Poetry for Python: Installation, Configuration, and Usage
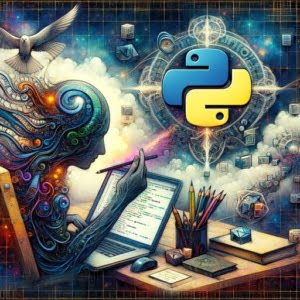
Does the thought of managing Python project dependencies make you rethink your career choices? You’re not alone. This task can indeed be challenging, especially for complex projects. However, there’s a tool that can make this process smooth and painless. Welcome to the world of Poetry.
Poetry is designed to make Python dependency management efficient and elegant. It tackles many hurdles Python developers encounter when managing project dependencies, including version control, package installation, and dependency resolution.
In this blog post, we aim to demystify Poetry. We’ll guide you through understanding, installing, and effectively using Poetry for Python dependency management. Whether you’re a seasoned Python developer or embarking on your coding journey, this guide will help you master Poetry and make dependency management a breeze.
TL;DR: What is Poetry in Python?
Poetry is a Python tool designed to manage project dependencies. It simplifies version control, package installation, and dependency resolution. It’s ideal for Python developers aiming for efficient project management. For more in-depth information, tips, and tricks, continue reading this comprehensive guide.
# To install Poetry
curl -sSL https://install.python-poetry.org | python3
# To check Poetry version
poetry --version
Table of Contents
Poetry For Dependency Management
Poetry is a versatile tool in the Python ecosystem that simplifies dependency management. But what exactly is dependency management? In the Python universe, dependency management refers to the process of managing the packages (or ‘dependencies’) on which your project relies to function correctly.
These could range from libraries to frameworks, and managing them involves tracking their versions, installing them, and ensuring they don’t conflict with each other.
Poetry offers a straightforward and concise way of specifying the packages your project needs. It also ensures that these packages are installed in the correct versions that are compatible with each other and your project. Finally, Poetry allows you to easily install multiple versions of a Python library on the same server — essential when two Python scripts demand different versions.
Essentially, Poetry eliminates the guesswork from dependency management and frees up more time for you to focus on coding.
The Anatomy of a Poetry Project
A Poetry project typically comprises two main files: pyproject.toml
and poetry.lock
. The pyproject.toml
file is where you specify your project’s dependencies. It’s a clear and human-readable file that allows you to quickly see what your project needs to run. The poetry.lock
file, on the other hand, is generated by Poetry and is used to lock the versions of your dependencies, ensuring consistent environments across different setups.
Here is an example of the content you might find in a pyproject.toml
file:
[tool.poetry]
name = "my-awesome-project"
version = "0.1.0"
description = "My awesome project!"
authors = ["Your Name <[email protected]>"]
[tool.poetry.dependencies]
python = "^3.7"
requests = "^2.23"
[tool.poetry.dev-dependencies]
pytest = "^5.4"
[build-system]
requires = ["poetry-core>=1.0.0"]
build-backend = "poetry.core.masonry.api"
In this example, requests
is a run-time dependency and pytest
is a development dependency. The ^
symbol tells Poetry to update to the latest minor or patch version.
The content of the poetry.lock
file is automatically generated by Poetry and may look something like this:
[[package]]
name = "requests"
version = "2.23.0"
description = "Python HTTP for Humans."
category = "main"
optional = false
python-versions = ">=2.7, !=3.0.*, !=3.1.*, !=3.2.*, !=3.3.*, !=3.4.*, <4"
[[package]]
name = "pytest"
version = "5.4.1"
description = "pytest: simple powerful testing with Python"
category = "dev"
optional = false
python-versions = ">=3.5"
Here, the poetry.lock
file lists the exact versions of both run-time and development dependencies that your project should use.
The
poetry.lock
file plays a pivotal role in maintaining consistent project environments. This file, which is automatically generated by Poetry when you add a package, locks the versions of your project’s dependencies. This ensures that every time your project is installed by you or someone else, the exact same versions of the dependencies are installed, thereby providing a consistent environment.
Poetry’s Unique Ability to Resolve Dependency Conflicts
One of the standout features of Poetry is its ability to automatically resolve dependency conflicts. When you add a new package to your project, Poetry checks that package’s dependencies against your project’s existing dependencies.
If it detects a version conflict, it will try to find a version of the new package that is compatible with your project. This automatic conflict resolution can save you hours of debugging and frustration, making Poetry a valuable tool in any Python developer’s toolkit.
Let’s consider an example where you have a project that requires requests v2.23.0
, and you’re trying to add another package “SamplePackage” that requires requests v2.20.0
. If you were to attempt to add “SamplePackage” to your project, you might see a bash output somewhat like this:
$ poetry add SamplePackage
Using version ^1.0 for SamplePackage
Updating dependencies
Resolving dependencies... (25.8s)
[SolverProblemError]
Because my-awesome-project depends on SamplePackage (^1.0) which depends on requests (2.20.0), requests (2.20.0) is required.
