Sorting JSON Objects | jq ‘sort’ Function Guide
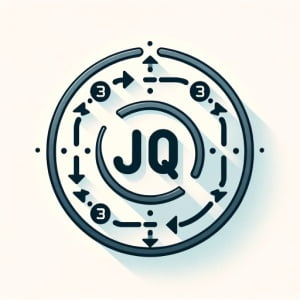
Are you finding it challenging to sort JSON data using jq? You’re not alone. Many developers grapple with this task, but there’s a function that can make this process a breeze.
Like a meticulous librarian arranging books, jq’s sort function can help you organize your JSON data with ease. These sorted data can make your code more readable and efficient, even in complex projects.
This guide will walk you through the process of using the sort function in jq, from the basics to more advanced techniques. We’ll explore jq sort’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering jq sort!
TL;DR: How Do I Sort with jq?
To sort an array in
jq
, you use thesort
function, with the syntax:[arrayOfData] | jq 'sort'
. a powerful tool that can help you organize your JSON data effectively.
Here’s a simple example:
echo '[3, 1, 2]' | jq 'sort'
# Output:
# [1, 2, 3]
In this example, we’re using the sort
function in jq to sort a simple JSON array. We pass the array ‘[3, 1, 2]’ to jq, and it returns the sorted array ‘[1, 2, 3]’.
This is just a basic way to use the
sort
function in jq, but there’s much more to learn about sorting complex JSON data. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with jq Sort
- Exploring Advanced jq Sort Functionality
- Diving Deeper: Alternative jq Sorting Techniques
- Troubleshooting jq Sort: Common Errors and Solutions
- Best Practices and Optimization Tips
- Understanding the Fundamentals of jq and JSON
- Applying jq Sort in Larger Projects
- Complementary jq Functions
- Wrapping Up: jq ‘sort’ Function
Getting Started with jq Sort
To understand jq sort, we first need to understand what jq is and how it interacts with JSON data. jq is a lightweight and flexible command-line JSON processor. It’s like sed for JSON data – you can use it to slice, filter, map, and transform structured data.
The sort
function in jq is used to sort JSON arrays in ascending order. It’s a simple yet powerful tool that can help you organize your JSON data effectively.
Let’s look at an example:
echo '["apple", "banana", "cherry"]' | jq 'sort'
# Output:
# ["apple", "banana", "cherry"]'
In this example, we’re using the sort
function in jq to sort a JSON array of strings. The array ‘apple’, ‘banana’, ‘cherry’ is already sorted in ascending order, so the output remains the same.
One of the key advantages of using the sort
function in jq is its simplicity and effectiveness. However, one potential pitfall is that it only sorts in ascending order. If you want to sort in descending order, you’ll need to use additional functions, which we’ll discuss in the advanced section.
In the next section, we’ll delve into more complex uses of the sort
function in jq, such as sorting nested arrays or objects.
Exploring Advanced jq Sort Functionality
As you become more comfortable with jq sort, you can start to explore its more advanced features. One of these is sorting nested arrays or objects. This can be a bit more complex, but it’s an essential skill for managing more complex JSON data.
Let’s look at an example of sorting a nested array:
echo '[{"name": "apple", "price": 1.2}, {"name": "banana", "price": 0.5}, {"name": "cherry", "price": 2.5}]' | jq 'sort_by(.price)'
# Output:
# [{"name": "banana", "price": 0.5}, {"name": "apple", "price": 1.2}, {"name": "cherry", "price": 2.5}]
In this example, we’re using the sort_by
function in jq, which allows us to sort a JSON array of objects based on the value of a specific key—in this case, ‘price’. The function returns the array sorted in ascending order of prices.
One of the advantages of using the sort_by
function is that it allows for more complex sorting operations. However, it can be a bit more challenging to use, especially if you’re new to jq or JSON data.
In the next section, we’ll discuss some alternative approaches to sorting JSON data with jq, including other related functions and techniques.
Diving Deeper: Alternative jq Sorting Techniques
While the sort
and sort_by
functions are powerful tools in jq, there are other related functions and techniques that can accomplish similar tasks. These alternatives can offer more flexibility or efficiency depending on your specific needs.
Using group_by
for Sorting
The group_by
function is one of these alternatives. This function groups input items into arrays by a key. Let’s look at an example:
echo '[{"name": "apple", "color": "red"}, {"name": "banana", "color": "yellow"}, {"name": "cherry", "color": "red"}]' | jq 'group_by(.color)'
# Output:
# [[{"name": "banana", "color": "yellow"}], [{"name": "apple", "color": "red"}, {"name": "cherry", "color": "red"}]]
In this example, we’re using the group_by
function to group a JSON array of objects based on the value of a specific key, in this case, ‘color’. The function returns the array grouped by color.
This approach offers the benefit of grouping, which can be useful in certain scenarios. However, it doesn’t sort within the groups. So, if you need to sort within groups, you’ll need to use sort
or sort_by
in combination with group_by
.
Using reverse
for Descending Order
Another alternative approach is to use the reverse
function in combination with sort
or sort_by
to sort in descending order. Here’s an example:
echo '[3, 1, 2]' | jq 'sort | reverse'
# Output:
# [3, 2, 1]
In this example, we’re using the sort
function to sort the array in ascending order, and then the reverse
function to reverse the order, resulting in a sorted array in descending order.
The advantage of this approach is that it allows for descending order sorting. However, it requires an additional step, which could potentially impact performance for large datasets.
In the next section, we’ll discuss common errors or obstacles and their solutions when using jq sort.
Troubleshooting jq Sort: Common Errors and Solutions
While jq sort is a powerful tool, it’s not without its potential pitfalls. Here, we’ll cover some common errors or obstacles you might encounter when using jq sort, along with their solutions.
