got NPM | Module Guide for HTTP Requests in Node.js
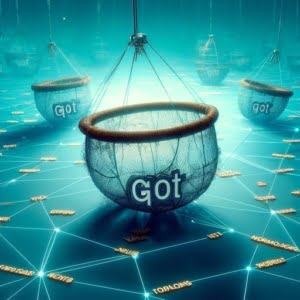
We often need to make HTTP requests in our software projects at IOFLOOD. To address this, we’ve explored the ‘got’ library in Node.js. We’ve found that the library can make tasks like fetching data from a remote server or sending information to an API a breeze. As we believe this library can aid developers on our bare metal cloud servers, we’ve provided this detailed guide with practical examples.
This guide will walk you through the essentials of using ‘got’ to streamline your web requests, making your coding journey smoother and more efficient. With ‘got’, you’ll find that managing HTTP requests becomes not just manageable, but a genuinely enjoyable part of your Node.js projects.
Let’s dive into the world of making HTTP requests with ‘got’ in Node.js!
TL;DR: How Do I Make an HTTP Request in Node.js Using ‘got’?
To make an HTTP request in Node.js using ‘got’, install the ‘got library’ with,
npm install got
, and require it in your Node.js script,const got = require('got');
. You can then use it to fetch data from a URL.
Here’s a quick example to get you started:
const got = require('got');
(async () => {
const response = await got('https://example.com');
console.log(response.body);
})();
# Output:
# 'The HTML content of the example.com homepage'
In this example, we import the ‘got’ library and use an asynchronous function to make a GET request to ‘https://example.com’. The response is then logged to the console, showing the HTML content of the requested page. This is a straightforward way to start making HTTP requests with ‘got’.
Ready to dive deeper into ‘got’ and explore more of its capabilities? Continue reading for more detailed instructions and advanced usage tips.
Table of Contents
Getting Started: ‘got’ HTTP Requests
To harness the power of ‘got’ in your Node.js applications, your journey begins with its installation. The ‘got’ library is a concise, human-friendly way to make HTTP requests, perfect for newcomers and seasoned developers alike.
Installing ‘got’ via NPM
Before diving into making requests, you need to install ‘got’. Assuming you have Node.js and npm installed, open your terminal and run:
npm install got
This command fetches ‘got’ from the npm registry and adds it to your project, allowing you to start making HTTP requests.
Making a GET Request
After installation, making a GET request to fetch data is straightforward. Here’s how:
const got = require('got');
(async () => {
try {
const response = await got('https://api.example.com/data');
console.log(JSON.parse(response.body));
} catch (error) {
console.log(error.response.body);
}
})();
# Output:
# {"data": "Sample response from the API"}
In this example, we use ‘got’ to make an asynchronous GET request to a hypothetical API. The response is parsed from JSON and logged, showing the data retrieved. This demonstrates how ‘got’ simplifies fetching data from the web.
Making a POST Request
Sending data is just as easy with ‘got’. Here’s a basic example of making a POST request:
const got = require('got');
(async () => {
const response = await got.post('https://api.example.com/submit', {
json: {
username: 'user123',
password: 'securepassword123'
},
responseType: 'json'
});
console.log(response.body);
})();
# Output:
# {"success": true, "message": "Data submitted successfully"}
This snippet illustrates submitting data to an API using a POST request. The ‘json’ option simplifies sending JSON data, and ‘responseType: ‘json” ensures the response is automatically parsed. This showcases ‘got’s ability to not only fetch but also send data with ease.
Understanding these basics equips you with the knowledge to start leveraging ‘got’ in your Node.js projects, making HTTP requests more manageable and your development process more efficient.
Advanced Features of ‘got’
When you’ve got the basics down, ‘got’ offers advanced features that can significantly enhance your HTTP request handling in Node.js. Let’s explore how to implement retries, set request timeouts, and utilize streams for handling large responses.
