AWK NF Usage Guide | Manipulating Fields Based on Count
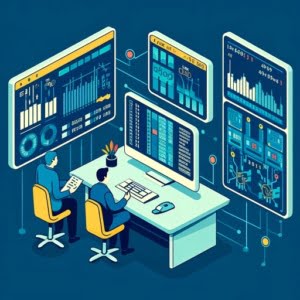
In the steady quest to improve our in-house software at IOFLOOD, we have begun to utilize AWK for various text processing tasks. When needing to access fields of data, we have found that NF, which stands for Number of Fields, plays a vital role by representing the number of fields in each input record. In this article, we’ll dive into the concept of NF in AWK, to aid our bare metal hosting customers and fellow developers in harnessing the power of NF.
This guide will navigate you through the intricacies of using the NF variable in AWK, from basic usage to advanced techniques. We’ll cover everything from understanding what NF is, how it works, to more complex uses such as manipulating fields in a record. We’ll even explore alternative approaches and discuss common issues and their solutions.
So, let’s dive in and start mastering AWK NF!
TL;DR: What is NF in AWK?
In AWK, NF, represented by
$NF
orNF
is a built-in variable that represents the total number of fields in a record.It’s like a field counter for your data records.
Here’s a simple example:
echo 'Hello World' | awk '{print $NF}'
# Output:
# 'World'
In this example, we’re using the echo command to print ‘Hello World’, and then we pipe this output to AWK. Inside AWK, we’re using the NF variable to print the last field in the record, which in this case is ‘World’.
This is just a basic usage of the NF variable in AWK. There’s much more to learn about NF, including more complex uses and alternative approaches. So, let’s dive deeper!
Table of Contents
Getting Started with AWK NF
To understand AWK NF, it’s important to understand what AWK is and what it does. AWK is a powerful text processing language. It’s often used for data extraction and reporting in shell scripting.
The NF variable in AWK is a built-in variable that holds the number of fields in a record. Essentially, it’s a counter for the number of fields in your data.
AWK NF in Action
Let’s take a look at a basic example of how the NF variable works:
echo 'Hello AWK NF' | awk '{print $NF}'
# Output:
# 'NF'
In this example, we’re using the echo command to print ‘Hello AWK NF’, and then we pipe this output to AWK. Inside AWK, we’re using the NF variable to print the last field in the record, which in this case is ‘NF’.
Advantages and Pitfalls of AWK NF
One of the main advantages of using the NF variable is that it allows you to easily count the number of fields in a record. This can be incredibly useful when working with large data sets where manual counting would be impractical. However, it’s important to note that AWK considers any space to be a field separator by default. This means that if your data includes spaces within fields, you may get unexpected results.
Let’s take a look at an example to illustrate this:
echo 'Hello AWK NF' | awk 'BEGIN {FS=" "} {print $NF}'
# Output:
# 'NF'
In this case, we’ve specified that the field separator (FS) is a space. As a result, AWK considers each word to be a separate field, and the NF variable returns ‘NF’, the last field in the record.
This is something to keep in mind when working with the NF variable in AWK. In the next section, we’ll explore more complex uses of the NF variable.
Advanced Usage of AWK NF
As we dive deeper into the world of AWK NF, we’ll encounter more complex uses of this built-in variable. NF is not just a counter; it can be a powerful tool for manipulating fields in a record.
Manipulating Fields with AWK NF
Let’s consider a scenario where we have a data record with several fields, and we want to print the last two fields. Here’s how we can achieve this using the NF variable:
echo 'Hello AWK NF Guide' | awk '{print $(NF-1), $NF}'
# Output:
# 'NF Guide'
In this code snippet, we’re using the NF variable to print the last two fields in the record. The expression $(NF-1) prints the second last field, and $NF prints the last field. As a result, the output is ‘NF Guide’.
This example illustrates the flexibility of the NF variable. It’s not just a counter; it can be a dynamic reference to any field in your data record.
