How to Check If a Directory Exists: Bash Scripting Guide
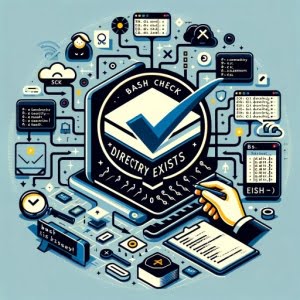
Ever found yourself in a situation where you need to verify the existence of a directory in Bash? You’re not alone. Many developers and system administrators encounter this task, but Bash, like a seasoned detective, can help you find out if a directory exists or not.
Think of Bash’s directory existence check as a compass – guiding you through the filesystem, helping you navigate through directories, and confirming their existence. This is a fundamental skill in Bash scripting, often used in various tasks like error handling, conditional processing, and system administration.
In this guide, we’ll walk you through the process of checking if a directory exists in Bash, from basic usage to advanced techniques. We’ll cover everything from using the ‘-d’ test operator, handling multiple directories, to dealing with common issues and their solutions.
So, let’s dive in and start mastering Bash directory existence checks!
TL;DR: How Do I Check if a Directory Exists in Bash?
In Bash, you can verify if a directory exists by using the
'-d'
test operator followed by the directory path, such as,-d '/path/to/directory'
. Here’s a quick example:
if [ -d '/path/to/directory' ]; then
echo 'Directory exists'
else
echo 'Directory does not exist'
fi
# Output:
# 'Directory exists' (if the directory exists)
# 'Directory does not exist' (if the directory does not exist)
In this example, we use the ‘-d’ test operator in an if-else statement to check if a directory exists at the specified path. If the directory exists, ‘Directory exists’ is printed to the console. If not, ‘Directory does not exist’ is printed instead.
This is a basic way to check if a directory exists in Bash, but there’s much more to learn about handling directories and errors in Bash. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Bash Directory Checks: The ‘-d’ Test Operator
- Advanced Bash Directory Checks: Handling Multiple Directories
- Error Handling in Bash Directory Checks
- Exploring Alternative Methods: ‘find’ and ‘ls’ Commands
- Troubleshooting Bash Directory Checks: Common Issues
- Understanding Bash Scripting, Directory Structure, and File Permissions
- The Bigger Picture: Directory Checks in Larger Scripts and Projects
- Wrapping Up: Directory Checks in Bash
Bash Directory Checks: The ‘-d’ Test Operator
In Bash, the ‘-d’ test operator is a powerful tool in your arsenal for checking if a directory exists. It’s like a trusty sidekick in your Bash adventures, always ready to help you verify the existence of directories.
Understanding the ‘-d’ Test Operator
The ‘-d’ test operator checks if a file exists and is a directory. You can use it in conditional expressions to control the flow of your script based on whether a directory exists or not.
Here’s an example:
dirname='/path/to/directory'
if [ -d "$dirname" ]; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist, or is not a directory."
fi
# Output:
# '/path/to/directory exists and is a directory.' (if the directory exists)
# '/path/to/directory does not exist, or is not a directory.' (if the directory does not exist or is not a directory)
In this code block, we first define a variable dirname
with the path of the directory we want to check. Then, we use the ‘-d’ test operator inside an if-else statement. If the directory at the path stored in dirname
exists, we print a message stating that it exists and is a directory. If it does not exist or is not a directory, we print a different message.
Advantages and Potential Pitfalls
Using the ‘-d’ test operator is straightforward and efficient, making it a great choice for simple directory existence checks. However, keep in mind that it only checks if the file is a directory. If a file with the same name exists but is not a directory, the ‘-d’ test will return false. This is why the error message in our example specifies ‘does not exist, or is not a directory.’
Advanced Bash Directory Checks: Handling Multiple Directories
As you progress in your Bash scripting journey, you’ll often find yourself needing to check multiple directories. Bash allows you to handle multiple directories efficiently, saving you time and making your scripts more robust.
Checking Multiple Directories
Suppose you have a list of directories and you want to check if each one exists. Bash allows you to do this using a for loop. Here’s an example:
directories=("/path/to/dir1" "/path/to/dir2" "/path/to/dir3")
for dir in "${directories[@]}"; do
if [ -d "$dir" ]; then
echo "$dir exists and is a directory."
else
echo "$dir does not exist, or is not a directory."
fi
done
# Output:
# '/path/to/dir1 exists and is a directory.' (if dir1 exists)
# '/path/to/dir2 does not exist, or is not a directory.' (if dir2 does not exist or is not a directory)
# '/path/to/dir3 exists and is a directory.' (if dir3 exists)
In this example, we first define an array directories
with the paths of the directories we want to check. Then, we loop over each directory in the array and use the ‘-d’ test operator to check if it exists and is a directory. We print a message for each directory based on the result.
