exec Linux Command | Shell Scripting Guide
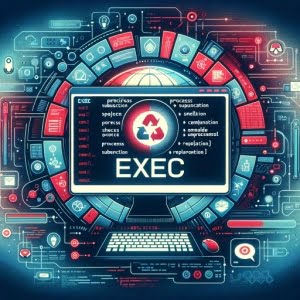
Are you finding it challenging to understand the ‘exec’ command in Linux? You’re not alone. Many users find themselves puzzled when it comes to handling this powerful command, but we’re here to help. Think of the ‘exec’ command in Linux as a master key – it can open up new possibilities in your shell scripting, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the ‘exec’ command in Linux, from its basic usage to advanced techniques. We’ll cover everything from the fundamentals of the ‘exec’ command, its advantages, potential pitfalls, to more complex uses and alternative approaches.
Let’s get started and master the ‘exec’ command in Linux!
TL;DR: How Do I Use the ‘exec’ Command in Linux?
The
'exec'
command in Linux is used to replace the current shell with a new command. It’s a powerful tool that can significantly enhance your shell scripting capabilities.
Here’s a simple example:
exec ls
# Output:
# [Expected output from the 'ls' command]
In this example, we use the ‘exec’ command followed by ‘ls’. This replaces the current shell with the ‘ls’ command, which lists the contents of the current directory.
This is just a basic way to use the ‘exec’ command in Linux, but there’s so much more to it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the ‘exec’ Command: Basic Use
- Unleashing the Power of ‘exec’: Advanced Use
- Exploring Alternatives to ‘exec’ Command
- Troubleshooting ‘exec’ Command: Common Issues and Solutions
- Understanding Shell Scripting and Process Management
- The ‘exec’ Command in Larger Scripts and Projects
- Wrapping Up: Linux ‘exec’ How-to Guide
Understanding the ‘exec’ Command: Basic Use
The ‘exec’ command in Linux is a built-in utility of the Bash shell. It is used to replace the current shell process with a new process. This means that when you use the ‘exec’ command, the current shell process terminates, and a new process starts.
Let’s see it in action with a simple example:
exec echo 'Hello, World!'
# Output:
# Hello, World!
In this case, we’ve used the ‘exec’ command followed by the ‘echo’ command. The ‘echo’ command simply prints its argument, in this case, ‘Hello, World!’. This command replaces the current shell with the ‘echo’ command.
One advantage of using the ‘exec’ command is that it allows you to manage your system’s resources more efficiently. Since the ‘exec’ command replaces the current shell instead of creating a new one, it uses less memory and processing power.
However, a potential pitfall is that once the ‘exec’ command is executed, the current shell process terminates. This means that any command that follows the ‘exec’ command in a script will not be executed unless the exec command fails. This is something you should keep in mind while writing your scripts.
So, the ‘exec’ command in Linux is a powerful tool for shell scripting, but it requires careful handling to avoid unexpected results.
Unleashing the Power of ‘exec’: Advanced Use
As you grow more comfortable with the ‘exec’ command, you’ll discover that it’s not just a simple command—it’s a powerful tool that can significantly enhance your shell scripting capabilities. The ‘exec’ command in Linux has a range of advanced uses, from redirecting input and output to executing multiple commands.
Before we dive into the advanced uses of ‘exec’, let’s familiarize ourselves with some of the command-line arguments or flags that can modify the behavior of the ‘exec’ command. Here’s a table with some of the most commonly used ‘exec’ arguments.
Argument | Description | Example |
---|---|---|
>filename | Redirects standard output to a file. | exec > output.txt |
<filename | Redirects standard input from a file. | exec filename | Redirects standard error to a file. | exec 2> error.txt |
&>filename | Redirects both standard output and error to a file. | exec &> output_and_error.txt |
command [arg1 arg2 ...] | Replaces the shell with the specified command. | `exec echo ‘Hello, World!’ |
Now that we have a basic understanding of ‘exec’ command line arguments, let’s dive deeper into the advanced use of ‘exec’.
Redirecting Output
One of the powerful features of ‘exec’ is its ability to redirect output. Here’s an example:
exec > output.txt
echo 'Hello, World!'
# Output in 'output.txt':
# Hello, World!
In this example, we first redirect the standard output to a file named ‘output.txt’. Then, when we execute the ‘echo’ command, its output is written to ‘output.txt’.