Sorting Non-Array JSON Data
One common error is trying to sort non-array JSON data. The sort
function in jq only works with arrays. If you try to sort a JSON object or a non-array data type, you’ll get an error.
echo '{"name": "apple", "price": 1.2}' | jq 'sort'
# Output:
# jq: error: sort/0 is not defined at <top-level>, line 1:
# sort
# jq: 1 compile error
In this example, we’re trying to sort a JSON object, which results in a compile error. To fix this, you’ll need to ensure that you’re only using sort
with JSON arrays.
Sorting Arrays with Mixed Data Types
Another common error is trying to sort arrays with mixed data types. jq sort can only compare values of the same type. If you try to sort an array with mixed types, you’ll get an error.
echo '["apple", 1, 2.5]' | jq 'sort'
# Output:
# jq: error (at <stdin>:1): Cannot compare string and number
In this example, we’re trying to sort an array with mixed types, which results in an error. To fix this, you’ll need to ensure that your array only contains values of the same type.
Best Practices and Optimization Tips
When using jq sort, there are a few best practices and optimization tips to keep in mind:
- Use the right function for the job. While
sort
is a powerful tool, it’s not always the best choice. Depending on your needs, you might be better off usingsort_by
,group_by
,reverse
, or another jq function. Be mindful of performance. Sorting can be a resource-intensive operation, especially for large datasets. If performance is a concern, you might need to consider alternative approaches or optimize your jq scripts.
Understand your data. Before you can effectively sort your JSON data, you need to understand its structure and content. Spend some time exploring your data before you start writing your jq scripts.
In the next section, we’ll delve deeper into the fundamentals of jq and JSON data, providing you with a solid foundation for understanding and using jq sort.
Understanding the Fundamentals of jq and JSON
Before diving deeper into the sort function of jq, it’s crucial to understand the basics of jq and JSON data.
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It’s based on a subset of JavaScript but is language-independent, with parsers available for virtually every programming language.
{
"fruit": "Apple",
"size": "Large",
"color": "Red"
}
In this example, we have a JSON object that describes an apple. It’s composed of key-value pairs, with keys being strings and values being valid JSON data types (string, number, object, array, boolean, or null).
What is jq?
jq is a lightweight and flexible command-line JSON processor. It’s like sed for JSON data – you can use it to slice, filter, map, and transform structured data. It’s built to process JSON data, but it’s also a powerful tool for manipulating other text-based data formats.
echo '{"fruit": "Apple", "size": "Large", "color": "Red"}' | jq '.color'
# Output:
# "Red"
In this example, we’re using jq to extract the ‘color’ value from a JSON object. The ‘.’ is a filter that takes input and produces it unchanged as output. The ‘.color’ filter extracts the ‘color’ value from the input.
Other jq Functions
While this guide focuses on the sort
function, jq offers a wide range of other functions for manipulating JSON data. These include map
, reduce
, select
, has
, length
, and many more. Each of these functions has its own use cases and can be combined in powerful ways to process and analyze JSON data.
In the next section, we’ll explore the application of the sort
function in larger scripts or projects and suggest other jq functions that often accompany the sort
function in typical use cases.
Applying jq Sort in Larger Projects
The sort
function in jq is not only useful for simple data manipulation tasks but also plays a vital role in larger scripts or projects. By sorting JSON data, you can make your code more readable and efficient, which is particularly crucial in complex projects.
Let’s look at an example where the sort
function is used in a larger script:
jq -n '{items: [inputs | .item] | sort_by(.price)}' items.json
# Output:
# Sorted list of items from the items.json file
In this example, we’re using the sort_by
function in a larger jq script to sort a list of items from a JSON file. This kind of sorting can be particularly useful in larger projects where you need to manage and manipulate large amounts of JSON data.
Complementary jq Functions
In addition to sort
, there are several other jq functions that often accompany it in typical use cases. These include map
, reduce
, select
, has
, length
, and many more. Each of these functions has its own use cases and can be combined in powerful ways to process and analyze JSON data.
Further Resources for jq
To continue your journey towards mastering jq sort, here are some additional resources that offer more in-depth information about this topic:
- jq Manual (official documentation): This is the official jq manual, which provides comprehensive information about all jq functions, including
sort
. Learn jq With jq.play: This is an interactive jq playground that allows you to experiment with jq functions and see the results in real time.
Baeldung’s jq Guide: This is a detailed tutorial that covers various aspects of jq, including the
sort
function.
Wrapping Up: jq ‘sort’ Function
In this comprehensive guide, we’ve journeyed through the world of jq sort, a powerful function for sorting JSON data in jq.
We began with the basics, learning how to sort simple JSON arrays using the sort
function. From there, we ventured into more advanced territory, exploring how to sort nested arrays and objects using the sort_by
function. We also looked at alternative approaches, such as group_by
and reverse
, offering you a broader toolkit for sorting JSON data with jq.
Along the way, we tackled common challenges you might face when using jq sort, such as sorting non-array JSON data and arrays with mixed data types, providing you with solutions for each issue. We also offered best practices and optimization tips to help you use jq sort effectively and efficiently.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
sort | Simple and effective | Only sorts in ascending order |
sort_by | Allows for complex sorting operations | Can be challenging to use for beginners |
group_by | Useful for grouping data | Doesn’t sort within groups |
reverse | Allows for descending order sorting | Requires an additional step |
Whether you’re just starting out with jq sort or you’re looking to level up your JSON data manipulation skills, we hope this guide has given you a deeper understanding of jq sort and its capabilities.
With its balance of simplicity, flexibility, and power, jq sort is an essential tool for any developer working with JSON data. Happy coding!