Bash $? Special Variable: Command Exit Status Guide
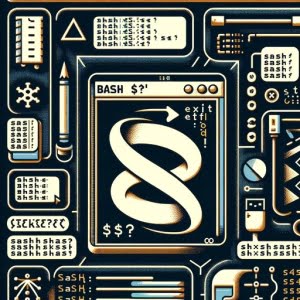
Have you ever stumbled upon the $? variable in bash and wondered what it does? You’re not alone. Many developers encounter this special variable but don’t fully understand its purpose or how to use it effectively.
Think of the $? variable as a diligent auditor. It keeps track of the exit status of the last command executed in bash, providing valuable feedback about your script’s operations.
This guide will take you through the ins and outs of using the $? variable in bash. We’ll start with the basics, then dive into more advanced uses, and even explore alternative approaches. We’ll also discuss common issues and their solutions, and provide practical examples along the way.
So, let’s get started and master the $? variable in bash!
TL;DR: What Does $? Mean in Bash?
In bash,
$?
is a special variable that holds theexit status of the last command executed
. It can be used with the syntax,echo $?
. It’s a way for the system to communicate whether the last operation was successful or not.
Here’s a simple example:
echo Hello
echo $?
# Output:
# Hello
# 0
In this example, we first execute the echo Hello
command. Then, we use echo $?
to display the exit status of the last command. The output 0
indicates that the previous command (echo Hello
) executed successfully without any errors.
This is just a basic introduction to the $? variable in bash. If you want to understand more about $? and how to use it effectively in various scenarios, keep reading. We’ll delve into more complex uses, alternative approaches, troubleshooting, and much more.
Table of Contents
Getting Started with Bash $?
In bash, the $? variable is a special variable that provides the exit status of the last command executed. The exit status, also known as a return status, is a numerical value returned by a command upon its completion.
The key thing to remember is that an exit status of 0
indicates that the command was successful. Any non-zero status typically signals an error or abnormal termination.
Let’s take a look at an example:
ls /home
echo $?
# Output:
# Documents Downloads Pictures Videos
# 0
In this example, we first execute the ls /home
command, which lists the contents of the /home directory. Then, we use echo $?
to display the exit status of the ls
command. The output 0
indicates that the ls
command executed successfully.
Now, let’s look at an example where the command fails:
ls /nonexistentdirectory
echo $?
# Output:
# ls: cannot access '/nonexistentdirectory': No such file or directory
# 2
This time, we’re trying to list the contents of a directory that doesn’t exist. The ls
command fails and returns an exit status of 2
, which echo $?
then displays. This non-zero exit status indicates an error occurred.
Understanding the $? variable and the concept of exit statuses is fundamental in bash scripting. It allows you to handle errors and control the flow of your scripts effectively.
Advanced Usage of Bash $?
As you get more comfortable with the $? variable, you can start using it in more complex scenarios. For instance, it can be used in conditional statements or loops to control the flow of your script based on the success or failure of commands.
Conditional Execution with Bash $?
Let’s say you have a script that needs to execute a command, and based on whether that command is successful or not, it should perform different actions. Here’s how you can use the $? variable in this case:
ls /home
if [ $? -eq 0 ]; then
echo 'Command succeeded'
else
echo 'Command failed'
fi
# Output (if /home exists):
# Documents Downloads Pictures Videos
# Command succeeded
In this example, we first execute the ls /home
command. Then, we use a conditional if
statement to check the exit status of the ls
command. If the command was successful (i.e., $? equals 0), it prints ‘Command succeeded’. Otherwise, it prints ‘Command failed’.
Using Bash $? in Loops
Another common use case for the $? variable is within loops. Let’s say you have a list of directories and you want to check if they exist:
directories=( '/home' '/nonexistentdirectory' '/var' )
for dir in "${directories[@]}"; do
ls $dir > /dev/null 2>&1
if [ $? -eq 0 ]; then
echo "$dir exists"
else
echo "$dir does not exist"
fi
done
# Output:
# /home exists
# /nonexistentdirectory does not exist
# /var exists
In this script, we have an array of directories. We loop over this array, and for each directory, we try to list its contents with the ls
command. We redirect the standard output and error of the ls
command to /dev/null
to suppress them. Then, we use the $? variable to check if the ls
command was successful. If it was, we print that the directory exists. Otherwise, we print that it does not exist.
