‘-f’ Flag in Bash Explained | Linux Shell Scripting Guide
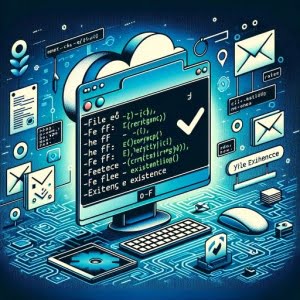
Ever felt puzzled about the ‘-f’ option in bash? You’re not alone. Many users find themselves scratching their heads when it comes to understanding this bash option. Think of the ‘-f’ option as a detective in the world of bash – it’s used to check if a file exists. It’s a simple command, but it holds a lot of power when it comes to scripting and automation.
In this guide, we’ll walk you through the usage of -f in bash, from basic to advanced techniques. We’ll cover everything from a simple file existence check to more complex scenarios, as well as alternative approaches. We’ll also discuss common issues and their solutions.
So, let’s dive in and start mastering the ‘-f’ option in bash!
TL;DR: What Does -f Do in Bash?
In bash,
-f
is an option used to check if a file exists. It is commonly used with anif
statement, such asif [ -f /tmp/test.txt ]
. It’s a simple yet powerful tool that can be used in various scripting and automation tasks.
Here’s a simple example:
if [ -f /tmp/test.txt ]
then
echo 'File exists.'
fi
# Output:
# If /tmp/test.txt exists, it will print 'File exists.'
In this example, we use the -f option to check if the file ‘/tmp/test.txt’ exists. If the file exists, it prints ‘File exists.’ to the console.
This is just a basic use of -f in bash, but there’s much more to learn about this option. Continue reading for more detailed usage and advanced scenarios.
Table of Contents
- Getting Started with -f in Bash
- Diving Deeper: Intermediate Uses of -f in Bash
- Exploring Alternatives: Other Ways to Check File Existence in Bash
- Navigating Pitfalls: Troubleshooting -f in Bash
- Bash and Command Options: The Foundation of -f
- Applying -f in Larger Scripts and Projects
- Wrapping Up: Mastering -f in Bash
Getting Started with -f in Bash
Now that we understand what -f does in bash, let’s delve into its basic usage. This is crucial for beginners who are just starting to explore the world of bash scripting.
The -f option in bash is used in conditional expressions to check if a specific file exists. Here’s a simple example:
filename='/path/to/your/file'
if [ -f $filename ]
then
echo 'Your file exists.'
else
echo 'Your file does not exist.'
fi
# Output:
# Depending on if /path/to/your/file exists, it will print 'Your file exists.' or 'Your file does not exist.'
In this example, we first define a variable filename
with the path to our target file. We then use the -f option in an if statement to check if the file at the path stored in filename
exists. Depending on the existence of the file, it will print a corresponding message to the console.
One common pitfall for beginners is forgetting to quote the variable in the test. If the filename contains spaces and you don’t quote it, bash will treat each word as a separate argument and the test will fail. So always remember to quote your variables like so: "$filename"
.
Pitfall: Forgetting to Quote Variables
Let’s see what happens when we forget to quote a filename that contains spaces:
filename='/path/to/your file with spaces'
if [ -f $filename ]
then
echo 'Your file exists.'
else
echo 'Your file does not exist.'
fi
# Output:
# bash: [: /path/to/your: binary operator expected
As you can see, bash throws an error because it treats each word as a separate argument. To avoid this, always quote your variables when dealing with filenames.
Diving Deeper: Intermediate Uses of -f in Bash
As you become more familiar with bash, you’ll find that the -f option can be combined with other bash commands and options for more complex scenarios. Let’s explore some of these advanced uses of -f.
Combining -f with Other Bash Commands
An interesting use case is combining -f with the &&
operator to execute a command only if a file exists. Here’s how you can do it:
filename='/path/to/your/file'
[ -f "$filename" ] && echo 'Your file exists.'
# Output:
# If /path/to/your/file exists, it will print 'Your file exists.'
In this example, we use the &&
operator to execute the echo
command only if the preceding condition [ -f "$filename" ]
is true. This is a neat way to perform an action contingent on the existence of a file.
Using -f with Bash Loops
Another powerful use of -f is within bash loops. For instance, you can iterate over a directory and perform actions only on files, ignoring directories. Here’s an example:
for item in /path/to/your/directory/*
do
if [ -f "$item" ]
then
echo "$item is a file."
else
echo "$item is not a file."
fi
done
# Output:
# Prints whether each item in /path/to/your/directory is a file or not.
In this script, we use a for loop to iterate over all items in a directory. For each item, we use -f to check if it’s a file. Depending on the result, we print a corresponding message.
These are just a few examples of the many ways you can use -f in bash. As you gain more experience, you’ll discover even more possibilities.
Exploring Alternatives: Other Ways to Check File Existence in Bash
While -f is a powerful tool for checking file existence in bash, it’s not the only method available. Let’s explore some alternatives, including the -e option, and discuss when you might want to use them.
Using -e to Check File or Directory Existence
The -e option is similar to -f, but it checks whether a file or directory exists, regardless of its type. Here’s how you can use -e:
filename='/path/to/your/file_or_directory'
if [ -e "$filename" ]
then
echo 'Your file or directory exists.'
else
echo 'Your file or directory does not exist.'
fi
# Output:
# Depending on if /path/to/your/file_or_directory exists, it will print 'Your file or directory exists.' or 'Your file or directory does not exist.'
In this script, we use -e instead of -f to check the existence of an item, be it a file or a directory. This can be useful when you don’t care about the type of the item, just its existence.
