NPM TypeScript Package | Quick Setup Guide
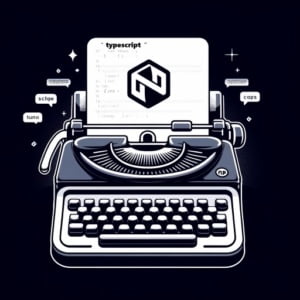
In our experience developing software for IOFLOOD, maintaining code quality and reducing errors is crucial. We’ve found that using TypeScript with npm enables static typing and modern JavaScript features, which is great for reliable and scalable applications. To assist those developing on our hosted services, we will be addressing the ‘typescript’ package in today’s informative article.
This guide will walk you through the essentials of using npm to manage your TypeScript projects, from installation to advanced package management. By the end of this journey, you’ll have a solid understanding of how to leverage npm in your TypeScript projects, making your development process more efficient and your applications more robust.
Let’s dive into how to integrate npm with TypeScript and build scalable applications with confidence!
TL;DR: How Do I Use npm with TypeScript?
To use npm with TypeScript, start by installing TypeScript globally via npm with
npm install -g typescript
. Then, initialize your project withtsc --init
to create a tsconfig.json file.
Here’s a basic example of compiling a TypeScript file using npm scripts:
"scripts": {
"build": "tsc"
}
# Output:
# Successfully compiled TypeScript project.
In this example, we’ve added a script named build
to the package.json
file. When you run npm run build
, it triggers the TypeScript compiler (tsc
) to compile the project based on the settings in tsconfig.json
.
This is a basic way to use npm with TypeScript, but there’s much more to explore about managing TypeScript projects with npm. Continue reading for a deeper dive into npm features and how they can streamline your TypeScript development.
Table of Contents
The Basics: TypeScript & npm
Embarking on your TypeScript journey begins with understanding how to effectively use npm, the Node Package Manager, to set up and manage your TypeScript projects. npm acts as a bridge, connecting you to a vast repository of packages and tools that can significantly enhance your development workflow. Let’s dive into the basics of getting started with TypeScript using npm.
Installing TypeScript via npm
The first step is to install TypeScript. This can be easily done through npm by running the following command in your terminal:
npm install typescript --save-dev
# Output:
# + typescript@latest
This command installs TypeScript as a development dependency in your project, ensuring that it’s available for compiling your TypeScript files but not included in the production build. It’s a crucial step for keeping your development environment clean and focused.
Setting Up a Basic TypeScript Project
Once TypeScript is installed, you’re ready to set up your project. This involves creating a tsconfig.json
file, which is the heart of a TypeScript project. It tells the TypeScript compiler how to handle your code. Initialize your project by running:
tsc --init
# Output:
# message TS6071: Successfully created a tsconfig.json file.
This command generates a tsconfig.json
file with default settings, which you can customize according to your project’s needs. It’s a pivotal moment in your journey, as it marks the transition from setup to actual development.
Your First TypeScript Code
With your environment set up, it’s time to write some TypeScript. Create a simple file named hello.ts
with the following content:
function greet(person: string): void {
console.log(`Hello, ${person}!`);
}
greet('World');
# Output:
# Hello, World!
This example demonstrates a basic TypeScript function that takes a string parameter and logs a greeting to the console. Compiling this file is straightforward with an npm script. Add the following line to your package.json
in the scripts
section:
"build": "tsc hello.ts"
Running npm run build
will compile your hello.ts
file to JavaScript, ready to be executed in any JavaScript environment. This process illustrates the seamless integration between TypeScript and npm, highlighting the ease with which you can start building robust applications.
By mastering these initial steps, you’ve laid the foundation for your TypeScript development with npm. The journey ahead is filled with learning and discovery as you explore more advanced features and techniques.
Advanced npm Features for TypeScript
As you become more comfortable with TypeScript and npm, it’s time to explore the advanced capabilities that can further optimize your development process. npm isn’t just for installing packages; it’s a powerful tool for managing dependencies, automating tasks, and integrating with other tools. Let’s delve into some intermediate-level techniques that will elevate your TypeScript projects.
