bin bash m bad interpreter no such file or directory
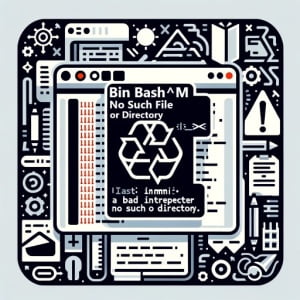
Ever found yourself stuck with the '/bin/bash^M: bad interpreter: No such file or directory'
error while executing your shell script? It’s like a misdialed phone number, your script is trying to reach an interpreter that doesn’t exist or isn’t where it’s supposed to be. Many developers find themselves in this predicament, but we’re here to help.
Think of the shell script’s shebang line as a dialing code – it’s the way your script connects to the interpreter it needs to run. If it’s incorrect or points to a non-existent location, you’ll encounter the ‘bin bash m bad interpreter’ error.
This guide will walk you through the steps to resolve this common shell scripting issue. We’ll explore the root cause of the error, delve into basic and advanced solutions, and even discuss alternative approaches and troubleshooting.
So, let’s dive in and start tackling the ‘/bin/bash^M: bad interpreter: No such file or directory’ error!
TL;DR: Solving the ‘bad interpreter: No such file or directory’ Error
This error can be solved by ensuring that the shebang (
#!
) points to the correct location of the bash interpreter, for example,#!/bin/bash
. The error usually happens when the shebang (#!
) in your script points to a non-existent or wrongly located shell interpreter.
Here’s a simple example:
#!/bin/bash
# This is a simple script
echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this example, we’ve used the correct shebang line #!/bin/bash
at the start of our script. This tells the system to use the bash interpreter located at /bin/bash
to execute the script. The script then prints ‘Hello, World!’ to the console.
But there’s much more to shell scripting and handling different errors that might come up. Continue reading for a more detailed explanation and advanced solutions.
Table of Contents
- Locating the Bash Interpreter and Correcting the Shebang Line
- Making Shell Scripts Portable Across Systems
- Exploring Beyond the Shebang Line: Other Potential Causes
- Navigating Common Shell Scripting Issues
- Understanding Shell Scripting and the Role of the Shebang Line
- Writing Robust and Portable Shell Scripts
- Concluding Thoughts: Decoding the ‘No such file or directory’ Error
Locating the Bash Interpreter and Correcting the Shebang Line
Let’s start with the basics. If you’re new to shell scripting, the ‘/bin/bash^M: bad interpreter: No such file or directory’ error might seem daunting. But, don’t worry! It’s usually a simple fix. This error typically means that the shebang line in your script is pointing to a non-existent or wrongly located bash interpreter.
The shebang line is the first line in your script and it looks like this: #!
. It tells the system which interpreter to use to execute the script. If the shebang line is incorrect, you’ll get the ‘bin bash m bad interpreter’ error.
So, how do we fix this? First, we need to find out where the bash interpreter is located in your system. You can do this by running the following command in your terminal:
which bash
# Output:
# /bin/bash
The which bash
command will return the path to the bash interpreter, usually /bin/bash
. This is the path you should use in your shebang line.
Let’s say you have a script that’s giving you the ‘bin bash m bad interpreter’ error. It might look something like this:
#!/bin/bash/m
echo 'Hello, World!'
# Output:
# '/bin/bash^M: bad interpreter: No such file or directory'
Here, the shebang line is incorrect because it includes an extra /m
at the end. To correct it, you should change the shebang line to point to the correct bash interpreter location. Here’s the corrected script:
#!/bin/bash
echo 'Hello, World!'
# Output:
# 'Hello, World!'
Now, when you run the script, it should print ‘Hello, World!’ to the console without any errors. That’s it! You’ve just resolved the ‘/bin/bash^M: bad interpreter: No such file or directory’ error.
Making Shell Scripts Portable Across Systems
As you delve deeper into shell scripting, you’ll realize that not all Unix-like systems are the same. The location of the bash interpreter can vary across systems, which can cause the ‘/bin/bash^M: bad interpreter: No such file or directory’ error when you try to run your script on a different system. This is where portability comes into play.
Portability refers to the ability of your script to run correctly on different systems without needing modifications. To make your shell scripts portable, you can use env
in your shebang line. The env
command is used to run a program in a modified environment, and in this case, it helps locate the bash interpreter irrespective of its location on the system.
