GCC Command Guide: How-To Compile C Code in Linux
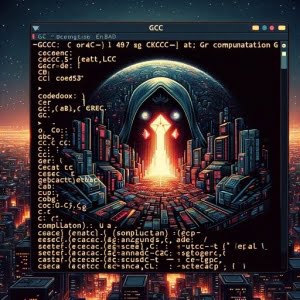
Are you finding it challenging to navigate the ‘gcc’ command in Linux? You’re not alone. Many developers find themselves in a maze when it comes to using the ‘gcc’ command, but we’re here to help.
Think of the ‘gcc’ command as a skilled craftsman in the Linux world – it’s a powerful tool that can help you build and compile your C and C++ programs, turning your code into executable files.
In this guide, we’ll walk you through the process of using the gcc command in Linux, from the basics to more advanced techniques. We’ll cover everything from compiling simple C programs, using different flags and options, to troubleshooting common issues and exploring alternative approaches.
Let’s get started and master the gcc command in Linux!
TL;DR: How Do I Use the GCC Command in Linux?
The
gcc
command in Linux is used to compile C and C++ programs. A simple way to use it is:gcc filename.c -o outputfile
. This command compiles the ‘filename.c’ file and outputs an executable named ‘outputfile’.
Here’s a simple example:
gcc hello.c -o hello
# Output:
# An executable file named 'hello' is created.
In this example, we use the gcc command to compile the ‘hello.c’ file. The -o
option is used to specify the name of the output file. In this case, an executable named ‘hello’ is created.
This is just a basic way to use the gcc command in Linux. There’s much more to learn about this powerful tool, including its various options and flags, troubleshooting techniques, and alternative approaches. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with GCC Linux Command
The gcc command is an integral part of Linux, especially for developers working with C and C++ programs. Let’s break down its basic usage.
Compiling a Simple C Program
Imagine you have a simple C program that prints ‘Hello, World!’. The code looks like this:
#include <stdio.h>
int main() {
printf("Hello, World!
");
return 0;
}
To compile this program using the gcc command, you would use the following command:
gcc hello_world.c -o hello_world
# Output:
# Creates an executable file named 'hello_world'
In this command, ‘hello_world.c’ is the source file that contains your C code. The -o
option followed by ‘hello_world’ specifies the name of the output file. This will create an executable file named ‘hello_world’.
Advantages and Potential Pitfalls
The gcc command is a powerful tool with many advantages. It’s widely used, well-documented, and capable of compiling both C and C++ programs. It also provides a variety of options to control the compilation process.
However, beginners might face some pitfalls. For instance, forgetting to include the -o
option will cause gcc to output the compiled file as ‘a.out’ by default. Also, not understanding error messages can lead to confusion. As we progress, we’ll explore how to handle such issues.
Advance Use of the GCC Command
As you become more comfortable with the basic GCC command, you’ll find that its true power lies in its advanced features. GCC’s flexibility allows it to handle more complex tasks, such as using different flags and options to control the compilation process. Let’s explore some of these advanced uses.
Before we dive deeper into the advanced usage of GCC, let’s familiarize ourselves with some of the command-line arguments or flags that can modify the behavior of the GCC command. Here’s a table with some of the most commonly used GCC arguments.
Argument | Description | Example |
---|---|---|
-Wall | Enables all compiler’s warning messages. | gcc -Wall hello.c -o hello |
-Werror | Turns warnings into errors. | gcc -Werror hello.c -o hello |
-o | Specifies the output file. | gcc hello.c -o hello |
-g | Generates debug information for use with GDB. | gcc -g hello.c -o hello |
-O | Enables optimization. | gcc -O hello.c -o hello |
-std | Specifies the version of the C language standard. | gcc -std=c99 hello.c -o hello |
-c | Compiles or assembles the source files, but does not link. | gcc -c hello.c |
-I | Adds include directory of header files. | gcc -I /path/to/include hello.c -o hello |
-L | Adds directory of library files. | gcc -L /path/to/library hello.c -o hello |
-l | Links with the library. | gcc hello.c -lmylib -o hello |
-D | Defines a preprocessor macro. | gcc -DDEBUG hello.c -o hello |
Now that we have a basic understanding of GCC command line arguments, let’s dive deeper into the advanced use of GCC.
Using the -Wall
and -Werror
Flags
The -Wall
flag in GCC enables all the warning messages. This is extremely useful in catching potential issues in your code. Here’s an example:
gcc -Wall hello.c -o hello
This command will compile the ‘hello.c’ file and output an executable named ‘hello’, showing all warning messages.
