Check If a Variable Is Empty in Bash: Shell Script How-to
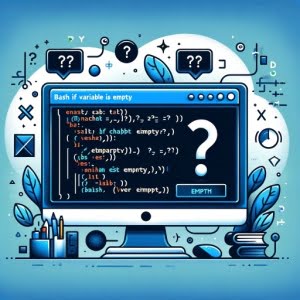
Are you finding it challenging to determine if a variable is empty in Bash? You’re not alone. Many developers find themselves in a bind when it comes to handling such checks in Bash, but we’re here to help.
Think of Bash as a detective, capable of finding out if a variable holds a value or not. It’s a powerful tool that can help you manage your scripts more effectively and avoid potential errors that could arise from unassigned or empty variables.
In this guide, we’ll walk you through how to use Bash to check if a variable is empty, from basic use to advanced techniques. We’ll cover everything from the basics of using the ‘-z’ option in an if statement, to dealing with different scenarios such as checking if a variable is unset or if it contains only whitespace, and even alternative approaches.
Let’s dive in and start mastering Bash variable checks!
TL;DR: How Do I Check if a Variable is Empty in Bash?
You can check if a Bash variable is empty by using an
if
statement and the'-z'
option,if [[ -z "$var" ]];
. This option checks if the length of the string is zero, indicating an empty variable. Here’s a simple example:
var=""
if [[ -z "$var" ]]; then
echo "Variable is empty"
fi
# Output:
# Variable is empty
In this example, we’ve declared a variable var
and assigned it an empty string. We then use an if statement with the ‘-z’ option to check if var
is empty. If the condition is true, it echoes ‘Variable is empty’.
This is a basic way to check if a variable is empty in Bash, but there’s much more to learn about handling variables and conditionals in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Checking if a Bash Variable is Empty: The Basics
- Dealing with Unset Variables and Whitespace in Bash
- Exploring Alternative Methods for Empty Variable Checks in Bash
- Navigating Common Issues in Bash Variable Checks
- Bash Scripting, Variables, and Conditionals: The Fundamentals
- Expanding Your Bash Scripting Skills Beyond Empty Variable Checks
- Wrapping Up: Mastering Bash Variable Checks
Checking if a Bash Variable is Empty: The Basics
For beginners in Bash scripting, a common task you’ll encounter is checking if a variable is empty. Bash provides a built-in solution for this using the ‘-z’ option in an if statement. The ‘-z’ option checks if the length of the string is zero, indicating an empty variable.
Let’s look at an example:
name=""
if [[ -z "$name" ]]; then
echo "Name variable is empty"
else
echo "Name variable is not empty"
fi
# Output:
# Name variable is empty
In this example, we’ve declared a variable name
and assigned it an empty string. We then use an if statement with the ‘-z’ option to check if name
is empty. If the condition is true, it echoes ‘Name variable is empty’. If the condition is false, it echoes ‘Name variable is not empty’.
The advantage of this method is its simplicity and readability. It’s easy to understand what the script is doing even for beginners. However, one potential pitfall is that it only checks if the variable is empty, not if it’s unset. An unset variable and an empty variable are not the same, and in some scenarios, you may want to handle them differently. We’ll cover this in more detail in the ‘Advanced Use’ section.
Dealing with Unset Variables and Whitespace in Bash
As you gain more experience in Bash scripting, you’ll encounter more complex scenarios. Two such cases involve dealing with unset variables and variables containing only whitespace. Let’s break down these situations and how to handle them.
Checking if a Variable is Unset in Bash
An unset variable is different from an empty variable in Bash. An unset variable has never been declared, whereas an empty variable has been declared but not assigned a value.
Here’s how you can check if a variable is unset:
if [ -z "${var+x}" ]; then
echo "var is unset";
else
echo "var is set";
fi
# Output:
# var is unset
In this example, ${var+x}
is a parameter expansion which evaluates to nothing if var
is unset, and substitutes the string x
otherwise. The -z
test returns true if the length of the string is zero. Therefore, if var
is unset, the statement will echo ‘var is unset’.
