Bash Exit Codes: Script Debugging and Flow Control Guide
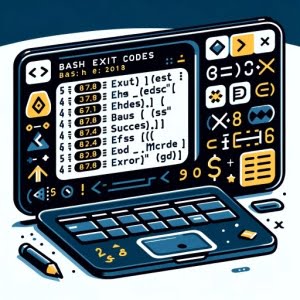
Have you ever been puzzled by the numbers that appear when a bash command fails? You’re not alone. Many developers find themselves scratching their heads when they encounter these cryptic numbers. Think of bash exit codes as a traffic light system – they provide signals to indicate the success or failure of a command.
This guide will help you decipher these codes and use them effectively in your bash scripts. We’ll explore everything from the basics of exit codes to advanced usage scenarios, as well as alternative approaches and troubleshooting tips.
So, let’s dive in and start mastering bash exit codes!
TL;DR: What Are Bash Exit Codes?
Bash exit codes are numerical codes that a bash command returns when it finishes executing such as
0
which indicatessuccess
, and5
which indicatesInput/output error
. You can retrieve exit codes with the special variable$?
.
Here’s a simple example:
$ ls /nonexistentdirectory
ls: cannot access '/nonexistentdirectory': No such file or directory
$ echo $?
2
In this example, we tried to list the contents of a directory that doesn’t exist. The ls
command failed and returned an exit code of 2. We used the special variable $?
to retrieve this exit code.
This is just a basic introduction to bash exit codes. There’s much more to learn about how to use them effectively in your bash scripts. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Retrieving Exit Codes: The Basics
In bash, we can retrieve the exit code of the last executed command using the special variable $?
. This variable holds the exit status of the most recently completed foreground command.
Let’s look at an example. Suppose we execute the ls
command to list the contents of a directory:
$ ls /home
Desktop Documents Downloads Music Pictures Public Templates Videos
$ echo $?
0
In this case, the ls
command successfully listed the contents of the /home
directory, so the exit code is 0, which we retrieved using echo $?
.
The $?
variable is extremely useful for understanding how your commands have executed, particularly within scripts where error handling is crucial.
However, there is a caveat: the $?
variable only holds the exit status of the last command executed. If another command is executed before you check $?
, the exit code from the previous command will be lost. For instance:
$ ls /nonexistentdirectory
ls: cannot access '/nonexistentdirectory': No such file or directory
$ ls /home
Desktop Documents Downloads Music Pictures Public Templates Videos
$ echo $?
0
In this example, we tried to check the exit code for the failed ls /nonexistentdirectory
command, but because we ran ls /home
before checking $?
, the exit code we retrieved was from the successful ls /home
command instead.
So, remember to check the $?
variable immediately after the command you’re interested in to avoid any confusion.
Controlling Script Flow with Exit Codes
Bash exit codes are not just for understanding command results. They can also be used to control the flow of execution in your bash scripts. This is particularly useful when you want to perform certain actions based on the success or failure of a command.
Consider the following script:
#!/bin/bash
cd /nonexistentdirectory
if [ $? -eq 0 ]; then
echo 'Directory changed successfully.'
else
echo 'Failed to change directory.'
fi
In this script, we try to change the current directory to /nonexistentdirectory
. After the cd
command, we use an if
statement to check the exit code. If the exit code is 0 (indicating success), we echo a success message. If the exit code is anything other than 0, we echo a failure message.
This is a basic example, but you can imagine how this could be expanded in a larger script. For example, you could use exit codes to decide whether to continue execution, retry a command, or exit the script entirely.
Remember, the $?
variable should be checked immediately after the command whose exit code you want to check. If you execute another command before checking $?
, you’ll get the exit code of that command instead.
Alternative Methods for Handling Exit Codes
Beyond the basic use of the $?
variable, there are other ways to handle exit codes in bash. Two of these methods are the set -e
command and the trap
command.
Using set -e
The set -e
command is a useful tool in bash scripting. When you use set -e
in your script, bash will exit the script immediately if any command exits with a non-zero status.
Here’s an example:
#!/bin/bash
set -e
cd /nonexistentdirectory
echo 'This will not be printed.'
In this script, the cd /nonexistentdirectory
command will fail because the directory does not exist. Because we used set -e
, bash will exit immediately after this command fails. The echo
command will not be executed.
Using trap
The trap
command allows you to catch signals and execute code when they occur. You can use trap
to catch the EXIT
signal, which occurs whenever your script exits. This allows you to run cleanup code or handle errors before your script ends.
Here’s an example:
#!/bin/bash
function handle_exit {
echo 'An error occurred. Cleaning up...'
}
trap handle_exit EXIT
cd /nonexistentdirectory
In this script, the handle_exit
function will be called whenever the EXIT
signal is caught. The cd /nonexistentdirectory
command will fail, triggering the EXIT
signal and calling the handle_exit
function.
Both set -e
and trap
provide powerful ways to handle errors in your bash scripts. However, they should be used with caution. set -e
can make your scripts brittle by causing them to exit unexpectedly, and trap
can make your scripts complex and difficult to understand. As always, it’s important to understand these tools and use them appropriately.
Troubleshooting Common Bash Exit Code Issues
As you work with bash exit codes, there are some common issues you might encounter. Let’s discuss these potential pitfalls and how to handle them.