Redirecting Input
Similarly, ‘exec’ can also redirect input from a file. Here’s how it works:
exec < input.txt
cat
# Output:
# [Contents of 'input.txt']
In this case, we first redirect the standard input from a file named ‘input.txt’. Then, when we execute the ‘cat’ command, it reads from ‘input.txt’.
Executing Multiple Commands
The ‘exec’ command can also replace the shell with a new command that executes multiple commands. Here’s an example:
exec bash -c 'echo Hello, World!; echo Goodbye, World!'
# Output:
# Hello, World!
# Goodbye, World!
In this example, we use ‘exec’ to replace the shell with a new bash shell that executes two ‘echo’ commands.
So, as you can see, the ‘exec’ command in Linux is a powerful tool for advanced shell scripting. With it, you can manage input and output, execute multiple commands, and more.
Exploring Alternatives to ‘exec’ Command
While the ‘exec’ command is a powerful tool in shell scripting, there are other commands that you can use instead. These alternatives, such as ‘bash’, ‘sh’, or ‘source’, can provide different benefits and have their own unique considerations.
The ‘bash’ Command
The ‘bash’ command is used to invoke the Bash shell. It can also be used to execute commands in a new shell.
bash -c 'echo Hello, World!'
# Output:
# Hello, World!
In this example, we use ‘bash’ to start a new shell and execute the ‘echo’ command. Unlike ‘exec’, ‘bash’ does not replace the current shell, but starts a new one.
The ‘sh’ Command
Similar to ‘bash’, the ‘sh’ command is used to invoke the Bourne shell. It can also be used to execute commands in a new shell.
sh -c 'echo Hello, World!'
# Output:
# Hello, World!
In this example, we use ‘sh’ to start a new shell and execute the ‘echo’ command. Again, ‘sh’ does not replace the current shell but starts a new one.
The ‘source’ Command
The ‘source’ command is used to read and execute commands from a file in the current shell. It’s different from ‘exec’ in that it does not replace the current shell.
echo 'echo Hello, World!' > test.sh
source test.sh
# Output:
# Hello, World!
In this example, we first create a script file named ‘test.sh’ with the ‘echo’ command. Then, we use the ‘source’ command to execute the commands in ‘test.sh’ in the current shell.
Each of these alternatives has its own benefits and drawbacks. The choice between ‘exec’ and its alternatives depends on your specific needs and the context. Understanding these different commands and how they work can help you make an informed decision and write more effective shell scripts.
Troubleshooting ‘exec’ Command: Common Issues and Solutions
While the ‘exec’ command is a powerful tool in shell scripting, it can sometimes lead to unexpected results or errors. Understanding these potential pitfalls can help you write more effective scripts. Let’s explore some common issues and their solutions.
Running Commands After ‘exec’
One common issue is trying to run commands after ‘exec’ in a script. As ‘exec’ replaces the current shell, any commands after it will not be executed unless the ‘exec’ command fails. Here’s an example:
exec echo 'Hello, World!'
echo 'This will not be printed'
# Output:
# Hello, World!
In this example, the second ‘echo’ command is not executed because the ‘exec’ command replaces the current shell.
Redirecting Without a Filename
Another common issue is trying to redirect output or input without specifying a filename. This will result in an error. Here’s an example:
exec >
# Output:
# bash: syntax error near unexpected token 'newline'
In this example, we try to redirect the output using ‘exec’, but we don’t specify a filename. This results in a syntax error.
Using ‘exec’ Without a Command
Finally, using ‘exec’ without a command will not result in an error, but it will not do anything either. Here’s an example:
exec
# Output:
# [No output]
In this example, we use ‘exec’ without a command. This doesn’t do anything and doesn’t produce any output.
Understanding these common errors and obstacles can help you avoid them and use the ‘exec’ command more effectively in your shell scripts.
Understanding Shell Scripting and Process Management
To fully grasp the power of the ‘exec’ command in Linux, it’s essential to understand the fundamentals of shell scripting and process management in Linux.
What is Shell Scripting?
Shell scripting is a way of writing command line instructions in a script file to automate tasks. These scripts are executed by a shell, which is a program that interprets these commands. In Linux, the most common shell is the Bash shell, but there are others like the Korn shell, C shell, and more.