Checking if a Variable Contains Only Whitespace in Bash
In some cases, a variable might not be empty but may contain only whitespace characters. Here’s how you can check for this scenario:
var=" "
if [[ -z "${var// /}" ]]; then
echo "var contains only whitespace"
else
echo "var contains some characters"
fi
# Output:
# var contains only whitespace
In this example, ${var// /}
is a parameter expansion which replaces all spaces in the variable var
. If var
contains only spaces, this will result in an empty string, and the -z
test will return true.
These advanced techniques allow you more control and precision in your scripts. Remember, the key to effective scripting is understanding and correctly handling all possible scenarios.
Exploring Alternative Methods for Empty Variable Checks in Bash
As you progress in your Bash scripting journey, it’s beneficial to learn about other methods for checking if a variable is empty. Two such alternatives include using the ‘-n’ option and the ‘test’ command. Let’s delve into these techniques.
Using ‘-n’ Option in Bash
The ‘-n’ option in Bash checks if the string length is non-zero, which indicates that the variable is not empty. Here’s an example:
var="Hello"
if [[ -n "$var" ]]; then
echo "Variable is not empty"
else
echo "Variable is empty"
fi
# Output:
# Variable is not empty
In this script, we’ve declared a variable var
and assigned it the string ‘Hello’. The if statement with the ‘-n’ option checks if var
is not empty. If the condition is true, it echoes ‘Variable is not empty’. If the condition is false, it echoes ‘Variable is empty’.
This method is the opposite of the ‘-z’ option. It’s equally simple and readable, making it a good alternative for checking if a variable is not empty.
Using ‘test’ Command in Bash
The ‘test’ command in Bash is another way to check if a variable is empty. It’s an older but still widely used method. Here’s how you can use it:
var=""
test -z "$var" && echo "Variable is empty" || echo "Variable is not empty"
# Output:
# Variable is empty
In this code snippet, the ‘test’ command checks if var
is empty using the ‘-z’ option. If the test returns true, it echoes ‘Variable is empty’. If the test returns false, it echoes ‘Variable is not empty’.
The ‘test’ command is less readable than the ‘-z’ and ‘-n’ options, especially for beginners. However, it’s a powerful tool that can handle more complex conditions, making it a useful method to know.
Remember, the best method to use depends on your specific needs and the complexity of your script. It’s beneficial to be aware of these alternatives and understand when to use each one.
While checking if a variable is empty in Bash is straightforward, you might encounter some common issues. These can include syntax errors or unexpected behavior. Let’s explore these problems and how to address them.
Dealing with Syntax Errors
A common issue in Bash scripting is syntax errors. These errors occur when the script doesn’t adhere to the correct syntax rules of Bash. Here’s an example:
var=""
if [ -z $var ]; then
echo "Variable is empty"
fi
# Output:
# syntax error: unexpected end of file
In this example, we forgot to wrap the variable var
in double quotes inside the if statement. This leads to a syntax error when the script is run. The correct syntax should be if [ -z "$var" ]; then
.
Handling Unexpected Behavior
Sometimes, your script might run without errors, but it doesn’t behave as expected. This can happen when a variable is unset:
unset var
if [[ -z "$var" ]]; then
echo "Variable is empty"
fi
# Output:
# Variable is empty
In this example, the variable var
is unset. When the script checks if var
is empty, it returns true. However, this might not be the expected behavior, as an unset variable is different from an empty variable. To handle this scenario, you can use the technique we discussed in the ‘Advanced Use’ section.
Remember, troubleshooting is a critical skill in Bash scripting. The key is to understand the syntax rules and how Bash handles variables. With this knowledge, you can navigate these issues and ensure your scripts run as expected.
Bash Scripting, Variables, and Conditionals: The Fundamentals
To fully grasp the task of checking if a variable is empty in Bash, it’s essential to understand the fundamentals of Bash scripting, variables, and conditional statements.
Bash Scripting: The Basics
Bash, or Bourne Again SHell, is a command language and Unix shell. It’s widely used for its ability to control job control, shell functions and scripts, and command-line editing.
A Bash script is a plain text file containing a series of commands. These scripts allow us to automate tasks and manage system configurations. Here’s a simple Bash script example:
#!/bin/bash
echo "Hello, world!"
