How to Delete Files in Bash: Your Guide to the Bash Shell
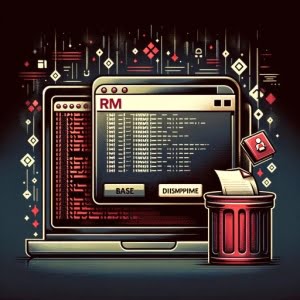
Are you finding it challenging to delete files using bash? You’re not alone. Many users find themselves puzzled when it comes to handling file deletion in bash, but we’re here to help.
Think of bash as a powerful file manager – allowing us to keep our system clean and organized. With bash, you can manage your files effectively, including deleting them when they are no longer needed.
In this guide, we’ll walk you through the process of deleting files using bash, from the basics to more advanced techniques. We’ll cover everything from the simple rm
command to more complex scenarios, as well as alternative approaches.
Let’s get started and master file deletion in bash!
TL;DR: How Do I Delete a File with Bash?
To delete a file in bash, you use the
rm
command followed by the file name, such asrm myfile.txt
.
Here’s a simple example:
rm myfile.txt
# Output:
# (No output, but the file 'myfile.txt' is deleted)
In this example, we’ve used the rm
command to delete a file named ‘myfile.txt’. The rm
command is followed by the name of the file you want to delete. After running this command, the file ‘myfile.txt’ is deleted from the current directory.
But there’s more to deleting files in bash than just the
rm
command. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the rm Command
- Deleting Multiple Files and Directories with Bash
- Exploring Alternatives: The unlink Command and Third-Party Tools
- Common Issues and Solutions in Bash File Deletion
- Understanding Bash and Its File Management Capabilities
- The Bigger Picture: File Deletion in System Administration and Script Writing
- Wrapping Up: Mastering File Deletion in Bash
Understanding the rm
Command
The rm
command is the most basic and commonly used command when it comes to deleting files in bash. The term rm
stands for ‘remove’. As simple as it sounds, it’s a powerful tool in bash, and understanding how it works is the first step in mastering file deletion.
Let’s look at a simple usage of the rm
command:
rm report.txt
# Output:
# (No output, but the file 'report.txt' is deleted)
In this example, the rm
command is followed by the name of the file we want to delete, ‘report.txt’. After running this command, the file ‘report.txt’ is deleted from the current directory.
Advantages and Potential Pitfalls of rm
The rm
command is straightforward and easy to use, making it a go-to for most bash users. It’s efficient and does the job well. However, it’s important to be cautious when using rm
. The command is irreversible, meaning once a file is deleted, it cannot be recovered. Always double-check the filename and the command before hitting enter.
In the next section, we’ll discuss more advanced uses of the rm
command and how to handle multiple files and directories.
Deleting Multiple Files and Directories with Bash
As you become more comfortable with the rm
command, you’ll find that it can do more than just delete single files. It can also be used to delete multiple files, directories, and even use wildcards for broader file deletion.
Deleting Multiple Files
To delete multiple files, you simply list them after the rm
command, separated by spaces:
rm report.txt notes.docx image.jpg
# Output:
# (No output, but the files 'report.txt', 'notes.docx', and 'image.jpg' are deleted)
In this example, we’ve deleted three files: ‘report.txt’, ‘notes.docx’, and ‘image.jpg’. This can be a real time-saver when you need to delete multiple files at once.
Deleting Directories
To delete a directory and all its contents, you use the -r
(or --recursive
) option with the rm
command:
rm -r myDirectory
# Output:
# (No output, but the directory 'myDirectory' and all its contents are deleted)
Here, we’ve deleted a directory named ‘myDirectory’ and everything within it. Be careful with this command – once a directory is deleted, it can’t be recovered.
Using Wildcards
The rm
command also supports wildcards, which can be incredibly handy. For example, to delete all .txt
files in a directory:
rm *.txt
# Output:
# (No output, but all '.txt' files in the current directory are deleted)
In this example, the *
is a wildcard that matches any character. So *.txt
matches any file that ends with .txt
, and all these files are deleted.
These are just a few examples of the power of the rm
command. With these techniques, you can efficiently manage and delete files and directories in bash. Remember, though, with great power comes great responsibility – always double-check your commands before running them.
Exploring Alternatives: The unlink
Command and Third-Party Tools
While the rm
command is a powerful tool for deleting files in bash, there are alternative methods that can provide more control or offer different features. Let’s explore some of these alternatives, such as the unlink
command and third-party tools.
The unlink
Command
The unlink
command is another way to delete a file in bash. It operates similarly to rm
, but it only accepts a single argument, meaning it can only delete one file at a time.
Here’s an example of how to use unlink
:
unlink report.txt
# Output:
# (No output, but the file 'report.txt' is deleted)
In this example, we’ve used unlink
to delete a file named ‘report.txt’. Like rm
, unlink
is irreversible, so use it with care.
Third-Party Tools
There are also third-party tools available that provide a graphical user interface (GUI) for file management, including deletion. Tools like Midnight Commander (mc
) or Ranger can be installed on most systems and provide a visual way to manage files, which some users may find easier or more intuitive.
Here’s an example of how to install and use Midnight Commander:
sudo apt-get install mc
mc
In this example, we first install Midnight Commander using apt-get
, then run it with the mc
command. From the interface, you can navigate to the file you want to delete and follow the on-screen prompts.
While these tools can be easier for some users, they do require installation and may not be available on all systems. They also remove you from the command line, which may not be ideal for all users or all situations.
In conclusion, while rm
is the most common method for deleting files in bash, there are alternatives available. Depending on your comfort level with the command line and your specific needs, these alternatives may be worth exploring.
