‘If’ and ‘And’ Operators in Bash: Conditional Logic Guide
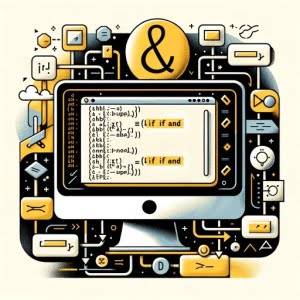
Are you finding it challenging to grasp conditional logic in Bash? You’re not alone. Many developers find themselves puzzled when it comes to using ‘if’ and ‘and’ operators in Bash scripting, but we’re here to help.
Think of Bash as a decision-making machine. The ‘if’ and ‘and’ operators are the gears that help it make choices. They allow you to create scripts that can make decisions based on certain conditions, making your scripts more dynamic and versatile.
In this guide, we’ll help you understand and master the use of ‘if’ and ‘and’ operators in Bash scripting. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches. We’ll also discuss common issues and their solutions.
So, let’s dive in and start mastering conditional logic in Bash!
TL;DR: How Do I Use ‘if’ and ‘and’ in Bash Scripting?
In Bash, you can use
'if'
and'and'
(&&
) to create complex conditional statements such as,if [statement 1] && [statement2]; then...
. These operators allow you to test multiple conditions at once and execute certain commands based on the results.
Here’s a simple example:
var=15
if [ $var -gt 10 ] && [ $var -lt 20 ]; then
echo 'Var is between 10 and 20'
fi
# Output:
# 'Var is between 10 and 20'
In this example, we have a variable var
set to 15. The if
statement checks if var
is greater than 10 and less than 20. Since both conditions are true, the command echo 'Var is between 10 and 20'
is executed, and the output is ‘Var is between 10 and 20’.
This is just a basic way to use ‘if’ and ‘and’ in Bash scripting, but there’s much more to learn about creating and managing conditional statements in Bash. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of ‘If’ and ‘And’ in Bash Scripting
- Expanding Your Toolkit: ‘If’, ‘And’, and ‘Or’
- Alternative Ways to Handle Conditional Logic in Bash
- Overcoming Challenges: Troubleshooting ‘If’ and ‘And’ in Bash
- Bash Scripting and Conditional Logic: The Foundation
- Expanding Your Bash Toolkit: ‘If’ and ‘And’ in Larger Projects
- Wrapping Up: Mastering ‘If’ and ‘And’ in Bash Scripting
Understanding the Basics of ‘If’ and ‘And’ in Bash Scripting
To understand the basic use of ‘if’ and ‘and’ in Bash scripting, let’s start with a simple script example. We’ll use ‘if’ and ‘and’ to check if a file exists and if it is readable. This is a common use case in Bash scripting, especially when dealing with file operations.
filename='test.txt'
if [ -e $filename ] && [ -r $filename ]; then
echo 'The file exists and is readable'
else
echo 'The file does not exist or is not readable'
fi
# Output:
# 'The file exists and is readable'
In this example, we have a variable filename
set to ‘test.txt’. The if
statement checks if the file named ‘test.txt’ exists -e
and if it is readable -r
. If both conditions are true, the command echo 'The file exists and is readable'
is executed, and the output is ‘The file exists and is readable’. If either of the conditions is false, the else
clause is executed, and the output is ‘The file does not exist or is not readable’.
The advantage of this approach is that it allows us to check for multiple conditions before executing a command. This can make our scripts more robust and prevent errors. However, a potential pitfall is that if we don’t properly handle the case where one or both of the conditions are false, our script might not behave as expected. In the next section, we’ll discuss more complex uses of ‘if’ and ‘and’ in Bash scripting.
Expanding Your Toolkit: ‘If’, ‘And’, and ‘Or’
Now that we’ve covered the basics, let’s dive into more complex uses of ‘if’ and ‘and’ in Bash scripting. Specifically, we’ll explore how to use these operators in conjunction with the ‘or’ operator to create more complex conditional statements.
