc
file.
Multiple Python Versions
You might have multiple versions of Python installed on your system. In such cases, using python --version
might not show the version you’re interested in. You can use python2 --version
and python3 --version
to check the versions of Python 2 and Python 3 respectively.
Python Version in Virtual Environments
If you’re using a virtual environment, the Python version might be different from the one installed globally on your system. You can check the Python version in the virtual environment using the same commands, but ensure that the virtual environment is activated first.
Understanding these common issues and their solutions can help you effectively navigate any hurdles you encounter when checking your Python version. Remember, the key to troubleshooting is understanding the problem, so always take a moment to read and understand any error messages you encounter.
Python Versions: Why Do They Matter?
Python, like any other programming language, evolves over time. New features are introduced, old ones are deprecated, and sometimes, there are significant changes that make a new version incompatible with the older ones. This is why Python versions are important.
The Journey of Python Versions
Python’s journey started with Python 1.0 in 1994. This version laid the foundation of Python with its emphasis on code readability and simplicity. Python 2.0, released in 2000, introduced new features like list comprehensions and garbage collection system.
Python 3.0, released in 2008, was a major upgrade that was not backwards compatible with Python 2. It addressed and rectified fundamental design flaws of the language. Since then, Python 3 has been updated regularly with new features and optimizations.
Why Knowing Your Python Version is Crucial
Different Python versions can behave differently, especially when it comes to Python 2 and Python 3. For instance, the print statement in Python 2 (e.g., print 'Hello, World!'
) is a function in Python 3 (e.g., print('Hello, World!')
).
Knowing your Python version is crucial to ensure that your code runs as expected. If you’re using a feature that’s only available in a newer version, your code won’t run on an older version. Here’s how you can check your Python version:
python --version
# Output:
# Python 2.7.16
python3 --version
# Output:
# Python 3.8.5
The output indicates the Python version installed on your system. This way, you can ensure that you’re using the correct Python version for your project.
Python Version Knowledge: A Key to Larger Projects
Knowing your Python version isn’t just about running a command and noting down the version number. It’s about understanding your development environment and ensuring your code runs smoothly, especially when you’re working on larger projects or scripts.
Python Version in Large Projects
In large projects, you might be using several external libraries. These libraries often have their own Python version requirements. For instance, some libraries might only support Python 3 and above, while others might still support Python 2. Knowing your Python version will help you choose the right libraries for your project.
Python Version in Scripts
When writing scripts, you might use features that are only available in certain Python versions. For example, the async
and await
keywords are only available in Python 3.5 and later. If you’re using these keywords in your script, you need to ensure that you’re running a compatible Python version.
Here’s how you can check your Python version within a script:
import sys
print(sys.version)
# Output:
# 3.8.5 (default, Jan 27 2021, 15:41:15)
The output ‘3.8.5’ signifies that Python version 3.8.5 is being used.
Understanding your Python version is a crucial part of Python programming. It ensures that your code runs as expected, helps you choose the right tools and libraries, and ultimately, leads to successful projects.
Further Resources for Version and Environment Management
Eager to expand your knowledge on managing versions and environments in Python? The following resources offer in-depth insights and practical tips to help you excel in these areas:
- Python Installation | Ubuntu Users’ Handbook – Learn how to manage Python versions on your Ubuntu system and keep them up to date.
Python Version Managers Made Easy – A Guide on how a Python version manager can simplify managing different Python installations.
Exploring Poetry as a Dependency Manager – Elevate your Python development by using Poetry for Python projects, including packaging and publishing.
Python Environments in Visual Studio Code – Discover how to manage Python environments in Visual Studio Code.
Pyenv Guide – Acquaint yourself with creating and managing multiple Python versions using Pyenv.
Managing Python Virtual Environments with Pyenv – A comprehensive guide on how to manage virtual environments using Pyenv.
Leveraging these resources, combined with your experiences from this guide, will set you on a strong path towards proficiency in Python version and environment management. With continued learning and application, you’ll soon become a master in managing your Python development spaces.
Unraveling Python Versions: A Recap
Throughout this guide, we’ve journeyed from the basics of checking your Python version to tackling advanced techniques. We’ve explored how to check Python versions in different environments, using various tools, and even within Python scripts. We’ve also discussed the importance of knowing your Python version, especially when working on large projects or scripts.
Here’s a quick recap of some key commands we’ve learned:
python --version
# Output:
# Python 2.7.16
python3 --version
# Output:
# Python 3.8.5
import sys
print(sys.version)
# Output:
# 3.8.5 (default, Jan 27 2021, 15:41:15)
We’ve also addressed common issues you might encounter when checking your Python version and provided solutions to navigate these hurdles.
Remember, understanding your Python version is not just about running a command—it’s about ensuring your code runs smoothly, choosing the right libraries for your project, and ultimately, building successful Python projects. Armed with the knowledge from this guide, you’re now well-equipped to check your Python version like a pro!