Find Directory Path of Bash Script | Shell Scripting How-to
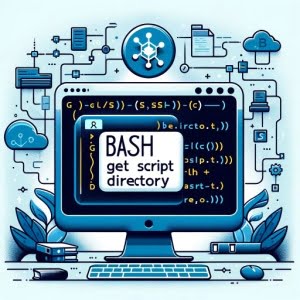
Are you finding it difficult to locate the directory of your Bash script? You’re not alone. Many developers find themselves in a maze when it comes to navigating directories in Bash, but we’re here to guide you.
Think of Bash’s built-in methods as a GPS – guiding you to the exact location of your script directory, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of getting the script directory in Bash, from the basics to more advanced techniques. We’ll cover everything from using simple commands to dealing with relative and absolute paths, and even alternative approaches.
Let’s dive in and start mastering Bash!
TL;DR: How Do I Get the Script Directory in Bash?
To get the script directory in Bash, you can use the
dirname $0
command. This command will return the path of your Bash script.
Here’s a simple example:
#!/bin/bash
# This will print the directory of the script
echo $(dirname $0)
# Output:
# /path/to/your/script
In this example, we’ve used the dirname $0
command to print the directory of the script. The dirname
command extracts the directory and $0
represents the script itself. So, dirname $0
will give you the directory of the script.
This is just the basic way to get the script directory in Bash. There’s much more to learn about navigating directories in Bash, dealing with relative and absolute paths, and even alternative approaches. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Getting Started: Basic Use of dirname $0
- Navigating Paths: Relative and Absolute
- Exploring Alternatives: PWD, pushd, and popd
- Overcoming Hurdles: Troubleshooting Common Issues
- Bash Scripting and File Handling: A Closer Look
- Bash Scripting: The Bigger Picture
- Wrapping Up: Mastering the Art of Locating Script Directory in Bash
Getting Started: Basic Use of dirname $0
The dirname $0
command is your first step to navigate directories in Bash. It’s a simple, yet powerful command that can help you locate the directory of your script.
Let’s break it down:
dirname
: This is a standard Unix command that strips the last component from the file path, effectively giving you the directory name.$0
: This is a special variable in Bash that contains the full path of the script itself.
So, when you use dirname $0
, you’re asking Bash to give you the directory name of the script you’re running.
Here’s a different example to illustrate this:
#!/bin/bash
# Store the script directory in a variable
script_dir=$(dirname $0)
echo "The script is located in: $script_dir"
# Output:
# The script is located in: /path/to/your/script
In this example, we’re storing the directory of the script in the script_dir
variable and then printing it. This way, you can use script_dir
later in your script if needed.
However, beware of a common pitfall: dirname $0
will give you a relative path if you called the script with a relative path. This means that the output will change depending on how you call the script. We’ll discuss how to handle this in the ‘Advanced Use’ section.
As you dive deeper into Bash scripting, you’ll encounter relative and absolute paths. Understanding these is crucial when working with script directories.
A relative path is defined in relation to the current directory, while an absolute path is a complete path from the root directory to the file or directory in question.
Remember the pitfall we mentioned about dirname $0
giving a relative path? Here’s where we tackle that issue. We can use the realpath
and readlink
commands to get the absolute path of the script directory.
Let’s take a look at an example using realpath
:
#!/bin/bash
# Store the absolute path of the script directory in a variable
script_dir=$(realpath $(dirname $0))
echo "The absolute path to the script is: $script_dir"
# Output:
# The absolute path to the script is: /absolute/path/to/your/script
In this example, we’ve wrapped dirname $0
inside realpath
. This gives us the absolute path to the script, no matter how we call the script.
Now, let’s explore the readlink
command:
#!/bin/bash
# Store the absolute path of the script directory in a variable
script_dir=$(readlink -f $(dirname $0))
echo "The absolute path to the script is: $script_dir"
# Output:
# The absolute path to the script is: /absolute/path/to/your/script
The -f
option with readlink
does the same job as realpath
, giving us the absolute path to the script.
