How to Use Classnames NPM for Dynamic CSS
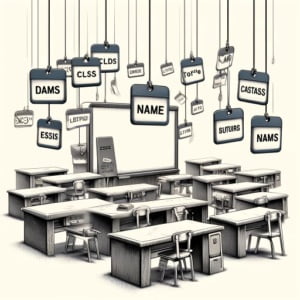
Are you finding the task of managing CSS class names in your JavaScript or React projects more like a juggling act? You’re not alone. Many developers face this challenge, but the ‘classnames’ npm package can simplify this process significantly.
While developing software at IOFLOOD, we’ve explored the ‘classnames’ package as a solution to enhance code readability and maintainability in our projects. We understand many customers on our dedicated servers may face similar concerns, so we have provided practical guidance and code examples to help you learn how classnames
could fit your needs.
This guide will walk you through the basics to advanced usage of ‘classnames’, making your code cleaner and your life easier. From simple concatenation to conditional class name management, ‘classnames’ offers a streamlined approach to handling CSS in your projects. By the end of this guide, you’ll have a solid understanding of how to leverage ‘classnames’ to its full potential.
Let’s dive into ‘classnames’ and learn how it can help us craft dynamic and visually engaging user interfaces.
TL;DR: How Do I Use ‘classnames’ in My Project?
To dynamically manage your CSS class names in JavaScript or React projects, install ‘classnames’ via npm and utilize it for conditional class name concatenation. Here’s a quick example to get you started:
import classNames from 'classnames';
const buttonClass = classNames('btn', {'btn-primary': isPrimary});
console.log(buttonClass);
# Output:
# 'btn btn-primary' (if isPrimary is true)
In this example, we import the classnames
module and use it to dynamically assign ‘btn-primary’ class to our button if the isPrimary
condition is true. This results in a cleaner and more readable way to handle conditional class names in your projects.
Eager to dive deeper into making your web projects sleek and efficient with ‘classnames’? Keep reading for a comprehensive guide on leveraging this powerful npm package for both basic and advanced scenarios.
Table of Contents
Getting Started with Classnames
Installing Classnames
Before diving into the coding part, let’s ensure ‘classnames’ is added to your project. For those new to npm (Node Package Manager), it’s a package manager for JavaScript that allows you to install third-party libraries like ‘classnames’. To install, open your terminal and run the following command:
npm install classnames
This command fetches the ‘classnames’ package from the npm repository and adds it to your project, making it available for use.
Your First Classname Concatenation
Now that ‘classnames’ is part of your project, let’s explore its basic functionality: concatenating class names. Imagine you’re building a user interface and need a button with multiple classes for styling. Here’s how ‘classnames’ simplifies the process:
import classNames from 'classnames';
const buttonClasses = classNames('btn', 'btn-large', 'btn-success');
console.log(buttonClasses);
# Output:
# 'btn btn-large btn-success'
In this example, we import ‘classnames’ and use it to combine three class names into one string. This is particularly useful for managing multiple classes without manually concatenating strings, making your code more readable and maintainable. The classNames
function takes any number of arguments and intelligently combines them, even filtering out falsy values automatically. This initial demonstration of ‘classnames’ showcases its simplicity and the immediate benefits it offers for your web projects.
Advanced Classnames Usage
Conditional Class Names
When developing web applications, especially with React, you often encounter scenarios where you need to apply CSS class names conditionally. This is where ‘classnames’ truly shines, offering a clean and concise syntax. Let’s dive into an example where we conditionally apply classes based on component state:
import classNames from 'classnames';
class MyComponent extends React.Component {
render() {
const buttonClass = classNames({
'btn': true,
'btn-primary': this.props.isPrimary,
'btn-disabled': !this.props.isEnabled
});
return <button className={buttonClass}>Click Me!</button>;
}
}
# Output:
# '<button class="btn btn-primary">Click Me!</button>' (if isPrimary is true and isEnabled is true)
# '<button class="btn btn-disabled">Click Me!</button>' (if isPrimary is false and isEnabled is false)
In this example, we use an object to pass class names to classNames
, where the key is the class name and the value is a condition that determines if the class should be applied.
Combining Classes
In a UI component library, you may have different variants of a button, such as primary, secondary, and tertiary. Depending on the context, you might want to apply additional styling, like size variations or disabled states.
import classNames from 'classnames';
const Button = ({ variant, size, disabled }) => {
const buttonClasses = classNames(
'button',
{ 'button-primary': variant === 'primary' },
{ 'button-secondary': variant === 'secondary' },
{ 'button-tertiary': variant === 'tertiary' },
{ 'button-large': size === 'large' },
{ 'button-disabled': disabled }
);
return (
<button className={buttonClasses} disabled={disabled}>
Click me
</button>
);
};
## Expected Output:
## If variant is 'primary' and size is 'large':
## buttonClasses will be 'button button-primary button-large'
In this example, we define a React functional component named ‘Button’, which accepts props like ‘variant’, ‘size’, and ‘disabled’. We use the ‘classNames’ function from the ‘classnames’ package to conditionally generate a string of class names based on the props passed to the component. Depending on the values of props like ‘variant’ and ‘size’, different class names are conditionally applied to the button element. The resulting ‘buttonClasses’ string contains a combination of base class names (‘button’) and additional class names based on the props, allowing for dynamic styling of the button component.
