Bash Colors | Color Codes and Syntax Cheat Sheet
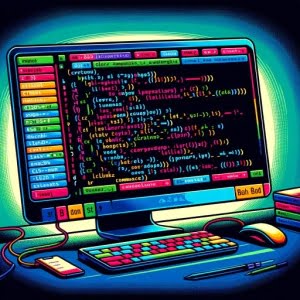
Are you finding it challenging to add a splash of color to your Bash scripts? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Just like an artist uses a palette, Bash provides a range of colors to make your scripts more readable and engaging. These colors can transform your scripts, making them more visually appealing and easier to understand.
This guide will walk you through the process of using colors in Bash, from the basics to more advanced techniques. We’ll explore Bash’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Bash color!
TL;DR: How Do I Use Colors in Bash?
To use colors in Bash, you can utilize
escape sequences
with the syntax,echo -e "\e[{colorCode}mSample Text\e[0m"
. The-e
enables backslash escapes interpretation, the\e
(or sometimes\033
) denotes the start of color modifications,{colorCode}m
is where you will place the desired color code, and\e[0m
marks the end of color modifications. These sequences allow you to change the color of your text output in the terminal.
Here’s a simple example:
echo -e "\033[31m Hello, World!\033[0m"
# Output:
# Hello, World! (in red color)
In this example, we’ve used an escape sequence \033[31m
to change the color of the text ‘Hello, World!’ to red. The \033[0m
sequence resets the color back to the terminal’s default.
This is a basic way to use colors in Bash, but there’s much more to learn about colorizing your scripts. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Bash Color Basics: Coloring Text with Escape Sequences
- Advanced Bash Color: Coloring Text and Backgrounds
- Exploring Alternative Approaches to Bash Coloring
- Troubleshooting Bash Color Issues
- Understanding Bash Colors: The Fundamentals
- Bash Color in Real-World Scenarios
- Exploring Related Concepts
- Further Resources for Bash Color Mastery
- Wrapping Up: Mastering Bash Color for Effective Scripting
Bash Color Basics: Coloring Text with Escape Sequences
Bash provides a simple way to add color to your text output using escape sequences. These sequences are a series of characters that Bash interprets as instructions to perform specific actions, like changing the text color.
How to Colorize Text in Bash
Let’s look at a basic example of how to use escape sequences to colorize text in Bash:
echo -e "\033[32m Welcome to Bash Color Basics!\033[0m"
# Output:
# Welcome to Bash Color Basics! (in green color)
In this code, \033[32m
is an escape sequence that instructs Bash to change the color of the following text to green. The \033[0m
sequence resets the color back to the terminal’s default.
Advantages of Using Escape Sequences
Using escape sequences to colorize text in Bash has several advantages:
- It provides a visual distinction, making scripts more readable and engaging.
- It can help in debugging by highlighting important information.
Potential Pitfalls
While using escape sequences is a simple and effective method to colorize text in Bash, it also has a few potential pitfalls. For instance, different terminals may interpret escape sequences differently, leading to inconsistent results. Also, if not reset properly, an escape sequence can affect the color of all subsequent text.
In the next sections, we’ll delve deeper into more advanced techniques of using colors in Bash.
Advanced Bash Color: Coloring Text and Backgrounds
As you become more comfortable with Bash color basics, you can start exploring more advanced techniques. One of these techniques involves using different color codes and background colors in your Bash scripts.
Color Codes in Bash
In Bash, each color is associated with a specific code. For example, the code for red is 31, and the code for green is 32. By changing the number in the escape sequence, you can change the color of your text.
Here’s an example that uses different color codes:
echo -e "\033[31m This is red text!\033[0m"
echo -e "\033[32m This is green text!\033[0m"
echo -e "\033[33m This is yellow text!\033[0m"
# Output:
# This is red text! (in red color)
# This is green text! (in green color)
# This is yellow text! (in yellow color)
In each line, we’re changing the number in the escape sequence to change the color of the text.
Background Colors in Bash
In addition to changing the text color, you can also change the background color of your text in Bash. The codes for background colors are slightly different from the text color codes.