These examples demonstrate how the $? variable can be used in more complex scenarios to control the flow of your scripts based on the success or failure of commands.
Exploring Alternatives to Bash $?
While the $? variable is a powerful tool for checking the exit status of commands in Bash, it’s not the only way. There are alternative approaches you can use, such as the ‘set -e’ option.
Using ‘set -e’ in Bash Scripts
The ‘set -e’ option in bash scripts causes the script to exit immediately if any command exits with a non-zero status. This can be particularly useful in scripts where you want to ensure all commands execute successfully, and if not, stop the script immediately to prevent further errors.
Here’s an example:
set -e
ls /home
ls /nonexistentdirectory
# Output:
# ls: cannot access '/nonexistentdirectory': No such file or directory
In this script, we first enable the ‘set -e’ option. Then, we try to list the contents of the /home directory (which likely exists) and a directory that does not exist. The ‘ls /nonexistentdirectory’ command fails, and because ‘set -e’ is enabled, the script exits immediately without continuing to the next command.
Benefits and Drawbacks of ‘set -e’
The ‘set -e’ option can be a powerful tool, but it’s not always the best choice. It’s important to understand its benefits and drawbacks to make an informed decision.
Benefits:
- It simplifies error handling in your scripts. You don’t need to check the exit status after every command; the script will stop automatically if a command fails.
It can help to prevent further errors. If a command fails, it might lead to unexpected results in subsequent commands. By stopping the script immediately, ‘set -e’ can prevent these problems.
Drawbacks:
- It can make debugging more difficult. If your script stops at the first error, it might be harder to understand the context of the error and how it affects subsequent commands.
It’s not suitable for all scripts. In some cases, you might want your script to continue even if a command fails. For example, if you’re running cleanup commands at the end of your script, you might want them to run regardless of whether previous commands were successful.
In conclusion, while the $? variable is a common and flexible way to check the exit status of commands in Bash, it’s not the only approach. Depending on your specific needs and the complexity of your scripts, alternatives like ‘set -e’ might be more suitable. Always consider the specific requirements of your script and the trade-offs of different approaches when deciding which to use.
Troubleshooting Common Issues with Bash $?
While the $? variable is a powerful tool in bash scripting, it’s not without its quirks. Let’s discuss some common issues you might encounter when using it and how to solve them.
Issue: Overwriting the Exit Status
One common pitfall is overwriting the exit status before you’ve had a chance to check it. Remember, $? gives the exit status of the last command executed. If you run another command before checking $?, you’ll lose the exit status you were interested in.
ls /nonexistentdirectory
echo 'Hello'
echo $?
# Output:
# ls: cannot access '/nonexistentdirectory': No such file or directory
# Hello
# 0
In this example, we’re trying to list the contents of a directory that doesn’t exist. However, before we check the exit status of the ls
command, we execute another command (echo 'Hello'
). As a result, when we check $?, it shows the exit status of the echo
command, not the ls
command.
Solution: Always check $? immediately after the command you’re interested in.
Issue: Misunderstanding Exit Statuses
Another common issue is misunderstanding what different exit statuses mean. While an exit status of 0 indicates success, different non-zero exit statuses can indicate different types of errors.
ls /nonexistentdirectory
echo $?
# Output:
# ls: cannot access '/nonexistentdirectory': No such file or directory
# 2
In this example, the ls
command fails because the directory does not exist, and it returns an exit status of 2. However, not all non-zero exit statuses mean the same thing. You’ll need to check the documentation for the specific command to understand what different exit statuses mean.
Solution: Always refer to the command’s documentation to understand what different exit statuses mean.
By being aware of these common issues and how to solve them, you can use the $? variable more effectively in your bash scripts.
Digging Deeper: The Importance of Exit Statuses
Exit statuses, or return codes, are a fundamental concept in bash scripting and programming in general. They provide a way for a command, program, or script to communicate its outcome to the calling entity.
