Bash ‘Declare’ Command Guide for Variable Assignment
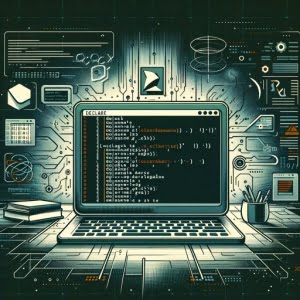
Are you finding it challenging to master the use of bash declare? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled craftsman, bash declare helps you shape your variables with precision. These variables can be used in any bash script, making bash declare an essential tool for any bash programmer.
This guide will walk you through the use of bash declare, from basic syntax to advanced techniques. We’ll explore bash declare’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering bash declare!
TL;DR: How Do I Use Bash Declare?
The bash
declare
command is used to declare variable types or assign them attributes, with the syntax,declare -[option] variableName=value
. It’s a powerful tool that can help you manage your bash scripting more effectively.
Here’s a simple example:
declare -i num=10
echo $num
# Output:
# 10
In this example, we use the bash declare command to declare an integer variable num
and assign it a value of 10. The -i
option tells bash that num
is an integer. When we echo num
, it outputs the value 10.
This is just a basic usage of bash declare, but there’s much more to it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Grasping the Basics of Bash Declare
- Diving Deeper: Advanced Usage of Bash Declare
- Exploring Alternatives: The ‘typeset’ Command
- Troubleshooting Common Errors with Bash Declare
- The Foundation: Bash Scripting and Variables
- Bash Declare in Real-World Applications
- Wrapping Up: Mastering Bash Declare for Efficient Scripting
Grasping the Basics of Bash Declare
Bash declare is a built-in command in the bash shell that allows you to declare variable types or assign attributes to variables. It’s a fundamental tool for any bash programmer, and understanding its basic syntax and functionality is crucial.
Let’s take a look at a simple example of how to use bash declare:
declare -r var="Hello, World!"
echo $var
# Output:
# Hello, World!
In this example, we use bash declare to declare a read-only variable var
and assign it a string value ‘Hello, World!’. The -r
option tells bash that var
is read-only, meaning it can’t be modified or unset once it’s been set. When we echo var
, it outputs the string ‘Hello, World!’.
Understanding the basic usage of bash declare is the first step towards mastering more complex bash scripting. It gives you control over your variables, allowing you to define their behavior and prevent unwanted changes. However, it’s important to note that declaring a variable as read-only can lead to errors if you later attempt to modify it. Therefore, it’s crucial to use this feature carefully.
Diving Deeper: Advanced Usage of Bash Declare
As you become more comfortable with bash declare, you can start exploring its more advanced features. Bash declare isn’t limited to defining read-only variables; it can also be used to declare integer variables, array variables, and more.
Declaring Integer Variables
One such advanced usage is declaring integer variables. This can be useful when you need to perform arithmetic operations in your bash script. Let’s take a look at an example:
declare -i num
default=5
num=$default*10
echo $num
# Output:
# 50
In this code block, we first declare an integer variable num
. We then assign a default value of 5 to num
and multiply it by 10. When we echo num
, it outputs the result of the multiplication: 50. This demonstrates how bash declare can be used to perform arithmetic operations directly within the assignment.
Declaring Read-Only Variables
Another advanced use of bash declare is declaring read-only variables. This can be beneficial when you want to prevent a variable from being modified after its initial assignment. Here’s an example:
declare -r var="Hello, World!"
var="Goodbye, World!"
echo $var
# Output:
# bash: var: readonly variable
In this code block, we declare a read-only variable var
and attempt to change its value. However, bash prevents us from doing so, and instead outputs an error message. This highlights the power of bash declare in preserving the integrity of your variables.
Keep in mind, while these advanced features of bash declare can be quite useful, they should be used judiciously. Declaring a variable as an integer or read-only can restrict its functionality, so it’s important to consider the implications before using these options.
Exploring Alternatives: The ‘typeset’ Command
While bash declare
is an incredibly versatile tool, it’s not the only command available for managing variable types and attributes in bash scripting. Another command that offers similar functionality is typeset
.
The ‘typeset’ Command and Its Usage
The typeset
command, like bash declare
, allows you to declare variable types or assign attributes. In fact, in many cases, typeset
can be used as a substitute for bash declare
. Let’s look at an example:
typeset -i num=20
echo $num
# Output:
# 20
In this code block, we use typeset
to declare an integer variable num
and assign it a value of 20. When we echo num
, it outputs the value 20. This is similar to how we used bash declare
to declare and assign an integer variable in the earlier examples.
Weighing the Pros and Cons
While typeset
can be used as an alternative to bash declare
, it’s worth noting that typeset
is older and less commonly used in modern bash scripting. However, it remains a viable option, especially for scripts that need to be compatible with older versions of bash.
On the other hand, bash declare
is more widely recognized and used in modern bash scripting. It’s also more intuitive for those who are new to bash scripting, as its name clearly indicates its purpose.
In conclusion, while typeset
is a viable alternative to bash declare
, it’s generally recommended to use bash declare
unless you have a specific reason to use typeset
. Understanding both commands, however, can give you more flexibility and control in your bash scripting.
