How-To Use Command-Line Parameters ($@) in Bash
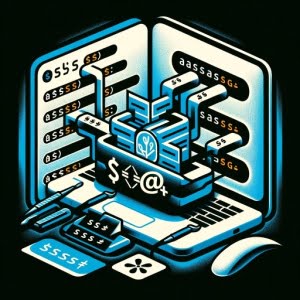
Are you finding it challenging to understand the $@ symbol in your bash scripts? You’re not alone. Many developers find this special variable a bit puzzling, but it’s actually a powerful tool in bash scripting.
Think of $@ in bash as a skilled juggler, handling multiple command-line arguments at once. It’s a special variable that holds all the positional parameters in a script, providing a versatile and handy tool for various tasks.
In this guide, we’ll demystify the $@ variable and show you how to use it effectively in your bash scripts. We’ll cover everything from the basics of using $@ to handle positional parameters to more advanced techniques, as well as alternative approaches.
Let’s get started and master $@ in Bash!
TL;DR: What is $@ in Bash and How Do I Use It?
In Bash,
$@
is a special variable that holdsall the positional parameters of a script
. The simplest syntax for this variable isecho $@
, which will output all command-line arguments in your scripts. Here’s a simple example:
#!/bin/bash
echo $@
# Run the script with arguments
./script.sh Hello World
# Output:
# Hello World
In this example, we’ve created a simple bash script that uses the $@ variable to print all the command-line arguments. When we run the script with ‘Hello’ and ‘World’ as arguments, it prints ‘Hello World’.
This is just a basic way to use $@ in Bash, but there’s much more to learn about handling command-line arguments effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding $@: Bash’s Positional Parameters Handler
- Advanced $@: Loops and Shift Command
- Alternative Approaches: Utilizing Other Special Variables in Bash
- Troubleshooting $@: Common Pitfalls and Solutions
- Bash Scripting: Positional Parameters and Command-Line Arguments
- $@ in Larger Scripts: Enhancing Your Bash Projects
- Wrapping Up: Commanding Bash with $@
Understanding $@: Bash’s Positional Parameters Handler
The $@ variable in bash is a special variable designed to handle positional parameters. In simpler terms, it holds all the command-line arguments passed to your script. It’s like a container that can hold multiple items (arguments) at once.
Here’s an example that demonstrates how $@ works:
#!/bin/bash
for arg in $@
do
echo Argument: $arg
done
# Run the script with arguments
./script.sh Apple Banana Cherry
# Output:
# Argument: Apple
# Argument: Banana
# Argument: Cherry
In this script, we use a for loop to iterate over all the arguments stored in the $@ variable. Each argument is printed on a new line. When we run the script with ‘Apple’, ‘Banana’, and ‘Cherry’ as arguments, each fruit is printed separately.
The $@ variable is incredibly useful when you need to handle multiple command-line arguments in your bash scripts. It’s flexible, easy to use, and can handle any number of arguments. However, it’s important to remember that $@ doesn’t keep track of argument order. If the order of arguments is important in your script, you’ll need to handle them individually using $1, $2, etc.
In the next section, we’ll dive deeper into more advanced usage scenarios of $@ in bash scripts.
Advanced $@: Loops and Shift Command
As you become more comfortable with $@, you can start using it in more complex ways. One such use is in combination with loops and the shift command.
Using $@ with Loops
Loops are a powerful tool in bash scripting that allow you to repeat actions for each item in a list. When combined with $@, you can perform actions for each command-line argument. Here’s an example:
#!/bin/bash
for arg in $@
do
echo Argument: $arg
done
# Run the script with arguments
./script.sh Apple Banana Cherry
# Output:
# Argument: Apple
# Argument: Banana
# Argument: Cherry
In this script, we use a for loop to iterate over all the arguments stored in the $@ variable. Each argument is printed on a new line. When we run the script with ‘Apple’, ‘Banana’, and ‘Cherry’ as arguments, each fruit is printed separately.
