Bash Exit Command Explained: Script Terminating Tutorial
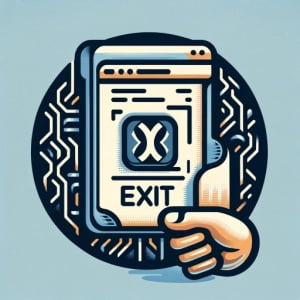
Are you grappling with the Bash exit command? It’s not uncommon. Many developers find themselves in a similar situation. Think of the Bash exit command as a courteous visitor – it knows exactly when it’s time to leave, making it a crucial part of well-written scripts.
In this guide, we’ll help you understand the Bash exit command, its usage, and its importance in scripting. We’ll start from the basics and gradually delve into more advanced topics, providing you with a comprehensive understanding of the Bash exit command.
So, let’s get started on our journey to master the Bash exit command!
TL;DR: What is the Bash exit
Command?
The
exit
command in Bash is used with the syntax,exit [n]
. Its function is to terminate a script and return a value to the parent script or shell. It’s a way to signal the end of a script’s execution and optionally return a status code to the calling process.
Here’s a simple example:
#!/bin/bash
echo 'Hello, World!'
exit 0
# Output:
# 'Hello, World!'
In this example, we’ve created a simple Bash script that prints ‘Hello, World!’ to the console and then terminates with an exit status of 0. The exit 0
command signals successful execution of the script.
This is a basic way to use the exit command in Bash, but there’s much more to learn about controlling script execution and handling exit statuses. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Bash Exit Command: A Beginner’s Guide
- Exit Statuses: Signaling Success or Failure in Bash Scripts
- Exploring Alternatives to Bash Exit Command
- Troubleshooting Bash Exit Command Issues
- Bash Scripting Fundamentals: Understanding Exit Command
- The Role of Bash Exit Command in Larger Scripts
- Wrapping Up: Bash exit Command
Bash Exit Command: A Beginner’s Guide
The exit command in Bash is used to terminate a script and return a value to the parent shell. It’s a fundamental part of Bash scripting, allowing you to control when your script ends and what it returns to the system.
The basic syntax for the exit command is straightforward:
exit [n]
Here, [n]
represents an exit status that the script returns to the shell or parent script. This is an optional parameter. If not provided, the exit command will return the status of the last command executed.
Let’s look at a simple example:
#!/bin/bash
echo 'Starting the script'
exit 0
echo 'This will not be printed'
# Output:
# 'Starting the script'
In this script, we first print ‘Starting the script’, then we execute the exit 0
command. This command terminates the script, so ‘This will not be printed’ is never executed.
The exit 0
command signals successful execution of the script. The number 0 is used to denote successful execution, while other numbers (1 to 255) are typically used to indicate various types of errors. This is the concept of exit statuses in Unix-like systems, which we’ll explore further in the next sections.
Exit Statuses: Signaling Success or Failure in Bash Scripts
As we’ve learned, the exit command in Bash can return a status to the parent shell or script. This status is an integer between 0 and 255, where 0 typically signals success, and any other number indicates some sort of failure or error.
This feature of the exit command is extremely useful for error handling in scripts. By checking the exit status of a command, we can make our scripts more robust and responsive to errors.
Let’s look at an example where we use different exit statuses to signal success or failure:
#!/bin/bash
if [ -f /etc/passwd ]; then
echo 'File exists'
exit 0
else
echo 'File does not exist'
exit 1
fi
# Output (if file exists):
# 'File exists'
# Output (if file does not exist):
# 'File does not exist'
In this script, we’re checking if the file ‘/etc/passwd’ exists. If it does, we print ‘File exists’ and exit with a status of 0, signaling success. If the file doesn’t exist, we print ‘File does not exist’ and exit with a status of 1, signaling an error.
This is a simple example, but it illustrates how you can use different exit statuses to control the flow of your scripts and indicate success or failure. In more complex scripts, you can use different exit statuses to indicate different types of errors, and then handle these errors appropriately in the parent script or shell.