Troubleshooting Common Errors with Bash Declare
While bash declare is a powerful tool, it’s not without its quirks and potential pitfalls. Here, we’ll explore some common errors you might encounter when using bash declare, and provide solutions to overcome them.
Error: Modifying a Read-Only Variable
One common error occurs when you try to modify a read-only variable. Let’s look at an example:
declare -r var="Hello, World!"
var="Goodbye, World!"
echo $var
# Output:
# bash: var: readonly variable
In this code block, we declare a read-only variable var
and then attempt to change its value. However, bash prevents us from doing so, outputting an error message. The solution here is simple: avoid modifying read-only variables once they’ve been declared.
Error: Incorrect Usage of Integer Variables
Another common mistake is incorrectly using integer variables. For example:
declare -i num="Hello"
echo $num
# Output:
# 0
In this case, we’ve declared num
as an integer variable but attempted to assign a string value to it. Bash defaults the value to 0, as it expects an integer. To avoid this, ensure you assign appropriate values to your variables based on their declared type.
Best Practices and Optimization
To avoid common errors and optimize your use of bash declare, here are a few tips:
- Always assign appropriate values to your variables based on their declared type.
- Avoid modifying read-only variables once they’ve been declared.
- Use meaningful variable names to make your code more readable and maintainable.
By keeping these considerations in mind, you can avoid common pitfalls and make the most of bash declare in your scripting.
The Foundation: Bash Scripting and Variables
To fully understand the power of bash declare, it’s essential to grasp the fundamentals of bash scripting and variables.
Bash scripting is a form of programming that uses the bash shell, a command-line interface for Unix-based systems. It allows you to automate tasks, manipulate files, and perform complex operations.
At the heart of bash scripting are variables. Variables in bash are like containers that hold information. This information can be a number, a string, or even the result of a command. Variables are declared and assigned values using the equals (=) sign, like so:
var="Hello, World!"
echo $var
# Output:
# Hello, World!
In this example, we declare a variable var
and assign it a string value ‘Hello, World!’. When we echo var
, it outputs the string ‘Hello, World!’.
The Importance of Variable Types and Attributes
In bash scripting, variables are untyped by default, meaning they can hold any type of data. However, sometimes it’s useful to specify a variable’s type or assign it certain attributes. This is where the bash declare command comes into play.
By using bash declare, you can define a variable’s type (such as integer or read-only), which can help prevent errors and make your code more robust. For example, declaring a variable as an integer ensures that it can only hold integer values, which can be useful when performing arithmetic operations.
In addition, bash declare allows you to assign attributes to variables, such as making them read-only or assigning them a default value. These attributes can help control how your variables behave and prevent unwanted modifications.
In conclusion, understanding the fundamentals of bash scripting and the importance of variable types and attributes is key to mastering bash declare. With this knowledge, you can write more effective and error-free bash scripts.
Bash Declare in Real-World Applications
Bash declare is not just a standalone command; it’s a vital part of larger scripts and projects. It can be used in conjunction with other commands and functions to create more complex and powerful bash scripts.
Applying Bash Declare in Larger Scripts
In larger scripts, bash declare can be used to manage variables more effectively. For instance, you might use bash declare to set default values for variables, declare them as integers for arithmetic operations, or even make them read-only to prevent unwanted modifications.
Complementary Commands and Functions
Bash declare often works hand in hand with other commands and functions. For example, the echo
command is frequently used with bash declare to output the value of a variable. Similarly, the unset
command can be used to unset a variable that has been declared but is no longer needed.
Further Resources for Mastering Bash Declare
If you’re looking to delve deeper into bash declare and related topics, here are a few resources that might help:
- Advanced Bash-Scripting Guide: This guide offers in-depth information on bash scripting, including the use of bash declare.
- GNU Bash Reference Manual: This is the official reference manual for bash, featuring a comprehensive section on bash declare.
- Bash Guide for Beginners: This guide is perfect for beginners looking to understand the basics of bash scripting, including bash declare.
With these resources and the information provided in this guide, you’re well on your way to mastering bash declare and its applications in bash scripting.
Wrapping Up: Mastering Bash Declare for Efficient Scripting
In this comprehensive guide, we’ve navigated the intricacies of bash declare, a powerful command in bash scripting for managing variable types and attributes.
We began with the basics, learning how to use bash declare for simple tasks like declaring variables and assigning them values. We then delved into more advanced usage, exploring how to declare integer variables, read-only variables, and more. Along the way, we provided practical code examples to illustrate each concept and its application.
We also tackled common challenges you might encounter when using bash declare, such as modifying a read-only variable or incorrectly using integer variables, providing solutions for each issue. Additionally, we explored the ‘typeset’ command as an alternative to bash declare, giving you a broader view of the tools available for variable management in bash scripting.
Here’s a quick comparison of bash declare and typeset:
Command | Versatility | Modern Usage | Compatibility |
---|---|---|---|
bash declare | High | Widely used | High |
typeset | Moderate | Less common | High with older bash versions |
Whether you’re just starting out with bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of bash declare and its capabilities.
With its ability to manage variable types and attributes, bash declare is a vital tool for efficient and robust bash scripting. Now, you’re well equipped to harness its power in your scripts. Happy coding!