Both realpath
and readlink
are handy tools to handle relative and absolute paths when getting the script directory in Bash. They help ensure the consistency of the script’s behavior, regardless of how it’s called.
Exploring Alternatives: PWD
, pushd
, and popd
While dirname $0
, realpath
, and readlink
are powerful tools, Bash offers other methods to get the script directory. Let’s explore alternatives like the PWD
variable and the pushd
and popd
commands.
The Power of PWD
The PWD
variable in Bash holds the path of the current directory. This can be used as an alternative way to get the script directory if the script is run from its own directory.
Here’s how you can use PWD
:
#!/bin/bash
# Store the current directory in a variable
script_dir=$PWD
echo "The script is located in: $script_dir"
# Output:
# The script is located in: /path/to/your/script
In this example, we’re storing the current directory in the script_dir
variable and then printing it. This approach is simple and straightforward, but it assumes that the script is run from its own directory. If the script is called from another directory, PWD
will not give you the script directory.
Navigating with pushd
and popd
The pushd
and popd
commands are other alternatives that can help you navigate directories in Bash. These commands allow you to change the current directory and return to the previous directory.
Let’s see how to use pushd
and popd
:
#!/bin/bash
# Change the current directory to the script directory
pushd $(dirname $0) > /dev/null
# Store the current directory in a variable
script_dir=$PWD
echo "The script is located in: $script_dir"
# Return to the previous directory
popd > /dev/null
# Output:
# The script is located in: /path/to/your/script
In this example, we’re using pushd
to change the current directory to the script directory, storing the current directory in the script_dir
variable, and then using popd
to return to the previous directory. This approach ensures that the script directory is captured correctly, regardless of where the script is called from.
Each of these methods has its advantages and disadvantages, and the best one to use depends on your specific use case. The PWD
variable is simple and straightforward, but it assumes that the script is run from its own directory. The pushd
and popd
commands are more versatile, but they change the current directory, which may not be desirable in all scripts. Understanding these differences will help you choose the best method for your needs.
Overcoming Hurdles: Troubleshooting Common Issues
While Bash provides several ways to get the script directory, you may encounter some common issues. These can range from dealing with symbolic links to handling spaces in directory names. Let’s discuss these problems and their solutions.
Dealing with Symbolic Links
When your script is a symbolic link (or symlink), using dirname $0
or realpath $(dirname $0)
might not give you the expected directory. Instead, they return the directory of the symlink. To get the directory of the actual script, you can use readlink
with the -f
option.
Here’s an example:
#!/bin/bash
# Get the directory of the actual script when it's a symlink
script_dir=$(dirname $(readlink -f $0))
echo "The script is located in: $script_dir"
# Output:
# The script is located in: /path/to/actual/script
In this example, readlink -f $0
gives the full path of the actual script, and dirname
extracts the directory from this path.
Handling Spaces in Directory Names
Spaces in directory names can cause issues in Bash scripts. To handle these, you can enclose the dirname $0
command in double quotes.
Here’s how you can do this:
#!/bin/bash
# Handle spaces in the script directory
script_dir="$(dirname $0)"
echo "The script is located in: $script_dir"
# Output:
# The script is located in: /path/to your/script
In this example, we’ve enclosed dirname $0
in double quotes. This ensures that the command works correctly even if the script directory includes spaces.
By understanding these common issues and their solutions, you can ensure that your Bash scripts work reliably and as expected, regardless of the specific circumstances.
Bash Scripting and File Handling: A Closer Look
To fully grasp the concept of getting the script directory in Bash, it’s essential to understand the basics of Bash scripting and file handling. Let’s explore these fundamentals.
Bash Scripting: A Quick Refresher
Bash scripting is a powerful tool for automating tasks in Unix-based systems. A Bash script is a plain text file containing a series of commands that can be executed by the Bash command-line interpreter.
Here’s a simple Bash script example:
#!/bin/bash
echo 'Hello, Bash!'