Why are Exit Statuses Important?
The $? variable in bash holds the exit status of the last command executed. It’s a form of communication between the command and the script. By checking this variable, your script can determine whether a command was successful or not, and make decisions accordingly.
For example, if a script is supposed to process a file, but the file does not exist, the command to open the file will fail, and the exit status will indicate this failure. The script can then decide to terminate, skip to the next file, or take any other appropriate action.
Exit Statuses in Other Programming Languages
The concept of exit statuses is not unique to bash or even to shell scripting. Many programming languages have similar concepts, although they might be implemented differently or called by different names.
For instance, in Python, a script can call the sys.exit()
function to terminate the script and return an exit status to the calling process. Here’s an example:
import sys
try:
# Try to open a nonexistent file
with open('nonexistentfile.txt') as f:
content = f.read()
except FileNotFoundError:
print('File not found')
sys.exit(1)
# Output:
# File not found
In this Python script, we try to open a file that does not exist. When the FileNotFoundError
is raised, we print an error message and call sys.exit(1)
to terminate the script and return an exit status of 1.
Just like in bash, the exit status can then be checked by the calling process to determine whether the script was successful.
In conclusion, exit statuses are a fundamental concept in programming that allow commands, scripts, and programs to communicate their outcome. Understanding this concept and how to use the $? variable effectively is crucial for writing robust and reliable bash scripts.
Expanding the Use of Bash $?
The $? variable in bash is not just for simple scripts. In larger projects and more complex scripts, it can play a crucial role in error handling and control flow.
Integrating $? in Larger Scripts
In larger scripts, you might have multiple commands whose success or failure can influence the script’s flow. The $? variable allows you to check the exit status of each command and take appropriate action.
For instance, you might have a script that processes multiple files. If opening a file fails, you could use the $? variable to skip that file and continue with the next one.
files=( 'file1.txt' 'nonexistentfile.txt' 'file2.txt' )
for file in "${files[@]}"; do
cat $file > /dev/null 2>&1
if [ $? -eq 0 ]; then
echo "Processing $file"
# Add file processing commands here
else
echo "Skipping $file"
fi
done
# Output:
# Processing file1.txt
# Skipping nonexistentfile.txt
# Processing file2.txt
In this script, we loop over an array of files. For each file, we try to display its contents with the cat
command. We then check the $? variable to see if the cat
command was successful. If it was, we proceed to process the file. If it wasn’t (because the file does not exist), we skip the file and continue with the next one.
Further Resources for Bash Scripting Mastery
If you’re interested in learning more about bash scripting and how to use special variables like $?, here are some resources that might help:
- GNU Bash Reference Manual: This is the official reference manual for bash. It’s quite comprehensive and covers everything from basic to advanced topics.
Advanced Bash-Scripting Guide: This is a free book that covers almost every aspect of bash scripting. It’s a great resource for beginners and experienced bash scripters alike.
TecAdmin’s Guide on Bash Special Variables – This guide provides a comprehensive overview of Bash special variables, such as
$0
,$*
, and more.
Wrapping Up: Unraveling the Mystery of Bash $?
In this comprehensive guide, we’ve delved into the depths of the $? variable in bash, a special variable that holds the exit status of the last command executed.
We started with the basics, learning how to use the $? variable in simple scenarios and understanding the concept of exit statuses. We then moved on to more advanced uses, such as using $? in conditional statements and loops, and even explored alternative approaches, like the ‘set -e’ option.
Along the way, we tackled common issues that you might encounter when using the $? variable and provided solutions for each one. We also took a step back to understand the importance of exit statuses in programming and how they are used in other languages.
Method | Pros | Cons |
---|---|---|
Using $? | Provides exact exit status, versatile in use | Can be overwritten unintentionally |
Using ‘set -e’ | Automatically exits script on error | Can make debugging difficult |
Whether you’re a beginner just starting out with bash scripting, or an experienced developer looking to deepen your understanding, we hope this guide has shed light on the $? variable and its many uses.
Mastering the $? variable is an important step in becoming proficient in bash scripting. It allows you to control the flow of your scripts, handle errors effectively, and write more robust and reliable code. Happy scripting!