Intro to Bash Script | Linux Shell Scripting Tutorial
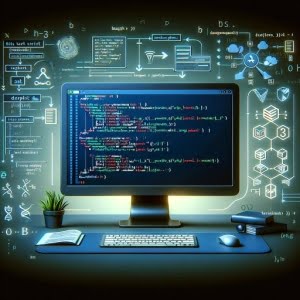
Are you finding it challenging to automate tasks on your Linux system? You’re not alone. Many system administrators and developers grapple with this task, but there’s a tool that can make this process a breeze.
Think of Bash scripting as a skilled puppeteer controlling a marionette. It’s a powerful tool that can help you control your system with ease, performing tasks automatically and efficiently. It’s like having an extra pair of hands that can work tirelessly, without ever making a mistake.
In this guide, we’ll introduce you to the world of Bash scripting. We’ll start from the basics and gradually move on to more advanced techniques. We’ll cover everything from writing your first Bash script, understanding its syntax and commands, to exploring more complex scripting techniques such as using variables, conditional statements, and loops.
So, let’s dive in and start mastering Bash scripting!
TL;DR: How Do I Write a Bash Script?
To write a bash script you will need to create a file, define the interpreter with
#!/bin/bash
, and define your commands, such asecho 'Hello, world!'
. You can then run the script by typingbash
followed by the .sh file name such as,bash helloWorld.sh
. A bash script is a text file containing a series of commands, writing one is as easy as writing a new text file.
Here’s a simple example:
#!/bin/bash
# This is a simple bash script
echo 'Hello, world!'
# Output:
# 'Hello, world!'
In this example, we create a bash script that prints ‘Hello, world!’ to the console. The #!/bin/bash
at the top of the script tells the system that this is a bash script. The echo
command is used to print the string ‘Hello, world!’ to the console.
This is a basic way to write a bash script, but there’s much more to learn about bash scripting. Continue reading for more detailed examples and advanced scripting techniques.
Table of Contents
- Writing Your First Bash Script
- Advanced Bash Scripting Techniques
- Exploring Other Scripting Languages and Tools
- Common Bash Scripting Errors and Solutions
- Bash Scripting Best Practices and Optimization
- Understanding the Bash Shell
- The Role of Scripting in System Administration
- Expanding Your Shell Scripting Horizons
- Wrapping Up: Mastering Bash Scripting
Writing Your First Bash Script
The Foundation of a Bash Script
A bash script is a plain text file which contains a series of commands. These commands are a sequence of orders such as reading, writing, or manipulating file data. They are executed by the bash shell and are stored in a file. The bash shell reads this file and carries out the commands as if they have been entered directly on the command line.
Here’s an example of a basic bash script:
#!/bin/bash
# This is a comment
echo 'This is our first bash script'
# Output:
# 'This is our first bash script'
In this script, #!/bin/bash
is a special directive which tells the system that this is a bash shell script. The #
symbol is used to add comments in the script. The echo
command is used to output the string ‘This is our first bash script’ to the console.
Understanding Bash Script Syntax
The syntax of a bash script is very important. A single misplaced symbol or an incorrect command can lead to errors. Therefore, understanding the syntax is crucial for writing a bash script.
Here’s an example of bash script syntax:
#!/bin/bash
# Declare variable
GREETING='Hello'
# Use variable
echo $GREETING, world!
# Output:
# 'Hello, world!'
In this script, we declare a variable GREETING
and assign the value ‘Hello’ to it. Then we use this variable in our echo
command to print ‘Hello, world!’ to the console. The $
symbol is used to access the value of the variable.
This is a basic introduction to bash scripting. In the next sections, we’ll delve into more advanced techniques and explore the full potential of bash scripting.
Advanced Bash Scripting Techniques
Utilizing Variables
Variables are an essential part of any programming language or scripting language. They allow us to store data that we can use later. Here’s an example of using variables in a bash script:
#!/bin/bash
# Declare a variable
NAME='John'
# Use the variable
echo "Hello, $NAME"
# Output:
# 'Hello, John'
In this script, we declare a variable NAME
and assign the value ‘John’ to it. We then use this variable in our echo
command to print ‘Hello, John’ to the console.
