NPM Mongoose Guide | MongoDB Toolkit for Node.js
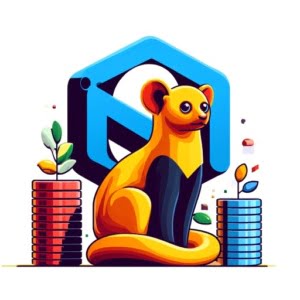
Here at IOFLOOD, we’ve frequently managed MongoDB databases in our Node.js projects. To help make this process easier, we’ve explored Mongoose, a powerful MongoDB object modeling tool. Through our experience, we have created this guide with step-by-step instructions on how to install and utilize Mongoose with npm. Our hopes are that this guide will help you utilize Mongoose for efficient data modeling and interaction with MongoDB databases.
This guide will introduce you to Mongoose for MongoDB in Node.js applications, providing a comprehensive overview of how Mongoose serves as a bridge between MongoDB and the Node.js environment. By the end of this article, you’ll have a solid understanding of how to leverage Mongoose for efficient database management, ensuring your applications run smoothly and efficiently.
Let’s dive in to database management together!
TL;DR: What is Mongoose and How Do I Use It with npm?
Mongoose is an Object Data Modeling (ODM) library that aids with MongoDB database management and interactions in Node.js. You can use it by; installing Mongoose (
npm install mongoose
), requiring it in your app, connecting to MongoDB (mongoose.connect('mongodb://localhost:27017/myDatabase')
), defining schemas (const Schema = mongoose.Schema
), and creating models for CRUD operations (const User = mongoose.model('User', userSchema)
).
Here’s a quick start example:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/my_database');
# Output:
# 'Connected to MongoDB at mongodb://localhost/my_database'
In this example, we import the Mongoose library and connect to a MongoDB database located at ‘mongodb://localhost/my_database’. This demonstrates the ease with which Mongoose can be integrated into your Node.js applications to manage your MongoDB database interactions.
Eager to dive deeper into Mongoose’s capabilities? Keep reading for a comprehensive guide on leveraging this powerful ODM library for your database management needs.
Table of Contents
Basic Installation of Mongoose
Embarking on your journey with Mongoose and MongoDB in a Node.js environment begins with a few foundational steps. Understanding these steps is crucial for setting up a robust database management system. Let’s dive into the basics of installing Mongoose, connecting to MongoDB, and defining your first schema.
Installing Mongoose with npm
To start, you’ll need to install Mongoose in your Node.js project. Mongoose acts as a bridge between MongoDB and your Node.js application, simplifying database operations. Here’s how you can install Mongoose using npm:
npm install mongoose
# Output:
# + [email protected]
# added 1 package in 2.528s
This command installs Mongoose and adds it to your project’s dependencies. The output indicates the version of Mongoose installed and the time taken for the installation.
Connecting to MongoDB
Once Mongoose is installed, you can easily connect to your MongoDB database. Here’s a simple connection example:
const mongoose = require('mongoose');
mongoose.connect('mongodb://yourMongoDBUri', {useNewUrlParser: true, useUnifiedTopology: true});
mongoose.connection.once('open', function() {
console.log('Connected to the database.');
});
# Output:
# 'Connected to the database.'
In this example, we’re connecting to MongoDB using a connection string. The useNewUrlParser
and useUnifiedTopology
options are set to ensure compatibility and use of the new MongoDB driver’s features.
Defining a Simple Schema
With Mongoose, defining a schema for your data is straightforward. A schema represents the structure of your data in MongoDB. Here’s how to define a simple schema:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const UserSchema = new Schema({
name: String,
age: Number
});
const User = mongoose.model('User', UserSchema);
# Output:
# Schema definition successful.
This code snippet creates a User
schema with name
and age
fields. Schemas are essential for ensuring data consistency and validation in your application.
Creating and Saving a Model
After defining your schema, the next step is to create and save a model to the database. A model represents a document in the MongoDB database that can be manipulated using CRUD operations. Here’s an example of creating and saving a user model:
const newUser = new User({
name: 'John Doe',
age: 30
});
newUser.save(function(err) {
if (err) return console.error(err);
console.log('User saved successfully.');
});
# Output:
# 'User saved successfully.'
This example demonstrates creating a new user instance and saving it to the database. Successfully saving the model indicates that you’ve effectively integrated Mongoose with your MongoDB database, laying the foundation for more complex operations.
By mastering these basic steps, you’re well on your way to leveraging Mongoose for efficient database management in your Node.js applications. These initial steps form the bedrock of more advanced Mongoose functionalities and database operations you’ll encounter as you progress.