Let’s take a look at an example that changes both the text and background colors:
echo -e "\033[37;44m White text on a blue background!\033[0m"
# Output:
# White text on a blue background! (white text on a blue background)
In this example, 37
is the code for white text, and 44
is the code for a blue background. We’ve combined them in the escape sequence to create white text on a blue background.
Best Practices for Using Colors in Bash
While using colors in Bash can make your scripts more engaging and readable, it’s important to use them sparingly and consistently. Too many colors can make your scripts confusing and hard to read. Also, make sure to always reset your colors using the \033[0m
escape sequence to avoid unintentionally coloring your subsequent text.
Exploring Alternative Approaches to Bash Coloring
While using escape sequences is a common method to add colors in Bash, there are other techniques you can use. These alternative methods, such as the tput
command and third-party libraries, offer more flexibility and control over your Bash colors.
Coloring Bash with tput
The tput
command is a powerful tool that can manipulate the terminal’s capabilities, including changing the text color. It’s a more portable and flexible alternative to escape sequences.
Here’s an example of using tput
to change the text color:
tput setaf 2; echo "This is green text!"; tput sgr0
# Output:
# This is green text! (in green color)
In this code, tput setaf 2
sets the text color to green, and tput sgr0
resets the color back to the default. The setaf
command stands for ‘set ANSI foreground’, and the number 2
corresponds to the color green.
Using Third-Party Libraries for Bash Coloring
There are also third-party libraries available that can simplify the process of adding colors to your Bash scripts. These libraries, such as bash-colors
, provide predefined color variables that you can use in your scripts.
Here’s an example of using bash-colors
to colorize your text:
source bash-colors.sh
echo "${green}This is green text!${reset}"
# Output:
# This is green text! (in green color)
In this example, we’re sourcing the bash-colors.sh
script to use its predefined color variables. The ${green}
variable changes the text color to green, and the ${reset}
variable resets the color back to the default.
Choosing the Best Approach for Bash Coloring
Each method of adding colors in Bash has its advantages and disadvantages. Escape sequences are simple and straightforward, but they can be hard to read and are not always portable. The tput
command is more flexible and portable, but it requires more typing. Third-party libraries are easy to use and read, but they require an external dependency.
The best approach depends on your specific needs and constraints. If you’re writing a short script, escape sequences might be the simplest solution. If you’re writing a larger script or a script that needs to be portable, you might want to consider using the tput
command or a third-party library.
Troubleshooting Bash Color Issues
While using colors in Bash can enhance your scripts, it’s not without its challenges. You may encounter issues such as compatibility problems with different terminals or unexpected color changes. Let’s discuss some of these common problems and their solutions.
Terminal Compatibility Issues
One common issue when using colors in Bash is compatibility problems with different terminals. Not all terminals interpret escape sequences in the same way, which can lead to inconsistent results.
For instance, here’s a simple script that changes the text color to red:
echo -e "\033[31m This is red text!\033[0m"
# Output in most terminals:
# This is red text! (in red color)
In most terminals, this script will output ‘This is red text!’ in red color. However, in some terminals, the text might not appear in red, or the escape sequence might be printed as literal text.
To solve this issue, you can use the tput
command, which is more portable and consistent across different terminals:
tput setaf 1; echo "This is red text!"; tput sgr0
# Output in all terminals:
# This is red text! (in red color)
Unexpected Color Changes
Another common issue when using colors in Bash is unexpected color changes. If you forget to reset your colors using the \033[0m
escape sequence or the tput sgr0
command, the color change can affect all subsequent text.
To avoid this issue, always make sure to reset your colors after changing them. This will ensure that your color changes only affect the intended text and not the rest of your script.
Understanding Bash Colors: The Fundamentals
To effectively use colors in Bash, it’s crucial to grasp the underlying concepts and mechanisms. Let’s dive into how Bash handles colors, the role of escape sequences, and the importance of ANSI color codes.
How Bash Handles Colors
Bash, like many other command-line interfaces, uses the ANSI color codes to display colors. These codes are interpreted by the terminal, which then changes the color of the text accordingly.
Here’s a simple example that changes the text color to blue:
echo -e "\033[34m This is blue text!\033[0m"
# Output:
# This is blue text! (in blue color)
In this code, \033[34m
is an ANSI color code that instructs the terminal to change the text color to blue.