Common Issues and Solutions in Bash File Deletion
While the rm
and unlink
commands are powerful tools in bash, you may encounter some common issues when deleting files. Let’s discuss these problems and provide solutions and workarounds.
‘Permission Denied’ Error
One common issue is the ‘Permission denied’ error. This typically happens when you don’t have the necessary permissions to delete a file.
Here’s an example of what this might look like:
rm important.txt
# Output:
# rm: cannot remove 'important.txt': Permission denied
To solve this, you can use the sudo
command to run rm
with superuser permissions:
sudo rm important.txt
# Output:
# (No output, but the file 'important.txt' is deleted)
In this example, we’ve used sudo
to run the rm
command, which allows us to delete ‘important.txt’ despite the initial permission issue.
Problems with Wildcards
Another common issue arises when using wildcards. For example, you might accidentally delete more files than intended if you’re not careful with your wildcard usage.
To prevent this, always double-check your command before running it. Additionally, you can use the -i
(or --interactive
) option with rm
to confirm each file deletion:
rm -i *.txt
# Output:
# rm: remove regular file 'file1.txt'?
In this example, bash asks for confirmation before deleting each .txt
file. This can prevent accidental deletions when using wildcards.
Remember, the rm
command is powerful and irreversible. Always double-check your commands, and consider using options like -i
for safety.
Understanding Bash and Its File Management Capabilities
To fully comprehend the process of deleting files in bash, it’s essential to understand the basics of bash itself and its file management capabilities.
Bash, short for ‘Bourne Again SHell’, is a command-line interpreter for Unix-based systems. It’s a powerful tool that allows users to interact directly with their system, performing tasks ranging from basic file navigation to complex programming tasks.
File Permissions in Bash
One fundamental concept in bash (and Unix systems in general) is file permissions. These permissions control who can read, write, and execute a file.
You can view a file’s permissions using the ls -l
command:
ls -l example.txt
# Output:
# -rw-r--r-- 1 user group 0 Jan 1 00:00 example.txt
In this example, the -rw-r--r--
string represents the file’s permissions. The first character (-
) indicates the file type (a dash -
for regular files, d
for directories). The next three characters (rw-
) represent the owner’s permissions (read and write in this case). The next three (r--
) are the group’s permissions, and the final three (r--
) are the permissions for all other users.
Understanding file permissions is crucial when deleting files, as you’ll need write permission to delete a file.
Wildcards in Bash
Another important concept is wildcards. In bash, wildcards are symbols that represent other characters – they’re like placeholders. The most common wildcard is the asterisk (*
), which represents any number of any characters.
Here’s an example of using a wildcard to list all .txt
files:
ls *.txt
# Output:
# report.txt notes.txt test.txt
In this example, *.txt
matches any file that ends with .txt
. Wildcards are incredibly useful when dealing with multiple files, but they should be used carefully to avoid unintended matches.
By understanding these fundamental concepts, you’ll be better equipped to manage and delete files in bash.
The Bigger Picture: File Deletion in System Administration and Script Writing
Deleting files might seem like a simple task, but it’s a crucial skill in many areas of IT, especially in system administration and script writing. Understanding the rm
command and its alternatives not only helps you manage your files but also lays the foundation for more complex tasks.
System Administration
In system administration, keeping a clean and well-organized system is crucial. Old and unnecessary files can take up storage space and slow down your system. Knowing how to delete these files efficiently using bash can significantly improve your system’s performance.
Script Writing
In script writing, you might need to delete temporary files created during the script’s execution. Understanding how to delete files using bash commands allows you to automate this process, making your scripts more efficient and cleaner.
Exploring Related Concepts
Mastering file deletion is just the beginning. There are many related concepts worth exploring, like file permissions and directory management. Understanding these concepts will give you a more in-depth knowledge of bash and its capabilities.
Further Resources for Bash Mastery
If you’re interested in learning more about bash and file management, here are a few resources to get you started:
- GNU Bash Reference Manual: This is the official manual for bash, providing a comprehensive guide to its features and capabilities.
Advanced Bash-Scripting Guide: This guide goes beyond the basics and explores advanced topics in bash scripting.
Earth Data Science – Bash Commands for Managing Directories and Files: This resource offers a concise introduction to Bash commands for managing directories and files.
Remember, practice is key when it comes to mastering bash. Don’t be afraid to experiment with different commands and techniques. Happy coding!
Wrapping Up: Mastering File Deletion in Bash
In this comprehensive guide, we’ve delved deep into the process of deleting files using bash, exploring everything from the basic rm
command to more advanced techniques and alternative methods.
We began with the basics, understanding the rm
command and its simple usage to delete files. We then moved onto more advanced usage of rm
, learning how to delete multiple files, directories, and using wildcards for broader file deletion. We also tackled common issues like ‘Permission Denied’ errors and problems with wildcards, providing solutions and workarounds to help you navigate these challenges.
Furthermore, we explored alternative approaches to file deletion in bash, introducing the unlink
command and discussing third-party tools for file management. These alternatives offer different features and can be a good fit for specific situations, adding more tools to your bash toolkit.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
rm Command | Simple, powerful, can handle multiple files and directories | Irreversible, requires caution when using wildcards |
unlink Command | Simple, can delete individual files | Only supports one file at a time |
Third-Party Tools | Provide a GUI, more intuitive for some users | Requires installation, may not be available on all systems |
Whether you’re just starting out with bash or you’re looking to level up your file management skills, we hope this guide has given you a deeper understanding of file deletion in bash. With this knowledge, you’re well equipped to manage your files effectively and efficiently. Happy coding!