# Output:
# Hello, Bash!
This script simply prints ‘Hello, Bash!’ to the console. The first line, #!/bin/bash
, is called a shebang. It tells the system that this script should be executed with Bash.
File Handling in Bash
Bash provides several commands for file handling, such as cd
for changing directories, ls
for listing directory contents, and pwd
for printing the current directory. These commands are fundamental to navigating the file system in Bash.
Here’s an example of file handling commands in Bash:
#!/bin/bash
cd /path/to/directory
ls
pwd
# Output:
# file1 file2 file3
# /path/to/directory
In this script, we first navigate to /path/to/directory
using the cd
command. Then, we list the contents of this directory with the ls
command. Finally, we print the current directory using the pwd
command.
Understanding Paths: Relative and Absolute
In Bash, a path can be either relative or absolute. A relative path is defined in relation to the current directory, while an absolute path is a complete path from the root directory to the file or directory in question.
For example, if you’re in the directory /home/user/documents
, the relative path to the reports
directory inside documents
would be simply reports
. The absolute path to the same directory would be /home/user/documents/reports
.
Bash handles these paths differently. While a command like cd reports
would work from within the documents
directory, you would need to use the full absolute path cd /home/user/documents/reports
to navigate to the reports
directory from elsewhere in the file system.
Understanding these fundamentals of Bash scripting, file handling, and path navigation is crucial to mastering the process of getting the script directory in Bash.
Bash Scripting: The Bigger Picture
Understanding how to get the script directory in Bash is more than a simple command—it’s a fundamental concept that can significantly impact larger scripts or projects.
File Management and Modular Scripting
Consider a scenario where you have a large project with scripts in different directories. You might need to refer to other scripts or files relative to the current script. In such cases, knowing the directory of the current script becomes crucial.
Also, if you’re working with modular scripts—where a main script calls several smaller scripts—you will often need to get the script directory to correctly reference these smaller scripts. This is another situation where the techniques we’ve discussed come in handy.
Expanding Your Bash Knowledge
Getting the script directory is just one aspect of Bash scripting. There are many related concepts that you might find useful to explore, such as Bash file operations and script parameters.
- Bash file operations include commands for creating, deleting, copying, and moving files and directories. These operations form the backbone of many scripts.
Script parameters are inputs that you can pass to your script when you run it. These parameters can affect the behavior of the script, making it more flexible and reusable.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting, consider exploring these resources:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and detailed, making it a great resource for learning Bash scripting.
Bash Get Location Within Script: Baeldung offers a tutorial on how to get the location within a Bash script.
Bash Scripting Tutorial: This tutorial is a beginner-friendly introduction to Bash scripting. It covers the basics and gradually moves to more advanced topics.
Wrapping Up: Mastering the Art of Locating Script Directory in Bash
In this comprehensive guide, we’ve explored the different ways to get the script directory in Bash, a fundamental concept in Bash scripting.
We began with the basics, learning how to use the dirname $0
command to get the script directory. We then delved into more advanced territory, dealing with relative and absolute paths using realpath
and readlink
. We also explored alternative approaches such as using the PWD
variable and the pushd
and popd
commands.
Along the way, we tackled common issues that you might encounter, such as dealing with symbolic links and spaces in directory names, and provided solutions to help you overcome these challenges.
We also took a broader look at Bash scripting and file handling, understanding the fundamentals that underpin the process of getting the script directory. This background knowledge will help you understand and troubleshoot Bash scripts more effectively.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
dirname $0 | Simple and easy to use | Gives relative path if script is called with a relative path |
realpath and readlink | Provide absolute path | Require additional command |
PWD | Simple and straightforward | Only works if script is run from its own directory |
pushd and popd | Work regardless of where script is called from | Change the current directory |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to get the script directory in Bash.
Getting the script directory is a fundamental skill in Bash scripting, and mastering it will make you a more effective Bash scripter. Happy scripting!