Advanced Usage of Mongoose
As you become more comfortable with Mongoose basics, it’s time to dive into its more advanced features. These capabilities allow you to handle complex queries, enhance data validation, and manage relationships between documents more effectively. Let’s explore advanced schema types, virtuals, and middleware, and see how they can add sophistication to your MongoDB operations in Node.js.
Advanced Schema Types
Mongoose offers a variety of schema types that go beyond the basics, enabling more nuanced data representation. For instance, you can define arrays, nested objects, and even set custom types. Here’s an example of using an advanced schema type:
const mongoose = require('mongoose');
const { Schema } = mongoose;
const BlogPostSchema = new Schema({
title: String,
author: String,
body: String,
comments: [{ body: String, date: Date }],
date: { type: Date, default: Date.now },
hidden: Boolean
});
# Output:
# 'Advanced schema defined successfully.'
This schema includes an array of comments, each with its own structure, and utilizes the default
option to automatically set the date. This level of detail in schema design allows for more complex data structures and default values, enhancing data integrity and usability.
Virtuals for Dynamic Properties
Virtuals are a powerful feature in Mongoose that allow you to define virtual properties not stored in MongoDB. They can be used for computed properties on documents. For example, to add a full name property to a user model:
const UserSchema = new Schema({
firstName: String,
lastName: String
});
UserSchema.virtual('fullName').get(function () {
return `${this.firstName} ${this.lastName}`;
});
# Output:
# 'Virtual fullName defined successfully.'
This virtual fullName
property dynamically generates a user’s full name from their first and last names. It’s a powerful way to add functionality to your models without modifying the underlying data structure.
Middleware for Pre and Post Processing
Middleware (also known as pre and post hooks) are functions that are passed control during execution of asynchronous functions. Middleware allows you to perform actions before or after certain events. An example is adding a pre-save hook to hash a user’s password before saving it to the database:
const bcrypt = require('bcrypt');
UserSchema.pre('save', async function(next) {
const user = this;
if (user.isModified('password')) {
user.password = await bcrypt.hash(user.password, 8);
}
next();
});
# Output:
# 'Pre-save middleware added successfully.'
In this example, the middleware hashes the password before saving the user document, adding a layer of security to your application. Middleware are invaluable for ensuring data integrity and implementing complex validation and processing logic.
By leveraging these advanced features, you can significantly enhance the functionality and efficiency of your Node.js applications using Mongoose. These tools offer powerful ways to model, validate, and process your data, setting a strong foundation for building sophisticated applications.
Comparing Other ODM Libraries
When it comes to managing MongoDB databases in Node.js applications, Mongoose is a popular choice, but it’s not the only option. Understanding when to use Mongoose versus other Object Data Modeling (ODM) libraries or the native MongoDB driver can significantly impact the performance and scalability of your applications.
Mongoose and Other ODMs
Other ODM libraries, such as Typegoose
and Mongorito
, offer different approaches and features. For instance, Typegoose leverages TypeScript decorators to define schemas and models, providing a more type-safe way of working with MongoDB. Here’s a brief comparison:
// Typegoose example
import { getModelForClass, prop } from '@typegoose/typegoose';
class User {
@prop()
public name?: string;
}
const UserModel = getModelForClass(User);
# Output:
# 'UserModel ready for Type-Safe operations.'
This example demonstrates how Typegoose allows for defining a schema using TypeScript classes and decorators, offering a type-safe approach to MongoDB interactions. The expected output indicates that the UserModel
is ready for operations, highlighting the ease of integrating TypeScript into your MongoDB projects.
Mongoose vs. Native MongoDB Driver
While Mongoose provides a high-level abstraction over MongoDB with features like schemas, validation, and middleware, the native MongoDB driver offers more direct access to MongoDB’s capabilities. The choice between Mongoose and the native driver depends on your project’s needs. For projects requiring fine-tuned performance optimizations or direct use of MongoDB’s latest features, the native driver might be the better choice.
Integrating Mongoose in Large-Scale Applications
Mongoose excels in managing data relationships, schema validations, and offering a simplified API for CRUD operations, making it suitable for large-scale applications. However, performance considerations such as index management, query optimization, and connection pooling should be carefully managed. Here’s an example of optimizing a Mongoose query:
const query = User.find({ age: { $gt: 18 } }).limit(10).sort({ name: 1 });
query.exec((err, users) => {
if (err) throw err;
console.log(users);
});
# Output:
# 'Array of users older than 18, limited to 10, sorted by name.'
This query example demonstrates how to retrieve a specific subset of users, showcasing Mongoose’s ability to handle complex queries efficiently. Properly indexing your MongoDB collections and optimizing your queries are crucial for maintaining performance in large-scale applications.
