What is Shebang in Bash? | Your Script Interpreter Guide
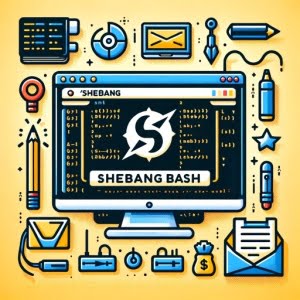
Ever scratched your head over the ‘#!’ at the beginning of a bash script? You’re not alone. Many developers overlook this small but crucial component of bash scripting. Think of the shebang line as a backstage director – setting the stage for the script’s execution, guiding the performance from behind the scenes.
This guide will delve into the purpose and usage of the shebang in bash scripting. We’ll explore the shebang’s core functionality, delve into its advanced usage, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the shebang in bash scripting!
TL;DR: What is the Shebang in Bash Scripting?
The shebang
'#!'
at the start of a bash script specifies the interpreter for the script, for example,#!/bin/bash
. It’s a directive to the system indicating which interpreter to use when executing the script.
Here’s a simple example:
#!/bin/bash
echo 'Hello, world!'
# Output:
# Hello, world!
In this example, the shebang line is #!/bin/bash
. This tells the system to use the bash shell to interpret the script. The script then executes the echo
command, printing ‘Hello, world!’ to the console.
This is a basic use of the shebang in bash scripting, but there’s much more to learn about its advanced usage and related concepts. Continue reading for more detailed information and examples.
Table of Contents
- A Deep Dive into the Shebang Line
- Expanding the Shebang: Different Interpreters
- Exploring Alternatives: The ‘exec’ Command
- Navigating Shebang Pitfalls: Common Issues and Solutions
- Delving into Script Interpreters
- Understanding File Permissions and Executable Scripts
- The Shebang Line in Larger Projects
- Going Beyond the Shebang in Bash Scripting
- Wrapping Up: Mastering the Shebang (#!) in Bash Scripting
A Deep Dive into the Shebang Line
The shebang line, also referred to as the hashbang, is a special kind of comment. It’s the first line in a script, starting with ‘#!’ followed by the path to the interpreter. The purpose of this line is to tell the system what interpreter to use to execute the script.
For instance, if you’re writing a bash script, the shebang line would look like this:
#!/bin/bash
Let’s look at a simple script that uses the shebang line:
#!/bin/bash
echo 'This is a bash script'
# Output:
# This is a bash script
In this script, #!/bin/bash
is the shebang line. This tells the system to use the bash shell to interpret the script. The script then executes the echo
command, printing ‘This is a bash script’ to the console.
The shebang line is crucial because it ensures that your script is executed with the correct interpreter, regardless of the current shell of the user. Without the shebang line, the script might not work as intended if the user’s default shell is not bash.
Expanding the Shebang: Different Interpreters
The shebang line is not limited to bash. You can specify any interpreter available on your system. This is where the shebang line becomes truly powerful, allowing you to write scripts in different languages and ensuring they are interpreted correctly.
For example, if you’re writing a script in Python, the shebang line would look like this:
#!/usr/bin/env python
print('This is a Python script')
# Output:
# This is a Python script
Here, #!/usr/bin/env python
is the shebang line, which tells the system to use Python to interpret the script. The script then executes the print
command, displaying ‘This is a Python script’ in the console.
Another common interpreter is /bin/sh
, which is often used for shell scripts:
#!/bin/sh
echo 'This is a shell script'
# Output:
# This is a shell script
In this case, #!/bin/sh
is the shebang line. The script then executes the echo
command, printing ‘This is a shell script’ to the console.
The key takeaway here is that the shebang line provides flexibility in scripting. You can write scripts in different languages and ensure they are interpreted correctly by specifying the appropriate interpreter in the shebang line.
Exploring Alternatives: The ‘exec’ Command
While the shebang line is a powerful tool in scripting, there are alternative approaches to achieve the same goal. One such approach is using the ‘exec’ command. The ‘exec’ command replaces the current shell process with a new process specified by the command argument.
For instance, consider a bash script without a shebang line:
exec /bin/bash
echo 'This is a bash script using exec'
# Output:
# This is a bash script using exec
Here, we use the ‘exec’ command to specify the interpreter. The ‘exec /bin/bash’ line replaces the current shell process with a bash process. The script then executes the ‘echo’ command, printing ‘This is a bash script using exec’ to the console.
While this approach works, it comes with a caveat. The ‘exec’ command terminates the current shell process, which may not be desirable in certain scenarios, such as when you want to run multiple scripts in the same shell process. On the other hand, the shebang line does not terminate the current shell process, making it a more versatile tool in scripting.
In conclusion, while the ‘exec’ command provides an alternative way to specify the interpreter, the shebang line offers more flexibility and is generally preferred in most scripting scenarios.
While the shebang line is a powerful tool in bash scripting, it can sometimes lead to unexpected issues. Let’s discuss some common issues related to the shebang line and how to resolve them.