Implementing Retries
Network unreliability is a common issue. ‘got’ provides a retry mechanism out of the box. Here’s how to set it up:
const got = require('got');
const client = got.extend({
retry: { limit: 2, methods: ['GET', 'POST'] },
timeout: 10000
});
(async () => {
try {
const response = await client.get('https://api.example.com/unstable');
console.log(response.body);
} catch (error) {
console.error('Request failed:', error);
}
})();
# Output:
# 'Request failed: TimeoutError: Request timed out'
This example demonstrates setting up retries with ‘got’. By extending the ‘got’ instance with a retry
policy, you ensure that the request attempts are retried up to 2 times for GET and POST methods if they fail. The timeout
option is also set to 10 seconds to prevent hanging requests.
Setting Request Timeouts
To avoid indefinitely waiting on a response, setting timeouts is crucial. Here’s how to configure them in ‘got’:
const got = require('got');
(async () => {
try {
const response = await got('https://api.example.com/data', { timeout: 5000 });
console.log('Data received:', response.body);
} catch (error) {
console.error('Request timed out', error);
}
})();
# Output:
# 'Request timed out: TimeoutError: Request timed out'
This snippet configures a 5-second timeout for a GET request. If the server does not respond within this timeframe, ‘got’ throws a TimeoutError, demonstrating how ‘got’ helps manage long or unresponsive requests efficiently.
Using Streams for Large Responses
Handling large responses efficiently is where Node.js shines, and ‘got’ leverages this with stream support. Here’s a streamlined way to process large datasets:
const got = require('got');
const fs = require('fs');
(async () => {
const stream = got.stream('https://api.example.com/large-data');
stream.pipe(fs.createWriteStream('largeData.json'));
stream.on('end', () => console.log('Download complete.'));
})();
# Output:
# 'Download complete.'
In this example, ‘got’ is used to stream a large dataset directly to a file, largeData.json
. This method is highly efficient for handling large amounts of data without overwhelming memory. It showcases ‘got’s ability to work seamlessly with Node.js streams, providing a powerful tool for data-intensive applications.
By mastering these advanced features, you can take full advantage of ‘got’ in your Node.js projects, making your HTTP request handling more robust and efficient.
Exploring Alternatives to ‘got’
While ‘got’ is a powerful tool for handling HTTP requests in Node.js, it’s not the only player in the field. Let’s dive into alternative libraries like ‘axios’ and ‘request’, comparing their features and use cases to help you choose the right tool for your project.
‘axios’ vs. ‘got’
‘axios’ is another popular HTTP client for Node.js and browser environments. Here’s a quick example of making a GET request with ‘axios’:
const axios = require('axios');
axios.get('https://api.example.com/data')
.then(response => console.log(response.data))
.catch(error => console.error(error));
# Output:
# {"data": "Sample response from the API"}
This example highlights ‘axios’s promise-based syntax, which differs from ‘got’s promise and async/await approach. A key feature of ‘axios’ is its ability to run on both server and client sides, making it a versatile choice for full-stack development.
‘request’ vs. ‘got’
Note: As of my last update, the ‘request’ library has been deprecated. However, it’s worth mentioning for historical context and understanding the evolution of HTTP request libraries in Node.js.
const request = require('request');
request('https://api.example.com/data', (error, response, body) => {
if (!error && response.statusCode == 200) {
console.log(body);
}
});
# Output:
# '{"data": "Sample response from the API"}'
‘request’ was known for its callback-based approach to HTTP requests, which contrasts with the promise-based and async/await patterns seen in ‘got’ and ‘axios’. Though no longer maintained, ‘request’ played a significant role in Node.js HTTP client history.
Choosing the Right Tool
When deciding between ‘got’, ‘axios’, and other libraries, consider factors like syntax preference (callback vs. promise vs. async/await), environment compatibility (Node.js vs. full-stack), and specific features (streaming support, retry mechanisms). Each project’s requirements will guide your choice, ensuring you pick the library that best fits your development needs.