What is Process Management?
In Linux, every command you run is a process. Process management involves starting, stopping, and monitoring these processes. Each process has a unique process ID (PID), and the system uses this PID to manage the process.
The Role of ‘exec’ in Process Management
The ‘exec’ command plays a unique role in process management. Unlike other commands that create a new process when run, ‘exec’ replaces the current shell process with a new process.
Here’s an example to illustrate this:
echo $$
exec bash
echo $$
# Output:
# 12345
# 12345
In this example, we first print the PID of the current shell using ‘echo $$’. Then, we use ‘exec’ to replace the current shell with a new Bash shell. Finally, we print the PID of the new shell.
You’ll notice that the PID remains the same before and after the ‘exec’ command. This is because ‘exec’ replaces the current shell process instead of creating a new one.
Understanding these fundamentals can help you better understand the ‘exec’ command and use it more effectively in your shell scripts.
The ‘exec’ Command in Larger Scripts and Projects
The ‘exec’ command in Linux isn’t limited to simple, one-off tasks. It’s a powerful tool that can be used in larger scripts and projects to manage processes more efficiently.
Complementary Commands to ‘exec’
There are several commands that often accompany the ‘exec’ command in typical use cases. For example, the ‘ps’ command can be used to view the status of processes, and the ‘kill’ command can be used to terminate processes.
Here’s an example where we use ‘exec’ and ‘ps’ together:
exec bash &
ps
# Output:
# PID TTY TIME CMD
# 12345 pts/0 00:00:00 bash
# 12346 pts/0 00:00:00 ps
In this example, we first use ‘exec’ to start a new Bash shell in the background. Then, we use ‘ps’ to display the status of current processes.
Applying ‘exec’ in Larger Scripts
In larger scripts, the ‘exec’ command can be used to manage input and output, execute complex commands, and more. Here’s an example of a script that uses ‘exec’ to manage output:
exec > output.txt
for i in {1..5}; do
echo "Loop iteration: $i"
done
# Output in 'output.txt':
# Loop iteration: 1
# Loop iteration: 2
# Loop iteration: 3
# Loop iteration: 4
# Loop iteration: 5
In this script, we first redirect the standard output to a file named ‘output.txt’. Then, we run a loop that echoes a message five times. The output of the loop is written to ‘output.txt’.
Further Resources for Mastering the ‘exec’ Command
If you’re interested in learning more about the ‘exec’ command and related topics, here are a few resources that can help:
- GNU Bash Manual: This is the official manual for the Bash shell, which includes detailed information about the ‘exec’ command and other shell built-ins.
Linux Command Library: This online library provides a wealth of information about Linux commands, including ‘exec’.
Advanced Bash-Scripting Guide: This guide provides in-depth information about shell scripting in Bash, including advanced topics like process management and input/output redirection.
Wrapping Up: Linux ‘exec’ How-to Guide
In this comprehensive guide, we’ve delved into the world of the ‘exec’ command in Linux, a powerful tool for shell scripting and process management. We’ve explored its basic usage, advanced techniques, common issues and their solutions, and alternative approaches.
We started with the basics, understanding how the ‘exec’ command works and how to use it in simple scenarios. We then delved into more advanced usage, including redirecting input and output and executing multiple commands.
Along the way, we tackled common challenges you might encounter when using the ‘exec’ command, such as trying to run commands after ‘exec’ in a script, redirecting without a filename, and using ‘exec’ without a command, providing you with solutions and considerations for each issue.
We also looked at alternative approaches to the ‘exec’ command, comparing ‘exec’ with other commands like ‘bash’, ‘sh’, and ‘source’. Here’s a quick comparison of these methods:
Method | Replaces Current Shell | Executes Multiple Commands |
---|---|---|
‘exec’ | Yes | Yes |
‘bash’ | No | Yes |
‘sh’ | No | Yes |
‘source’ | No | No |
Whether you’re just starting out with the ‘exec’ command or you’re looking to level up your shell scripting skills, we hope this guide has given you a deeper understanding of the ‘exec’ command and its capabilities.
With its ability to replace the current shell with a new process, the ‘exec’ command is a powerful tool for efficient process management in Linux. Happy scripting!