Bash ‘Echo’ Command | Print to Terminal in Shell Scripting
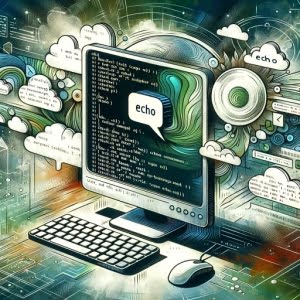
Ever found yourself puzzled by the echo command in Bash? You’re not alone. Many developers find the echo command a bit cryptic at first, but it’s actually a powerful tool in your Bash toolkit.
Think of the echo command as a town crier in Bash – it broadcasts your messages loud and clear in the terminal, making it a vital tool for debugging and displaying information.
In this guide, we’ll walk you through the process of mastering the echo command in Bash, from the basics to more advanced techniques. We’ll cover everything from simple text printing, handling variables, to using escape sequences and even alternative approaches.
So, let’s dive in and start mastering the echo command in Bash!
TL;DR: How Do I Use the Echo Command in Bash?
The
echo
command in Bash is used to print text or variables to the terminal with the syntax,echo 'sample text'
. It’s a simple yet powerful tool that can be used in a variety of ways to enhance your scripting capabilities.
Here’s a simple example:
echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this example, we use the echo command to print the string ‘Hello, World!’ to the terminal. The text within the single quotes is the argument for the echo command, and it gets printed exactly as it is.
But there’s so much more to the echo command than just printing simple text. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with the Echo Command
- Advanced Echo Commands: Escape Sequences and Variables
- Exploring Alternatives: The printf Command
- Troubleshooting the Echo Command
- Understanding Standard Output and the Echo Command
- Echo in the Larger Context: Scripts and Projects
- Further Resources for Mastering Bash Echo
- Wrapping Up: Mastering the Bash Echo Command
Getting Started with the Echo Command
The echo
command in Bash is one of the most basic and frequently used commands. It’s used to output the arguments it is being passed. These arguments could be a string of text, variables, or files.
Let’s start with a simple example:
echo 'Hello, Bash!'
# Output:
# 'Hello, Bash!'
In this example, we’re passing the string ‘Hello, Bash!’ to the echo command. The command then prints this string to the terminal.
Echo and Variables
The echo command can also be used to print the value of a variable. Here’s an example:
name='Bash'
echo "Hello, $name!"
# Output:
# 'Hello, Bash!'
In this case, we first define a variable name
and assign it the value ‘Bash’. We then use the echo command to print a string that includes the value of this variable. The $
symbol is used to denote that name
is a variable.
The echo command is simple, yet versatile. It’s a fundamental part of Bash scripting and mastering it will make your scripts more informative and easier to debug. However, like every command, it has its quirks which we’ll explore in the advanced section.
Advanced Echo Commands: Escape Sequences and Variables
After mastering the basics, it’s time to dive deeper into the echo command. We’ll explore more complex uses, such as using escape sequences and variables.
Echo with Escape Sequences
Escape sequences are characters that are interpreted by the echo command to perform a certain function. For example, the newline (\n
) and the tab (\t
) escape sequences.
echo -e "Hello,\nBash!"
# Output:
# Hello,
# Bash!
In this example, we use the -e
option with the echo command to enable the interpretation of the escape sequences. The \n
sequence creates a new line, so the word ‘Bash!’ is printed on a new line.
Echo with Variables and Command Substitution
The echo command can also be used in conjunction with command substitution. This allows you to assign the output of a command to a variable.
files=$(ls)
echo "The files in the current directory are: $files"
# Output:
# The files in the current directory are: file1 file2 file3
In this example, we use $(ls)
to assign the output of the ls
command (which lists the files in the current directory) to the files
variable. We then print this variable using the echo command.
These advanced techniques extend the functionality of the echo command, making it an even more powerful tool in your Bash scripting toolbox.
Exploring Alternatives: The printf Command
While the echo command is versatile and easy to use, there are other commands that offer more control and formatting options. One such command is printf
.
The printf Command: A More Powerful Alternative
The printf
command is similar to echo in that it prints text to the terminal. However, it offers more control over the output format.
printf "Hello, %s!
" "Bash"
# Output:
# Hello, Bash!
In this example, we use the printf
command to print a formatted string. The %s
is a format specifier, which in this case indicates a string. The \n
is an escape sequence for a new line. The string ‘Bash’ is passed as an argument and replaces the %s
in the output.
Benefits and Drawbacks
The main benefit of printf
over echo
is its ability to handle complex formatting. It can handle a variety of format specifiers, allowing you to control the output in detail. This makes it a powerful tool for generating formatted output in scripts.
However, printf
is also more complex to use. Unlike echo
, it doesn’t automatically add a new line at the end of the output, so you need to add manually if you want a new line.
Making the Right Choice
Choosing between echo
and printf
depends on your needs. If you’re simply printing text or variables, echo
is the easier choice. But if you need control over the output format, printf
is the more powerful tool. Understanding both commands will give you more flexibility in your Bash scripting.