Managing Dependencies Like a Pro
One of the key strengths of npm is its ability to manage project dependencies efficiently. For instance, when working on a TypeScript project, you might need to install a type definition for a library. This can be done using the @types
scope. Here’s how you would add type definitions for Node.js:
npm install @types/node --save-dev
# Output:
# + @types/node@latest
This command adds the Node.js type definitions to your project, allowing TypeScript to understand and compile code that uses Node.js features. It’s an essential step for projects that intend to run on a Node.js environment.
Automation with npm Scripts
npm scripts can transform your workflow by automating repetitive tasks. An advanced use case involves compiling TypeScript files and watching for changes. Here’s a more complex npm script setup in your package.json
:
"scripts": {
"build": "tsc",
"watch": "tsc --watch"
}
Running npm run watch
will initiate the TypeScript compiler in watch mode, automatically recompiling your files whenever they’re modified. This feature is invaluable for development, as it saves time and increases productivity by eliminating the need to manually recompile after each change.
Integrating with Build Tools
Beyond npm scripts, integrating TypeScript with build tools like Webpack can streamline the build process for complex projects. For example, setting up TypeScript with Webpack involves adding a ts-loader
to your Webpack configuration, which allows Webpack to compile TypeScript files as part of the build process. Here’s a basic snippet from a webpack.config.js
that incorporates ts-loader
:
module.exports = {
module: {
rules: [
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/
}
]
}
};
This configuration tells Webpack to use ts-loader
for any files ending in .ts
or .tsx
, excluding anything in the node_modules
directory. It’s a powerful setup that leverages npm’s ecosystem to handle TypeScript compilation within a broader build process, making your projects more scalable and maintainable.
By mastering these advanced npm features and integration techniques, you’re well on your way to becoming a TypeScript and npm expert. The ability to manage dependencies effectively, automate tasks, and integrate with other tools will significantly enhance your development workflow, making your projects more efficient and your code more reliable.
Alternate Packaging Tools
While npm is a powerhouse for managing TypeScript projects, it’s not the only player in the field. As developers seek efficiency and performance, exploring alternative tools like Yarn for package management and Webpack for module bundling becomes essential. Understanding these alternatives can help you make informed decisions based on your project’s specific needs.
Yarn: A Speedy npm Alternative
Yarn emerged as a strong contender to npm, boasting faster package installation times and improved reliability. For TypeScript projects, Yarn offers seamless integration much like npm. Here’s how you would initiate a new TypeScript project with Yarn:
yarn add typescript
# Output:
# [1/4] Resolving packages...
# [2/4] Fetching packages...
# [3/4] Linking dependencies...
# [4/4] Building fresh packages...
# success Saved lockfile.
# success Saved 1 new dependency.
This command adds TypeScript to your project, similar to npm but with Yarn’s unique performance optimizations. The output messages provide a clear insight into the process, highlighting Yarn’s efficient handling of dependencies.
Webpack: Beyond Simple Compilation
Webpack takes your TypeScript project to the next level by bundling your modules into static assets. This is especially useful for large-scale applications that benefit from optimized loading times. Integrating TypeScript with Webpack requires adding ts-loader
, as mentioned before, but within the context of Webpack’s powerful ecosystem. Here’s a snippet to include ts-loader
in your Webpack configuration:
module.exports = {
entry: './src/index.ts',
module: {
rules: [
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/
}
]
},
resolve: {
extensions: ['.tsx', '.ts', '.js']
},
output: {
filename: 'bundle.js',
path: __dirname + '/dist'
}
};
This configuration not only compiles TypeScript files but also bundles them, optimizing your project for production. The entry
and output
properties define where Webpack starts processing your files and where the bundled files will be placed, respectively.
Making the Right Choice
Choosing between npm, Yarn, and Webpack depends on your project’s size, complexity, and specific requirements. For straightforward TypeScript compilation and dependency management, npm and Yarn are both excellent choices. However, for projects that require advanced module bundling and optimization, integrating Webpack into your workflow can provide significant benefits.