The -Werror
flag turns all warnings into errors. This means that GCC will refuse to compile your code if there are any warnings. This can be helpful in making sure your code is as clean as possible.
gcc -Werror hello.c -o hello
# Output:
# If there are warnings, GCC will show error messages and fail to compile 'hello.c'.
Using the -g
Flag for Debugging
When writing complex programs, debugging is an essential part of the process. The -g
flag allows you to generate debug information for use with GDB, the GNU Debugger.
gcc -g hello.c -o hello
# Output:
# Creates an executable file named 'hello' with debug information.
In this command, the -g
flag tells GCC to include extra information, such as the locations of all the variable declarations, which can be used by a debugger.
Using the -O
Flag for Optimization
GCC provides several levels of source code optimization. These optimization levels are not specific to any language. The -O
flag enables optimization.
gcc -O hello.c -o hello
# Output:
# Creates an optimized executable file named 'hello'.
In this command, the -O
flag tells GCC to optimize the code for performance. This can make your code run faster, but it may take longer to compile.
By understanding and using these advanced features of the GCC command, you can greatly enhance your coding and debugging efficiency in Linux.
Exploring Alternatives to GCC Linux Command
While GCC is an efficient and widely used tool for compiling C and C++ programs, it’s not the only option. There are other tools available that can be used as alternatives to the GCC command, such as Clang. Let’s take a closer look at Clang and how it compares to GCC.
Introduction to Clang
Clang is a compiler front end for the programming languages C, C++, Objective-C, Objective-C++, OpenMP, OpenCL, and CUDA. It uses LLVM as its back end and has been part of the LLVM release cycle since LLVM 2.6.
It can be used as a drop-in replacement for GCC and offers several advantages, such as improved error reporting.
Here’s an example of compiling a C program using Clang:
clang hello.c -o hello
# Output:
# Creates an executable file named 'hello'.
In this command, we use Clang to compile the ‘hello.c’ file. The -o
option is used to specify the name of the output file. In this case, an executable named ‘hello’ is created.
Benefits and Drawbacks of Clang
Clang provides several benefits over GCC, including faster compilation and improved error and warning messages. It’s also more permissive about mixing code written in different languages.
However, Clang also has some drawbacks. For example, it may not fully support all of the GCC extensions. This means that some code that compiles with GCC might not compile with Clang.
Making the Right Decision
When choosing between GCC and Clang, consider your specific needs and circumstances. If you’re working on a large project that uses many GCC extensions, GCC might be the better choice. On the other hand, if you’re starting a new project and want faster compilation and better error messages, Clang might be the way to go.
As with any tool, using the GCC command in Linux can sometimes result in errors or issues. These can arise due to a variety of reasons, such as syntax errors in your code, incorrect use of GCC options, or missing files. Let’s explore some of the common issues you might encounter when using the GCC command and how to resolve them.
Dealing with Syntax Errors
One common issue when using GCC is encountering syntax errors in your C or C++ code. Let’s consider a simple example where we have a missing semicolon in our code:
#include <stdio.h>
int main() {
printf("Hello, World!")
return 0;
}
When we try to compile this code with GCC, we get an error:
gcc hello.c -o hello
# Output:
# hello.c: In function ‘main’:
# hello.c:4:5: error: expected ‘;’ before ‘return’
The error message tells us that GCC expected a semicolon before the ‘return’ statement. By adding the missing semicolon, we can fix the syntax error.
Incorrect Use of GCC Options
Another common issue is the incorrect use of GCC options. For example, if you use the -o
option but forget to specify the output file, you’ll get an error.
gcc hello.c -o
# Output:
# gcc: fatal error: no input files
# compilation terminated.
The error message tells us that no input files were specified. To resolve this issue, we need to specify the output file after the -o
option.
Missing Files
If you try to compile a file that doesn’t exist, GCC will give you an error.
gcc missing_file.c -o missing_file
# Output:
# gcc: error: missing_file.c: No such file or directory
# gcc: fatal error: no input files
# compilation terminated.
The error message tells us that ‘missing_file.c’ does not exist. To resolve this issue, make sure that the file you’re trying to compile actually exists and that you’ve spelled its name correctly.
By understanding these common issues and their solutions, you can troubleshoot problems more effectively when using the GCC command in Linux.
Real-World Relevance of the GCC Linux Command
The GCC command, also known as the GNU Compiler Collection, is a key component of the Linux ecosystem. It’s not just a simple command but a full-fledged collection of compilers for various programming languages, with C and C++ being the most commonly used.
A Glimpse into GCC’s History
GCC was first released in 1987 by Richard Stallman, the founder of the GNU Project. The main goal of GCC was to provide a free and open-source compiler that supports multiple languages. Over the years, GCC has evolved and matured, becoming an indispensable tool for developers working in a Linux environment.