Working with Conditional Statements
Conditional statements allow us to make decisions in our bash scripts. We can execute different parts of the script based on certain conditions. Here’s an example of using a conditional statement in a bash script:
#!/bin/bash
# Declare a variable
AGE=20
# Use a conditional statement
if [ $AGE -gt 18 ]
then
echo 'You are old enough to vote'
else
echo 'You are not old enough to vote'
fi
# Output:
# 'You are old enough to vote'
In this script, we declare a variable AGE
and assign the value 20 to it. We then use a conditional statement to check if the age is greater than 18. If the condition is true, we print ‘You are old enough to vote’ to the console. If the condition is false, we print ‘You are not old enough to vote’.
Exploring Loops
Loops allow us to execute a block of code multiple times. This can be extremely useful for automating repetitive tasks. Here’s an example of using a loop in a bash script:
#!/bin/bash
# Use a loop
for i in {1..5}
do
echo "Number: $i"
done
# Output:
# 'Number: 1'
# 'Number: 2'
# 'Number: 3'
# 'Number: 4'
# 'Number: 5'
In this script, we use a ‘for’ loop to print the numbers 1 to 5 to the console. The loop iterates over the numbers 1 to 5, and for each iteration, it prints the current number to the console.
Exploring Other Scripting Languages and Tools
While Bash scripting is a powerful tool for automating tasks on your Linux system, it’s not the only tool available. There are other scripting languages and tools, such as Python and Perl, which can also be used for similar tasks. Let’s explore these alternatives and compare them with Bash scripting.
Python: A Versatile Language
Python is a high-level, interpreted programming language that is known for its readability and simplicity. It’s a versatile language that can be used for a wide range of tasks, from web development to data analysis.
Here’s an example of a Python script that does the same job as our previous Bash script:
# Declare a variable
name = 'John'
# Use the variable
print(f'Hello, {name}')
# Output:
# 'Hello, John'
In this script, we declare a variable name
and assign the value ‘John’ to it. We then use this variable in our print
function to print ‘Hello, John’ to the console.
Python has a more intuitive syntax than Bash and is easier to learn for beginners. However, for simple tasks like file manipulation or running system commands, Bash might be more efficient.
Perl: A Powerful Scripting Language
Perl is another powerful scripting language that is often used for text manipulation. It’s a bit more complex than Bash or Python, but it offers more advanced features and is very efficient for certain tasks.
Here’s an example of a Perl script that does the same job as our previous Bash script:
#!/usr/bin/perl
# Declare a variable
my $name = 'John';
# Use the variable
print "Hello, $name
";
# Output:
# 'Hello, John'
In this script, we declare a variable name
and assign the value ‘John’ to it. We then use this variable in our print
function to print ‘Hello, John’ to the console.
Perl is a bit more complex to learn, but it’s very powerful and efficient for text manipulation tasks. However, for simple system administration tasks, Bash might be more suitable.
In conclusion, while Bash scripting is a very useful tool for automating tasks on your Linux system, there are other alternatives available. The best tool to use depends on the specific task and your personal preference.
Common Bash Scripting Errors and Solutions
Missing Shebang
One of the most common errors in bash scripting is forgetting to include the shebang (#!/bin/bash
) at the top of the script. The shebang tells the system that this is a bash script and should be executed with the bash shell.
Here’s an example of an error caused by a missing shebang:
# Missing shebang
echo 'Hello, world!'
# Output:
# Command 'echo' not found
In this script, we forgot to include the shebang. As a result, the system doesn’t know that this is a bash script and fails to execute the echo
command.
Unmatched Quotes
Another common error is unmatched quotes. If you open a quote but don’t close it, the bash shell will get confused and throw an error.
Here’s an example of an error caused by unmatched quotes:
#!/bin/bash
# Unmatched quotes
echo 'Hello, world!
# Output:
# Unexpected EOF while looking for matching `''
In this script, we opened a single quote but didn’t close it. As a result, the bash shell throws an error.
Bash Scripting Best Practices and Optimization
Use Comments
Comments are a great way to document your code and make it easier to understand. You can add a comment in a bash script by using the #
symbol.
Check for Errors
Always check your scripts for errors before running them. You can use the bash -n
command to check a script for syntax errors without running it.
Use Functions
Functions can help you organize your code and make it more readable. They also allow you to reuse code, which can make your scripts more efficient.
Optimize for Performance
Bash scripts can be slow if they’re not optimized. Try to minimize the use of external commands, as they can slow down your script. Also, use loops sparingly, as they can be resource-intensive.
Understanding the Bash Shell
The Bash shell is a command-line interpreter that allows you to interact with your operating system. It’s the default shell for most Linux distributions and is also available on other Unix-like systems and Windows.