By understanding the strengths and limitations of ‘got’ in comparison to alternatives like ‘axios’ and ‘request’, you can make informed decisions on the best tools for your Node.js projects, optimizing your development workflow and outcomes.
Troubleshooting ‘got’ in Node.js
Even with a powerful library like ‘got’, you might encounter challenges such as network errors, JSON parsing issues, and redirects handling. Let’s explore some common pitfalls and how to navigate them effectively.
Handling Network Errors
Network errors are inevitable. Here’s how you can handle them gracefully with ‘got’:
const got = require('got');
(async () => {
try {
const response = await got('https://api.example.com/unreachable');
} catch (error) {
console.error('Network error:', error.message);
}
})();
# Output:
# 'Network error: Request failed with status code 404'
In this example, attempting to access an unreachable URL triggers a catch block, allowing us to log a custom error message. Handling errors this way ensures your application can respond to network issues without crashing.
Parsing JSON Responses
‘got’ automatically parses JSON responses if the responseType is set to ‘json’. However, it’s crucial to handle parsing errors. Here’s an example:
const got = require('got');
(async () => {
try {
const response = await got('https://api.example.com/data', { responseType: 'json' });
console.log(response.body);
} catch (error) {
console.error('Parsing error:', error.message);
}
})();
# Output:
# {"data": "Sample JSON response"}
This snippet demonstrates ‘got’s ability to parse JSON responses seamlessly. By specifying { responseType: 'json' }
, ‘got’ takes care of parsing, reducing boilerplate code and potential errors.
Managing Redirects
Redirects are common in HTTP requests. Here’s how ‘got’ handles them:
const got = require('got');
(async () => {
const response = await got('https://api.example.com/redirect', { followRedirect: false });
console.log('Redirected to:', response.headers.location);
})();
# Output:
# 'Redirected to: https://api.example.com/new-location'
By setting { followRedirect: false }
, ‘got’ will not follow redirects automatically. Instead, it provides the location header of the redirect, giving you control over how to handle it. This feature is particularly useful for tracking redirect chains or handling specific redirect scenarios.
Understanding these troubleshooting techniques and considerations will empower you to use ‘got’ more effectively in your Node.js projects, ensuring smoother development and more robust applications.
The Role of HTTP Requests
HTTP requests are the backbone of web development, facilitating communication between clients and servers. Every time you visit a website, submit a form, or interact with a web application, HTTP requests are at work behind the scenes. Understanding these requests is crucial for developers, as they are fundamental to fetching data, submitting data, and interacting with APIs.
Why Use Libraries like ‘got’?
While Node.js includes the native http
module to handle HTTP requests, libraries like ‘got’ offer a more intuitive and feature-rich interface. Here’s a comparison to illustrate the difference:
// Using Node.js' native http module
const http = require('http');
http.get('http://example.com', (resp) => {
let data = '';
// A chunk of data has been received.
resp.on('data', (chunk) => {
data += chunk;
});
// The whole response has been received.
resp.on('end', () => {
console.log(data);
});
}).on("error", (err) => {
console.log("Error: " + err.message);
});
# Output:
# 'The HTML content of example.com'
This code block demonstrates fetching data using Node.js’ native http
module. While effective, the process involves handling streams and manually concatenating data chunks, which can be cumbersome and error-prone.
Now, let’s see how ‘got’ simplifies this:
// Using 'got'
const got = require('got');
(async () => {
try {
const response = await got('http://example.com');
console.log(response.body);
} catch (error) {
console.error('Error:', error);
}
})();
# Output:
# 'The HTML content of example.com'
In this example, ‘got’ abstracts the complexities of handling HTTP requests, offering a more readable and concise syntax. The library automatically handles data streams and errors, making the developer’s job significantly easier.
