The Ultimate jq Tutorial | Master JSON Processing
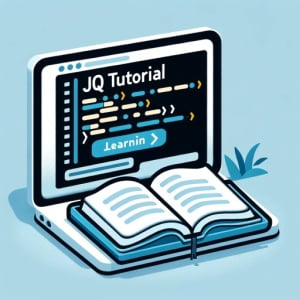
Are you struggling to manipulate JSON data from the command line? You’re not alone. Many developers find this task challenging, but there’s a tool that can simplify this process.
Think of jq as the Swiss Army knife for JSON data. It’s a powerful tool that lets you parse, filter, and transform JSON data with ease. Whether you’re dealing with simple or complex JSON data, jq is your go-to solution.
In this tutorial, we’ll guide you through the basics to advanced usage of jq, making JSON data manipulation a breeze. We’ll delve into jq’s core functionality, explore its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering jq!
TL;DR: How Do I Use jq for JSON Processing?
jq
is a command-line JSON processor that allows you toparse
,filter
, andtransform
JSON data. Here’s a simple example of how to use it:
echo '{"foo": "bar"}' | jq .
# Output:
# {"foo": "bar"}
In this example, we’re using the echo
command to create a JSON object with a single property foo
set to bar
. We then pipe this JSON object into jq
, which pretty-prints the JSON to the console.
This is just a basic way to use jq for JSON processing, but there’s much more to learn about parsing and manipulating JSON data with jq. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with jq
- Parsing JSON Data with jq
- Filtering JSON Data with jq
- Advanced jq Techniques: Transforming JSON Data
- Advanced jq Techniques: Using Filters
- Exploring Alternatives to jq
- Troubleshooting Common jq Errors
- jq Best Practices and Optimization Tips
- JSON: The Cornerstone of Modern Data Exchange
- Why jq is Essential for JSON Data Manipulation
- Applying jq in Larger Projects
- Complementary Command-Line Tools
- Wrapping Up: Using jq for Efficient JSON Manipulation
Getting Started with jq
Before we can start manipulating JSON data, we first need to install jq. The installation process varies depending on your operating system.
Installing jq on Linux
For Linux users, you can install jq with the following command:
sudo apt-get install jq
Installing jq on MacOS
If you’re using MacOS, you can install jq using Homebrew with the following command:
brew install jq
Installing jq on Windows
For Windows users, you can download jq from its GitHub page here.
Now that we have jq installed, let’s dive into parsing and filtering JSON data.
Parsing JSON Data with jq
Let’s start with a simple JSON object:
echo '{"name": "John", "age": 30, "city": "New York"}' | jq .
# Output:
# {
# "name": "John",
# "age": 30,
# "city": "New York"
# }
In this example, we’re creating a JSON object with name
, age
, and city
properties. We then pipe this JSON object into jq
, which pretty-prints the JSON to the console.
Filtering JSON Data with jq
jq allows you to filter JSON data using a simple syntax. Here’s how you can extract the name
property from our JSON object:
echo '{"name": "John", "age": 30, "city": "New York"}' | jq .name
# Output:
# "John"
This command filters the JSON data to only output the value of the name
property, which is John
.
In the next section, we’ll cover more advanced usage of jq.
Advanced jq Techniques: Transforming JSON Data
jq is not just a tool for parsing and filtering JSON data; it’s also a powerful tool for transforming JSON data. Let’s take a look at how we can use jq to modify the structure of our JSON data.
Let’s say we have the following JSON data:
echo '{"employees": [{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]}' | jq .
# Output:
# {
# "employees": [
# {
# "name": "John",
# "age": 30
# },
# {
# "name": "Jane",
# "age": 25
# }
# ]
# }
We can use jq to transform this data into a different structure. For instance, let’s say we want to create a new JSON object where the employee names are the keys and their ages are the values. We can achieve this with the following command:
echo '{"employees": [{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]}' | jq '{( .employees[] | .name ): .age}'
# Output:
# {
# "John": 30,
# "Jane": 25
# }
In this command, we’re using the map
function in jq to transform each item in the employees
array into a new object where the key is the employee’s name and the value is the employee’s age.
Advanced jq Techniques: Using Filters
jq also allows you to use advanced filters to extract specific data from your JSON. For example, you can use the select
function in jq to filter data based on a condition.
Let’s say we want to extract all employees who are older than 25. We can do this with the following command:
echo '{"employees": [{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]}' | jq '.employees[] | select(.age > 25)'
# Output:
# {
# "name": "John",
# "age": 30
# }
In this command, we’re using the select
function in jq to filter the employees
array for employees whose age is greater than 25.
As you can see, jq is a powerful tool for manipulating JSON data. In the next section, we’ll explore alternative approaches to handling JSON data.
Exploring Alternatives to jq
While jq is a powerful tool for JSON processing, it’s not the only option available. Let’s explore a few alternatives and see how they compare to jq.
JSON Processing with Python
Python is a versatile language that has built-in support for JSON processing. Here’s how you can parse and filter JSON data using Python:
import json
json_string = '{"name": "John", "age": 30, "city": "New York"}'
data = json.loads(json_string)
print(data['name'])
# Output:
# John
In this example, we’re using Python’s json
module to parse the JSON string and extract the name
property. While Python is more verbose than jq, it offers more flexibility and is a good option if you’re already working within a Python environment.
JSON Processing with JavaScript
JavaScript, being the language of the web, naturally has excellent support for JSON processing. Here’s how you can parse and filter JSON data using JavaScript:
var json_string = '{"name": "John", "age": 30, "city": "New York"}';
var data = JSON.parse(json_string);
console.log(data.name);
// Output:
// John
In this example, we’re using JavaScript’s JSON.parse
method to parse the JSON string and log the name
property to the console. JavaScript is a good option if you’re working with JSON data in a web context.