Troubleshooting the Echo Command
While the echo command is generally straightforward, there are some common pitfalls that you might encounter.
Dealing with Special Characters
One common issue is dealing with special characters. For example, what if you want to print a string that includes a dollar sign ($)?
echo 'I have $100'
# Output:
# I have 100
In this example, the dollar sign ($) is not printed because in Bash, it’s used to denote a variable. To print the dollar sign, you need to escape it using a backslash (\
).
echo 'I have $100'
# Output:
# I have $100
Echo with Unset Variables
Another common issue is trying to print an unset variable.
echo $unset_var
# Output:
#
In this case, the echo command doesn’t print anything because the variable unset_var
is not set. To avoid this, you can use parameter expansion to provide a default value.
echo ${unset_var:-'Default Value'}
# Output:
# Default Value
In this example, if the variable unset_var
is not set, the string ‘Default Value’ is printed instead.
Best Practices and Optimization
When using the echo command, it’s best to always quote your strings. This prevents potential issues with special characters and white space. Also, when printing variables, use curly braces ({}) to clearly denote the variable name. This is especially important when the variable is followed by text.
Remember, mastering a command is not just about knowing how to use it, but also understanding how to troubleshoot common issues and apply best practices.
Understanding Standard Output and the Echo Command
To fully grasp the power of the echo command in Bash, it’s crucial to understand the concept of standard output and how the echo command interacts with it.
The Concept of Standard Output in Bash
In Bash, and Unix-like systems in general, there are three standard streams: standard input (stdin), standard output (stdout), and standard error (stderr). These streams are pre-opened input and output communication channels between a program and its environment.
Standard output, or stdout, is the stream where a program writes its output data. By default, this is the terminal.
How the Echo Command Interacts with Standard Output
The echo command in Bash sends its arguments to the standard output, which by default, is the terminal. This is why when you type echo 'Hello, World!'
, you see ‘Hello, World!’ printed in your terminal.
echo 'Understanding Bash Echo'
# Output:
# 'Understanding Bash Echo'
In this example, the echo command takes the string ‘Understanding Bash Echo’ as an argument and sends it to the standard output, which is your terminal.
But the standard output can be redirected. For instance, you can redirect the standard output to a file using the ‘>’ operator.
echo 'Understanding Bash Echo' > output.txt
In this case, there’s no output in the terminal because the standard output from the echo command has been redirected to the file output.txt.
Understanding these fundamentals of Bash and the standard output gives you a deeper insight into how the echo command works and how you can use it more effectively.
Echo in the Larger Context: Scripts and Projects
The echo command, while simple, plays a crucial role in larger Bash scripts and projects. Its ability to print variables, strings, and command outputs makes it a handy tool for debugging and providing informative messages to users.
Echo in Scripting
In larger Bash scripts, the echo command is often used to print status messages or the values of variables at certain points in the script. This can be invaluable for debugging and understanding the flow of the script.
#!/bin/bash
# A simple Bash script using echo
echo 'Starting the script...'
var='Bash Script'
echo "Running $var..."
# Output:
# Starting the script...
# Running Bash Script...
In this script, we use the echo command to print a status message at the beginning, and then to print the value of a variable.
Echo and Related Commands
In typical use cases, the echo command is often accompanied by other commands. For example, the read
command, which reads a line from the standard input and assigns it to a variable, is often used with echo.
#!/bin/bash
echo 'Enter your name:'
read name
echo "Hello, $name!"
# Output:
# Enter your name:
# [User enters 'Bash']
# Hello, Bash!
In this script, we use echo to prompt the user to enter their name. We then use the read command to read the user’s input and assign it to the variable name
. Finally, we use echo to print a greeting that includes the user’s name.
Further Resources for Mastering Bash Echo
To continue your journey in mastering the echo command in Bash, here are some additional resources:
- Bash scripting “echo” command: This GeeksforGeeks article explores the “echo” command in Bash scripting, covering its syntax, different options and flags.
GNU Bash Manual: The official manual for Bash, including a section on the echo command.
Advanced Bash-Scripting Guide: A comprehensive guide to Bash scripting, with many examples and exercises.
Wrapping Up: Mastering the Bash Echo Command
In this comprehensive guide, we’ve journeyed through the world of Bash, focusing specifically on the echo command.
We began with the basics, learning how to use the echo command to print text and variables to the terminal. We then ventured into more advanced territory, exploring complex uses of the echo command, such as using escape sequences and command substitution.
Along the way, we tackled common challenges you might face when using the echo command, providing solutions and workarounds for each issue. We also looked at alternative approaches to printing in Bash, comparing echo with the printf command.
Here’s a quick comparison of these commands:
Command | Simplicity | Flexibility | Formatting Control |
---|---|---|---|
echo | High | Moderate | Low |
printf | Moderate | High | High |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of the echo command and its capabilities.
With its simplicity and flexibility, the echo command is a powerful tool for Bash scripting. Now, you’re well equipped to use it effectively in your scripts. Happy coding!