When you type a command into the Bash shell, it interprets that command and communicates with the operating system to execute it. For example, when you type the ls
command, the Bash shell interprets this command and displays the list of files and directories in the current directory.
# Example of using the ls command
ls
# Output:
# 'file1.txt' 'file2.txt' 'directory1'
In this example, we use the ls
command to list the contents of the current directory. The Bash shell interprets this command and displays the list of files and directories.
The Role of Scripting in System Administration
Scripting plays a crucial role in system administration and automation. Scripts are used to automate repetitive tasks, like creating multiple users, updating system configurations, or backing up data. This automation saves time and reduces the chance of errors.
For example, a system administrator might write a bash script to backup certain files every night at midnight. This script would use commands to copy the files to a backup location, and it would be scheduled to run automatically using a tool like cron.
#!/bin/bash
# This is a simple backup script
cp /path/to/file /path/to/backup
# Output:
# No output if the command is successful
In this script, we use the cp
command to copy a file to a backup location. This script could be scheduled to run at a specific time using a tool like cron, automating the backup process.
In conclusion, the Bash shell is a powerful tool for interacting with your operating system, and scripting is a crucial skill for system administration and automation. By mastering Bash scripting, you can automate tasks, save time, and reduce the chance of errors.
Expanding Your Shell Scripting Horizons
The World of Shell Scripting
Bash scripting is just one facet of the larger world of shell scripting. Shell scripting encompasses various scripting languages used to automate tasks in different shell environments, not just Bash. Other shells include the Korn shell (ksh), the C shell (csh), and the Z shell (zsh), each with their own scripting languages.
While the syntax and features may vary, the fundamental concept remains the same: automating tasks to save time and reduce errors. Whether it’s automating system administration tasks, data analysis, or web scraping, shell scripting is a vital skill in the toolbox of any programmer or system administrator.
Applications in Task Automation and System Administration
Shell scripting shines in task automation and system administration. Imagine having to perform system updates on hundreds of servers manually, or analyzing massive amounts of data by hand. These tasks would be time-consuming and error-prone. With shell scripting, these tasks can be automated, saving time and ensuring accuracy.
Here’s an example of a bash script that automates the process of updating a Linux system:
#!/bin/bash
# Update the system
sudo apt-get update -y && sudo apt-get upgrade -y
# Output:
# 'Reading package lists... Done'
# 'Building dependency tree... Done'
# 'Reading state information... Done'
# 'Calculating upgrade... Done'
# '0 upgraded, 0 newly installed, 0 to remove and 0 not upgraded.'
In this script, we use the apt-get
command to update the system and upgrade all installed packages. This script could be scheduled to run at a specific time, ensuring that the system is always up to date.
Further Resources for Bash Scripting Proficiency
If you’re interested in diving deeper into the world of Bash scripting, here are a few resources that can help you on your journey:
- GNU Bash Manual: This is the official manual for Bash. It’s a bit dense, but it’s a comprehensive resource for all things Bash.
DevHints – Bash Cheat Sheet: This handy cheat sheet provides a quick reference for various Bash commands, syntax, and concepts.
- Linux Command: This website offers a wealth of information on Linux commands and shell scripting. It’s a great resource for beginners and experienced users alike.
Wrapping Up: Mastering Bash Scripting
In this comprehensive guide, we’ve journeyed through the dynamic world of Bash scripting, an essential tool for automating tasks on your Linux system.
We began with the basics, learning how to write our first Bash script and understanding the syntax. We then ventured into more advanced territory, exploring techniques such as using variables, conditional statements, and loops. We also delved into common challenges you might face when writing Bash scripts, offering solutions to help you overcome these obstacles.
Along the way, we looked at alternative approaches to scripting, comparing Bash with other scripting languages like Python and Perl. Here’s a quick comparison of these languages:
Language | Ease of Use | Versatility | Best Used For |
---|---|---|---|
Bash | High | Moderate | System administration tasks |
Python | High | High | Wide range of applications |
Perl | Moderate | High | Text manipulation tasks |
Whether you’re just starting out with Bash scripting or looking to level up your skills, we hope this guide has given you a deeper understanding of Bash scripting and its capabilities.
With its balance of ease of use and versatility, Bash scripting is a powerful tool for automating tasks on your Linux system. Now, you’re well equipped to harness the power of Bash scripting. Happy coding!