By mastering these advanced uses of ‘classnames’, you’re equipped to handle complex styling scenarios with ease, making your web applications more dynamic and responsive to user interactions.
Exploring Alternatives to Classnames
Other Methods for Managing CSS
While ‘classnames’ is a powerful tool for managing CSS class names in JavaScript and React projects, it’s not the only option available. Let’s explore some alternative methods and npm packages you might consider, and compare them with ‘classnames’ to help you make the best choice for your specific project needs.
One popular alternative is using template literals in ES6, which can offer a straightforward way to concatenate class names conditionally without any additional packages. Here’s how you might achieve similar functionality with template literals:
const isPrimary = true;
const buttonClass = `btn ${isPrimary ? 'btn-primary' : ''}`;
console.log(buttonClass);
# Output:
# 'btn btn-primary'
In this example, we use a template literal to conditionally add ‘btn-primary’ to the class list based on the isPrimary
variable. This method provides a native JavaScript approach to conditional class names but lacks the utility and ease of use found in ‘classnames’ for more complex scenarios.
Other NPM Packages
Besides ‘classnames’, there are other npm packages designed to help with class name management, such as styled-components
for styled components in React. Styled-components allow for CSS-in-JS styling, enabling developers to write CSS code directly within their JavaScript files, which can be a powerful approach for component-specific styling.
import styled from 'styled-components';
const Button = styled.button`
background: ${(props) => (props.primary ? 'blue' : 'gray')};
color: white;
`;
<Button>Primary Button</Button>
This code snippet demonstrates how styled-components
allows for dynamic styling based on props, offering a different approach to managing styles in React applications. While powerful, this method introduces a different paradigm of styling that may not suit every project or developer preference.
Making the Right Choice
Choosing between ‘classnames’ and its alternatives depends on your project’s complexity, your comfort with JavaScript or React, and your specific styling needs. ‘classnames’ offers simplicity and ease of use for dynamically managing class names, making it an excellent choice for many projects. However, exploring alternatives like template literals or styled-components
can provide additional flexibility and power for projects that might benefit from a more integrated styling approach.
Optimizing ‘Classnames’ Usage
Avoid Common Pitfalls
While ‘classnames’ is a robust tool for managing CSS class names in your web projects, there are common pitfalls that can hinder its effectiveness. Understanding these pitfalls and how to avoid them is crucial for optimizing your usage of ‘classnames’ for better performance and readability.
One common issue is overcomplication. It’s easy to fall into the trap of using ‘classnames’ for every single class name, even when it’s not necessary. This can lead to unnecessarily verbose code. Here’s how you might simplify:
// Overcomplicated usage
const buttonClass = classNames({ 'btn': true, 'btn-primary': isPrimary, 'hidden': isHidden });
// Simplified usage
const buttonClass = classNames('btn', { 'btn-primary': isPrimary, 'hidden': isHidden });
# Output:
# 'btn btn-primary' or 'btn hidden'
In this example, we show an overcomplicated way of using ‘classnames’ compared to a simplified approach. By directly including always-true classes (‘btn’ in this case) outside the object, the code becomes more readable and easier to maintain. This demonstrates the importance of selectively using ‘classnames’ to combine class names, especially when some class names are not conditional.
Performance Considerations
Another consideration is the impact of ‘classnames’ on performance. While ‘classnames’ is lightweight and generally does not significantly affect performance, excessive or unnecessary use in large-scale applications can add up. To mitigate this, review your usage of ‘classnames’ and ensure it’s used only when it provides clear benefits over traditional class name management methods.
By being mindful of these common pitfalls and performance considerations, you can make the most out of ‘classnames’ in your web development projects. Remember, the goal is to keep your codebase clean, readable, and performant, and ‘classnames’ is a tool that, when used correctly, can help you achieve just that.
Exploring Class Name Management
Why Classnames Matter in Web Development
In the evolving landscape of web development, efficient management of CSS class names is more than just a convenience—it’s a necessity. As projects grow in complexity, the ability to dynamically manage class names becomes crucial for maintaining a clean, readable, and scalable codebase. This is where ‘classnames’, an npm package, steps in as a game-changer.