Decision-Making Considerations
When deciding between jq and its alternatives, consider the following factors:
- Environment: If you’re working within a Python or JavaScript environment, it might make more sense to use these languages’ built-in JSON processing capabilities.
- Complexity: For complex JSON processing tasks, a full-fledged programming language like Python or JavaScript might be more suitable.
- Performance: If performance is a concern, jq is generally faster than Python or JavaScript for JSON processing tasks.
In the next section, we’ll discuss common issues you might encounter when using jq and how to troubleshoot them.
Troubleshooting Common jq Errors
While jq is a powerful tool, it can sometimes throw errors that might seem confusing. Let’s discuss some common issues and their solutions.
Error: “Cannot iterate over null (null)”
This error occurs when you’re trying to iterate over a null value. For example, consider the following command:
echo '{"name": "John", "age": null}' | jq '.age[]'
# Output:
# jq: error (at <stdin>:1): Cannot iterate over null (null)
In this example, we’re trying to iterate over the age
property, which is set to null. To fix this error, we need to ensure that we’re not trying to iterate over a null value.
Error: “Cannot index number with string “name””
This error occurs when you’re trying to access a property on a number. For example, consider the following command:
echo '{"name": "John", "age": 30}' | jq '.age.name'
# Output:
# jq: error (at <stdin>:1): Cannot index number with string "name"
In this example, we’re trying to access the name
property on the age
property, which is a number. To fix this error, we need to ensure that we’re only trying to access properties on objects.
jq Best Practices and Optimization Tips
Here are a few best practices and optimization tips to help you get the most out of jq:
- Use Compact Output: By default, jq pretty-prints its output. If you’re dealing with large JSON data, consider using the
-c
option to produce compact output. - Use Raw Output: If you’re extracting a single string value, consider using the
-r
option to produce raw output without quotes. - Avoid Unnecessary Piping: Each pipe in a jq command adds overhead. Try to accomplish your task with as few pipes as possible.
In the next section, we’ll dive deeper into the fundamentals of JSON and why tools like jq are important for handling JSON data.
JSON: The Cornerstone of Modern Data Exchange
JSON, standing for JavaScript Object Notation, has become the de facto standard for data exchange on the web. Its simplicity and compatibility with many programming languages have propelled its popularity, making it a crucial part of modern web development.
JSON data is organized into key-value pairs, similar to a dictionary in Python or an object in JavaScript. This structure makes it intuitive to work with and easy to understand. Here’s an example of what JSON data might look like:
{
"name": "John",
"age": 30,
"city": "New York"
}
In this example, name
, age
, and city
are the keys, and John
, 30
, and New York
are the corresponding values.
Why jq is Essential for JSON Data Manipulation
While JSON’s simplicity is one of its strengths, working with JSON data can become complex when dealing with large or nested JSON data. This is where jq shines.
jq allows you to parse, filter, and transform JSON data right from the command line. Its powerful syntax enables you to drill down into complex JSON data structures, extract the information you need, and even transform the data into a new structure.
For instance, you could use jq to extract a list of names from an array of user data, transform an array of objects into a single object, or filter an array of products based on price. The possibilities are endless.
As more and more applications continue to rely on JSON for data exchange, tools like jq will only become more essential in the world of software development.
Applying jq in Larger Projects
jq’s power truly shines when applied within larger scripts or projects. Its ability to parse and manipulate JSON data makes it a valuable tool in any developer’s arsenal. Whether you’re working with data from an API or handling configuration files, jq can help streamline your workflow.
For instance, you can use jq to parse the JSON response from an API call within a bash script, allowing you to automate data retrieval and processing. Here’s an example:
response=$(curl -s 'https://api.github.com/users/octocat')
username=$(echo "$response" | jq -r '.login')
echo "Username: $username"
# Output:
# Username: octocat
In this example, we’re using curl
to send a GET request to GitHub’s API, retrieving information about a user. We then use jq to parse the JSON response and extract the login
property, which is the username.
Complementary Command-Line Tools
While jq is powerful on its own, its utility can be enhanced when used in conjunction with other command-line tools. Tools like curl
for making HTTP requests, grep
for searching within files, and sed
for text manipulation often accompany jq in typical use cases, forming a powerful toolkit for data manipulation and processing.
Further Resources for jq
To continue your learning journey with jq, consider the following resources:
- jq’s Official Documentation: A comprehensive guide to jq’s syntax and features.
- Learn jq With jq.play: An interactive tool for learning and experimenting with jq.
- Mastering jq Course on Udemy: A complete course on jq, covering everything from basics to advanced topics.
Wrapping Up: Using jq for Efficient JSON Manipulation
In this comprehensive guide, we’ve delved deep into the world of jq, a powerful command-line tool for parsing, filtering, and transforming JSON data.
We embarked on our journey with the basics, learning how to install jq and use it to parse and filter JSON data. As we progressed, we explored more advanced usage scenarios, including transforming JSON data and using advanced filters. Along the way, we tackled common challenges that you might face when using jq, providing you with solutions and workarounds for each issue.
We also ventured into alternative approaches to JSON processing, comparing jq with other tools like Python and JavaScript. Here’s a quick comparison of these methods:
Method | Flexibility | Ease of Use | Performance |
---|---|---|---|
jq | Moderate | High | High |
Python | High | Moderate | Moderate |
JavaScript | High | Moderate | Moderate |
Whether you’re just starting out with jq or you’re looking to level up your JSON processing skills, we hope this guide has given you a deeper understanding of jq and its capabilities.
With its balance of ease of use, flexibility, and performance, jq is an indispensable tool for JSON data manipulation. Now, you’re well equipped to handle any JSON data that comes your way. Happy coding!