Here’s how you can modify the shebang line to use env
:
#!/usr/bin/env bash
echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this script, the shebang line #!/usr/bin/env bash
tells the system to use env
to locate the bash interpreter. The env
command will search the system’s PATH
for the bash interpreter and use it to execute the script. This way, even if the bash interpreter is located in a different place on another system, the script will still run correctly.
Now, your script is more robust and can handle different Unix-like systems. You’ve taken a significant step in preventing the ‘/bin/bash^M: bad interpreter: No such file or directory’ error and making your shell scripts more portable.
Exploring Beyond the Shebang Line: Other Potential Causes
While the shebang line is often the culprit of the ‘/bin/bash^M: bad interpreter: No such file or directory’ error, it’s not always the case. More advanced shell scripting scenarios can lead to this error due to other factors. Let’s explore some of these scenarios and how you can address them.
File Permissions and Their Impact
File permissions can prevent your script from being executed, leading to errors. If your script doesn’t have execute permissions, you’ll need to grant them using the chmod
command.
chmod +x your_script.sh
This command gives execute (x
) permissions to the script, allowing it to run.
Line Endings: A Tale of Different Operating Systems
Transferring scripts between different operating systems (like Windows and Linux) can introduce invisible characters into your script, such as carriage returns (). These characters can cause the ‘/bin/bash^M: bad interpreter: No such file or directory’ error.
You can use the dos2unix
command to remove these characters and fix your script:
sudo apt-get install dos2unix # Install dos2unix
dos2unix your_script.sh
This command converts the line endings in your script from Windows format to Unix format, resolving the error.
Text Editor Issues and Their Workarounds
Sometimes, the text editor you use to write your script could be the problem. Some text editors add invisible characters or use a format that’s not compatible with Unix-like systems.
To avoid this, you can use text editors that are designed for coding, like Vim, Nano, or Visual Studio Code. These editors won’t add any extra characters that could cause the ‘/bin/bash^M: bad interpreter: No such file or directory’ error.
By understanding these alternative causes and their solutions, you can write robust scripts that are less likely to encounter errors. And even if they do, you’ll be well-equipped to troubleshoot and fix them.
As you continue your journey with shell scripting, you’ll likely encounter various issues. Understanding these common problems and knowing how to troubleshoot them is crucial. Here, we’ll discuss some frequently seen issues and provide tips on how to avoid them in the future.
The Invisible Character Trap
Invisible characters can often sneak into your scripts and cause unexpected errors. These can be introduced when copying and pasting code or when using certain text editors. They can be tricky to spot, but tools like cat -v
can help. This command displays non-printing characters, making it easier to identify any that may be causing issues.
cat -v your_script.sh
# Output:
# 'Hello, World!^M'
In this example, ^M
is a carriage return character that’s not visible in most editors. Removing these can often resolve script errors.
The Path Variable Pitfall
The PATH
variable is crucial in Unix-like systems. It tells the system where to look for executable files. If your PATH
doesn’t include the location of the bash interpreter, you’ll encounter the ‘/bin/bash^M: bad interpreter: No such file or directory’ error. You can check your PATH
with the echo
command:
echo $PATH
# Output:
# /usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
Here, the PATH
includes /bin
, which is where the bash interpreter is usually located. If /bin
is missing from your PATH
, you’ll need to add it.
The Shebang Line Slip
As we’ve discussed, the shebang line is essential in shell scripts. However, it’s easy to mistype or forget it, leading to errors. Always double-check the shebang line in your scripts, and remember to use #!/usr/bin/env bash
for portability.
By keeping these troubleshooting tips in mind and understanding the common issues, you’ll be better prepared to tackle any problems that come your way in your shell scripting journey.
Understanding Shell Scripting and the Role of the Shebang Line
Shell scripting is a powerful tool for automating tasks on Unix-like systems. It involves writing scripts, which are essentially lists of commands that the shell can execute.
The Shebang Line: A Crucial First Step
Every shell script begins with a shebang line, denoted by #!
. This line tells the system which interpreter to use to execute the script. The shebang line is followed by the path to the intended interpreter. For instance, #!/bin/bash
indicates that the script should be run using the bash interpreter located at /bin/bash
.
Here’s a simple example of a shell script:
#!/bin/bash
echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this script, the shebang line is #!/bin/bash
, which specifies the bash interpreter. The echo
command is then used to print ‘Hello, World!’ to the console.
