How-To Append to a File | Bash File Editing Guide
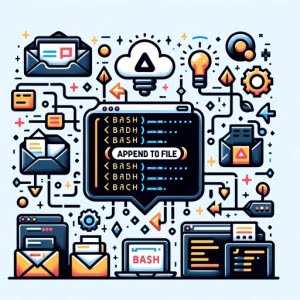
Are you finding it difficult to append data to a file using Bash? You’re not alone. Many developers find this task a bit challenging, but there’s a simple command in Bash that can make this process straightforward.
Think of Bash’s append command as a diligent scribe, able to easily add information to the end of a file without disturbing the existing content. This feature is incredibly useful for logging, data storage, and more.
This guide will walk you through the process of appending to a file in Bash, from the basics to more advanced techniques. We’ll cover everything from using the ‘>>’ operator, handling different types of data, to even troubleshooting common issues.
So, let’s dive in and start mastering the art of appending to a file in Bash!
TL;DR: How Do I Append to a File in Bash?
To append to a file in Bash, you can use the
'>>'
operator with the syntax,echo 'Text to Append' >> file.txt
. This operator allows you to add data to the end of a file without overwriting the existing content.
Here’s a simple example:
echo 'Hello, World!' >> file.txt
# Output:
# This will append 'Hello, World!' to the end of file.txt.
In this example, we use the ‘echo’ command to generate the string ‘Hello, World!’, and then the ‘>>’ operator to append this string to the end of the file named ‘file.txt’. If ‘file.txt’ doesn’t exist, Bash will create it for us.
This is just a basic way to append to a file in Bash, but there’s much more to learn about file handling in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the ‘>>’ Operator in Bash
- Advantages and Potential Pitfalls of the ‘>>’ Operator
- Appending Data from One File to Another
- Appending Command Output to a File
- Appending Data Within a Script
- Exploring Alternative Commands for Appending in Bash
- Troubleshooting Bash Append Issues
- Optimizing Bash Appends
- Understanding the Bash Shell
- File Handling in Unix/Linux
- The Concept of Redirection in Bash
- Expanding Your Bash Skills: Beyond Appending to a File
- Wrapping Up: Mastering Bash Append to File
Understanding the ‘>>’ Operator in Bash
In Bash, the ‘>>’ operator is used to append data to a file. It’s a simple yet powerful tool that can make your data handling tasks more efficient.
Let’s take a look at a basic example of how this operator works:
echo 'Bash is awesome!' >> myfile.txt
cat myfile.txt
# Output:
# Bash is awesome!
In this code block, we’re using the ‘echo’ command to generate a string ‘Bash is awesome!’. Then, we’re using the ‘>>’ operator to append this string to the end of a file named ‘myfile.txt’. If ‘myfile.txt’ doesn’t exist, Bash will create it for us. The ‘cat’ command is then used to display the contents of ‘myfile.txt’, confirming that our string was indeed appended.
Advantages and Potential Pitfalls of the ‘>>’ Operator
One major advantage of the ‘>>’ operator is that it allows you to add data to a file without overwriting any existing content. This makes it ideal for tasks such as logging, where you want to keep a record of events over time.
However, there can be potential pitfalls. For example, if you don’t have write permissions for the file you’re trying to append to, Bash will throw an error. It’s crucial to ensure that you have the necessary permissions before attempting to append data to a file.
Appending Data from One File to Another
In Bash, you can append the contents of one file to the end of another file. Here’s an example:
echo 'Hello, Bash!' > file1.txt
echo 'How are you?' > file2.txt
cat file1.txt file2.txt > file3.txt
cat file3.txt
# Output:
# Hello, Bash!
# How are you?
In this example, we first create two files, ‘file1.txt’ and ‘file2.txt’, each with a different string. Then, we use the ‘cat’ command and the ‘>’ operator to concatenate the contents of ‘file1.txt’ and ‘file2.txt’ and write them to a new file, ‘file3.txt’.
Appending Command Output to a File
You can also append the output of a command to a file. This is particularly useful for logging purposes. Here’s how you can do it:
ls -l >> log.txt
cat log.txt
# Output:
# total 0
# -rw-r--r-- 1 user group 0 Jan 1 00:00 file1.txt
# -rw-r--r-- 1 user group 0 Jan 1 00:00 file2.txt
# -rw-r--r-- 1 user group 0 Jan 1 00:00 log.txt
In this scenario, we’re appending the output of the ‘ls -l’ command, which lists files in the current directory, to a file named ‘log.txt’.