Exploring Alternatives to Bash Exit Command
While the exit
command is a standard way to end a Bash script, there are alternative methods to terminate a script, such as the return
command or using kill
with the script’s Process ID (PID). These alternatives can be more appropriate in certain scenarios.
Using the ‘return’ Command
The return
command is used to exit a shell function. It can also provide a return status (just like the exit
command). However, unlike exit
, it does not terminate the shell where it’s called. Instead, it only exits from the current function or script.
Here’s an example:
#!/bin/bash
function hello() {
echo 'Hello, World!'
return 0
}
hello
echo 'This will be printed'
# Output:
# 'Hello, World!'
# 'This will be printed'
In this script, we define a function hello
that prints ‘Hello, World!’ and then returns with a status of 0. After calling the function, we print ‘This will be printed’. Unlike with exit
, this line is executed because return
only exits the function, not the entire script.
Using ‘kill’ with the Script’s PID
Another way to terminate a script is to use the kill
command with the script’s PID. This method is more drastic and should be used carefully, as it can abruptly terminate the script and any child processes it has spawned.
Here’s an example:
#!/bin/bash
echo 'Starting the script'
kill -9 $$
echo 'This will not be printed'
# Output:
# 'Starting the script'
In this script, we print ‘Starting the script’, then we use kill -9 $$
to terminate the script. The $$
variable in Bash holds the PID of the current shell, so kill -9 $$
sends a SIGKILL signal to the current shell, terminating the script. As a result, ‘This will not be printed’ is never executed.
Troubleshooting Bash Exit Command Issues
While the Bash exit command is straightforward to use, there are common issues that can arise, such as unexpected script termination or challenges in handling exit statuses. Let’s discuss these problems and provide solutions and best practices.
Unexpected Script Termination
One common issue is scripts terminating unexpectedly due to an exit
command. This can happen if you’re not careful about where and when you’re using exit
.
Consider the following script:
#!/bin/bash
if [ -f /etc/passwd ]; then
echo 'File exists'
exit 0
fi
echo 'This will not be printed'
# Output:
# 'File exists'
In this script, if the file ‘/etc/passwd’ exists, the script will terminate immediately after printing ‘File exists’. The line ‘This will not be printed’ will never be executed, even though it might seem safe at first glance.
To prevent unexpected termination, ensure that you’re using exit
command only where necessary and you’re aware of its implications.
Handling Exit Statuses
Another common issue is not properly handling exit statuses. If a script or command doesn’t execute successfully, and you’re not checking the exit status, you might not realize that there was an error.
Here’s an example:
#!/bin/bash
cd /nonexistent_directory
# Check the exit status
if [ $? -ne 0 ]; then
echo 'An error occurred'
exit 1
fi
# Output:
# 'An error occurred'
In this script, we attempt to change the directory to ‘/nonexistent_directory’, which doesn’t exist. We then check the exit status of the cd
command. If it’s not 0 (indicating an error), we print ‘An error occurred’ and exit with a status of 1.
By checking the exit status ($?
), we can detect errors and handle them appropriately. This is a best practice when using the exit
command in Bash scripts.
Bash Scripting Fundamentals: Understanding Exit Command
To fully grasp the use and importance of the exit
command in Bash, it’s crucial to understand some fundamental concepts of Bash scripting. This includes the concept of parent and child processes, the role of exit statuses in Unix-like systems, and the lifecycle of a Bash script.
Parent and Child Processes in Bash
In Unix-like systems, every process has a parent process, except for the initial process (init), which is created when the system boots up. When a parent process spawns a new process, the new process is called a child process.
Bash scripts work in the same way. When you run a script, it becomes a child process of the shell or script that ran it. The parent shell waits for the child script to finish before it continues.
Here’s a simple example:
#!/bin/bash
echo 'Parent process ID: '$$
bash -c 'echo "Child process ID: "$$'
# Output:
# Parent process ID: [PID of parent]
# Child process ID: [PID of child]
In this script, we print the PID of the parent process ($$
), then we run a new Bash shell with bash -c
, which becomes a child process. We print the PID of the child process, which will be different from the PID of the parent process.