The Role of GCC in the Linux Ecosystem
GCC plays a crucial role in the Linux ecosystem. It is the standard compiler for most Unix-like operating systems, including Linux. GCC is used to turn source code written in C, C++, and other supported languages into executable programs.
Understanding the Compilation Process
The process of compiling a C or C++ program using GCC can be broken down into four stages: preprocessing, compilation, assembly, and linking.
- Preprocessing: In this stage, the preprocessor takes your source code and processes directives, such as
#include
and#define
. The output of this stage is a single intermediate file that is passed to the next stage.
gcc -E hello.c -o hello.i
# Output:
# Creates an intermediate preprocessed file named 'hello.i'.
- Compilation: The compiler takes the preprocessed code and translates it into assembly code specific to your machine’s architecture.
gcc -S hello.i -o hello.s
# Output:
# Creates an assembly code file named 'hello.s'.
- Assembly: The assembler takes the assembly code and translates it into machine code, resulting in an object file.
gcc -c hello.s -o hello.o
# Output:
# Creates an object file named 'hello.o'.
- Linking: The linker takes one or more object files and combines them into a single executable program.
gcc hello.o -o hello
# Output:
# Creates an executable file named 'hello'.
Each stage of the compilation process plays a critical role in transforming your source code into an executable program. By understanding these stages, you can gain a deeper insight into how the GCC command works under the hood.
Further Research: GCC in Linux
The GCC command, while simple at its core, is an incredibly powerful tool when it comes to larger projects and the overall software development process. Its versatility and depth make it a crucial asset in a developer’s toolkit.
GCC in Larger Projects
In larger projects, the GCC command is not just used for compiling individual C or C++ files. It becomes part of a larger build system, often working in conjunction with tools like Makefiles. A Makefile is a special file (usually named ‘Makefile’) containing shell commands for an automatic generation of executables.
Here’s a simple example of a Makefile that uses the GCC command to compile a C program:
# Makefile
all: hello
hello: hello.c
gcc -o hello hello.c
clean:
rm hello
# Output:
# Creates an executable file named 'hello' using the 'make all' command.
# Removes the 'hello' executable using the 'make clean' command.
In this Makefile, we define two targets: ‘all’ and ‘clean’. The ‘all’ target uses GCC to compile the ‘hello.c’ file into an executable named ‘hello’. The ‘clean’ target removes the ‘hello’ executable.
Debugging with GDB
GCC’s -g
option allows you to generate debug information for use with GDB, the GNU Debugger. This can be incredibly useful for debugging your C and C++ programs.
gcc -g hello.c -o hello
gdb hello
# Output:
# Creates an executable file named 'hello' with debug information.
# Starts a GDB session with the 'hello' executable.
In this example, we first use GCC with the -g
option to compile the ‘hello.c’ file into an executable named ‘hello’ with debug information. We then start a GDB session with the ‘hello’ executable.
Further Resources for Mastering GCC Linux Command
- GNU GCC Official Documentation: The official documentation for GCC. It provides in-depth information about all aspects of GCC.
Linux Command Tutorial: This tutorial provides a comprehensive overview of the Linux command line, including the use of GCC.
GCC and Make: This guide provides a detailed explanation of how to use GCC and Make in the context of C/C++ programming.
By exploring these resources and diving deeper into the capabilities of the GCC command, you can further enhance your skills and efficiency in the Linux environment.
Wrapping Up: The Journey through GCC Linux Command
In this comprehensive guide, we’ve navigated the landscape of the GCC Linux command, a powerful tool used for compiling C and C++ programs in Linux.
We started with the basics, learning how to use the GCC command to compile simple C programs. We then delved into more advanced territory, exploring the use of different flags and options to control the compilation process. We also tackled common issues that you might encounter when using the GCC command, providing solutions and workarounds for each issue.
We didn’t stop there. We went beyond the traditional use of GCC and looked at alternative approaches, such as using Clang as a compiler. We compared these methods, giving you a comprehensive understanding of the various tools available for compiling C and C++ programs in Linux.
Here’s a quick comparison of GCC and Clang:
Compiler | Speed | Flexibility | Error Reporting |
---|---|---|---|
GCC | Fast | High | Moderate |
Clang | Fast | Moderate | High |
Whether you’re a beginner just starting out with GCC or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the GCC command and its capabilities.
Understanding and mastering the GCC command can significantly enhance your productivity and efficiency in the Linux environment. Equipped with this knowledge, you’re now ready to tackle more complex programming tasks. Happy coding!