To understand its importance, consider a scenario where you’re handling multiple class names based on different states or props in a React component. Without a utility like ‘classnames’, your code might look cluttered and be prone to errors:
const buttonClass = `btn ${isActive ? 'active' : ''} ${isDisabled ? 'disabled' : ''}`;
Though this approach works, it quickly becomes unwieldy as conditions and class names increase. ‘Classnames’ simplifies this with a more intuitive syntax:
import classNames from 'classnames';
const buttonClass = classNames('btn', { 'active': isActive, 'disabled': isDisabled });
console.log(buttonClass);
# Output:
# 'btn active' (if isActive is true and isDisabled is false)
# 'btn disabled' (if isActive is false and isDisabled is true)
In this example, ‘classnames’ allows for a cleaner, more readable way to conditionally apply class names, demonstrating its utility in enhancing code quality. By providing a straightforward API for combining class names, ‘classnames’ addresses a common pain point in web development, making it an indispensable tool in the modern developer’s toolkit.
Classnames in the Web Dev Toolkit
‘Classnames’ fits into the broader context of web development tools and practices by offering a solution that is both simple and powerful. Its ability to integrate seamlessly with frameworks like React, and its support for both JavaScript and TypeScript, makes it a versatile choice for developers. As web applications continue to evolve, tools like ‘classnames’ play a pivotal role in ensuring that developers can keep up with the demand for more dynamic, responsive, and user-friendly interfaces. Embracing ‘classnames’ is a step towards writing more maintainable and efficient code, a goal that resonates with the core objectives of modern web development.
Practical Usage with Classnames
Combining Classnames with Other Tools
As your web projects grow in size and complexity, ‘classnames’ continues to be a valuable ally, but its true power shines when combined with other tools and libraries. Imagine integrating ‘classnames’ with a CSS-in-JS library like Styled Components or Emotion. This combination allows for dynamic styling with scoped CSS, offering an even more powerful and flexible development workflow.
Consider this example where ‘classnames’ and Styled Components are used together in a React project:
import styled from 'styled-components';
import classNames from 'classnames';
const StyledButton = styled.button`
background: blue;
&.big {
font-size: 20px;
}
&.small {
font-size: 12px;
}
`;
const buttonClass = classNames({ 'big': isBig, 'small': !isBig });
Click Me
# Output:
# '<button class="big">Click Me</button>' (if isBig is true)
# '<button class="small">Click Me</button>' (if isBig is false)
In this code snippet, ‘classnames’ is used to dynamically add ‘big’ or ‘small’ class names to the StyledButton
component based on the isBig
state. This demonstrates how ‘classnames’ can be seamlessly integrated with styled components to manage class names dynamically, offering a scalable solution for styling in larger projects.
Further Resources for Classnames Mastery
To continue your journey towards mastering ‘classnames’ and enhancing your web development skills, here are three hand-picked resources that delve deeper into the topic and related areas:
- Classnames GitHub: Visit the official ‘classnames’ GitHub page for comprehensive documentation, usage examples, and community contributions.
CSS Tricks Flexbox Guide: Understanding CSS Flexbox is crucial for modern web development. This guide by CSS Tricks offers an in-depth look at Flexbox, complementing your knowledge of ‘classnames’.
Inverted Triangle CSS: Learn about ITCSS (Inverted Triangle CSS), a scalable and maintainable approach to CSS architecture, which can be enhanced by utilities like ‘classnames’.
By exploring these resources, you’ll gain further insights into ‘classnames’ and related web development practices, empowering you to build more dynamic, responsive, and user-friendly web applications.
Recap: Classnames for npm Projects
In this comprehensive guide, we’ve explored the versatility and utility of ‘classnames’, an indispensable npm package for managing CSS class names in your web development projects. From simplifying the process of concatenating class names to offering a dynamic way of handling classes based on conditions, ‘classnames’ has proven to be a valuable tool in the developer’s toolkit.
We began with the basics, showing you how to install ‘classnames’ and use it for simple class name concatenation. We then delved into more advanced usage, demonstrating how ‘classnames’ can be used to conditionally apply class names, making your code cleaner and more maintainable.
Next, we explored alternative approaches for managing class names, comparing ‘classnames’ with other methods and npm packages. This comparison highlighted the unique benefits of ‘classnames’, such as its simplicity and flexibility, while also acknowledging situations where other tools might be more appropriate.
Approach | Simplicity | Flexibility | Use Case |
---|---|---|---|
Classnames | High | High | Dynamic class names |
Template Literals | Moderate | Low | Simple concatenations |
Styled Components | Low | High | CSS-in-JS styling |
Whether you’re just starting out with ‘classnames’ or looking to enhance your web development skills, we hope this guide has provided you with a deeper understanding of how to use ‘classnames’ effectively in your projects.
With ‘classnames’, you have a powerful tool at your disposal for managing CSS class names, enabling you to create more dynamic, responsive, and user-friendly web applications. We encourage you to experiment with ‘classnames’ in different scenarios, pushing the boundaries of what you can achieve with your web projects. Happy coding!