Understanding AWK NF in Multi-line Records
The NF variable can also be useful when working with multi-line records. Consider a scenario where we have a file with several lines of data, and we want to print the last field of each line.
echo -e 'Hello\nAWK NF\nGuide' | awk '{print $NF}'
# Output:
# 'Hello'
# 'NF'
# 'Guide'
In this example, we’re using the echo command with the -e option to interpret backslash escapes, allowing us to print multiple lines. Then, we pipe this output to AWK, where we’re using the NF variable to print the last field of each line.
This demonstrates the power of AWK NF when dealing with multi-line records. It can simplify tasks that would otherwise require more complex code.
Exploring Alternatives to AWK NF
While AWK NF is a powerful tool for field manipulation, it’s not the only game in town. There are other built-in variables and techniques in AWK that can accomplish similar tasks.
Leveraging AWK’s NR Variable
Let’s take a look at the NR variable, which stands for ‘Number of Records’. It works similarly to NF, but instead of counting fields, NR counts records.
Here’s an example:
echo -e 'Hello\nAWK NF\nGuide' | awk 'NR==2 {print $0}'
# Output:
# 'AWK NF'
In this example, we’re using the NR variable to print the second line (or record) from the input. The $0 variable represents the entire line.
The Power of AWK Arrays
Another powerful feature of AWK is its associative arrays. These allow you to store data in key-value pairs, which can be useful for more complex data manipulation tasks.
Here’s an example of how you might use an associative array in AWK:
echo -e 'Hello\nAWK NF\nGuide' | awk '{arr[NR]=$0} END {for (i in arr) print i, arr[i]}'
# Output:
# '1 Hello'
# '2 AWK NF'
# '3 Guide'
In this example, we’re creating an array where the keys are the line numbers and the values are the lines themselves. We then print out the array in the END block, which is executed after all input has been processed.
Deciding Between AWK NF and Alternatives
Choosing between AWK NF and alternatives like NR or arrays depends on your specific use case. If you’re dealing with field manipulation within records, NF is likely your best bet. If you’re working with records themselves, NR might be more appropriate. And for complex data manipulation tasks, arrays can be incredibly powerful.
The beauty of AWK is its flexibility. With a deep understanding of its built-in variables and features, you can accomplish a wide range of data processing tasks with relative ease.
Troubleshooting Tips: AWK NF
Like any tool, AWK NF comes with its own set of challenges and potential roadblocks. Let’s explore some common issues you might encounter when using AWK NF, along with their solutions.
Dealing with Unexpected White Spaces
One common issue with AWK NF is dealing with unexpected white spaces. As AWK considers any space to be a field separator by default, it might result in unexpected field counts. Let’s see how this issue can occur:
echo 'Hello AWK NF' | awk '{print NF}'
# Output:
# 5
In this example, we have extra spaces between ‘Hello’ and ‘AWK’. As a result, AWK counts these as separate fields, and NF returns 5 instead of 3.
The Solution
To solve this issue, we can set the field separator (FS) to a single space. This tells AWK to consider only single spaces as field separators, not multiple spaces.
echo 'Hello AWK NF' | awk 'BEGIN {FS=" "} {print NF}'
# Output:
# 3
Now, AWK correctly counts three fields, despite the extra spaces.
Best Practices and Optimization Tips
When using AWK NF, there are a few best practices and optimization tips to keep in mind:
- Always be mindful of your field separators. If your data includes spaces within fields, consider setting a different field separator.
- Use the
-v
option to set variables before execution. For example,awk -v FS="," '{print NF}'
sets the field separator to a comma before executing the script. - Remember that AWK NF is case-sensitive. Make sure to use the correct case when referencing the NF variable.
By understanding these common issues and best practices, you can use AWK NF more effectively and avoid potential pitfalls.
Grasping AWK & Built-in Variables
To truly grasp the power and utility of the NF variable in AWK, it’s essential to understand the fundamentals of AWK and its built-in variables.
Understanding AWK
AWK is a versatile programming language designed for pattern scanning and processing. It’s extensively used for data extraction and reporting. One of the key features of AWK is its ability to handle data on a per-record basis. A record in AWK is typically a line of input data.