Incorrect Interpreter Paths
One common issue is specifying an incorrect path to the interpreter in the shebang line. For instance, if you specify #!/bin/bash
but bash is not located in /bin
on your system, the script will fail to execute.
#!/bin/wrongpath/bash
echo 'Hello, world!'
# Output:
# bash: ./script.sh: /bin/wrongpath/bash: bad interpreter: No such file or directory
In this example, the shebang line is incorrect because bash is not located in /bin/wrongpath
. As a result, the script fails to execute and the system outputs an error message.
The solution is to ensure that the path to the interpreter in the shebang line is correct. You can use the which
command to find the correct path to the interpreter:
which bash
# Output:
# /bin/bash
Permissions Issues
Another common issue is not having the necessary permissions to execute the script. Even if the shebang line is correct, the script will fail to execute if it does not have execute permissions.
#!/bin/bash
echo 'Hello, world!'
# Trying to run the script:
# bash: ./script.sh: Permission denied
In this case, the script fails to execute because it does not have execute permissions. The solution is to use the chmod
command to give the script execute permissions:
chmod +x script.sh
After running this command, the script should have the necessary permissions to execute.
These are just a few examples of the common issues you might encounter when using the shebang line in bash scripting. The key is to understand the purpose of the shebang line and how it works, which will enable you to troubleshoot and resolve any issues that arise.
Delving into Script Interpreters
To fully understand the shebang line’s significance, we need to delve into the concept of script interpreters. An interpreter is a program that reads and executes code. In the context of bash scripting, the interpreter reads your script line by line and executes the commands.
Consider a simple bash script:
echo 'Hello, world!'
# Output:
# Hello, world!
In this script, echo 'Hello, world!'
is a command that the bash interpreter reads and executes, printing ‘Hello, world!’ to the console.
The shebang line tells the system which interpreter to use to execute the script. This is crucial because different interpreters can interpret the same script differently. By specifying the interpreter in the shebang line, you ensure that your script is interpreted as intended, regardless of the user’s default shell.
Understanding File Permissions and Executable Scripts
Another related concept is file permissions. In Unix-like systems, file permissions determine who can read, write, or execute a file. For a script to be executable, it must have execute permissions.
You can use the chmod
command to give a script execute permissions:
chmod +x script.sh
After running this command, script.sh
has execute permissions and can be run as a script.
./script.sh
# Output:
# Hello, world!
In this example, ./script.sh
runs script.sh
as a script, using the interpreter specified in the shebang line. The script then executes the echo
command, printing ‘Hello, world!’ to the console.
In conclusion, the shebang line, script interpreters, and file permissions are interconnected concepts in bash scripting. Understanding these concepts is key to mastering bash scripting and troubleshooting any issues that arise.
The Shebang Line in Larger Projects
The shebang line might seem like a minor detail in scripting, but its relevance grows as your scripts become more complex and diverse. In larger scripts or projects, the shebang line ensures that your scripts are interpreted correctly, regardless of the user’s environment or default shell.
Imagine working on a project that uses scripts written in different languages. Without the shebang line, you would have to manually specify the interpreter each time you run a script. With the shebang line, you can run any script with the confidence that it will be interpreted with the correct interpreter.
Going Beyond the Shebang in Bash Scripting
Mastering the shebang line is just one step in your journey to becoming a bash scripting expert. There are many other advanced techniques to explore, such as writing functions, handling user input, and error handling. Each of these techniques will make your scripts more robust and versatile.
Further Resources for Bash Scripting Mastery
Ready to take your bash scripting skills to the next level? Here are some resources that provide in-depth information and tutorials on bash scripting:
- Advanced Bash-Scripting Guide: This guide covers a wide range of topics, from basic syntax to advanced features.
Bash Scripting Tutorial: This tutorial provides a hands-on approach to learning bash scripting, with plenty of examples and exercises.
Google’s Shell Style Guide: This style guide provides best practices for writing clean and maintainable scripts.
Wrapping Up: Mastering the Shebang (#!) in Bash Scripting
In this comprehensive guide, we’ve delved into the world of the shebang line in bash scripting, exploring its purpose, usage, and significance.
We started with the basics, unveiling the mystery behind the ‘#!’ at the beginning of a bash script. We then journeyed into more advanced territory, discussing different interpreters that can be specified in the shebang line and alternative approaches like using the ‘exec’ command.
Along the way, we tackled common issues related to the shebang line, such as incorrect interpreter paths and permissions issues, providing solutions to help you navigate these pitfalls.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Shebang | Versatile, supports different interpreters | Requires correct interpreter path |
Exec | Direct, replaces current shell process | Terminates current shell process |
Whether you’re just starting out with bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the shebang line and its importance in bash scripting.
The shebang line might seem like a minor detail, but as we’ve seen, it plays a crucial role in ensuring that your scripts are interpreted correctly. Now, you’re well equipped to leverage the power of the shebang line in your bash scripts. Happy scripting!