Error Handling in Bash Directory Checks
When checking if a directory exists in Bash, you might encounter errors like permission issues. You can handle these errors using a try-catch-like mechanism with command exits and traps. Here’s an example:
dirname='/path/to/directory'
{ # try
if [ -d "$dirname" ]; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist, or is not a directory."
fi
} || { # catch
echo 'Error occurred while checking directory.'
}
# Output:
# '/path/to/directory exists and is a directory.' (if the directory exists and no error occurs)
# 'Error occurred while checking directory.' (if an error occurs)
In this example, we use {}
to group commands and ||
to catch errors. If an error occurs while executing the commands in the ‘try’ block, the ‘catch’ block is executed and an error message is printed.
Exploring Alternative Methods: ‘find’ and ‘ls’ Commands
While the ‘-d’ test operator is a handy tool for checking if a directory exists in Bash, it’s not the only tool available. There are other commands like ‘find’ and ‘ls’ that can be used to achieve the same goal. Let’s explore these alternatives.
Using the ‘find’ Command
The ‘find’ command is a powerful tool that can search for files and directories based on various criteria. Here’s how you can use it to check if a directory exists:
dirname='/path/to/directory'
if find "$dirname" -type d -print -quit | grep -q .; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist, or is not a directory."
fi
# Output:
# '/path/to/directory exists and is a directory.' (if the directory exists)
# '/path/to/directory does not exist, or is not a directory.' (if the directory does not exist)
In this example, ‘find’ searches for the directory and ‘grep’ checks if any output was produced. If ‘grep’ finds any output, the directory exists.
Using the ‘ls’ Command
The ‘ls’ command lists directory contents. You can use it with a conditional statement to check if a directory exists:
dirname='/path/to/directory'
if ls "$dirname" >/dev/null 2>&1; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist, or is not a directory."
fi
# Output:
# '/path/to/directory exists and is a directory.' (if the directory exists)
# '/path/to/directory does not exist, or is not a directory.' (if the directory does not exist)
In this example, ‘ls’ tries to list the contents of the directory. If it succeeds, the directory exists. We redirect the output to ‘/dev/null’ to suppress it.
Weighing the Alternatives
While ‘find’ and ‘ls’ provide alternative ways to check if a directory exists, they come with their own advantages and disadvantages. The ‘find’ command is powerful and flexible but can be slower than ‘-d’ or ‘ls’ for large directories. On the other hand, ‘ls’ is fast and simple, but it can produce unwanted output if not properly redirected.
In the end, the best method depends on your specific needs and the context in which you’re working. The ‘-d’ test operator is usually sufficient for simple directory checks, but ‘find’ and ‘ls’ can be useful in more complex scenarios.
Troubleshooting Bash Directory Checks: Common Issues
Even with the best-laid plans, you might encounter issues when checking if a directory exists in Bash. This section aims to arm you with solutions and workarounds for common problems you may face.
Dealing with ‘Permission Denied’
If you don’t have read permissions for a directory, Bash will not be able to check if it exists and will return a ‘Permission denied’ error. Here’s an example:
dirname='/path/to/protected/directory'
if [ -d "$dirname" ]; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist, or is not a directory."
fi
# Output:
# 'bash: line 2: /path/to/protected/directory: Permission denied'
In this example, Bash tries to check a directory for which it doesn’t have read permissions, resulting in a ‘Permission denied’ error. You can handle this by catching the error and printing a custom message:
dirname='/path/to/protected/directory'
{ # try
if [ -d "$dirname" ]; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist, or is not a directory."
fi
} || { # catch
echo 'Error occurred while checking directory. Do you have the necessary permissions?'
}
# Output:
# 'Error occurred while checking directory. Do you have the necessary permissions?'
Handling ‘No Such File or Directory’
If the directory you’re checking does not exist, Bash will return a ‘No such file or directory’ message. However, this is not an error, but rather an expected outcome. You can handle this by providing a custom message when the ‘-d’ test returns false:
dirname='/path/to/nonexistent/directory'
if [ -d "$dirname" ]; then
echo "$dirname exists and is a directory."
else
echo "$dirname does not exist."
fi
# Output:
# '/path/to/nonexistent/directory does not exist.'
In this example, Bash checks a directory that does not exist. The ‘-d’ test returns false, and a custom message is printed.
Remember, troubleshooting is an essential part of any scripting process. Understanding these common issues and their solutions will help you write more robust and reliable Bash scripts.
Understanding Bash Scripting, Directory Structure, and File Permissions
Before diving deeper into the intricacies of checking directory existence in Bash, it’s important to grasp the fundamentals of Bash scripting, directory structure, and file permissions. These concepts form the backbone of many operations in Bash, including directory existence checks.