Appending Data Within a Script
Appending data to a file is a common operation in Bash scripting. Here’s an example of how it can be done:
#!/bin/bash
for i in {1..5}
do
echo "Appending line $i" >> script_output.txt
done
cat script_output.txt
# Output:
# Appending line 1
# Appending line 2
# Appending line 3
# Appending line 4
# Appending line 5
In this script, we’re using a for loop to append a series of lines to a file named ‘script_output.txt’. Each line contains the phrase ‘Appending line’ followed by the current iteration number.
Exploring Alternative Commands for Appending in Bash
While the ‘>>’ operator is the most straightforward way to append data to a file in Bash, there are other commands and functions that can achieve the same result. Let’s explore some of these alternatives.
Using ‘tee -a’ to Append Data
The ‘tee’ command is primarily used to read from standard input and write to standard output and files. When used with the ‘-a’ option, ‘tee’ appends the input to the given files rather than overwriting them. Here’s an example:
echo 'Hello, Bash!' | tee -a file.txt
cat file.txt
# Output:
# Hello, Bash!
In this example, we’re using the ‘echo’ command to generate a string ‘Hello, Bash!’, then piping this string to the ‘tee -a’ command to append it to ‘file.txt’.
Appending Data with ‘printf’
The ‘printf’ command can also be used to append data to a file. It’s a more flexible alternative to ‘echo’, allowing for better string formatting and control. Here’s how you can use ‘printf’ to append data:
printf 'Hello, %s!
' 'Bash' >> file.txt
cat file.txt
# Output:
# Hello, Bash!
In this scenario, we’re using ‘printf’ to format a string and append it to ‘file.txt’. The ‘%s’ in the string is a placeholder that gets replaced by ‘Bash’.
Combining ‘cat’ with Redirection for Appending
The ‘cat’ command, combined with the ‘>>’ operator, can append the contents of one file to another. This is particularly useful when you need to concatenate files:
cat file1.txt >> file2.txt
cat file2.txt
# Output:
# Contents of file1.txt appended to file2.txt
In this example, we’re using ‘cat’ to read ‘file1.txt’ and then the ‘>>’ operator to append this content to ‘file2.txt’.
While these alternative commands can provide more flexibility in certain scenarios, the ‘>>’ operator remains the go-to choice for appending to a file in Bash due to its simplicity and efficiency.
Troubleshooting Bash Append Issues
While appending data to a file in Bash is generally straightforward, you might occasionally run into issues. Let’s explore some common problems and their solutions.
Permission Denied Error
One of the most common errors you might encounter is the ‘Permission denied’ error. This error occurs when you don’t have write permissions for the file you’re trying to append to.
echo 'Hello, Bash!' >> /root/file.txt
# Output:
# bash: /root/file.txt: Permission denied
In this example, we’re trying to append a string to a file in the ‘/root’ directory, which typically requires superuser permissions. Since we’re not running the command as a superuser, Bash throws a ‘Permission denied’ error.
The solution is to either run the command as a superuser using ‘sudo’ or to append to a file in a directory where you have write permissions.
No Space Left on Device Error
Another common issue is the ‘No space left on device’ error, which occurs when there’s insufficient disk space to write to the file.
echo 'Hello, Bash!' >> file.txt
# Output:
# bash: echo: write error: No space left on device
In this scenario, the solution is to free up some disk space. You can do this by deleting unnecessary files or moving some files to another storage device.
Optimizing Bash Appends
When appending data to a file in Bash, it’s important to consider performance. If you’re appending data in a loop, it’s more efficient to redirect the output once, rather than on each iteration. This reduces the number of times Bash has to open and close the file, leading to faster execution times.
Here’s an example of how to do this:
for i in {1..5}; do echo "Appending line $i"; done >> file.txt
cat file.txt
# Output:
# Appending line 1
# Appending line 2
# Appending line 3
# Appending line 4
# Appending line 5
In this script, we’re redirecting the output of the entire loop to ‘file.txt’, rather than redirecting the output of each ‘echo’ command individually.
Understanding the Bash Shell
The Bash shell is a command-line interpreter that allows you to interact with your operating system. It’s an essential tool for developers and system administrators, providing a powerful interface for executing commands, scripting tasks, and managing files.
Bash offers a wide range of commands and operators for file handling, and one of these is the append operator ‘>>’. This operator is part of Bash’s redirection capabilities, which allow you to control where the input to a command comes from and where the output goes.
