How to Get Length of a Bash Array: Scripting Syntax Guide
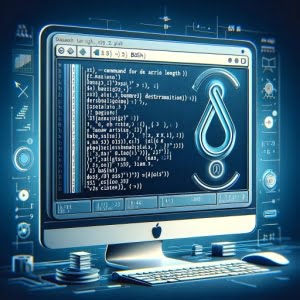
Ever found yourself puzzled when trying to determine the length of an array in Bash? You’re not alone. Many developers find this task a bit tricky, but Bash, like a skilled tailor, provides tools to measure the length of an array.
Bash arrays, akin to a string of pearls, can hold multiple values, each associated with a unique index. Understanding the length of these arrays is crucial when it comes to iterating over them or performing operations on each element.
This guide will walk you through the process of determining the length of an array in Bash, from basic usage to advanced techniques. We’ll cover everything from the basics of Bash arrays, how to measure their length, to dealing with multi-dimensional arrays and even troubleshooting common issues.
So, let’s dive in and start mastering Bash array length!
TL;DR: How Do I Find the Length of an Array in Bash?
To find the length of an array in Bash, you can use the code,
${#array[@]}
. This command will return the number of elements in the array.
Here’s a simple example:
array=("apple" "banana" "cherry")
echo "${#array[@]}"
# Output:
# 3
In this example, we’ve created an array with three elements: ‘apple’, ‘banana’, and ‘cherry’. The ${#array[@]}
syntax is then used to determine the length of the array, which in this case, returns ‘3’.
This is a basic way to find the length of an array in Bash, but there’s much more to learn about handling arrays in Bash. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding the ${#array[@]} Syntax
- Handling Multi-Dimensional and Associative Arrays
- Exploring Alternative Methods for Array Length Determination
- Troubleshooting Common Issues with Bash Arrays
- Bash Arrays: A Brief Overview
- The Relevance of Array Length Determination
- Wrapping Up: Mastering Bash Array Length
Understanding the ${#array[@]}
Syntax
The ${#array[@]}
syntax is a powerful tool in Bash for determining the length of an array. It may seem a bit cryptic at first glance, but once you break it down, it’s quite straightforward.
Let’s dissect it:
array
is the name of the array.[@]
is a special parameter that expands to all indices in the array.#
is a prefix that, when used before a variable, returns its length.
Therefore, ${#array[@]}
returns the length of the array.
Let’s see this in action with a different example:
fruits=("apple" "banana" "cherry" "date" "elderberry")
echo "${#fruits[@]}"
# Output:
# 5
In this example, our fruits
array contains five elements. When we use the ${#fruits[@]}
syntax, it returns ‘5’, the number of elements in the array.
This syntax is quick, efficient, and easy to use. However, it’s important to remember that it will only return the number of elements in the array and not the indices. So if you have an array with non-sequential indices, this syntax will not return the highest index number.
Next, we’ll delve into more complex scenarios, such as dealing with multi-dimensional arrays and associative arrays.
Handling Multi-Dimensional and Associative Arrays
Once you’ve mastered the basics of determining the length of a simple array in Bash, you can start exploring more complex scenarios. This includes dealing with multi-dimensional arrays and associative arrays.
Multi-Dimensional Arrays
In Bash, multi-dimensional arrays aren’t supported directly. However, you can simulate them using one-dimensional arrays. But how do you determine the length of such an array?
Let’s consider an example:
multi_array=("apple banana" "cherry date" "elderberry fig")
echo "${#multi_array[@]}"
# Output:
# 3
Here, we have an array with three elements, each containing two fruit names. Using the ${#multi_array[@]}
syntax, we get ‘3’, which is the number of elements in the array, not the total number of fruit names.
Associative Arrays
Associative arrays in Bash allow you to use strings as indices instead of integers. Let’s see how we can determine the length of an associative array:
declare -A fruits
fruits=( [apple]='red' [banana]='yellow' [cherry]='red' )
echo "${#fruits[@]}"
# Output:
# 3
In this example, we’ve created an associative array fruits
where each fruit (the key) is associated with a color (the value). Using the ${#fruits[@]}
syntax, we get ‘3’, which is the number of key-value pairs in the array.
Whether you’re dealing with simple, multi-dimensional, or associative arrays, the ${#array[@]}
syntax is a powerful tool for determining the length of the array. However, it’s important to understand its limitations and use it correctly to get accurate results.
Exploring Alternative Methods for Array Length Determination
While the ${#array[@]}
syntax is a powerful and efficient tool for determining the length of an array in Bash, there are alternative approaches that can be used. These include using loops or external tools like awk. Let’s explore these methods.
Using Loops
One way to determine the length of an array is by using a loop to iterate over the elements. Here’s an example:
fruits=("apple" "banana" "cherry" "date" "elderberry")
length=0
for fruit in "${fruits[@]}"
do
((length++))
done
echo $length
# Output:
# 5
In this example, we initialize a counter length
to zero. Then, we use a for loop to iterate over the elements in the fruits
array. For each iteration, we increment the length
counter. Finally, we echo the length
which gives us ‘5’, the number of elements in the array.
While this method is effective, it is more verbose and slower than the ${#array[@]}
syntax, especially for large arrays.
Using AWK
AWK is a powerful text processing tool that can also be used to determine the length of an array. Here’s how you can do it:
echo ${fruits[@]} | awk '{print NF}'
# Output:
# 5
In this example, we echo the elements of the fruits
array and pipe them to AWK. The NF
variable in AWK represents the number of fields (words) in the input, which corresponds to the number of elements in the array.