So, because my-awesome-project also depends on requests (2.23.0), version solving failed.
In this case, Poetry identified the version conflict and failed to add “SamplePackage” to your project because it couldn’t find a version of “SamplePackage” that was compatible with requests v2.23.0
.
If however, a compatible version of “SamplePackage” existed, you might see something like this:
$ poetry add SamplePackage
Using version ^1.1 for SamplePackage
Updating dependencies
Resolving dependencies... (15.4s)
Writing lock file
Package operations: 1 install, 0 updates, 0 removals
- Installing SamplePackage (1.1)
In this case, Poetry was able to find “SamplePackage v1.1” which was compatible with requests v2.23.0
, and successfully added it to your project.
Poetry Installation and Configuration
The installation process is straightforward. However, before you start, ensure that Python and pip, the Python package installer, are already installed on your system.
Once you’ve confirmed these prerequisites, you can proceed to install Poetry using the command:
curl -sSL https://install.python-poetry.org | python3
This command fetches a script from the official Poetry website and executes it using Python. Upon successful completion of the installation process, you can verify the installation by running:
poetry --version
This should display the installed version of Poetry, confirming that the installation was successful.
Setting Up Your Poetry Environment
With Poetry installed, the next step is configuration. This process involves creating the environment for your Python projects.
By default, Poetry creates a virtual environment for each project, effectively isolating its dependencies.
However, you can configure Poetry to create these environments inside your project directory by running:
poetry config virtualenvs.in-project true
Initiating a New Python Project with Poetry
To kickstart a new Python project with Poetry, navigate to the directory where you wish to house your project and execute the following command:
poetry new my-project
Replace ‘my-project’ with your preferred project name.
This command generates a new directory bearing the name of your project and establishes the basic structure for a Python project, including the
pyproject.toml
file where your project’s dependencies will be listed.
Adding, Removing, and Updating Packages
Poetry provides several customization options, allowing you to adjust the environment to your needs.
For instance, you can define the versions of Python and the packages that your project relies on. You also have the capability to add or remove packages from your project using the poetry add
and poetry remove
commands respectively.
Here’s an example of how you can add, remove, and list your dependencies in the command line by using poetry:
First, navigate to your project directory:
$ cd my-awesome-project
To add a dependency to your project, such as numpy
, use poetry add
:
$ poetry add numpy
If you want to add a package only for your development environment, such as pytest
, you can use --dev
:
$ poetry add --dev pytest
To remove a package, say numpy
, use poetry remove
:
$ poetry remove numpy
And to list all of your project’s dependencies, use poetry show
:
$ poetry show
To update a package, you utilize the poetry update
command followed by the name of the package. If you intend to update all packages, you can simply run poetry update
without specifying a package.
# To update a specific package
poetry update requests
# To update all packages
poetry update
All of these commands will automatically update your pyproject.toml
and poetry.lock
files, making dependency management a breeze.
Why Use Isolated Project Environments in Python?
One of the standout benefits of using Poetry is its emphasis on isolating project environments. By creating a distinct virtual environment for each project, Poetry ensures that the dependencies of one project do not interfere with those of another.
This isolation simplifies dependency management and prevents conflicts that can emerge when different projects depend on varying versions of the same package. This practice is crucial in Python development, and Poetry facilitates it seamlessly.
Easily Build and Publish Python Packages
Beyond managing project dependencies, Poetry also simplifies the process of building and publishing Python packages.
Here’s how you can use poetry
for packaging and publishing your project in the terminal.
Building a Package
To build a package, you use the poetry build
command. This command packages your project into a distributable format that can be installed by other users.
First, you would navigate to your project directory:
$ cd my-awesome-project
Then, to build a distributable package, you would run:
$ poetry build
This command will create two distribution package files in the dist/
directory- one .tar.gz
source archive file and one .whl
wheel (binary) distribution file.
Publishing a Package
To publish a package, you employ the poetry publish
command. This command uploads your package to PyPI, the Python Package Index, thereby making it accessible for other users to install.
But before you can use the poetry publish
command, you need to be authenticated to PyPI. You can do this with the poetry config
command:
$ poetry config pypi-token.pypi my-pypi-token
You will replace my-pypi-token
with the API token you obtained from your PyPI account.
After you’re authenticated, you can then upload your package using poetry publish
:
$ poetry publish
This command will automatically use the built distribution files in the dist/
directory.
Poetry boasts full integration with PyPI, thereby facilitating the easy publication of your Python packages. When you execute
poetry publish
, Poetry automatically packages your project, uploads it to PyPI, and updates the package version.
