Bash Script ‘-n’ Operator Explained: Evaluating Expressions
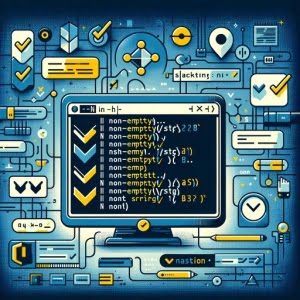
Have you ever come across the ‘-n’ option in bash scripting and wondered what it does? You’re not alone. Many developers encounter this option but don’t fully grasp its functionality. Think of the ‘-n’ option as a silent operator, subtly influencing your bash scripts in significant ways.
This guide will demystify the ‘-n’ option in bash scripting. We’ll provide clear examples and in-depth explanations to help you understand its effects and usage from basic to advanced.
So, let’s dive in and start mastering the ‘-n’ option in bash!
TL;DR: What Does ‘-n’ Do in Bash?
The
'-n'
option in bash is used to read commands but not execute them, such asecho -n 'Hello'
. It’s like a rehearsal for your bash scripts, allowing them to go through the motions without actually performing any actions.
Here’s a simple example:
echo -n 'Hello'
# Output:
# 'Hello'
In this example, the echo
command is used with the -n
option to print ‘Hello’ without a trailing newline. This means that the cursor stays on the same line after printing ‘Hello’, rather than moving to a new line as it usually would.
This is just a basic use of the ‘-n’ option in bash. There’s much more to learn about how ‘-n’ can be used in more complex scripts and in combination with other options. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Bash Scripting: The ‘-n’ Option Basics
- Delving Deeper: Advanced ‘-n’ Usage in Bash
- Exploring Alternatives: Beyond ‘-n’ in Bash
- Navigating Challenges: ‘-n’ Troubleshooting
- Bash Scripting Fundamentals: Understanding ‘-n’ and Beyond
- Expanding Horizons: ‘-n’ in Larger Bash Projects
- Wrapping Up: Mastering ‘-n’ in Bash Scripting
Bash Scripting: The ‘-n’ Option Basics
The ‘-n’ option in bash scripting is a powerful tool that can change how your scripts behave. At its most basic level, ‘-n’ is used to read commands but not execute them. This can be especially useful when you want to check a script for syntax errors without running it.
Let’s take a look at a simple example:
bash -n script.sh
# Output:
# If there are no syntax errors, there will be no output. If there are syntax errors, they will be printed to the terminal.
In this example, the bash -n
command is used to read the script.sh
file. The ‘-n’ option tells bash to read the commands in script.sh
but not execute them. If there are any syntax errors in script.sh
, this command will print them out without running the script.
The ‘-n’ option can be a beneficial tool in your bash scripting toolkit. It allows you to check your scripts for errors without the risk of executing potentially harmful commands. However, it’s important to remember that ‘-n’ only checks for syntax errors. It won’t catch logical errors in your script, such as incorrect variable usage or command sequences.
Delving Deeper: Advanced ‘-n’ Usage in Bash
As you become more comfortable with bash scripting, you’ll find that the ‘-n’ option can be used in more complex ways. For instance, it can be combined with other options or commands for more nuanced script behavior.
Consider the following script:
#!/bin/bash
read -n 1 -p "Enter a character: " char
echo -e "
You entered: $char"
# Output:
# Enter a character: a
# You entered: a
In this script, we’re using the -n
option with the read
command. The -n 1
option tells read
to accept only a single character of input. The -p
option allows us to display a prompt to the user. After the user enters a character, the script echoes the input back to them.
This example illustrates how the -n
option can be used with other commands and options to control script behavior more precisely. It’s a powerful tool for creating more interactive and user-friendly scripts.
Remember, the -n
option in bash scripting is versatile and can be used in many different contexts. As you gain more experience with bash, you’ll find even more ways to use -n
to improve your scripts.