# Output:
# Hello, world!
In this script, #!/bin/bash
is called a shebang, which tells the system that this script should be executed with Bash. The echo
command is used to print ‘Hello, world!’ to the console.
Understanding Variables in Bash
A variable in Bash is a symbol or name that stands for a value. Variables are case-sensitive and are typically in uppercase. Here’s how you can declare a variable in Bash:
#!/bin/bash
GREETING="Hello, world!"
echo $GREETING
# Output:
# Hello, world!
In this script, GREETING
is a variable that holds the value ‘Hello, world!’. We use the echo
command to print the value of GREETING
to the console.
Conditional Statements in Bash
Conditional statements in Bash allow us to make decisions in our scripts. The most common conditional statement is the if statement. Here’s an example:
#!/bin/bash
VAR="Hello"
if [[ "$VAR" == "Hello" ]]; then
echo "The variable VAR contains the string Hello"
else
echo "The variable VAR does not contain the string Hello"
fi
# Output:
# The variable VAR contains the string Hello
In this script, we’re using an if statement to check if VAR
contains the string ‘Hello’. If the condition is true, it echoes ‘The variable VAR contains the string Hello’. If the condition is false, it echoes ‘The variable VAR does not contain the string Hello’.
Understanding these fundamentals will help you better understand the task of checking if a variable is empty in Bash. With this knowledge, you can write effective scripts and avoid common pitfalls.
Expanding Your Bash Scripting Skills Beyond Empty Variable Checks
Checking if a variable is empty in Bash is a fundamental task, but it’s just the tip of the iceberg when it comes to Bash scripting. Understanding how to handle variables is crucial in larger scripts or projects where you’re dealing with multiple variables and complex conditions.
Exploring Related Concepts
Once you’ve mastered checking if a variable is empty in Bash, you can explore related concepts that will enhance your scripting skills. For instance, learning about string manipulation in Bash can help you modify and interact with strings more effectively. This can be useful when you’re handling variables that contain string values.
Another important concept is loops in Bash. Loops allow you to execute a block of code multiple times, which can be particularly useful when you’re working with arrays of variables. You can use loops to iterate over each element in the array and perform operations, such as checking if a variable is empty.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting, here are some resources that you might find helpful:
- Bash Guide for Beginners – This guide provides a comprehensive introduction to Bash scripting, covering everything from basic commands to advanced scripting techniques.
Advanced Bash-Scripting Guide – If you’re ready to take your Bash scripting skills to the next level, this guide offers in-depth explanations and examples on advanced topics.
Bash Academy – This interactive platform offers a hands-on approach to learning Bash scripting, with exercises and quizzes to reinforce your learning.
Remember, the journey to mastering Bash scripting is a marathon, not a sprint. Take your time to understand each concept, practice regularly, and don’t be afraid to seek help when you get stuck. Happy scripting!
Wrapping Up: Mastering Bash Variable Checks
In this comprehensive guide, we’ve explored the ins and outs of checking if a variable is empty in Bash, a fundamental yet crucial task in Bash scripting.
We began with the basics, learning how to check if a variable is empty using the ‘-z’ option in an if statement. We then delved into more advanced techniques, such as handling unset variables and variables containing only whitespace. Along the way, we’ve explored alternative methods for checking if a variable is empty, such as using the ‘-n’ option and the ‘test’ command.
Throughout this journey, we’ve tackled common issues you might face when checking if a variable is empty in Bash, such as syntax errors and unexpected behavior. We’ve provided solutions and workarounds for each issue, equipping you with the knowledge to navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘-z’ Option | Simple and readable | Only checks if variable is empty |
Unset Variable Check | Handles unset variables | More complex syntax |
Whitespace Check | Handles variables with only whitespace | More complex syntax |
‘-n’ Option | Checks if variable is not empty | Doesn’t handle unset variables |
‘test’ Command | Can handle complex conditions | Less readable |
Whether you’re just starting out with Bash scripting or you’re looking to refine your skills, we hope this guide has given you a deeper understanding of how to check if a variable is empty in Bash. With this knowledge, you’re well-equipped to write effective and robust Bash scripts. Happy scripting!