Understanding the fundamentals of HTTP requests and the advantages of using libraries like ‘got’ empowers developers to build more efficient and robust web applications. By simplifying common tasks and providing advanced features, ‘got’ becomes an invaluable tool in a developer’s arsenal, especially when dealing with the intricacies of web communication.
Integrating ‘got’ into Larger Projects
As your familiarity with ‘got’ grows, you’ll find it increasingly useful in larger Node.js applications. Its simplicity and powerful features can significantly streamline handling HTTP requests, whether you’re building APIs, web scrapers, or complex data-driven applications.
Streamlining API Development with ‘got’
When developing APIs, ‘got’ can be used to interact with other services seamlessly. Here’s an example of using ‘got’ to make concurrent requests to multiple APIs and aggregate the results:
const got = require('got');
(async () => {
try {
const [userResponse, weatherResponse] = await Promise.all([
got('https://api.example.com/users/1', { responseType: 'json' }),
got('https://api.weather.com/current', { responseType: 'json' })
]);
console.log('User:', userResponse.body, 'Weather:', weatherResponse.body);
} catch (error) {
console.error('Error:', error);
}
})();
# Output:
# 'User: {"name": "John Doe", "age": 30} Weather: {"temp": 68, "condition": "Sunny"}'
This code demonstrates the power of ‘got’ in handling concurrent HTTP requests efficiently. By leveraging JavaScript’s Promise.all
, we can make multiple requests in parallel, significantly speeding up the data retrieval process for applications that rely on external APIs.
Exploring Related Libraries
While ‘got’ is a fantastic tool, exploring related libraries can provide additional perspectives and tools for your projects. Libraries such as ‘axios’ for full-stack JavaScript applications, ‘node-fetch’ for a window.fetch-like API, and ‘superagent’ for more complex request handling scenarios offer diverse options for developers.
Further Resources for Mastering ‘got’
To deepen your understanding and mastery of ‘got’ and HTTP requests in Node.js, consider exploring the following resources:
- The official ‘got’ GitHub repository for detailed documentation and examples.
Node.js Design Patterns for advanced techniques in Node.js, including HTTP request handling.
The Art of Node for a broader understanding of Node.js concepts and best practices.
These resources offer a wealth of information for both beginners and experienced developers looking to expand their web development skills. By integrating ‘got’ into your projects and exploring further resources, you can enhance your capabilities and create more efficient, robust applications.
Recap: HTTP Requests with ‘got’
In this comprehensive guide, we’ve explored the ‘got’ library, a powerful and intuitive tool for making HTTP requests in Node.js applications. From simple GET and POST requests to handling more complex scenarios with retries, timeouts, and streams, ‘got’ offers a versatile solution for web developers.
We began with the basics of installing ‘got’ and making our first HTTP requests. This foundation set the stage for diving into more advanced features, such as error handling, response parsing, and managing redirects, which are essential for robust application development.
Next, we explored the advanced capabilities of ‘got’, including setting up retries for unreliable networks, configuring timeouts to avoid hanging requests, and leveraging streams for efficient data handling. These features underscore ‘got’s flexibility and power in managing HTTP communications.
While ‘got’ stands out for its simplicity and feature-rich interface, we also considered alternative libraries like ‘axios’ and ‘request’. Understanding the differences and use cases for each tool helps developers make informed decisions based on their specific project needs.
Library | Simplicity | Advanced Features | Use Case |
---|---|---|---|
‘got’ | High | Comprehensive | Node.js HTTP requests |
‘axios’ | Moderate | Full-stack (Node.js & browser) | Full-stack applications |
‘request’ (Deprecated) | Moderate | Basic | Historical context |
Whether you’re just starting with ‘got’ or looking to enhance your HTTP request handling, this guide has provided the insights and examples needed to leverage ‘got’s capabilities fully. With its balance of ease of use and advanced features, ‘got’ is a valuable addition to any Node.js developer’s toolkit.
Embrace the simplicity and power of ‘got’ for your next project, and enjoy the streamlined process of making HTTP requests. Happy coding!