Exploring Alternatives: Beyond ‘-n’ in Bash
While the ‘-n’ option in bash scripting is powerful, it’s not the only way to achieve similar results. There are other bash options and commands that can provide equivalent functionality, each with its own advantages and disadvantages.
For instance, you might use the set -n
command to enable the same ‘read but not execute’ behavior for an entire script. Here’s an example:
#!/bin/bash
set -n
echo "This will not be printed."
# Output:
# (No output)
In this script, the set -n
command enables the ‘read but not execute’ mode for all subsequent commands. The echo
command is read but not executed, so nothing is printed to the terminal.
This method has the advantage of being able to toggle the ‘read but not execute’ mode on and off within a script. However, it applies to all subsequent commands, not just one, which may not be what you want in all cases.
Another alternative is to use the :
command (also known as the ‘null’ command) to ignore a command or a block of commands. Here’s an example:
#!/bin/bash
: << 'END'
echo "This will not be printed."
END
# Output:
# (No output)
In this script, the :
command is used with a ‘here document’ (<< 'END'
) to ignore everything up to the matching ‘END’. The echo
command is ignored, so nothing is printed to the terminal.
This method has the advantage of being able to ignore multiple commands at once, but it can be more confusing to read, especially for beginners.
Remember, there’s no ‘one-size-fits-all’ approach in bash scripting. The best method depends on your specific needs and the context of your script.
As with any bash scripting tool, using the ‘-n’ option can sometimes lead to unexpected results. It’s important to understand these potential pitfalls and how to navigate them. Let’s discuss some common issues that may arise when using ‘-n’ in bash scripting, along with some solutions and workarounds.
Unexpected Behavior with Echo
One common issue with the ‘-n’ option arises when it’s used with the echo
command. The ‘-n’ option with echo
doesn’t add a newline at the end of the output, which can lead to unexpected results. For instance:
echo -n 'Hello'; echo ' World'
# Output:
# Hello World
In this example, ‘World’ is printed on the same line as ‘Hello’, because the ‘-n’ option prevents echo
from adding a newline after ‘Hello’. If you want ‘World’ to be printed on a new line, you can add an explicit newline with echo -e "
:
"
echo -n 'Hello'; echo -e "
"; echo 'World'
# Output:
# Hello
# World
Syntax Errors with ‘-n’
Another issue arises when using ‘-n’ to check for syntax errors. The ‘-n’ option only checks the syntax of commands, not their logic. This means that while ‘-n’ can catch syntax errors like missing parentheses or semicolons, it won’t catch logical errors like using a variable before it’s defined.
For instance, consider the following script:
#!/bin/bash
bash -n -c 'echo $(( 1 / 0 ))'
# Output:
# (No output, even though dividing by zero is a logical error)
In this script, the ‘bash -n’ command doesn’t catch the logical error of dividing by zero. To catch logical errors, you’ll need to actually run the script or use other debugging tools.
These are just a few examples of the issues you may encounter when using the ‘-n’ option in bash scripting. Understanding these potential pitfalls can help you use ‘-n’ more effectively and troubleshoot issues when they arise.
Bash Scripting Fundamentals: Understanding ‘-n’ and Beyond
Bash scripting is a powerful tool for automating tasks in Unix-like operating systems. It’s a command language that allows you to write scripts to control and automate the execution of commands. One of the fundamental aspects of bash scripting is the use of options, like ‘-n’, to modify the behavior of commands.
The Role of Options in Bash
Options, also known as flags or switches, are used to modify the behavior of commands in bash scripting. They’re typically preceded by a dash (‘-‘) and are used to specify different modes of operation for commands. For instance, the ‘-n’ option is used with the bash
command to read commands but not execute them.
Here’s an example:
bash -n script.sh
# Output:
# If there are no syntax errors, there will be no output. If there are syntax errors, they will be printed to the terminal.
In this example, the bash -n
command is used to check script.sh
for syntax errors. The ‘-n’ option tells bash to read the commands in script.sh
but not execute them.