This method can be useful if you’re already working with AWK in your script. However, it requires an external tool and may not be as efficient as the ${#array[@]}
syntax.
In conclusion, while there are alternative methods to determine the length of an array in Bash, the ${#array[@]}
syntax is generally the most efficient and recommended approach. However, understanding these alternatives can provide you with more flexibility and options in your scripting.
Troubleshooting Common Issues with Bash Arrays
While working with Bash arrays, you might encounter several issues that could lead to inaccurate results when determining the length of an array. This section will discuss common issues such as dealing with empty arrays or arrays with null values, and provide solutions and workarounds.
Dealing with Empty Arrays
What happens when you try to determine the length of an empty array? Let’s find out:
empty_array=()
echo "${#empty_array[@]}"
# Output:
# 0
As you can see, the ${#empty_array[@]}
syntax correctly returns ‘0’, indicating that the array is empty. Thus, this syntax handles empty arrays gracefully without any errors.
Arrays with Null Values
But what if your array contains null values? Will the ${#array[@]}
syntax still work? Let’s see an example:
null_array=("" "apple" "" "banana" "")
echo "${#null_array[@]}"
# Output:
# 5
In this example, the null_array
contains five elements, three of which are null. The ${#null_array[@]}
syntax still returns ‘5’, counting the null values as valid elements.
Therefore, when working with Bash arrays, it’s important to be aware of the nature of your data. If your array could contain null values and you don’t want to count them, you’ll need to add additional logic to your script to handle this scenario.
In conclusion, while the ${#array[@]}
syntax is a powerful tool for determining the length of a Bash array, it’s important to understand its behavior and limitations. By being aware of potential issues and how to troubleshoot them, you can write more robust and accurate scripts.
Bash Arrays: A Brief Overview
To fully grasp the concept of determining the length of an array in Bash, it’s essential to understand what arrays are and how they function in Bash. So let’s dive into the fundamentals of Bash arrays.
What are Arrays in Bash?
In Bash, an array is a variable that can hold multiple values. These values are stored at specific indices, starting from zero. Here’s an example of how you can declare an array in Bash:
fruits=("apple" "banana" "cherry")
In this example, ‘apple’ is stored at index 0, ‘banana’ at index 1, and ‘cherry’ at index 2.
Accessing Array Elements
You can access an element in the array using its index. For example:
echo "${fruits[0]}"
# Output:
# apple
This command will print ‘apple’, which is the element at index 0 in the fruits
array.
Understanding Bash Array Length
The length of a Bash array is the number of elements it contains. For instance, our fruits
array has three elements, so its length is 3. As we’ve seen, you can determine the length of an array using the ${#array[@]}
syntax.
Understanding these fundamentals of Bash arrays is crucial for working with them effectively. With this knowledge, you can now better appreciate the process of determining the length of an array in Bash.
The Relevance of Array Length Determination
Understanding the length of an array in Bash is not just a neat trick—it’s a fundamental skill that has a wide range of applications. From scripting to data processing, the ability to accurately determine array length is a key part of efficient and effective Bash programming.
Array Manipulation in Bash
Determining the length of an array is just one aspect of array manipulation in Bash. Other operations include adding, removing, or modifying elements, as well as sorting and filtering arrays. These operations, combined with the ability to determine array length, can enable you to handle complex data processing tasks with ease.
# Adding an element to the array
fruits+=("elderberry")
# Removing an element from the array
unset fruits[0]
# Modifying an element in the array
fruits[1]="blackberry"
# Printing the modified array
echo "${fruits[@]}"
# Output:
# blackberry cherry elderberry
In this example, we’ve performed various array manipulation operations on the fruits
array. Understanding these operations can help you make the most of Bash arrays.
Loop Constructs in Bash
Loop constructs are another essential feature of Bash that often go hand-in-hand with arrays. For instance, you might need to iterate over an array and perform an operation on each element. This is where understanding loop constructs, such as for loops, while loops, and until loops, becomes crucial.
# Iterating over an array with a for loop
for fruit in "${fruits[@]}"
do
echo $fruit
done
# Output:
# blackberry
# cherry
# elderberry
In this example, we’ve used a for loop to iterate over the fruits
array and print each element. This is a simple demonstration of how loop constructs can be used with arrays.
Further Resources for Bash Array Mastery
To deepen your understanding of Bash arrays and related concepts, here are some external resources that you might find useful:
- GNU Bash Manual: The official manual for Bash, including a detailed section on arrays.
- Advanced Bash-Scripting Guide: A comprehensive guide to scripting in Bash, with a focus on advanced features like arrays.
- Linux Handbook – Array Length in Bash: This article offers a concise explanation of how to determine the length of an array in Bash scripting.
Wrapping Up: Mastering Bash Array Length
In this comprehensive guide, we’ve delved into the process of determining the length of an array in Bash. We’ve explored the usage of the ${#array[@]}
syntax, discussed common issues, and their solutions, and even looked at alternative approaches to handle this task.
We began with the basics, learning how to use the ${#array[@]}
syntax to find the length of a simple array. We then ventured into more advanced territory, exploring how to handle multi-dimensional and associative arrays. Along the way, we tackled common challenges you might face when working with Bash arrays, such as dealing with empty arrays or arrays with null values, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to determining the length of an array, such as using loops or external tools like awk. These methods provide additional flexibility and options, but as we’ve seen, the ${#array[@]}
syntax is generally the most efficient and recommended approach.
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash array length and its importance in efficient and effective Bash programming.
With its balance of speed, simplicity, and power, Bash is a fundamental tool for any developer. Now, you’re well equipped to master Bash array length. Happy scripting!