Bash Scripting Basics
Bash, or the Bourne Again SHell, is a popular command-line interpreter. It allows you to automate tasks through scripts, enhancing productivity and reducing the possibility of errors. Here’s a simple Bash script that prints ‘Hello, World!’:
echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this script, ‘echo’ is a command that prints its argument to the console. This is a simple example, but Bash scripts can be much more complex, involving variables, conditional statements, loops, and more.
Directory Structure in Linux
Linux, like other Unix-like systems, organizes files in a hierarchical directory structure. At the top is the root directory (‘/’), with other directories branching off it. Here’s an example of a directory path:
/path/to/directory
In this path, ‘path’ is a directory in the root directory, ‘to’ is a directory in ‘path’, and ‘directory’ is a directory in ‘to’.
File Permissions in Linux
Linux systems, including those running Bash, have a robust system of file permissions. Each file and directory has a set of permissions that determine who can read, write, and execute it. Here’s an example of viewing file permissions with ‘ls’:
ls -l /path/to/directory
# Output:
# 'drwxr-xr-x 2 user group 4096 Jan 1 00:00 /path/to/directory'
In this example, ‘ls -l’ lists files in long format, showing file permissions. The first character ‘d’ indicates that this is a directory. The next nine characters represent the read (‘r’), write (‘w’), and execute (‘x’) permissions for the owner, group, and others, respectively.
Understanding these fundamentals will help you better understand the process of checking if a directory exists in Bash, as well as troubleshoot any issues you might encounter.
The Bigger Picture: Directory Checks in Larger Scripts and Projects
While checking if a directory exists in Bash might seem like a small task, it plays a crucial role in larger scripts and projects. Whether you’re automating system administration tasks, managing files, or developing software, directory existence checks are often a fundamental part of the process.
Directory Checks in File Handling
In file handling operations, checking if a directory exists can help prevent errors. For instance, before creating a file in a directory, you might want to check if the directory exists and create it if it doesn’t. This can prevent ‘No such file or directory’ errors and make your scripts more robust.
Here’s an example:
dirname='/path/to/directory'
filename='file.txt'
# Check if directory exists and create it if it doesn't
if [ ! -d "$dirname" ]; then
mkdir "$dirname"
fi
# Create file in directory
touch "$dirname/$filename"
# Output:
# No output if the directory and file are created successfully
In this script, we first check if a directory exists using the ‘! -d’ test operator. If the directory does not exist, we create it using ‘mkdir’. Then, we create a file in the directory using ‘touch’.
Directory Checks in Loops
In loops, you might use directory checks to process only existing directories. This can be useful in tasks like batch file processing, where you need to perform operations on files in multiple directories.
Here’s an example:
directories=("/path/to/dir1" "/path/to/dir2" "/path/to/dir3")
for dir in "${directories[@]}"; do
if [ -d "$dir" ]; then
echo "Processing $dir"
# Process files in directory
fi
done
# Output:
# 'Processing /path/to/dir1' (if dir1 exists)
# 'Processing /path/to/dir3' (if dir3 exists)
In this script, we loop over an array of directories and check if each one exists. If a directory exists, we print a message and process the files in the directory.
Further Resources for Bash Directory Checks Mastery
For further exploration of Bash scripting and directory checks, the following resources are highly recommended:
- GNU Bash Manual: A comprehensive guide to Bash from the creators of Bash.
- Advanced Bash-Scripting Guide: A detailed guide to advanced Bash scripting techniques.
- Guide on Checking if a Directory Exists in Linux: Baeldung’s guide explains various methods to check if a directory exists in Linux.
Remember, mastery comes with practice. So, keep scripting, keep exploring, and keep learning!
Wrapping Up: Directory Checks in Bash
In this comprehensive guide, we’ve delved into the process of checking if a directory exists in Bash, exploring the command usage, common issues, and their solutions.
We began with the basics, learning how to use the ‘-d’ test operator to check if a directory exists. We then explored more advanced techniques, such as handling multiple directories and error handling. Along the way, we tackled common issues like ‘Permission denied’ and ‘No such file or directory’, providing you with solutions for each problem.
We also examined alternative approaches to checking if a directory exists in Bash, such as using the ‘find’ and ‘ls’ commands. Each method has its own strengths and weaknesses, and the best choice depends on your specific needs and context.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘-d’ Test Operator | Simple, efficient | Only checks if the file is a directory |
‘find’ Command | Powerful, flexible | Can be slower for large directories |
‘ls’ Command | Fast, simple | Can produce unwanted output if not redirected |
Whether you’re just starting out with Bash scripting or you’re looking to level up your directory checking skills, we hope this guide has given you a deeper understanding of how to check if a directory exists in Bash.
The ability to check if a directory exists is a fundamental skill in Bash scripting, crucial for tasks like error handling, conditional processing, and system administration. With this guide, you’re well equipped to use this skill in your Bash scripting adventures. Happy coding!