By considering these alternatives, you can tailor your development process to suit your TypeScript project’s needs, ensuring efficiency, performance, and scalability.
Even with the best planning, developers might encounter issues when using npm with TypeScript. Understanding how to troubleshoot these problems is crucial for maintaining an efficient development workflow. Let’s explore common hurdles and their solutions.
Resolving Dependency Conflicts
Dependency conflicts can occur when multiple packages require different versions of the same dependency. This is a common scenario in active development environments. Here’s how to identify and resolve these conflicts using npm’s list
command:
npm list <dependency-name>
# Output:
# project-name@version
# └─┬ dependency-name@version
# └── conflicting-dependency@version
This command displays the dependency tree for a specific package, helping you identify where conflicts arise. Resolving these conflicts might involve updating packages to compatible versions or using npm’s dedupe
command to flatten dependency trees.
TypeScript Compiler Errors
TypeScript compiler errors can range from simple syntax mistakes to complex type mismatches. When faced with a compiler error, first ensure that your tsconfig.json
is correctly configured. Here’s an example of adjusting your tsconfig.json
to include strict type checking:
{
"compilerOptions": {
"strict": true,
...
}
}
Enabling strict type checking can help catch errors early in the development process. If errors persist, consult the TypeScript documentation or community forums for specific solutions. Understanding the nature of these errors is key to resolving them efficiently.
Debugging Tips for npm and TypeScript
Debugging is an integral part of the development process. When debugging TypeScript code, source maps are invaluable as they map the compiled JavaScript back to your original TypeScript source. Ensure your tsconfig.json
includes source map generation:
{
"compilerOptions": {
"sourceMap": true
}
}
With source maps enabled, you can use debugging tools to step through your TypeScript code, making it easier to identify and fix issues. Additionally, leveraging npm’s run
command to script common tasks, such as linting and testing, can help catch errors early.
By understanding these common issues and how to address them, developers can mitigate problems and maintain a productive development environment. Remember, the key to successful troubleshooting is a solid grasp of npm and TypeScript fundamentals, coupled with a methodical approach to problem-solving.
Understanding npm and TypeScript
To fully harness the power of npm with TypeScript, a solid understanding of the fundamentals is essential. npm isn’t just a tool for installing packages; it’s a comprehensive package manager that facilitates version control, dependency management, and script running for your projects. TypeScript, on the other hand, is a statically typed superset of JavaScript that compiles to plain JavaScript, offering optional static typing, classes, and interfaces.
Understanding npm
npm, or Node Package Manager, is the backbone of modern web development. It interacts with a vast repository of packages, allowing developers to install, update, and manage dependencies with ease. The core of npm’s functionality in a project is the package.json
file. This file keeps track of all the metadata related to your project, including dependencies, scripts, and version information. Here’s a basic example of a package.json
file:
{
"name": "my-typescript-project",
"version": "1.0.0",
"scripts": {
"build": "tsc"
},
"dependencies": {
"typescript": "^4.0.0"
}
}
# Output:
# This does not produce a command line output, but it's the blueprint of your project settings and dependencies.
This snippet shows a minimal package.json
setup for a TypeScript project. The scripts
section defines custom commands, like build
, which compiles the project’s TypeScript files. The dependencies
section lists the packages your project needs, with versions.
The Power of TypeScript
TypeScript enhances JavaScript by adding types, which can catch errors at compile time, before the code runs. This can significantly reduce runtime errors, making your code more robust and maintainable. Here’s a simple TypeScript example demonstrating the use of types:
function addNumbers(a: number, b: number): number {
return a + b;
}
const sum = addNumbers(2, 3);
# Output:
# 5
This function addNumbers
is defined with two parameters, a
and b
, both of which are expected to be numbers. The function returns a number as well. This example illustrates TypeScript’s capability to enforce types, ensuring that the function is used correctly throughout your codebase.