In each of these steps, Poetry takes care of the heavy lifting and details, ensuring your package is correctly built and uploaded to PyPI.
Poetry Troubleshooting
Despite Poetry being a powerful tool for Python dependency management, it’s not impervious to issues. However, understanding these common challenges and knowing how to troubleshoot them can significantly enhance your experience with Poetry.
Dependency Conflicts
A frequently encountered issue when using Poetry is dependency conflicts. This arises when two or more packages in your project require different versions of the same package. Poetry typically resolves these conflicts automatically.
However, in certain instances, you might need to manually intervene. This can be done by specifying a compatible version of the conflicting package in your pyproject.toml
file.
Package download failures
Another common issue is the failure of Poetry to install packages due to network problems. If you encounter this issue, verify your internet connection. If your internet connection is stable, the problem might stem from the package’s source, PyPI. In this case, patience might be required as you wait for the issue to be resolved on PyPI’s end.
Updating Poetry
Regularly updating Poetry is crucial for a seamless Python project management experience. Updates not only introduce new features but also rectify bugs and issues in the software. You can update Poetry to the latest version by executing poetry self update
.
Alternatives to Poetry for Dependency Management
While Poetry is a formidable tool for Python dependency management, it’s not the only player in the field. Other popular tools include pip, pipenv, and conda.
Each of these tools brings its own set of strengths and weaknesses to the table, and understanding them can help you select the right tool for your needs.
An Overview of pip, pipenv, and conda
pip is the default package manager for Python. It’s primarily used to install and manage Python packages from PyPI.
pipenv, on the contrary, is a tool that combines the best of all packaging worlds for Python developers. It leverages pip’s strengths for package management and incorporates features for managing virtual environments.
conda is a versatile, cross-platform package manager that simplifies the management of packages and environments for multiple languages, including Python.
How To Decide?
The choice between Poetry, pip, pipenv, and conda largely depends on your project’s requirements.
If you’re working on a simple project and don’t require advanced features like dependency resolution, pip might suffice.
For complex projects that utilize packages from languages other than Python, conda might be a more suitable choice.
pipenv serves as a good intermediary for projects that need more than pip but less than conda.
Poetry, with its balance of power and simplicity, is an excellent choice for most Python projects.
Tool | Dependency Resolution | Virtual Environment Management | Packaging and Distribution |
---|---|---|---|
Poetry | Yes | Yes | Yes |
pip | No | No | Yes |
pipenv | Yes | Yes | No |
conda | Yes | Yes | Yes |
All these tools share the same purpose—managing Python dependencies. Poetry provides a more seamless and integrated experience. pip, while powerful, lacks built-in support for virtual environments or dependency resolution. conda, although robust and versatile, might be excessive for simple Python projects.
Further Resources for Python Dependencies and Updates
Passionate about comprehending Python project management? We’ve gathered some insightful resources that can aid your learning process:
- IOFlood’s Article on Installing Python on Ubuntu can help you master the Ubuntu Python installation process and enhance your coding skills.
Ensuring Python Compatibility: How to Verify Your Python Version – Master the art of determining the Python version to troubleshoot issues and optimize code.
How to Keep Your Python Current – Python updates made simple. Learn how to ensure your development environment is up-to-date.
Python’s Official Guide to Importing – Official Python documentation detailing the import statement.
How to Change Python Versions – This resource offers practical tips to switch between different Python versions seamlessly.
Python Virtual Environment Tutorial – A concise guide by GeeksforGeeks that covers the setup and usage of Python’s virtual environments.
Through exploring these resources and applying the acquired knowledge from this guide, you’ll be well-equipped to navigate the complexities of Python dependencies and versions.
Concluding Thoughts
The task of managing dependencies in Python can appear intimidating. However, with the assistance of tools like Poetry, it transforms into an effortless activity.
Poetry streamlines Python dependency management by offering a unified interface to manage project dependencies. It adeptly resolves any dependency conflicts, ensures consistent project environments, and even simplifies the process of packaging and distributing your Python projects.
The installation and configuration of Poetry are straightforward, and its usage is intuitive. Whether you’re incorporating a new package, updating an existing one, or even publishing your package to PyPI, Poetry is equipped to handle it all.
While Poetry is a potent tool, it’s not the sole contender in the Python ecosystem. Tools like pip, pipenv, and conda also contribute significantly to Python dependency management. Each of these tools brings its own set of strengths and weaknesses to the table, and the most suitable tool for your project hinges on your specific needs and preferences.
In conclusion, developing proficiency in Python dependency management is an indispensable skill for any Python developer. With Poetry, this task not only becomes uncomplicated but also enjoyable. So, why not experiment with Poetry on your upcoming Python project? You might discover that managing Python dependencies is not a burdensome task, but a poetic endeavor.