Let’s imagine a scenario where we want to check if a file is either readable or writable. We can use ‘if’, ‘and’, and ‘or’ to accomplish this.
filename='test.txt'
if [ -r $filename ] || [ -w $filename ]; then
echo 'The file is either readable or writable'
else
echo 'The file is neither readable nor writable'
fi
# Output:
# 'The file is either readable or writable'
In this script, we’re checking if the file named ‘test.txt’ is either readable -r
or writable -w
. The ||
operator represents ‘or’. If either condition is true, the command echo 'The file is either readable or writable'
is executed, and the output is ‘The file is either readable or writable’. If neither condition is true, the else
clause is executed, and the output is ‘The file is neither readable nor writable’.
This example demonstrates the power of combining ‘if’, ‘and’, and ‘or’ in Bash scripting. By understanding and using these operators, you can create complex conditional statements that allow your scripts to make more nuanced decisions.
Alternative Ways to Handle Conditional Logic in Bash
While ‘if’ and ‘and’ are powerful tools in Bash scripting, there are other ways to handle conditional logic that you might find useful in certain situations. Let’s explore two of these alternatives: ‘case’ statements and the ‘[[ ]]’ test operator.
Using ‘Case’ Statements in Bash
‘Case’ statements provide a way to perform different actions based on the value of a variable. Here’s an example:
day='Monday'
case $day in
'Monday') echo 'Start of the work week.' ;;
'Friday') echo 'End of the work week.' ;;
*) echo 'It is not Monday or Friday.' ;;
esac
# Output:
# 'Start of the work week.'
In this script, we’re using a ‘case’ statement to perform different actions based on the value of the day
variable. If day
is ‘Monday’, it echoes ‘Start of the work week.’. If day
is ‘Friday’, it echoes ‘End of the work week.’. For any other value, it echoes ‘It is not Monday or Friday.’.
‘Case’ statements can be a more readable alternative to ‘if’ and ‘and’ when dealing with multiple conditions. However, they are less flexible and can’t handle complex conditions as ‘if’ and ‘and’ can.
Using the ‘[[ ]]’ Test Operator in Bash
The ‘[[ ]]’ test operator is a more modern, flexible alternative to the ‘[ ]’ test used in our previous examples. Here’s an example of how you might use it:
var=15
if [[ $var -gt 10 && $var -lt 20 ]]; then
echo 'Var is between 10 and 20'
fi
# Output:
# 'Var is between 10 and 20'
The ‘[[ ]]’ test operator allows us to use ‘&&’ (and) and ‘||’ (or) directly within the test, without needing to use separate ‘[ ]’ tests. It also handles variables and strings more reliably than the ‘[ ]’ test.
However, ‘[[ ]]’ is not as portable as ‘[ ]’. It’s not supported by all Unix-like operating systems or older versions of Bash. Therefore, you should consider your target environment before deciding to use it.
Overcoming Challenges: Troubleshooting ‘If’ and ‘And’ in Bash
Using ‘if’ and ‘and’ in Bash scripting can sometimes lead to unexpected results or errors. Let’s discuss some common issues you might encounter and how to resolve them.
Uninitialized Variables
One common pitfall is trying to use an uninitialized variable in a condition. Let’s see what happens in such a case:
if [ $var -gt 10 ] && [ $var -lt 20 ]; then
echo 'Var is between 10 and 20'
fi
# Output:
# bash: [: -gt: unary operator expected
In this example, we didn’t set a value for var
. When the if
statement tries to evaluate the conditions, it doesn’t know how to compare $var
to 10 and 20, leading to a ‘unary operator expected’ error.
To prevent this, you should always ensure that your variables are initialized before using them in conditions. You could set a default value, or check if the variable is set using the ‘-v’ test:
if [ -v var ] && [ $var -gt 10 ] && [ $var -lt 20 ]; then
echo 'Var is between 10 and 20'
else
echo 'Var is not set or not between 10 and 20'
fi
# Output:
# 'Var is not set or not between 10 and 20'
Incorrect Use of Operators
Another common issue is using incorrect operators in your conditions. For example, using ‘&’ instead of ‘&&’ for the ‘and’ operator:
var=15
if [ $var -gt 10 ] & [ $var -lt 20 ]; then
echo 'Var is between 10 and 20'
fi
# Output:
# bash: [: missing `]'
# bash: [15: command not found
In this example, we used ‘&’ instead of ‘&&’. Bash interprets this as trying to run two commands in parallel, leading to errors. To fix this, always ensure you use the correct operators in your conditions.