Using $@ with the Shift Command
The shift command is another handy tool to use with $@. The shift command shifts the positional parameters to the left, discarding the first parameter and reducing the total count by one. Here’s an example of how you can use $@ with the shift command:
#!/bin/bash
echo First Argument: $1
shift
echo Remaining Arguments: $@
# Run the script with arguments
./script.sh Apple Banana Cherry
# Output:
# First Argument: Apple
# Remaining Arguments: Banana Cherry
In this script, we first print the first argument ($1), then use the shift command to discard it. After the shift, $@ holds the remaining arguments, which we then print. When we run the script with ‘Apple’, ‘Banana’, and ‘Cherry’ as arguments, it prints ‘Apple’ as the first argument and ‘Banana Cherry’ as the remaining arguments.
Alternative Approaches: Utilizing Other Special Variables in Bash
While $@ is a powerful tool for handling multiple command-line arguments, bash scripting offers other special variables that can be used to manage arguments. These include $*, $1, $2, and so on. Understanding these alternatives can help you choose the right tool for your specific scripting needs.
The $* Variable
The $* variable, like $@, holds all positional parameters. However, when quoted, “$*” treats all the parameters as a single string, while “$@” treats each parameter as a separate string. Here’s an example demonstrating the difference:
#!/bin/bash
echo "Using $*": $*
echo "Using $@": $@
# Run the script with arguments
./script.sh Apple Banana Cherry
# Output:
# Using $*: Apple Banana Cherry
# Using $@: Apple Banana Cherry
In this script, both $* and $@ produce the same output when unquoted. However, when quoted, they behave differently. This subtle difference can have significant implications depending on your script’s requirements.
Individual Positional Parameters: $1, $2, etc.
If you need to access individual arguments, you can use $1, $2, etc., where the number corresponds to the argument’s position. Here’s an example:
#!/bin/bash
echo First Argument: $1
echo Second Argument: $2
# Run the script with arguments
./script.sh Apple Banana
# Output:
# First Argument: Apple
# Second Argument: Banana
In this script, $1 corresponds to the first argument (‘Apple’), and $2 corresponds to the second argument (‘Banana’). This approach gives you direct access to individual arguments, which can be useful in certain scenarios.
Choosing between $@, $*, $1, $2, etc., depends on your specific needs. If you need to handle all arguments as a group, $@ or $* might be the right choice. If you need to access individual arguments, $1, $2, etc., would be more appropriate. Understanding these options and their implications is key to effective bash scripting.
Troubleshooting $@: Common Pitfalls and Solutions
While $@ is a versatile tool in bash scripting, it’s not without its quirks. Understanding these potential pitfalls can help you avoid common errors and optimize your scripts.
Unquoted $@
One common mistake is using $@ unquoted in a situation where word splitting can occur. This can lead to unexpected behavior. Here’s an example:
#!/bin/bash
for arg in $@
do
echo Argument: $arg
done
# Run the script with arguments
./script.sh "Apple Pie" "Banana Split"
# Output:
# Argument: Apple
# Argument: Pie
# Argument: Banana
# Argument: Split
In this script, we expected ‘Apple Pie’ and ‘Banana Split’ to be treated as separate arguments. However, because $@ is unquoted, word splitting occurs at the spaces, treating ‘Apple’, ‘Pie’, ‘Banana’, and ‘Split’ as separate arguments.
The solution is to quote $@. This will preserve the original arguments, even if they contain spaces:
#!/bin/bash
for arg in "$@"
do
echo Argument: $arg
done
# Run the script with arguments
./script.sh "Apple Pie" "Banana Split"
# Output:
# Argument: Apple Pie
# Argument: Banana Split
With “$@”, the script treats ‘Apple Pie’ and ‘Banana Split’ as separate arguments, as intended.
Best Practices and Optimization
When using $@, it’s generally a good idea to always quote it to prevent word splitting. Additionally, remember that $@ doesn’t keep track of argument order. If the order of arguments is important, consider using $1, $2, etc., instead.
By understanding these considerations and best practices, you can use $@ more effectively in your bash scripts.
Bash Scripting: Positional Parameters and Command-Line Arguments
To truly grasp the power of $@ in bash, it’s important to understand some fundamental concepts: positional parameters and command-line arguments.