Unexpected Exit Code Values
Sometimes, you might encounter unexpected exit code values. For example, you might expect a command to fail (exit code other than 0) but it returns 0, or vice versa. This usually happens when a command doesn’t follow the standard convention of returning 0 on success and non-zero on failure.
For example:
$ false || echo 'This will be printed.'
This will be printed.
$ echo $?
0
In this example, even though the false
command always fails (returns non-zero), the overall exit code is 0. This is because the echo
command was executed due to the OR (||
) operator, and echo
succeeded.
Handling Exit Codes from Piped Commands
When you use a pipe (|
) in bash, the exit code of the entire pipeline is the exit code of the last command. But what if you want to check the exit code of a command in the middle of the pipeline?
Here’s an example:
$ ls /nonexistentdirectory | wc -l
ls: cannot access '/nonexistentdirectory': No such file or directory
0
$ echo $?
0
In this case, ls /nonexistentdirectory
fails, but the exit code is 0 because the last command (wc -l
) succeeded.
To handle this, you can use the PIPESTATUS
array, which holds the exit codes of all commands in the pipeline:
$ ls /nonexistentdirectory | wc -l
ls: cannot access '/nonexistentdirectory': No such file or directory
0
$ echo ${PIPESTATUS[0]}
2
In this example, ${PIPESTATUS[0]}
gives the exit code of the first command in the pipeline (ls /nonexistentdirectory
), which is 2.
Remember, bash exit codes are a powerful tool, but they require careful handling. By understanding these common issues and how to handle them, you can use bash exit codes effectively in your scripts.
The Foundation: Unix Exit Codes
Before we delve deeper into bash exit codes, it’s important to understand the concept of exit codes in Unix-like operating systems, which includes Linux, macOS, and others.
In Unix-like operating systems, every command that is executed returns an exit code, also known as an exit status. By convention, an exit code of 0 means that the command was successful. Any non-zero exit code indicates that an error occurred.
The specific meaning of a non-zero exit code depends on the command. However, there are some standard exit codes that have specific meanings across most Unix-like systems. Here are a few examples:
- Exit code 1: General errors, such as “divide by zero” and other impermissible operations
- Exit code 2: Misuse of shell builtins, according to Bash documentation
- Exit code 126: Command invoked cannot execute
- Exit code 127: “command not found”
- Exit code 128: Invalid argument to exit
- Exit codes 128+n: Fatal error signal “n”
Here’s an example of how you might encounter these exit codes in a bash script:
#!/bin/bash
ls /nonexistentdirectory
if [ $? -eq 0 ]; then
echo 'Directory exists.'
else
echo 'Directory does not exist.'
fi
# Output:
# ls: cannot access '/nonexistentdirectory': No such file or directory
# Directory does not exist.
In this script, the ls /nonexistentdirectory
command fails because the directory does not exist. This command returns an exit code of 2, which we check using the $?
variable. Because the exit code is not 0, we echo ‘Directory does not exist.’
By understanding the concept of exit codes and their meanings, you can write more robust bash scripts that handle errors effectively.
The Bigger Picture: Exit Codes and Debugging
Bash exit codes play a crucial role in error handling and debugging in bash scripts. By checking the exit codes of commands, you can understand where your script is failing and why. This can help you fix bugs and improve the robustness of your scripts.
Exploring Related Concepts
Exit codes are just one part of the larger picture of error handling in bash. There are other related concepts that you might find useful to explore, such as signal handling in bash and process management.
Signal handling allows you to catch and handle signals sent to your script. For example, you might want to catch the SIGINT
signal (which is sent when you press Ctrl+C) and perform some cleanup before your script exits.
Process management involves controlling and monitoring the processes that your script starts. This can include starting processes in the background, bringing background processes to the foreground, and stopping processes.
Further Resources for Mastering Bash Exit Codes
If you’re interested in learning more about bash exit codes and related concepts, here are some resources that you might find useful:
- Advanced Bash-Scripting Guide: This guide covers a wide range of topics in bash scripting, including exit codes.
- GNU Bash Manual: The official manual for bash includes detailed information about exit codes and other features of bash.
- GeeksforGeeks’ Guide on Using Exit Code to Read from Terminal in Scripts – This guide demonstrates how to use the exit codes to read input from the terminal within a script.
Wrapping Up: Bash Exit Codes
In this comprehensive guide, we have embarked on a journey to understand the world of bash exit codes. These numerical signals, though often overlooked, play a critical role in the execution and flow control of bash scripts.
We began with the basics, learning how to retrieve the exit code of a command using the special variable $?
. We then delved into more advanced usage, exploring how to use exit codes to control the flow of execution in bash scripts. Along the way, we tackled common challenges you might encounter when working with bash exit codes, such as unexpected exit code values and handling exit codes from piped commands, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handling exit codes, such as using the set -e
command and the trap
command. These methods provide powerful ways to handle errors in your bash scripts, but they should be used with caution.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
$? variable | Simple and straightforward | Only holds the exit status of the last command executed |
set -e | Causes script to exit immediately if any command fails | Can make scripts brittle by causing them to exit unexpectedly |
trap command | Can catch signals and execute code when they occur | Can make scripts complex and difficult to understand |
Whether you’re just starting out with bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of bash exit codes and their importance in scripting.
The ability to understand and effectively use bash exit codes is a powerful tool in any developer’s toolkit. Now, you’re well equipped to handle any bash scripting challenges that come your way. Happy scripting!