Bash Scripting and Conditional Logic: The Foundation
Bash scripting is a powerful tool for automating tasks on Unix-like operating systems. It allows you to write scripts that can perform complex tasks, making your work more efficient and less error-prone.
One of the fundamental concepts in Bash scripting is conditional logic. This is the ability of a script to make decisions based on certain conditions. It’s like a traffic light: if the light is green, you go; if it’s red, you stop.
In Bash, conditional logic is implemented using various operators, with ‘if’ and ‘and’ being among the most commonly used. The ‘if’ operator allows you to specify a condition that must be met, and the ‘and’ operator allows you to specify multiple conditions that must all be met.
Here’s a simple example that demonstrates this concept:
var=15
if [ $var -gt 10 ] && [ $var -lt 20 ]; then
echo 'Var is greater than 10 and less than 20'
fi
# Output:
# 'Var is greater than 10 and less than 20'
In this script, we’re using ‘if’ and ‘and’ to check if var
is greater than 10 and less than 20. If both conditions are met, the script echoes ‘Var is greater than 10 and less than 20’.
Understanding and mastering conditional logic is crucial for writing effective Bash scripts. It allows you to create scripts that can adapt to different situations, making them more versatile and powerful. In the next section, we’ll explore how to apply these concepts in larger scripts or projects.
Expanding Your Bash Toolkit: ‘If’ and ‘And’ in Larger Projects
While our examples thus far have been relatively simple, the ‘if’ and ‘and’ operators play a crucial role in larger scripts or projects. They’re the building blocks for creating complex conditional logic, which is the heart of any dynamic script or program.
In larger projects, ‘if’ and ‘and’ often work hand-in-hand with other commands and functions to achieve more complex tasks. For instance, they can be used in conjunction with ‘for’ loops to perform certain actions on a series of items, or with ‘while’ loops to keep performing an action until a certain condition is met.
Here’s an example of how you might use ‘if’ and ‘and’ in a larger script:
for file in *.txt; do
if [ -r $file ] && [ -w $file ]; then
echo 'Processing' $file
# Add your processing commands here
else
echo 'Cannot process' $file
fi
done
# Output (example):
# 'Processing test1.txt'
# 'Processing test2.txt'
# 'Cannot process test3.txt'
In this script, we’re using a ‘for’ loop to iterate over all ‘.txt’ files in the current directory. For each file, we use ‘if’ and ‘and’ to check if the file is readable and writable. If it is, we echo ‘Processing’ and the filename, and then we would add our processing commands. If the file is not readable or writable, we echo ‘Cannot process’ and the filename.
Further Resources for Mastering Bash Scripting
For more in-depth information about Bash scripting and conditional logic, consider checking out the following resources:
- Advanced Bash-Scripting Guide: This is a comprehensive guide to Bash scripting that covers everything from the basics to more advanced topics.
GNU Bash Manual: This is the official manual for Bash. It’s a bit dense, but it’s a great resource if you want to understand how everything works under the hood.
Bash if && Statement: This guide from Diskinternals focuses on the “if &&” statement in Bash scripting.
Wrapping Up: Mastering ‘If’ and ‘And’ in Bash Scripting
In this comprehensive guide, we’ve explored the use of ‘if’ and ‘and’ operators in Bash scripting. These operators form the cornerstone of conditional logic in Bash, enabling your scripts to make decisions based on specific conditions.
We began with the basics, learning how to use ‘if’ and ‘and’ in a simple Bash script. We then delved into more advanced usage, exploring how to use these operators with other operators like ‘or’ to create more complex conditional statements.
Along the way, we also discussed common issues you might encounter when using ‘if’ and ‘and’ in Bash scripting, such as uninitialized variables and incorrect use of operators, providing you with solutions for each problem.
We also looked at alternative approaches to handling conditional logic in Bash, such as using ‘case’ statements and the ‘[[ ]]’ test operator. Here’s a quick comparison of these methods:
Method | Flexibility | Readability | Portability |
---|---|---|---|
‘If’ and ‘And’ | High | Moderate | High |
‘Case’ Statements | Low | High | High |
‘[[ ]]’ Test Operator | High | High | Low |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to use ‘if’ and ‘and’ in Bash scripting.
Mastering these operators will allow you to create more dynamic and versatile Bash scripts. Keep practicing and experimenting, and you’ll soon become a pro at handling conditional logic in Bash. Happy scripting!