The Bash Interpreter: The Power Behind Your Scripts
The bash interpreter, or Bourne Again SHell, is a popular shell used in many Unix-like systems. It’s responsible for executing your shell scripts, interpreting the commands, and returning the results.
Different systems may have different default shell interpreters, or the same interpreter may be located in different paths. This is why it’s crucial to correctly specify the interpreter in the shebang line to prevent errors like ‘/bin/bash^M: bad interpreter: No such file or directory’.
By understanding these fundamentals, you’ll be better equipped to write effective shell scripts and troubleshoot any issues that arise.
Writing Robust and Portable Shell Scripts
As you continue to refine your shell scripting skills, you’ll want to focus on writing scripts that are not only functional but also robust and portable. This means your scripts should be able to handle a variety of scenarios and work across different Unix-like systems.
Shell Script Debugging: A Key Skill
One of the most crucial skills for a shell script developer is debugging. It involves identifying and fixing errors or ‘bugs’ in your scripts. You can use the -v
(verbose) and -x
(xtrace) options with the bash command to help debug your scripts.
bash -v your_script.sh
bash -x your_script.sh
These commands print each command to the terminal before it’s executed, helping you trace the flow of execution and identify any errors.
Advanced Bash Scripting Techniques
As you progress, you’ll want to learn advanced techniques like using functions, loops, and conditionals in your scripts. These can significantly enhance your scripts’ capabilities and efficiency.
#!/bin/bash
# A simple function
greet() {
echo 'Hello, '$1'!'
}
# Call the function with 'World' as the argument
greet 'World'
# Output:
# 'Hello, World!'
In this script, we’ve defined a function greet
that takes one argument and prints a greeting message. We then call this function with ‘World’ as the argument.
Exploring Other Shell Interpreters
While bash is widely used, there are other shell interpreters like sh, ksh, and zsh that you might find useful. Each has its own features and syntax, and understanding these can help you write more versatile scripts.
#!/bin/sh
echo 'Hello, World!'
# Output:
# 'Hello, World!'
This script uses the sh interpreter, indicated by the shebang line #!/bin/sh
. The script then prints ‘Hello, World!’ to the console.
Further Resources for Shell Scripting Proficiency
To continue your learning journey, you might find these resources helpful:
- GNU Bash Reference Manual: This is the official reference for the bash shell and a great resource for understanding its features and syntax.
Advanced Bash-Scripting Guide: This guide covers advanced topics in bash scripting, including functions, loops, and error handling.
Unix Shell Scripting Tutorial: This tutorial covers shell scripting basics and includes examples and exercises to practice what you’ve learned.
By exploring these resources and practicing your skills, you’ll be well on your way to becoming a proficient shell script developer.
Concluding Thoughts: Decoding the ‘No such file or directory’ Error
In this comprehensive guide, we’ve demystified the ‘/bin/bash^M: bad interpreter: No such file or directory’ error, a common stumbling block in Unix-like systems. We’ve explored its causes, delved into solutions, and shared best practices in shell scripting to avoid such issues in the future.
We began with the basics, understanding the error and how it stems from an incorrectly set shebang line. We learned how to locate the bash interpreter in your system and adjust the shebang line accordingly, thus resolving the error for beginners.
From there, we ventured into more advanced territory, discussing the importance of script portability. We saw how the shebang line could be modified to ensure scripts run correctly across different systems, thus avoiding the ‘bin bash m bad interpreter’ error.
We didn’t stop there; we explored alternative causes of the error, such as file permissions, line endings, and text editor issues, providing solutions for each. This knowledge equips you to handle a variety of scenarios that could lead to this error.
Approach | Pros | Cons |
---|---|---|
Correcting the Shebang Line | Simple and effective for most scripts | Doesn’t account for portability |
Using env in the Shebang Line | Ensures script portability | Slightly more complex |
Understanding Alternative Causes | Helps troubleshoot more complex scenarios | Requires a deeper understanding of shell scripting |
Whether you’re a beginner just starting out with shell scripting or an intermediate user looking to level up, we hope this guide has given you a deeper understanding of the ‘/bin/bash^M: bad interpreter: No such file or directory’ error and how to resolve it.
Shell scripting is a powerful tool, and understanding how to avoid common errors like this one is a crucial part of becoming proficient. With the knowledge you’ve gained from this guide, you’re well on your way to becoming a shell scripting expert. Happy scripting!