Fields: The Building Blocks of Records
Each record in AWK is divided into chunks known as fields. By default, a field is a string of characters separated by whitespace from the surrounding fields. AWK provides powerful tools to manipulate these fields, and one of these tools is the NF variable.
Delving into AWK’s NF Variable
In AWK, NF stands for ‘Number of Fields’. It’s a built-in variable that counts the number of fields in a record. This can be incredibly useful when dealing with complex data sets where manual counting would be impractical.
Let’s consider a scenario where we have a record with several fields, and we want to print the number of fields.
echo 'Hello AWK NF Guide' | awk '{print NF}'
# Output:
# 4
In this example, we’re using the echo command to print ‘Hello AWK NF Guide’, and then we pipe this output to AWK. Inside AWK, we’re using the NF variable to print the number of fields in the record. As a result, the output is ‘4’.
Understanding AWK and its built-in variables, particularly NF, can significantly enhance your data processing capabilities. As we continue to explore AWK NF in the following sections, keep these fundamentals in mind.
Practical Uses of NF
The NF variable in AWK isn’t just for small, one-off tasks. It’s also a powerful tool for larger scripts and projects. Whether you’re parsing log files or processing large datasets, NF can be instrumental in handling and manipulating data.
AWK NF in Large Scripts
In larger scripts, the NF variable can be used to parse complex data structures. For example, if you have a CSV file with varying numbers of fields per line, you can use NF to identify and handle these inconsistencies.
awk -F, '{if (NF != 5) print "Line " NR " has " NF " fields"}' data.csv
# Output:
# 'Line 4 has 6 fields'
# 'Line 7 has 4 fields'
In this example, we’re using AWK with a comma as the field separator to process a CSV file. We use the NF variable to check if each line has the expected number of fields (5 in this case). If not, it prints a message indicating the line number and the actual number of fields.
Complementary AWK Built-in Variables
In addition to NF, there are other built-in variables in AWK that can be used in conjunction with NF for more advanced data processing tasks. For example, the NR variable, which counts the number of records, can be used together with NF to provide a more detailed view of your data.
awk '{print "Line " NR " has " NF " fields"}' data.txt
# Output:
# 'Line 1 has 5 fields'
# 'Line 2 has 4 fields'
# 'Line 3 has 5 fields'
In this example, we’re using both NR and NF to print the number of fields in each line of a text file, along with the line number.
Further Resources for Mastering AWK NF
To continue your journey in mastering AWK and the NF variable, here are some additional resources you might find helpful:
- GNU Awk User’s Guide: This comprehensive guide covers all aspects of AWK, including its built-in variables like NF.
Effective AWK Programming: This book by Arnold Robbins offers a deep dive into AWK programming, including practical examples and use cases.
AWK Tutorial by The Geek Stuff: This tutorial provides a step-by-step introduction to AWK, with a focus on practical examples and exercises.
Remember, mastering a tool like AWK NF takes practice and patience. Don’t be afraid to experiment, make mistakes, and learn from them. Happy scripting!
Wrapping Up: How to Use AWK NF
In this comprehensive guide, we’ve explored the ins and outs of AWK NF, a built-in variable in AWK that represents the total number of fields in a record.
We began with the basics, understanding what NF is and how it works in AWK. We then delved into more advanced usage, exploring how NF can be used for more complex tasks such as manipulating fields within a record and handling multi-line records. Along the way, we tackled common issues you might encounter when using AWK NF, such as dealing with unexpected white spaces, and provided solutions to help you navigate these challenges.
We also took a look at alternative approaches to field manipulation in AWK, discussing other built-in variables like NR and the use of arrays. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
AWK NF | High | Low | Counting and referencing fields in a record |
AWK NR | Moderate | Low | Counting and referencing records |
AWK Arrays | High | High | Complex data manipulation tasks |
Whether you’re just starting out with AWK or looking to deepen your understanding of its built-in variables, we hope this guide has provided you with a deeper understanding of AWK NF and its capabilities.
With its flexibility and power, AWK NF is an invaluable tool for field manipulation in AWK. We hope this guide has empowered you to use AWK NF more effectively in your future projects. Happy scripting!