Mongoose with TypeScript
Mongoose can be used with TypeScript to add static types to your MongoDB models, enhancing code quality and maintainability. While Mongoose does not natively support TypeScript, you can use TypeScript interfaces to define schemas and models. This integration combines Mongoose’s powerful features with TypeScript’s type safety, offering a robust solution for modern web development.
By understanding the strengths and limitations of Mongoose in comparison to other ODM libraries and the native MongoDB driver, you can make informed decisions on the best approach for your Node.js applications. Whether you’re working on a small project or a large-scale application, selecting the right tools is key to achieving optimal performance and scalability.
Mongoose: Pitfalls and Performance
Even with a powerful tool like Mongoose for your MongoDB and Node.js applications, there are common pitfalls that can hinder your project’s success. Understanding these pitfalls and how to avoid them is crucial. This section will delve into connection issues, schema design mistakes, handling asynchronous operations, and provide tips for optimizing Mongoose’s performance along with security best practices.
Avoiding Connection Issues
One of the first hurdles you might encounter is connection issues to your MongoDB database. Ensuring a reliable connection is foundational to your application’s success. Here’s an example of handling connection errors gracefully:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/myapp', { useNewUrlParser: true, useUnifiedTopology: true })
.catch(err => {
console.error('Database connection error:', err);
});
# Output:
# 'Database connection error: [Error details]'
In this code snippet, we attempt to connect to a MongoDB database and catch any potential errors, logging them to the console. This approach ensures that your application can respond to connection issues appropriately, rather than failing silently.
Schema Design Considerations
A common mistake when using Mongoose involves schema design. Poorly designed schemas can lead to inefficient data access and bloated documents. It’s essential to plan your schemas thoughtfully, considering data normalization and document size. Utilize sub-documents and references judiciously to balance between query performance and document structure.
Handling Asynchronous Operations
Mongoose operations are asynchronous, making error handling and flow control critical. Improper handling can lead to uncaught exceptions and unpredictable application behavior. Here’s a pattern for managing asynchronous operations with async/await:
async function createUser(username) {
try {
const user = new User({ username });
await user.save();
console.log('User created successfully');
} catch (error) {
console.error('Error creating user:', error);
}
}
# Output:
# 'User created successfully'
This example demonstrates creating a new user and handling any errors that may occur. Using async/await simplifies working with asynchronous code, making it more readable and easier to debug.
Optimizing Performance
Performance optimization in Mongoose is vital, especially as your application scales. Indexing is a powerful tool for improving query performance. Ensure your schemas are properly indexed to speed up data retrieval. Additionally, be mindful of the lean()
method for queries that don’t require Mongoose documents, as it can significantly reduce overhead.
Security Best Practices
Security should never be an afterthought. With Mongoose, ensure that you validate and sanitize your data to protect against injection attacks. Use the built-in validation features or integrate libraries like express-validator
for input validation. Also, consider implementing access controls at the schema level to safeguard sensitive information.
By being aware of these considerations and troubleshooting tips, you can enhance the reliability, performance, and security of your Mongoose-based applications. Each of these aspects plays a crucial role in creating robust and efficient Node.js applications with MongoDB.
MongoDB and ODM Essentials
MongoDB, a leading NoSQL database, excels in handling vast amounts of data and complex structures. Its flexibility and scalability make it a top choice for modern web development. However, directly interacting with MongoDB can be challenging due to its schema-less nature. This is where Object Data Modeling (ODM) libraries, like Mongoose, come into play, offering a structured approach to MongoDB database interactions.
Why Mongoose Matters
Mongoose acts as a middleman between MongoDB and your Node.js application. It provides a schema-based solution to model your application data. This schema not only defines the structure and the rules your data will follow but also provides instance methods to interact with that data. Consider the following example where we define a simple user schema and use it to create a new user:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const userSchema = new Schema({
name: String,
email: { type: String, required: true, unique: true }
});
const User = mongoose.model('User', userSchema);
const newUser = new User({
name: 'Jane Doe',
email: '[email protected]'
});
newUser.save().then(doc => console.log(doc)).catch(err => console.error(err));
# Output:
# { _id: 5f47a0ac6d0e810b3a49a6d4, name: 'Jane Doe', email: '[email protected]', __v: 0 }
In this example, we define a User
schema with name
and email
fields, where email
is required and must be unique. Upon creating a new user with this schema and saving it to the database, Mongoose handles the data validation based on the schema rules. The output shows the saved document, including a generated _id
and the version key (__v
). This demonstrates how Mongoose simplifies creating and managing data, ensuring data integrity and reducing development time.