Understanding these fundamentals of npm and TypeScript not only sets the foundation for efficient development but also unlocks the potential to build more complex and reliable applications. As you delve deeper into npm and TypeScript, remember that these tools are designed to work together to streamline your development process, making it easier to manage dependencies, catch errors early, and maintain high standards of code quality.
Practical Usage: TypeScript with npm
As you grow more comfortable with TypeScript and npm, you’ll discover that the ecosystem around these tools is vast and full of opportunities for further exploration. From powerful libraries and frameworks designed to work seamlessly with TypeScript to advanced npm packages that can simplify your workflow, the potential for expanding your TypeScript projects is limitless.
Leveraging Popular Libraries and Frameworks
TypeScript’s compatibility with JavaScript means you can take advantage of a wide range of libraries and frameworks to enhance your projects. Libraries like Lodash for utility functions or Axios for HTTP requests become even more powerful when their types are clearly defined. Here’s how you might use Axios with TypeScript for making HTTP requests:
import axios from 'axios';
async function getUser() {
try {
const response = await axios.get('https://api.example.com/user');
console.log(response.data);
} catch (error) {
console.error('Error fetching user:', error);
}
}
getUser();
# Output:
# {"name":"John Doe","id":1}
In this example, Axios is used to fetch user data from an API, with TypeScript ensuring that the handling of the response is type-safe. This integration showcases the synergy between npm packages and TypeScript’s type system, enabling more reliable and maintainable code.
Tools That Complement TypeScript Development
Beyond libraries, several tools are specifically designed to enhance TypeScript development. For instance, TSLint (now deprecated in favor of ESLint with TypeScript support) helps maintain code quality, while ts-node allows you to run TypeScript files directly without pre-compiling. These tools, installed via npm, integrate smoothly into your TypeScript projects, streamlining development and ensuring code quality.
Further Resources for Advancing with npm and TypeScript
To continue your journey with npm and TypeScript, consider exploring these resources:
- The official TypeScript documentation offers comprehensive guides and tutorials for developers of all levels.
Explore the npm documentation and learn more about npm and how to leverage its full potential in your projects.
Check out Awesome TypeScript on GitHub, a curated list of awesome TypeScript frameworks, libraries, tools, and software. This is a great starting point for discovering new packages and resources. .
These resources are just the beginning. The TypeScript and npm ecosystems are constantly evolving, offering new tools, libraries, and best practices to explore. By staying curious and continuously learning, you can take your TypeScript projects to new heights.
Recap: npm TypeScript Guide
In our comprehensive exploration, we’ve tackled the essentials and intricacies of using npm to manage TypeScript projects. From the initial setup of your TypeScript environment to leveraging npm’s powerful features for advanced project management, we’ve covered a broad spectrum to equip you with the knowledge you need.
We began with the basics, illustrating how to install TypeScript globally and initialize your project. We delved into compiling TypeScript code using npm scripts, providing a solid foundation for beginners. Moving forward, we explored advanced npm features, including managing dependencies and automating tasks with scripts, to enhance your TypeScript projects.
Our journey then took us beyond the basics, where we examined alternative tools like Yarn and Webpack. We compared these with npm, highlighting their benefits and use cases, to give you a broader perspective on managing TypeScript projects. This exploration was aimed at helping you make informed decisions based on your project’s needs.
Tool | Strengths | Ideal Use Case |
---|---|---|
npm | Comprehensive package management | General TypeScript project management |
Yarn | Fast and reliable | Projects requiring speed in dependency management |
Webpack | Module bundling and optimization | Large-scale applications needing optimization |
In conclusion, whether you’re just starting out with TypeScript or looking to refine your npm skills, this guide has provided a pathway for growth. We’ve seen how npm can be a powerful ally in your TypeScript development, from simple projects to complex applications.
Mastering npm with TypeScript is a journey of continuous learning and improvement. As you advance, keep exploring new packages, tools, and practices. The TypeScript and npm ecosystems are vibrant and evolving, offering endless possibilities for developers. Happy coding!