File Handling in Unix/Linux
In Unix/Linux systems, everything is treated as a file. This includes not only text files and directories but also hardware devices and processes. Understanding how to handle files is a fundamental skill for working with these systems.
echo 'Hello, Bash!' > file.txt
cat file.txt
# Output:
# Hello, Bash!
In this example, we’re using the ‘echo’ command to generate a string ‘Hello, Bash!’, and the ‘>’ operator to write this string to a file named ‘file.txt’. The ‘cat’ command is then used to display the contents of ‘file.txt’.
The Concept of Redirection in Bash
Redirection is a feature in Bash that allows you to control where the input to a command comes from and where the output goes. It’s a powerful tool that can make your tasks more efficient and flexible.
There are three types of redirections in Bash: standard input (stdin), standard output (stdout), and standard error (stderr). The append operator ‘>>’ is used for redirecting stdout, allowing you to add data to the end of a file without overwriting the existing content.
echo 'Hello, Bash!' >> file.txt
cat file.txt
# Output:
# Hello, Bash!
In this scenario, we’re using the ‘echo’ command to generate a string ‘Hello, Bash!’, and the ‘>>’ operator to append this string to ‘file.txt’. The ‘cat’ command is then used to display the contents of ‘file.txt’.
Understanding these fundamental concepts is key to mastering file handling in Bash and making the most of the Bash append to file functionality.
Expanding Your Bash Skills: Beyond Appending to a File
Appending to a file is a fundamental operation in Bash, but it’s just the tip of the iceberg. Once you’ve mastered this skill, there are many other commands and functions you can learn to enhance your scripting capabilities.
Integrating Append Operations in Larger Scripts
Appending to a file is often used in larger scripts and projects. For instance, you might need to log the output of a script to a file for debugging or record-keeping purposes. Here’s an example:
#!/bin/bash
for ((i=1; i<=5; i++))
do
echo "Running operation $i"
echo "Operation $i completed successfully" >> log.txt
done
cat log.txt
# Output:
# Operation 1 completed successfully
# Operation 2 completed successfully
# Operation 3 completed successfully
# Operation 4 completed successfully
# Operation 5 completed successfully
In this script, we’re running a loop and appending a success message to ‘log.txt’ after each iteration. This allows us to keep a record of the operations that were completed successfully.
Related Commands and Functions
There are many other commands and functions in Bash that are often used in conjunction with the append operation. These include ‘grep’ for searching files, ‘awk’ for text processing, and ‘sed’ for text manipulation, among others. By combining these commands with the append operation, you can create powerful scripts to automate complex tasks.
Further Resources for Bash Mastery
If you’re interested in learning more about Bash and expanding your skills, here are some excellent resources to check out:
- The GNU Bash Reference Manual: This is the official manual for Bash, providing comprehensive documentation on all its features.
Linuxize’s Guide on Appending to a File in Bash: This guide provides clear explanations and examples on how to append content to a file in Bash.
Bash Scripting Guide on tldp.org: A detailed guide that covers various aspects of Bash scripting, from basic to advanced topics.
Wrapping Up: Mastering Bash Append to File
In this comprehensive guide, we’ve explored the process of appending to a file in Bash, from the basic use of the ‘>>’ operator to more advanced techniques and alternative approaches.
We started with the basics, learning how to use the ‘>>’ operator to append data to a file. We then delved into more advanced usage, such as appending data from one file to another, appending command output to a file, and appending data within a script. We also tackled common issues that you might encounter when appending to a file in Bash, such as ‘Permission denied’ and ‘No space left on device’ errors, providing solutions to these problems.
We also explored alternative commands for appending in Bash, such as ‘tee -a’, ‘printf’, and ‘cat’ with redirection. We compared these methods with the ‘>>’ operator, giving you a sense of the broader landscape of file handling in Bash.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘>>’ Operator | Simple, efficient, does not overwrite existing content | Requires write permissions, might fail if there’s insufficient disk space |
‘tee -a’ | Can read from standard input and write to standard output and files, does not overwrite existing content | Might be less intuitive for beginners |
‘printf’ | Provides better string formatting and control | Slightly more complex than the ‘>>’ operator |
‘cat’ with Redirection | Can concatenate and append files | Might be less intuitive for beginners |
Whether you’re just starting out with Bash or you’re looking to enhance your file handling skills, we hope this guide has helped you master the art of appending to a file in Bash.
Understanding how to append to a file is a fundamental skill in Bash scripting, and now you’re well equipped to use this skill in your projects. Happy scripting!