Positional Parameters in Bash
In bash scripting, positional parameters are a set of special variables ($0, $1, $2, …, $9) that hold the command-line arguments provided to the script. The variable $0 holds the name of the script itself, $1 holds the first argument, $2 the second, and so on.
Here’s a simple example of positional parameters in action:
#!/bin/bash
echo "Script Name: $0"
echo "First Argument: $1"
echo "Second Argument: $2"
# Run the script with arguments
./script.sh Apple Banana
# Output:
# Script Name: ./script.sh
# First Argument: Apple
# Second Argument: Banana
In this script, $0 holds the script’s name (‘./script.sh’), $1 holds the first argument (‘Apple’), and $2 holds the second argument (‘Banana’).
Command-Line Arguments in Bash
Command-line arguments are the values provided to a script when it’s executed. They allow you to customize the script’s behavior without modifying the script itself. In the above example, ‘Apple’ and ‘Banana’ are command-line arguments.
Beyond Positional Parameters: $@ and Other Special Variables
While positional parameters are great for handling a few arguments, they can be cumbersome when dealing with many arguments. This is where $@ and other special variables like $* come in.
As we’ve seen, $@ holds all the positional parameters (except the script’s name). This makes it a powerful tool for handling multiple command-line arguments in a flexible and efficient manner.
Understanding these fundamentals will give you a solid foundation to master $@ and other advanced bash scripting techniques.
$@ in Larger Scripts: Enhancing Your Bash Projects
While we’ve explored the basics and some advanced uses of $@, it’s worth noting that this special variable can play a significant role in larger scripts or projects. The use of $@ can greatly simplify the handling of command-line arguments, making your scripts more flexible and robust.
Related Commands and Functions
In real-world bash scripts, $@ often doesn’t work alone. It’s frequently accompanied by other commands and functions to create more complex and powerful scripts. For instance, the ‘getopts’ function is commonly used with $@ to parse command-line options.
Here’s a brief example:
#!/bin/bash
while getopts "a:b:" opt; do
case $opt in
a) a_arg="$OPTARG" ;;
b) b_arg="$OPTARG" ;;
esac
done
shift $((OPTIND -1))
# Remaining arguments are available in $@
for arg in "$@"; do
echo Argument: $arg
done
# Run the script with arguments
./script.sh -a Apple -b Banana Cherry Date
# Output:
# Argument: Cherry
# Argument: Date
In this script, we use ‘getopts’ to parse ‘-a’ and ‘-b’ options from the command-line arguments. After parsing the options, we use ‘shift’ to remove them, leaving only the remaining arguments in $@.
Further Resources for Bash Scripting Mastery
To deepen your understanding of $@ and bash scripting in general, consider exploring these additional resources:
- GNU Bash Reference Manual: A comprehensive guide to bash scripting from the creators of bash.
- Advanced Bash-Scripting Guide: An in-depth exploration of bash scripting, including many advanced topics.
- Baeldung’s Guide on Using Command Line Arguments in Bash Script: This guide explains how to use command line arguments in a Bash script.
Wrapping Up: Commanding Bash with $@
In this comprehensive guide, we’ve demystified the $@ variable in bash, a powerful tool that holds all the positional parameters of a script. We’ve journeyed from understanding its basic use to mastering more advanced techniques, providing you with a solid foundation to handle multiple command-line arguments in your bash scripts.
We started with the basics, showing how $@ works and how to use it in a simple bash script. We then delved into more advanced uses, demonstrating how $@ can be used with loops and the shift command to create more complex and powerful scripts.
We also explored alternative approaches, such as using $*, $1, $2, etc., giving you a broader understanding of the tools available for handling command-line arguments in bash. Along the way, we tackled common pitfalls and provided solutions to help you avoid errors and optimize your scripts.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Direct Access | Handling Spaces |
---|---|---|---|
$@ | High | No | Yes |
$* | High | No | No |
$1, $2, etc. | Low | Yes | Yes |
Whether you’re just starting out with bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of $@ and its capabilities.
With its flexibility and ease of use, $@ is a powerful tool for handling multiple command-line arguments in bash. Now, you’re well equipped to harness its power in your scripts. Happy scripting!