Decision-Making Considerations
Choosing between -f and -e (or other options) depends on your specific needs. If you need to check specifically for a file, use -f. If you just need to check if something (a file or directory) exists at a particular path, use -e.
Remember, bash provides a variety of options for different scenarios, so choose the one that best suits your needs. Understanding these alternatives allows you to write more flexible and robust bash scripts.
While -f is a valuable tool in bash, it’s not without its potential pitfalls. Understanding common errors and knowing how to troubleshoot them is crucial in mastering the use of -f. Let’s explore some of these issues and their solutions.
Unquoted Variables
As we discussed earlier, forgetting to quote a variable when checking for file existence can lead to errors, especially if your filename contains spaces. Let’s see an example:
filename='file with spaces.txt'
if [ -f $filename ]
then
echo 'File exists.'
fi
# Output:
# bash: [: too many arguments
In this example, bash throws an error because it treats each word as a separate argument. To avoid this, always quote your variables when dealing with filenames.
File Doesn’t Exist
Another common issue is trying to perform an operation on a file that doesn’t exist. For instance, trying to read from a non-existent file will result in an error. Let’s see an example:
filename='nonexistent_file.txt'
if [ -f "$filename" ]
then
cat "$filename"
else
echo 'File does not exist.'
fi
# Output:
# File does not exist.
In this example, we attempt to read from a file that doesn’t exist. Because we properly checked for the file’s existence with -f before trying to read it, we can handle this scenario gracefully and print a helpful error message.
Best Practices
Some best practices when using -f include:
- Always quote your variables to avoid issues with filenames that contain spaces or special characters.
- Always check for a file’s existence using -f before attempting to perform operations on it.
- Remember that -f only checks for files, not directories. If you need to check for either, consider using -e instead.
Bash and Command Options: The Foundation of -f
To fully understand the use of -f, we need to delve a bit into the fundamentals of bash and its command options. Bash, or the Bourne Again SHell, is a command interpreter and a shell scripting language. It provides a wide range of command options, like -f, which add versatility to your scripts.
Understanding Bash Command Options
Command options in bash are used to modify the behavior of commands. They are usually prefixed by a hyphen (-) and can be combined in a single command for more complex operations. For instance, in the command ls -l -a
, -l
and -a
are options modifying the behavior of the ls
command.
The -f option is a test operator used within a conditional expression to check if a file exists and is a regular file. It’s one of many file test operators available in bash, each serving a unique purpose. Here’s an example of how -f is used in a bash command:
filename='your_file.txt'
if [ -f "$filename" ]
then
echo 'Your file is a regular file.'
fi
# Output:
# If your_file.txt exists and is a regular file, it will print 'Your file is a regular file.'
In this example, we use the -f option to check if ‘your_file.txt’ is a regular file. If it is, we print a message to the console.
Understanding the basics of bash and command options helps you better grasp the use of -f and its role in bash scripting. It’s the foundation that allows you to write scripts that can interact with the file system, making tasks like automation and system administration significantly easier.
Applying -f in Larger Scripts and Projects
Mastering -f in bash is not just about understanding how it works in isolation. It’s also about knowing how to apply it effectively in larger scripts or projects. Let’s discuss some of the ways -f can be used in more complex scenarios.
Integrating -f in Automation Scripts
In automation scripts, -f can be a crucial component. For instance, before manipulating a file (like moving, copying, or deleting), you might want to ensure it exists to prevent errors. Here’s an example:
filename='your_file.txt'
if [ -f "$filename" ]
then
mv "$filename" /path/to/new/location
else
echo 'File does not exist, cannot move.'
fi
# Output:
# If your_file.txt exists, it will be moved to /path/to/new/location. If not, it will print 'File does not exist, cannot move.'
In this script, we use -f to check if ‘your_file.txt’ exists before trying to move it. This prevents the mv
command from throwing an error if the file doesn’t exist.
Related Bash Commands
Often, -f is used in conjunction with other bash commands. Some related commands that often accompany -f include if
, else
, then
, fi
, &&
, and ||
. These commands can be used to create more complex conditional expressions and control structures in your scripts.
Further Resources for Bash Scripting Mastery
To dive deeper into bash scripting and the use of -f, here are some additional resources:
- GNU Bash Manual: The official manual for bash, providing comprehensive documentation on all its features.
- Bash Academy: An interactive platform for learning bash scripting from scratch.
- Bash Scripting Guide: An in-depth guide to bash scripting, covering basic to advanced topics.
Wrapping Up: Mastering -f in Bash
In this comprehensive guide, we’ve delved into the usage of -f in bash, a handy option for checking file existence. From the basic usage to more advanced techniques, we’ve explored the many ways -f can be used in bash scripting.
We began with the basics, explaining what -f does in bash and providing a simple example for its usage. We then progressed to more complex scenarios, demonstrating how -f can be combined with other bash commands and used within loops. We also explored alternative approaches to checking file existence, such as using the -e option.
Along the way, we tackled common pitfalls and troubleshooting issues when using -f. From unquoted variables to nonexistent files, we’ve covered the common challenges you might face and provided solutions to handle them. We also delved into the fundamentals of bash and its command options, providing a deeper understanding of the -f option’s role in bash scripting.
Here’s a quick comparison of the methods we’ve discussed:
Method | Specificity | Use Case |
---|---|---|
-f | Checks for regular files | When you need to ensure a file exists |
-e | Checks for files or directories | When you need to check if anything exists at a particular path |
Whether you’re just starting out with bash scripting or you’re looking to improve your skills, we hope this guide has helped you understand and master the use of -f in bash.
The ability to check file existence is a fundamental part of bash scripting, and mastering -f equips you with a critical tool for your scripting toolkit. Happy scripting!