The Role of Escape Sequences
Escape sequences play a crucial role in coloring text in Bash. An escape sequence is a series of characters that starts with the escape character (represented as \033
or \e
in Bash). It gives a special meaning to the characters that follow it.
In the context of Bash colors, escape sequences are used to represent ANSI color codes. For example, the escape sequence \033[31m
represents the ANSI color code for red.
Understanding ANSI Color Codes
ANSI color codes are standardized codes that represent different colors. In Bash, these codes are used to change the color of the text and background in the terminal.
Each color has a specific code. For example, the code for black is 0, the code for red is 31, and the code for green is 32. By changing the number in the escape sequence, you can change the color of your text or background.
Here’s an example that uses different ANSI color codes:
echo -e "\033[0m This is black text!"
echo -e "\033[31m This is red text!"
echo -e "\033[32m This is green text!"
# Output:
# This is black text! (in black color)
# This is red text! (in red color)
# This is green text! (in green color)
In each line, we’re changing the number in the escape sequence to change the color of the text according to the corresponding ANSI color code.
Bash Color in Real-World Scenarios
Using colors in Bash isn’t just about making your scripts look pretty. It has practical applications in various real-world scenarios that can enhance your scripting skills and productivity.
Colorizing Logging
One common use of colors in Bash is in logging. By colorizing your log messages, you can make them easier to read and understand. For instance, you might use red for error messages, yellow for warnings, and green for success messages.
RED='\033[0;31m'
GREEN='\033[0;32m'
YELLOW='\033[0;33m'
NC='\033[0m' # No Color
echo -e "${GREEN}INFO: Process completed successfully.${NC}"
echo -e "${YELLOW}WARNING: Low disk space.${NC}"
echo -e "${RED}ERROR: File not found.${NC}"
# Output:
# INFO: Process completed successfully. (in green color)
# WARNING: Low disk space. (in yellow color)
# ERROR: File not found. (in red color)
In this script, we’re using different colors to highlight different types of log messages. This can make it easier to spot important information in your logs.
Enhancing User Prompts
Colors can also be used to enhance user prompts in Bash scripts. By using different colors for your prompts, you can guide the user’s attention and make your scripts more user-friendly.
echo -e "${GREEN}Enter your name:${NC}"
read name
echo -e "${YELLOW}Hello, ${name}!${NC}"
# Output:
# Enter your name: (in green color)
# Hello, John! (in yellow color)
In this example, we’re using green to highlight the prompt and yellow to display the user’s input. This can make the interaction more engaging and clear for the user.
Exploring Related Concepts
Once you’ve mastered the basics of using colors in Bash, you might want to explore related concepts like formatting text in Bash. This can involve changing the text style (bold, underline, etc.), positioning the cursor, or clearing the screen. These techniques can further enhance your Bash scripts and make them more dynamic and interactive.
Further Resources for Bash Color Mastery
To deepen your understanding of using colors in Bash, here are some additional resources you might find helpful:
- Bash scripting guide by The Linux Documentation Project
- Advanced Bash scripting guide by Mendel Cooper
- Bash scripting tutorial by Ryan Chadwick
Wrapping Up: Mastering Bash Color for Effective Scripting
In this comprehensive guide, we’ve delved into the world of using colors in Bash, an essential tool to make your scripts more readable and engaging.
We began with the basics, learning how to use escape sequences to add colors to your Bash scripts. We then delved into more advanced techniques, exploring different color codes, background colors, and even alternative methods like using the tput
command and third-party libraries.
Along our journey, we tackled common challenges you might encounter when using colors in Bash, such as terminal compatibility issues and unexpected color changes, providing you with solutions for each hurdle.
We also compared the different methods of adding colors in Bash, giving you a sense of the broader landscape of tools available for script coloring. Here’s a quick comparison of these methods:
Method | Flexibility | Portability | Ease of Use |
---|---|---|---|
Escape Sequences | Moderate | Low | High |
tput Command | High | High | Moderate |
Third-Party Libraries | High | Moderate | High |
Whether you’re just starting out with Bash color or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash color and its capabilities.
Using colors in Bash is a powerful tool for enhancing your scripts and making them more user-friendly. Now, you’re well equipped to add a splash of color to your Bash scripts. Happy scripting!