The Role of Mongoose in Web Development
Mongoose enhances Node.js applications by providing a structured way to interact with MongoDB. It supports features like data validation, query building, and business logic hooks (middleware), which are crucial for robust application development. By abstracting the complexities of direct database interactions, Mongoose allows developers to focus more on application logic rather than the nuances of database operations.
The integration of Mongoose in your Node.js projects paves the way for efficient database management, streamlined development processes, and ultimately, more scalable and maintainable applications. Understanding these fundamentals is key to leveraging Mongoose effectively in your projects, making your journey with MongoDB and Node.js a rewarding one.
Expanding Your Mongoose Ecosystem
As you grow more comfortable with Mongoose and its role in your Node.js applications, exploring the broader ecosystem can unlock even more potential. This ecosystem includes a variety of plugins, tools, and resources designed to enhance your MongoDB experience. Let’s explore some of these options and how they can contribute to more efficient, powerful applications.
Mongoose Plugins and Tools
The Mongoose community has developed numerous plugins that extend the functionality of Mongoose, from improving schema validations to adding new types of fields. For instance, mongoose-autopopulate
is a plugin that automatically populates your queries with referenced documents, simplifying your code and reducing boilerplate.
const mongoose = require('mongoose');
const autopopulate = require('mongoose-autopopulate');
const commentSchema = new Schema({
text: String,
postedBy: { type: Schema.Types.ObjectId, ref: 'User', autopopulate: true }
});
commentSchema.plugin(autopopulate);
const Comment = mongoose.model('Comment', commentSchema);
# Output:
# 'Comment model with autopopulate enabled.'
In this example, the autopopulate
plugin is applied to the commentSchema
, ensuring that any query on the Comment
model will automatically include the detailed information of the postedBy
user. This demonstrates how plugins can significantly streamline operations and enhance the functionality of your Mongoose models.
Monitoring and Managing MongoDB
For those looking to monitor and manage their MongoDB databases more effectively, tools like MongoDB Atlas and Compass provide comprehensive solutions. MongoDB Atlas offers a cloud-based database service with built-in monitoring and scaling options, while MongoDB Compass is a powerful GUI for managing your databases, allowing you to visualize and manipulate your data with ease.
Further Resources for Mastering Mongoose
To continue your journey with Mongoose and MongoDB, here are three recommended resources:
- Mongoose Official Documentation – An authoritative resource for learning Mongoose thoroughly.
MongoDB University – Offers free structured courses on MongoDB, including using Mongoose in your Node.js applications.
Mongoose Playlist on YouTube – Comprehensive Mongoose video series with practical, hands-on examples.
By leveraging these resources and tools, you can enhance your skills and understanding of Mongoose, MongoDB, and Node.js, paving the way for building more sophisticated and efficient applications.
Recap: Mongoose for MongoDB Guide
In this comprehensive guide, we’ve explored the power of Mongoose, an essential npm package that simplifies the interaction between MongoDB and Node.js applications. From establishing a connection to MongoDB, defining schemas, to performing CRUD operations, Mongoose serves as a robust toolkit for managing your database more efficiently.
We began with the basics of installing Mongoose and connecting to MongoDB, laying the foundation for any Node.js application that interacts with this popular NoSQL database. We delved into defining schemas and models, which are crucial for ensuring data integrity and structure within your applications.
Following that, we ventured into more advanced territories, such as utilizing advanced schema types, implementing virtuals for dynamic properties, and leveraging middleware for pre and post processing. These features allow for a more sophisticated manipulation of data, paving the way for complex applications.
We also discussed alternative approaches for those at an expert level, comparing Mongoose with other ODM libraries and the native MongoDB driver. This insight helps in making informed decisions based on the specific needs of your project, whether it’s performance optimization or leveraging TypeScript for type safety.
Aspect | Basic Use | Advanced Use | Expert Level Considerations |
---|---|---|---|
Schema Definition | Simple and intuitive | Supports advanced types and virtuals | Comparison with other ODMs, TypeScript integration |
Data Manipulation | CRUD operations | Complex queries, middleware | Performance optimization, large-scale application integration |
Learning Curve | Low | Moderate | High |
As we wrap up, it’s clear that Mongoose significantly enhances the development experience by providing a structured and intuitive approach to managing MongoDB databases in Node.js applications. Whether you’re just starting out or looking to deepen your understanding of database management, Mongoose offers the tools and flexibility needed to build efficient, scalable applications.
With the knowledge gained from this guide, you’re now better equipped to leverage Mongoose for effective database management. The journey from mastering the basics to exploring advanced features and considering expert-level strategies underscores the versatility and power of Mongoose in the modern web development landscape. Happy coding!