Related Concepts and Commands
Understanding the ‘-n’ option in bash scripting also involves understanding related concepts and commands. For instance, the echo
command is often used with ‘-n’ to print output without a trailing newline. The read
command can also be used with ‘-n’ to specify the number of characters to read from the input.
Here’s an example of using the echo
command with ‘-n’:
echo -n 'Hello, world'
# Output:
# 'Hello, world'
And here’s an example of using the read
command with ‘-n’:
read -n 1 -p "Enter a character: " char
echo -e "
You entered: $char"
# Output:
# Enter a character: a
# You entered: a
These examples illustrate how the ‘-n’ option can be used with different commands to achieve different results. Understanding these related concepts and commands will give you a broader understanding of bash scripting and how to use the ‘-n’ option effectively.
Expanding Horizons: ‘-n’ in Larger Bash Projects
As you grow in your understanding of bash scripting, you’ll find that the ‘-n’ option can be an invaluable tool in larger projects. It can help you debug complex scripts, streamline your code, and make your scripts more efficient.
Consider a large bash script that automates a series of tasks. You could use the ‘-n’ option to check the entire script for syntax errors before running it. This could save you a lot of time and trouble by catching errors before they cause problems in the execution of your script.
bash -n large_script.sh
# Output:
# If there are no syntax errors, there will be no output. If there are syntax errors, they will be printed to the terminal.
In this example, the bash -n
command is used to check large_script.sh
for syntax errors. The ‘-n’ option tells bash to read the commands in large_script.sh
but not execute them.
Exploring Related Bash Options and Commands
The ‘-n’ option is just one of many options available in bash scripting. There are many other options and commands that can help you write more efficient and effective scripts. For instance, the ‘-v’ option can be used to print shell input lines as they are read, and the ‘-x’ option can be used to print commands and their arguments as they are executed.
Further Resources for Bash Scripting Mastery
To deepen your understanding of bash scripting and the ‘-n’ option, here are a few resources that you might find helpful:
- Advanced Bash-Scripting Guide: This guide provides a detailed introduction to bash scripting, including a comprehensive list of bash options and commands.
Bash Guide for Beginners: This guide is a great starting point for beginners to bash scripting. It covers the basics of bash scripting, including the use of options like ‘-n’.
GNU Bash Manual: This is the official manual for bash, provided by the GNU Project. It provides a detailed description of all bash options and commands, including ‘-n’.
Wrapping Up: Mastering ‘-n’ in Bash Scripting
In this comprehensive guide, we’ve explored the use of the ‘-n’ option in bash scripting. We’ve delved into its role in reading commands without executing them, and how this functionality can be a game-changer in your bash scripting journey.
We started with the basics, understanding how the ‘-n’ option works with simple commands and scripts. We then ventured into more complex scenarios, combining ‘-n’ with other options and commands for more nuanced script behavior.
We also looked at common challenges you might encounter when using ‘-n’, from unexpected behavior with the echo
command to syntax errors, and provided solutions to help you navigate these issues. We even explored alternatives to ‘-n’, giving you a wider perspective on achieving similar results in bash scripting.
Method | Pros | Cons |
---|---|---|
Using ‘-n’ with bash | Checks scripts for syntax errors without executing them | Only checks for syntax errors, not logical errors |
Using set -n | Can enable ‘read but not execute’ mode for an entire script | Applies to all subsequent commands, not just one |
Using : command with ‘here document’ | Can ignore multiple commands at once | Can be confusing to read, especially for beginners |
Whether you’re just starting out with bash scripting or you’re looking to deepen your understanding of the ‘-n’ option, we hope this guide has provided you with a comprehensive understanding of its functionality and potential use cases.
The ‘-n’ option is a powerful tool in bash scripting. Understanding its usage and potential pitfalls can significantly enhance your scripting skills. Happy scripting!