Role of Exit Statuses in Unix-like Systems
Exit statuses are a fundamental concept in Unix-like systems. Every command that is executed returns an exit status, which signals success or failure. By convention, an exit status of 0 indicates success, while any other number (1 to 255) indicates an error. This is a powerful feature for error handling in scripts, as we’ve seen in the previous sections.
Lifecycle of a Bash Script
Understanding the lifecycle of a Bash script is crucial to using the exit
command effectively. A Bash script starts execution at the first line and continues line by line until it reaches an exit
command or the end of the script. The exit
command terminates the script immediately, even if there are more lines of code following it.
Here’s an example:
#!/bin/bash
echo 'Starting the script'
exit 0
echo 'This will not be printed'
# Output:
# 'Starting the script'
In this script, we print ‘Starting the script’, then we execute the exit 0
command. This command terminates the script, so ‘This will not be printed’ is never executed. This illustrates the lifecycle of a Bash script and the role of the exit
command in controlling it.
The Role of Bash Exit Command in Larger Scripts
Understanding the Bash exit command is not just about controlling the flow of small scripts. Its utility extends to larger scripts and programs, where it plays a vital role in ensuring their robustness and reliability.
Consider a large script with several functions. Each function might perform a specific task, such as downloading a file, processing data, or updating a database. If one of these tasks fails, it might not be appropriate to continue with the rest of the script.
In such cases, you can use the exit
command to terminate the script immediately when a function fails. You can also return an exit status that indicates the type of error, which can be logged for debugging purposes.
Here’s a hypothetical example:
#!/bin/bash
function download_file() {
# Attempt to download a file
# If the download fails, exit with status 1
wget https://example.com/file.txt || exit 1
}
function process_data() {
# Attempt to process the data
# If processing fails, exit with status 2
./process_data.py || exit 2
}
# Call the functions
download_file
process_data
# Output (if download fails):
# 'wget: unable to resolve host address ‘example.com’'
# Output (if processing fails):
# './process_data.py: No such file or directory'
In this script, we define two functions: download_file
and process_data
. Each function attempts to perform a task and uses the exit
command to terminate the script if the task fails.
Further Resources for Mastering Bash Exit Command
To deepen your understanding of the Bash exit command and related topics, consider exploring the following resources:
- GNU Bash Manual: The official manual for Bash, which covers the exit command and many other topics in detail.
- Bash Scripting Guide by The Linux Documentation Project: A comprehensive guide to Bash scripting, with many examples and exercises.
- Linuxize – Bash Exit Command: This tutorial explains the usage of the
exit
command in Bash scripting, and how to terminate a script and specify an exit status.
These resources can provide you with a deeper understanding of Bash scripting, helping you write more robust and reliable scripts.
Wrapping Up: Bash exit Command
In this comprehensive guide, we’ve delved into the Bash exit command, a fundamental part of Bash scripting. We’ve explored its basic usage, advanced techniques, and even some alternative approaches for terminating scripts.
We began with the basics, learning how to use the exit
command to terminate a script and return a value to the parent shell. We then delved into more advanced topics, such as using different exit statuses to indicate success or failure, and explored alternative methods to terminate a script, such as the return
command and using kill
with the script’s PID.
Along the way, we tackled common challenges you might encounter when using the exit
command, such as unexpected script termination or handling exit statuses, offering solutions and best practices to overcome these issues.
Method | Pros | Cons |
---|---|---|
exit command | Standard way to terminate scripts, can return a status | Can cause unexpected script termination if not used carefully |
return command | Only exits from the current function or script, can return a status | Can’t terminate the entire script |
kill command | Can terminate the script and any child processes | More drastic, can abruptly terminate the script |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the Bash exit command and its role in Bash scripting.
With its ability to control the flow of scripts and signal success or failure, the Bash exit command is a powerful tool in any scripter’s arsenal. Happy scripting!